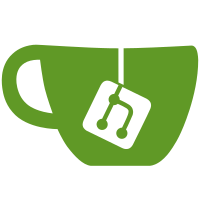
42 changed files with 2858 additions and 1464 deletions
@ -0,0 +1,38 @@
|
||||
package com.hnac.hzinfo.inspect.areamonthly.feign; |
||||
|
||||
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||
import com.hnac.hzinfo.inspect.areamonthly.feign.fallback.TaskFeignClientFallback; |
||||
import com.hnac.hzinfo.inspect.areamonthly.vo.TaskListQuery; |
||||
import com.hnac.hzinfo.inspect.areamonthly.vo.TaskVo; |
||||
import com.hnac.hzinfo.inspect.task.entity.TaskEntity; |
||||
import io.swagger.annotations.ApiOperation; |
||||
import org.springblade.core.mp.support.BladePage; |
||||
import org.springblade.core.mp.support.Query; |
||||
import org.springblade.core.tool.api.R; |
||||
import org.springframework.cloud.openfeign.FeignClient; |
||||
import org.springframework.web.bind.annotation.GetMapping; |
||||
import org.springframework.web.bind.annotation.RequestMapping; |
||||
import org.springframework.web.bind.annotation.RequestMethod; |
||||
import org.springframework.web.bind.annotation.RequestParam; |
||||
|
||||
import static com.hnac.hzinfo.inspect.Constants.APP_NAME; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 17:21 |
||||
*/ |
||||
@FeignClient(value = APP_NAME, fallback = TaskFeignClientFallback.class) |
||||
public interface TaskFeignClient { |
||||
|
||||
|
||||
@GetMapping("/task/list") |
||||
@ApiOperation(value = "分页显示任务列表", notes = "传入task") |
||||
public R<BladePage<TaskVo>> list(TaskListQuery task, Query query); |
||||
|
||||
|
||||
@RequestMapping(value = "/task/detail", method = {RequestMethod.GET, RequestMethod.POST}) |
||||
@ApiOperation(value = "查看,下面列表再调用eventController.list", notes = "传入taskid") |
||||
public R<TaskEntity> detail(@RequestParam Long id); |
||||
} |
@ -0,0 +1,27 @@
|
||||
package com.hnac.hzinfo.inspect.areamonthly.feign.fallback; |
||||
|
||||
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||
import com.hnac.hzinfo.inspect.areamonthly.feign.TaskFeignClient; |
||||
import com.hnac.hzinfo.inspect.areamonthly.vo.TaskListQuery; |
||||
import com.hnac.hzinfo.inspect.areamonthly.vo.TaskVo; |
||||
import lombok.extern.slf4j.Slf4j; |
||||
import org.springblade.core.mp.support.BladePage; |
||||
import org.springblade.core.mp.support.Query; |
||||
import org.springblade.core.tool.api.R; |
||||
import org.springframework.stereotype.Service; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 17:23 |
||||
*/ |
||||
@Slf4j |
||||
@Service |
||||
public class TaskFeignClientFallback implements TaskFeignClient { |
||||
@Override |
||||
public R<BladePage<TaskVo>> list(TaskListQuery task, Query query) { |
||||
|
||||
return R.fail("远程调用失败"); |
||||
} |
||||
} |
@ -0,0 +1,134 @@
|
||||
package com.hnac.hzinfo.inspect.areamonthly.vo; |
||||
import com.baomidou.mybatisplus.annotation.TableName; |
||||
import com.fasterxml.jackson.annotation.JsonFormat; |
||||
import com.hnac.hzinfo.inspect.utils.QueryField; |
||||
import com.hnac.hzinfo.inspect.utils.SqlCondition; |
||||
import io.swagger.annotations.ApiModel; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
import org.springblade.core.tenant.mp.TenantEntity; |
||||
import org.springframework.format.annotation.DateTimeFormat; |
||||
|
||||
import java.math.BigDecimal; |
||||
import java.util.Date; |
||||
|
||||
/** |
||||
* 实体类 |
||||
* |
||||
* @author |
||||
*/ |
||||
@Data |
||||
@TableName("hz_st_camera_info") |
||||
@EqualsAndHashCode(callSuper = true) |
||||
@ApiModel(value = "ImsMonCameraInfo对象", description = "视频巡检、机器人巡检摄像头信息") |
||||
public class CameraInfoEntity extends TenantEntity { |
||||
|
||||
private static final long serialVersionUID = 187639487673L; |
||||
|
||||
/** |
||||
* 摄像头名称 |
||||
*/ |
||||
@QueryField(condition = SqlCondition.LIKE) |
||||
@ApiModelProperty(value = "摄像头名称") |
||||
private String name; |
||||
|
||||
/** |
||||
* 摄像头类型 |
||||
*/ |
||||
@QueryField(condition = SqlCondition.EQUAL) |
||||
@ApiModelProperty(value = "摄像头类型") |
||||
private String type; |
||||
|
||||
/** |
||||
* 摄像头地址 |
||||
*/ |
||||
@QueryField(condition = SqlCondition.LIKE) |
||||
@ApiModelProperty(value = "摄像头地址") |
||||
private String address; |
||||
/** |
||||
* 配置拍照间隔时间 |
||||
*/ |
||||
@ApiModelProperty(value = "配置拍照间隔时间") |
||||
private BigDecimal spanTime; |
||||
/** |
||||
* 像素值(_x_代表使用原始值,640x480代表强制缩放到640x480) |
||||
*/ |
||||
@ApiModelProperty(value = "像素值(_x_代表使用原始值,640x480代表强制缩放到640x480)") |
||||
private String resolution; |
||||
/** |
||||
* 摄像头IP |
||||
*/ |
||||
@QueryField(condition = SqlCondition.LIKE) |
||||
@ApiModelProperty(value = "摄像头IP") |
||||
private String ip; |
||||
/** |
||||
* 摄像头端口 |
||||
*/ |
||||
@QueryField(condition = SqlCondition.EQUAL) |
||||
@ApiModelProperty(value = "摄像头端口") |
||||
private Integer port; |
||||
/** |
||||
* 摄像头账号 |
||||
*/ |
||||
@QueryField(condition = SqlCondition.LIKE) |
||||
@ApiModelProperty(value = "摄像头账号") |
||||
private String user; |
||||
/** |
||||
* 摄像头账号密码 |
||||
*/ |
||||
@ApiModelProperty(value = "摄像头账号密码") |
||||
private String password; |
||||
/** |
||||
* 告警检测项目(字典表值, 通过 ^ 隔开),字典类型:minitor_item |
||||
*/ |
||||
@QueryField(condition = SqlCondition.LIKE) |
||||
@ApiModelProperty(value = "告警检测项目(字典表值, 通过 ^ 隔开),字典类型:minitor_item") |
||||
private String monitorItems; |
||||
|
||||
|
||||
/** |
||||
* 视频流地址类型 |
||||
*/ |
||||
@ApiModelProperty(value = "视频流地址类型, 字典值:vedio_address_type") |
||||
private Long addressType; |
||||
/** |
||||
* 视频流地址 |
||||
*/ |
||||
@ApiModelProperty(value = "视频流地址") |
||||
private String livesourceaddress; |
||||
/** |
||||
* 监测点编号 |
||||
*/ |
||||
@QueryField(condition = SqlCondition.LIKE) |
||||
@ApiModelProperty(value = "监测点编号") |
||||
private String pointCode; |
||||
/** |
||||
* 最后请求时间 |
||||
*/ |
||||
@DateTimeFormat( |
||||
pattern = "yyyy-MM-dd HH:mm:ss" |
||||
) |
||||
@JsonFormat( |
||||
pattern = "yyyy-MM-dd HH:mm:ss" |
||||
) |
||||
@ApiModelProperty(value = "最后请求时间") |
||||
private Date lastRequesttime; |
||||
/** |
||||
* 检测点id |
||||
*/ |
||||
@ApiModelProperty(value = "检测点id") |
||||
private Long pointId; |
||||
/** |
||||
* 是否云台控制 |
||||
*/ |
||||
@ApiModelProperty(value = "是否云台控制") |
||||
private Integer isControl; |
||||
/** |
||||
* 是否平台接入 |
||||
*/ |
||||
@ApiModelProperty(value = "是否平台接入") |
||||
private Integer isHikvideo; |
||||
|
||||
} |
||||
|
@ -0,0 +1,54 @@
|
||||
package com.hnac.hzinfo.inspect.areamonthly.vo; |
||||
|
||||
import com.baomidou.mybatisplus.annotation.TableName; |
||||
import com.hnac.hzinfo.inspect.utils.QueryField; |
||||
import com.hnac.hzinfo.inspect.utils.SqlCondition; |
||||
import io.swagger.annotations.ApiModel; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
import org.springblade.core.tenant.mp.TenantEntity; |
||||
|
||||
/** |
||||
* @Author dfy |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 17:29 |
||||
*/ |
||||
@Data |
||||
@TableName("hz_st_robot") |
||||
@EqualsAndHashCode(callSuper = true) |
||||
@ApiModel(value = "Robot对象", description = "机器人巡检机器人实体类") |
||||
public class RobotEntity extends TenantEntity { |
||||
|
||||
private static final long serialVersionUID = 4503880134032836625L; |
||||
|
||||
/** |
||||
* 机器人用户ID |
||||
*/ |
||||
@QueryField(condition = SqlCondition.EQUAL) |
||||
@ApiModelProperty(value = "机器人用户ID") |
||||
private Long userId; |
||||
|
||||
/** |
||||
* 机器人带的摄像头ID |
||||
*/ |
||||
@QueryField(condition = SqlCondition.EQUAL) |
||||
@ApiModelProperty(value = "机器人带的摄像头ID, 多个摄像头通过 , 隔开") |
||||
private String cameraId; |
||||
|
||||
/** |
||||
* 机器人名称 |
||||
*/ |
||||
@QueryField(condition = SqlCondition.LIKE) |
||||
@ApiModelProperty(value = "机器人名称") |
||||
private String name; |
||||
|
||||
/** |
||||
* 描述信息 |
||||
*/ |
||||
@QueryField(condition = SqlCondition.LIKE) |
||||
@ApiModelProperty(value = "描述信息") |
||||
private Long remark; |
||||
|
||||
} |
@ -0,0 +1,30 @@
|
||||
package com.hnac.hzinfo.inspect.areamonthly.vo; |
||||
|
||||
import io.swagger.annotations.ApiModel; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
|
||||
import java.util.List; |
||||
|
||||
/** |
||||
* @Author dfy |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 17:29 |
||||
*/ |
||||
@Data |
||||
@EqualsAndHashCode(callSuper = true) |
||||
@ApiModel(value = "RobotVO", description = "机器人返回信息") |
||||
public class RobotVO extends RobotEntity { |
||||
|
||||
private static final long serialVersionUID = 3439398592744684238L; |
||||
|
||||
/** |
||||
* 摄像头信息 |
||||
*/ |
||||
@ApiModelProperty(value = "摄像头信息") |
||||
private List<CameraInfoEntity> cameraInfo; |
||||
|
||||
} |
||||
|
@ -0,0 +1,138 @@
|
||||
package com.hnac.hzinfo.inspect.areamonthly.vo; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 18:02 |
||||
*/ |
||||
|
||||
import com.fasterxml.jackson.annotation.JsonFormat; |
||||
import io.swagger.annotations.ApiModel; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Getter; |
||||
import lombok.Setter; |
||||
import org.springframework.format.annotation.DateTimeFormat; |
||||
|
||||
import java.io.Serializable; |
||||
import java.time.LocalDate; |
||||
import java.time.LocalDateTime; |
||||
import java.time.YearMonth; |
||||
|
||||
/** |
||||
* @author ninglong |
||||
* @create 2020-08-26 8:32 |
||||
*/ |
||||
@Getter |
||||
@Setter |
||||
@ApiModel(value="任务列表查询对象") |
||||
public class TaskListQuery implements Serializable { |
||||
private static final long serialVersionUID = 6980525036205124047L; |
||||
|
||||
/** |
||||
* 计划名称 |
||||
*/ |
||||
@ApiModelProperty(value = "计划名称") |
||||
private String planName; |
||||
|
||||
/** |
||||
* 值班id |
||||
*/ |
||||
@ApiModelProperty(value = "值班id") |
||||
private Long dutyId; |
||||
|
||||
/** |
||||
* 任务批次号 |
||||
*/ |
||||
@ApiModelProperty(value = "任务批次号") |
||||
private String batchNumber; |
||||
|
||||
@ApiModelProperty("任务状态 0未开始 1进行中 2暂停 3已完成 4未完成") |
||||
private Integer status; |
||||
|
||||
/** |
||||
* 计划周期 0: 从不 1:每天 2:每月 3:每季度 4:每年 5:每周 |
||||
*/ |
||||
@ApiModelProperty(value = "巡检周期 0: 每次 1:每天 2:每月 3:每季度 4:每年 5:每周") |
||||
private String cycle; |
||||
|
||||
/** |
||||
* 任务计划开始时间 |
||||
*/ |
||||
@DateTimeFormat( |
||||
pattern = "yyyy-MM-dd HH:mm:ss" |
||||
) |
||||
@JsonFormat( |
||||
pattern = "yyyy-MM-dd HH:mm:ss" |
||||
) |
||||
@ApiModelProperty(value = "任务计划开始时间-起") |
||||
private LocalDateTime planStartTime; |
||||
|
||||
/** |
||||
* 任务计划开始时间-止 |
||||
*/ |
||||
@DateTimeFormat( |
||||
pattern = "yyyy-MM-dd HH:mm:ss" |
||||
) |
||||
@JsonFormat( |
||||
pattern = "yyyy-MM-dd HH:mm:ss" |
||||
) |
||||
@ApiModelProperty(value = "任务计划开始时间-止") |
||||
private LocalDateTime planStartTimeEnd; |
||||
|
||||
/** |
||||
* 任务计划结束时间 |
||||
*/ |
||||
@DateTimeFormat( |
||||
pattern = "yyyy-MM-dd HH:mm:ss" |
||||
) |
||||
@JsonFormat( |
||||
pattern = "yyyy-MM-dd HH:mm:ss" |
||||
) |
||||
@ApiModelProperty(value = "任务计划结束时间") |
||||
private LocalDateTime planEndTime; |
||||
|
||||
@DateTimeFormat( |
||||
pattern = "yyyy-MM-dd HH:mm:ss" |
||||
) |
||||
@JsonFormat( |
||||
pattern = "yyyy-MM-dd HH:mm:ss" |
||||
) |
||||
@ApiModelProperty(value = "任务实际开始时间") |
||||
private LocalDateTime startTime; |
||||
|
||||
@ApiModelProperty(value = "租户id",hidden = true) |
||||
private String tenantId; |
||||
|
||||
@ApiModelProperty(value = "当前登录的用户",hidden = true) |
||||
private Long curUserId; |
||||
|
||||
@ApiModelProperty(value = "当前对象id,用于扫描对象接口") |
||||
private Long objectId; |
||||
|
||||
@ApiModelProperty(value = "任务报备 0 未报备 1已报备") |
||||
private String keepOnRecord; |
||||
|
||||
/** |
||||
* 巡检类型标识: 0普通巡检,1视频自动巡检,2机器人巡检 |
||||
*/ |
||||
@ApiModelProperty(value = "巡检类型标识: 0普通巡检,1视频自动巡检,2机器人巡检") |
||||
private String autoVideo; |
||||
|
||||
@ApiModelProperty("巡检任务 按逗号分隔") |
||||
private String taskIds; |
||||
|
||||
|
||||
/** |
||||
* 月份 |
||||
*/ |
||||
private YearMonth yearmonth; |
||||
|
||||
/** |
||||
* 区域Id |
||||
*/ |
||||
private String areaId; |
||||
|
||||
|
||||
} |
||||
|
@ -0,0 +1,29 @@
|
||||
package com.hnac.hzinfo.inspect.areamonthly.vo; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 17:28 |
||||
*/ |
||||
|
||||
import com.hnac.hzinfo.inspect.task.entity.TaskEntity; |
||||
import io.swagger.annotations.ApiModel; |
||||
import lombok.Data; |
||||
|
||||
/** |
||||
* @Author: dfy |
||||
*/ |
||||
@Data |
||||
@ApiModel(value="任务返回对象") |
||||
public class TaskVo extends TaskEntity { |
||||
|
||||
/** |
||||
* 是否能撤销领用 |
||||
*/ |
||||
private Boolean canCancel = false; |
||||
/** |
||||
* 机器人信息 |
||||
*/ |
||||
private RobotVO robot; |
||||
} |
@ -0,0 +1,24 @@
|
||||
package com.hnac.hzims.ticket.areamonthly.dto; |
||||
|
||||
import com.hnac.hzims.ticket.allTicket.entity.TicketInfoEvaluateEntity; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
|
||||
import java.io.Serializable; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 13:44 |
||||
*/ |
||||
@Data |
||||
@EqualsAndHashCode |
||||
public class TicketInfoEvaluateDto extends TicketInfoEvaluateEntity implements Serializable { |
||||
private static final long serialVersionUID = -4416736590841388580L; |
||||
|
||||
@ApiModelProperty("工作票种类") |
||||
private Integer ticketType; |
||||
|
||||
} |
@ -0,0 +1,60 @@
|
||||
package com.hnac.hzims.ticket.areamonthly.vo; |
||||
|
||||
import lombok.Data; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 11:06 |
||||
*/ |
||||
@Data |
||||
public class MaintenanceTaskVo { |
||||
|
||||
/** |
||||
* 编号 |
||||
*/ |
||||
private Long id; |
||||
|
||||
|
||||
/** |
||||
* 项目名称 |
||||
*/ |
||||
private String title; |
||||
|
||||
/** |
||||
* 维护内容 |
||||
*/ |
||||
private String content; |
||||
|
||||
/** |
||||
* 执行人 |
||||
*/ |
||||
private String disposerName; |
||||
|
||||
/** |
||||
* 类型 |
||||
*/ |
||||
private String typeCode; |
||||
|
||||
/** |
||||
* 类型名称 |
||||
*/ |
||||
private String typeCodeValue; |
||||
|
||||
/** |
||||
* 执行状态 |
||||
*/ |
||||
private Integer status; |
||||
|
||||
/** |
||||
* 执行状态名称 |
||||
*/ |
||||
private String statusValue; |
||||
|
||||
/** |
||||
* 任务类型 |
||||
*/ |
||||
private String taskType; |
||||
|
||||
} |
@ -0,0 +1,49 @@
|
||||
package com.hnac.hzims.ticket.areamonthly.vo; |
||||
|
||||
import lombok.Data; |
||||
|
||||
import java.time.YearMonth; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 12:00 |
||||
*/ |
||||
@Data |
||||
public class MaintenanceTaskWithAreaVo { |
||||
|
||||
|
||||
/** |
||||
* 区域编号 |
||||
*/ |
||||
private String areaId; |
||||
|
||||
|
||||
/** |
||||
* 月份 |
||||
*/ |
||||
private java.time.YearMonth YearMonth; |
||||
|
||||
/** |
||||
* 项目名称 |
||||
*/ |
||||
private String title; |
||||
|
||||
/** |
||||
* 维护内容 |
||||
*/ |
||||
private String content; |
||||
|
||||
/** |
||||
* 执行状态 |
||||
*/ |
||||
private Integer status; |
||||
|
||||
|
||||
/** |
||||
* 任务类型 |
||||
*/ |
||||
private String taskType; |
||||
|
||||
} |
@ -0,0 +1,45 @@
|
||||
package com.hnac.hzims.ticket.areamonthly.vo; |
||||
|
||||
import com.hnac.hzims.ticket.standardTicket.entity.StandardTicketInfoEntity; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
|
||||
import java.io.Serializable; |
||||
import java.util.List; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 13:31 |
||||
*/ |
||||
@EqualsAndHashCode |
||||
@Data |
||||
public class StandardTicketInfoVO extends StandardTicketInfoEntity implements Serializable { |
||||
private static final long serialVersionUID = -8870464581684939369L; |
||||
|
||||
@ApiModelProperty("创建人名称") |
||||
private String createUserName; |
||||
|
||||
@ApiModelProperty("更新人名称") |
||||
private String updateUserName; |
||||
|
||||
@ApiModelProperty("来源类型名称") |
||||
private String taskTypeName; |
||||
|
||||
@ApiModelProperty("发令人名称") |
||||
private String issueOrderPersonName; |
||||
|
||||
@ApiModelProperty("受令人名称") |
||||
private String accessOrderPersonName; |
||||
|
||||
@ApiModelProperty("负责人名称") |
||||
private String principalName; |
||||
|
||||
@ApiModelProperty("许可人名称") |
||||
private String guardianName; |
||||
|
||||
@ApiModelProperty("安全措施完成明细") |
||||
private List<StandardTicketMeasureVO> standardTicketMeasureVOList; |
||||
} |
@ -0,0 +1,28 @@
|
||||
package com.hnac.hzims.ticket.areamonthly.vo; |
||||
|
||||
import com.hnac.hzims.ticket.standardTicket.entity.StandardTicketMeasureEntity; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
|
||||
import java.io.Serializable; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 13:31 |
||||
*/ |
||||
@Data |
||||
@EqualsAndHashCode |
||||
public class StandardTicketMeasureVO extends StandardTicketMeasureEntity implements Serializable { |
||||
|
||||
@ApiModelProperty("创建人名称") |
||||
private String createUserName; |
||||
|
||||
@ApiModelProperty("更新人名称") |
||||
private String updateUserName; |
||||
|
||||
@ApiModelProperty("执行人名称") |
||||
private String executorName; |
||||
} |
@ -0,0 +1,72 @@
|
||||
package com.hnac.hzims.ticket.areamonthly.vo; |
||||
|
||||
import com.fasterxml.jackson.annotation.JsonFormat; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import org.springframework.format.annotation.DateTimeFormat; |
||||
|
||||
import java.time.LocalDateTime; |
||||
import java.time.YearMonth; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/11 12:55 |
||||
*/ |
||||
@Data |
||||
public class StandardTicketWithAreaVo { |
||||
|
||||
|
||||
/** |
||||
* 区域编号 |
||||
*/ |
||||
private String areaId; |
||||
|
||||
|
||||
/** |
||||
* 月份 |
||||
*/ |
||||
private YearMonth YearMonth; |
||||
|
||||
|
||||
/** |
||||
* 开票种类 |
||||
*/ |
||||
private String ticketType; |
||||
|
||||
|
||||
/** |
||||
* 编号 |
||||
*/ |
||||
private String code; |
||||
/** |
||||
* 开票来源 |
||||
*/ |
||||
private String taskType; |
||||
|
||||
|
||||
/** |
||||
* 状态 |
||||
*/ |
||||
private Integer status; |
||||
|
||||
|
||||
/** |
||||
* 开始时间 |
||||
*/ |
||||
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||
@ApiModelProperty("开始时间") |
||||
private LocalDateTime startTime; |
||||
|
||||
|
||||
/** |
||||
* 结束时间 |
||||
*/ |
||||
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||
@ApiModelProperty("结束时间") |
||||
private LocalDateTime endTime; |
||||
|
||||
} |
@ -0,0 +1,66 @@
|
||||
package com.hnac.hzims.ticket.areamonthly.vo; |
||||
|
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
|
||||
import java.io.Serializable; |
||||
import java.util.Date; |
||||
|
||||
/** |
||||
* @Author WL |
||||
* @Version v1.0 |
||||
* @Serial 1.0 |
||||
* @Date 2023/4/10 17:06 |
||||
*/ |
||||
@EqualsAndHashCode |
||||
@Data |
||||
public class StandardWorkVo implements Serializable { |
||||
|
||||
private static final long serialVersionUID = -8870464581684939369L; |
||||
|
||||
|
||||
/** |
||||
* 编号 |
||||
*/ |
||||
private Long id; |
||||
|
||||
|
||||
/** |
||||
* 开票种类 |
||||
*/ |
||||
private String type; |
||||
|
||||
/** |
||||
* 单位 |
||||
*/ |
||||
private String company; |
||||
/** |
||||
* 编号 |
||||
*/ |
||||
private String code; |
||||
/** |
||||
* 开票来源 |
||||
*/ |
||||
private String taskType; |
||||
/** |
||||
* 开票任务来源 |
||||
*/ |
||||
private String taskName; |
||||
|
||||
|
||||
/** |
||||
* 开票时间 |
||||
*/ |
||||
private Date createTime; |
||||
|
||||
/** |
||||
* 状态 |
||||
*/ |
||||
private Integer status; |
||||
|
||||
/** |
||||
* 是否评价 |
||||
*/ |
||||
private Integer isEvaluate; |
||||
} |
||||
|
@ -1,104 +0,0 @@
|
||||
// package com.hnac.hzims.middle.mybatisplus;
|
||||
//
|
||||
// import com.baomidou.mybatisplus.generator.AutoGenerator;
|
||||
// import com.baomidou.mybatisplus.generator.InjectionConfig;
|
||||
// import com.baomidou.mybatisplus.generator.config.*;
|
||||
// import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy;
|
||||
//
|
||||
// import java.util.ArrayList;
|
||||
// import java.util.List;
|
||||
//
|
||||
// /**
|
||||
// * @Author WL
|
||||
// * @Version v1.0
|
||||
// * @Serial 1.0
|
||||
// * @Date 2023/4/4 9:07
|
||||
// */
|
||||
// public class CodeGenerator {
|
||||
//
|
||||
//
|
||||
// public static void main(String[] args) {
|
||||
// // 代码生成器
|
||||
// AutoGenerator mpg = new AutoGenerator();
|
||||
//
|
||||
// // 全局配置
|
||||
// GlobalConfig gc = new GlobalConfig();
|
||||
// String projectPath = System.getProperty("user.dir") + "/hzims-service/hzims-middle";
|
||||
// gc.setOutputDir(projectPath + "/src/main/java");
|
||||
// gc.setAuthor("dfy");
|
||||
// gc.setOpen(false);
|
||||
// // gc.setSwagger2(true); 实体属性 Swagger2 注解
|
||||
// mpg.setGlobalConfig(gc);
|
||||
//
|
||||
// // 数据源配置
|
||||
// DataSourceConfig dsc = new DataSourceConfig();
|
||||
// dsc.setUrl("jdbc:mysql://192.168.1.20:3576/dev_hzims_middle?useSSL=false&useUnicode=true&characterEncoding=utf-8&zeroDateTimeBehavior=convertToNull&transformedBitIsBoolean=true&tinyInt1isBit=false&allowMultiQueries=true&serverTimezone=GMT%2B8&nullCatalogMeansCurrent=true&allowPublicKeyRetrieval=true");
|
||||
// // dsc.setSchemaName("public");
|
||||
// dsc.setDriverName("com.mysql.cj.jdbc.Driver");
|
||||
// dsc.setUsername("root");
|
||||
// dsc.setPassword("123");
|
||||
// mpg.setDataSource(dsc);
|
||||
//
|
||||
// // 包配置
|
||||
// PackageConfig pc = new PackageConfig();
|
||||
// pc.setModuleName("systemlog");
|
||||
// pc.setParent("com.hnac.hzims.middle");
|
||||
// mpg.setPackageInfo(pc);
|
||||
//
|
||||
// // 自定义配置
|
||||
// InjectionConfig cfg = new InjectionConfig() {
|
||||
// @Override
|
||||
// public void initMap() {
|
||||
// // to do nothing
|
||||
// }
|
||||
// };
|
||||
//
|
||||
// // 如果模板引擎是 freemarker
|
||||
// String templatePath = "/templates/mapper.xml.ftl";
|
||||
// // 如果模板引擎是 velocity
|
||||
// // String templatePath = "/templates/mapper.xml.vm";
|
||||
//
|
||||
// // 自定义输出配置
|
||||
// List<FileOutConfig> focList = new ArrayList<>();
|
||||
// cfg.setFileOutConfigList(focList);
|
||||
// mpg.setCfg(cfg);
|
||||
//
|
||||
// // 配置模板
|
||||
// TemplateConfig templateConfig = new TemplateConfig();
|
||||
//
|
||||
// // 配置自定义输出模板
|
||||
// //指定自定义模板路径,注意不要带上.ftl/.vm, 会根据使用的模板引擎自动识别
|
||||
// // templateConfig.setEntity("templates/entity2.java");
|
||||
// // templateConfig.setService();
|
||||
// // templateConfig.setController();
|
||||
//
|
||||
// // templateConfig.setXml(null);
|
||||
// mpg.setTemplate(templateConfig);
|
||||
//
|
||||
// // 策略配置
|
||||
// StrategyConfig strategy = new StrategyConfig();
|
||||
// strategy.setNaming(NamingStrategy.underline_to_camel);
|
||||
// strategy.setColumnNaming(NamingStrategy.underline_to_camel);
|
||||
// // strategy.setSuperEntityClass("你自己的父类实体,没有就不用设置!");
|
||||
// strategy.setEntityLombokModel(true);
|
||||
// strategy.setRestControllerStyle(true);
|
||||
// strategy.setEntityBooleanColumnRemoveIsPrefix(true);
|
||||
// strategy.setEntityLombokModel(true);
|
||||
// strategy.setEntitySerialVersionUID(true);
|
||||
// strategy.setChainModel(true);
|
||||
// strategy.setEntityTableFieldAnnotationEnable(true);
|
||||
// // strategy.setFieldPrefix("hzims_");
|
||||
// // 公共父类
|
||||
// // strategy.setSuperControllerClass("你自己的父类控制器,没有就不用设置!");
|
||||
// // 写于父类中的公共字段
|
||||
// // strategy.setSuperEntityColumns("id");
|
||||
// strategy.setInclude("hzims_statistics");
|
||||
// strategy.setControllerMappingHyphenStyle(true);
|
||||
// strategy.setTablePrefix("hzims_");
|
||||
// mpg.setStrategy(strategy);
|
||||
// // mpg.setTemplateEngine(new FreemarkerTemplateEngine());
|
||||
// mpg.execute();
|
||||
// }
|
||||
//
|
||||
// }
|
||||
//
|
Loading…
Reference in new issue