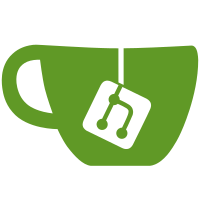
11 changed files with 493 additions and 1 deletions
@ -0,0 +1,39 @@
|
||||
package com.hnac.hzims.fdp.request; |
||||
|
||||
import io.swagger.annotations.ApiModel; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Builder; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
import lombok.NonNull; |
||||
|
||||
import javax.validation.constraints.NotNull; |
||||
import java.io.Serializable; |
||||
|
||||
/** |
||||
* @author hx |
||||
* @version 1.0 |
||||
* @date 2023/3/6 10:03 |
||||
*/ |
||||
@Data |
||||
@EqualsAndHashCode |
||||
@ApiModel("FDP站点信息请求") |
||||
@Builder |
||||
public class StationInfoReq implements Serializable { |
||||
|
||||
@ApiModelProperty(value = "站点类型",required = true) |
||||
@NotNull(message = "站点类型不能为空") |
||||
private Integer type; |
||||
|
||||
@ApiModelProperty("站点ID") |
||||
@NotNull(message = "站点ID不能为空") |
||||
private String stationId; |
||||
|
||||
@ApiModelProperty("站点名称") |
||||
@NotNull(message = "站点名称不能为空") |
||||
private String stationName; |
||||
|
||||
@ApiModelProperty("站点描述") |
||||
private String stationDesc; |
||||
|
||||
} |
@ -0,0 +1,60 @@
|
||||
package com.hnac.hzims.fdp.controller; |
||||
|
||||
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||
import com.hnac.hzims.fdp.request.SubmitAnswerReq; |
||||
import com.hnac.hzims.fdp.response.DevsetQuestionResp; |
||||
import com.hnac.hzims.fdp.service.IFdpQuestionService; |
||||
import io.swagger.annotations.Api; |
||||
import io.swagger.annotations.ApiOperation; |
||||
import io.swagger.annotations.ApiParam; |
||||
import lombok.AllArgsConstructor; |
||||
import org.springblade.core.boot.ctrl.BladeController; |
||||
import org.springblade.core.tool.api.R; |
||||
import org.springframework.validation.annotation.Validated; |
||||
import org.springframework.web.bind.annotation.*; |
||||
|
||||
import java.util.List; |
||||
|
||||
/** |
||||
* @author hx |
||||
* @version 1.0 |
||||
* @date 2023/3/6 11:52 |
||||
*/ |
||||
@RestController |
||||
@RequestMapping("/fdp/question") |
||||
@AllArgsConstructor |
||||
@Api(value = "智能诊断接站问答",tags = "智能诊断接站问答") |
||||
public class FdpQuestionController extends BladeController { |
||||
|
||||
private final IFdpQuestionService fdpQuestionService; |
||||
|
||||
@GetMapping("/submitStationInfo") |
||||
@ApiOperation("提交站点信息") |
||||
@ApiOperationSupport(order = 1) |
||||
public R<Boolean> submitStationInfo(@RequestParam @ApiParam(value = "站点编码",required = true) String stationCode, @RequestParam(required = false) @ApiParam(value = "描述") String stationDesc) { |
||||
return R.status(fdpQuestionService.submitStationInfo(stationCode,stationDesc)); |
||||
} |
||||
|
||||
@DeleteMapping("/deleteStationInfo/{stationCode}") |
||||
@ApiOperation("删除站点信息") |
||||
@ApiOperationSupport(order = 2) |
||||
public R<Boolean> deleteStationInfo(@PathVariable @ApiParam(value = "站点编码",required = true) String stationCode) { |
||||
return R.status(fdpQuestionService.deleteStationInfo(stationCode)); |
||||
} |
||||
|
||||
@GetMapping("/getDevsetQuestion") |
||||
@ApiOperation("获取设备推送Fdp问题") |
||||
@ApiOperationSupport(order = 3) |
||||
public R<List<DevsetQuestionResp>> getDevsetQuestion(@RequestParam @ApiParam(value = "设备类型,1:公用;2:机组",required = true) String deviceType, |
||||
@RequestParam @ApiParam(value = "站点类型",required = true) Integer stationType) { |
||||
return R.data(fdpQuestionService.getDevsetQuestion(deviceType,stationType)); |
||||
} |
||||
|
||||
@PostMapping("/submitDeviceAnswer") |
||||
@ApiOperation("提交设备问题答案") |
||||
@ApiOperationSupport(order = 4) |
||||
public R<Boolean> submitDeviceAnswer(@RequestBody @Validated SubmitAnswerReq submitAnswerReq) { |
||||
return R.data(fdpQuestionService.submitDeviceAnswer(submitAnswerReq)); |
||||
} |
||||
|
||||
} |
@ -0,0 +1,31 @@
|
||||
package com.hnac.hzims.fdp.request; |
||||
|
||||
import io.swagger.annotations.ApiModel; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
|
||||
import javax.validation.constraints.NotNull; |
||||
import java.io.Serializable; |
||||
|
||||
/** |
||||
* @author hx |
||||
* @version 1.0 |
||||
* @date 2023/3/6 16:12 |
||||
*/ |
||||
@Data |
||||
@ApiModel("提交答案格式") |
||||
@EqualsAndHashCode |
||||
public class AnswerFormatReq implements Serializable { |
||||
|
||||
@ApiModelProperty(value = "问题ID",required = true) |
||||
@NotNull |
||||
private String questionId; |
||||
|
||||
@ApiModelProperty(value = "答案ID,选择题需要传多选题答案ID用逗号分隔") |
||||
private String answerId; |
||||
|
||||
@ApiModelProperty("答案内容,填空题需要传") |
||||
private String inputValue; |
||||
|
||||
} |
@ -0,0 +1,42 @@
|
||||
package com.hnac.hzims.fdp.request; |
||||
|
||||
import com.alibaba.fastjson.annotation.JSONField; |
||||
import io.swagger.annotations.ApiModel; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
|
||||
import javax.validation.constraints.NotNull; |
||||
import javax.xml.soap.Name; |
||||
import java.io.Serializable; |
||||
import java.util.List; |
||||
|
||||
/** |
||||
* @author hx |
||||
* @version 1.0 |
||||
* @date 2023/3/6 16:19 |
||||
*/ |
||||
@ApiModel("提交答案") |
||||
@Data |
||||
@EqualsAndHashCode |
||||
public class SubmitAnswerReq implements Serializable { |
||||
|
||||
@ApiModelProperty(value = "站点类型",required = true) |
||||
@NotNull |
||||
private Integer stationType; |
||||
|
||||
private Integer type; |
||||
|
||||
@ApiModelProperty(value = "站点ID",required = true) |
||||
@NotNull |
||||
private String stationId; |
||||
|
||||
@ApiModelProperty(value = "设备类型",required = true) |
||||
@NotNull |
||||
private String deviceType; |
||||
|
||||
@ApiModelProperty(value = "站点问题数据",required = true) |
||||
@JSONField(name = "data") |
||||
private List<AnswerFormatReq> answerFormatList; |
||||
|
||||
} |
@ -0,0 +1,38 @@
|
||||
package com.hnac.hzims.fdp.response; |
||||
|
||||
import com.alibaba.fastjson.annotation.JSONField; |
||||
import io.swagger.annotations.ApiModel; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
|
||||
import java.io.Serializable; |
||||
import java.util.List; |
||||
|
||||
/** |
||||
* @author hx |
||||
* @version 1.0 |
||||
* @date 2023/3/6 14:40 |
||||
*/ |
||||
@Data |
||||
@EqualsAndHashCode |
||||
@ApiModel("机组问题答案") |
||||
public class DevsetAnswerResp implements Serializable { |
||||
|
||||
@ApiModelProperty("答案ID") |
||||
@JSONField(name = "AID") |
||||
private String aId; |
||||
|
||||
@ApiModelProperty("答案名称") |
||||
@JSONField(name = "ANAME") |
||||
private String aName; |
||||
|
||||
@ApiModelProperty("是否默认选中。0为不选中,1为选中") |
||||
@JSONField(name = "ADEFAULT") |
||||
private Integer aDefault; |
||||
|
||||
@ApiModelProperty("选中该答案需要继续回答的问题数组") |
||||
@JSONField(name = "QUESTION") |
||||
private List<DevsetQuestionResp> question; |
||||
|
||||
} |
@ -0,0 +1,43 @@
|
||||
package com.hnac.hzims.fdp.response; |
||||
|
||||
/** |
||||
* @author hx |
||||
* @version 1.0 |
||||
* @date 2023/3/6 14:35 |
||||
*/ |
||||
|
||||
import com.alibaba.fastjson.annotation.JSONField; |
||||
import io.swagger.annotations.ApiModel; |
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
import lombok.EqualsAndHashCode; |
||||
|
||||
import java.io.Serializable; |
||||
import java.util.List; |
||||
|
||||
@Data |
||||
@EqualsAndHashCode |
||||
@ApiModel("机组问题") |
||||
public class DevsetQuestionResp implements Serializable { |
||||
|
||||
@ApiModelProperty("问题ID") |
||||
@JSONField(name = "ID") |
||||
private String id; |
||||
|
||||
@ApiModelProperty("问题名称") |
||||
@JSONField(name = "NAME") |
||||
private String name; |
||||
|
||||
@ApiModelProperty("问题类型。当前有“单选”、“多选”、“参数填空”、“数量填空”") |
||||
@JSONField(name = "QTYPE") |
||||
private String qType; |
||||
|
||||
@ApiModelProperty("问题的父答案ID") |
||||
@JSONField(name = "FAID") |
||||
private String parentId; |
||||
|
||||
@ApiModelProperty("问题的答案列表") |
||||
@JSONField(name = "ANSWER") |
||||
private List<DevsetAnswerResp> answer; |
||||
|
||||
} |
@ -0,0 +1,48 @@
|
||||
package com.hnac.hzims.fdp.service; |
||||
|
||||
import com.hnac.hzims.fdp.request.SubmitAnswerReq; |
||||
import com.hnac.hzims.fdp.response.DevsetQuestionResp; |
||||
import io.swagger.annotations.ApiParam; |
||||
import org.springframework.web.bind.annotation.RequestBody; |
||||
import org.springframework.web.bind.annotation.RequestParam; |
||||
|
||||
import java.util.List; |
||||
|
||||
/** |
||||
* @author hx |
||||
* @version 1.0 |
||||
* @date 2023/3/6 10:18 |
||||
*/ |
||||
public interface IFdpQuestionService { |
||||
|
||||
/** |
||||
* 提交站点信息 |
||||
* @param stationCode 站点编码 |
||||
* @param stationDesc 站点描述 |
||||
* @return |
||||
*/ |
||||
boolean submitStationInfo(String stationCode,String stationDesc); |
||||
|
||||
/** |
||||
* 删除站点信息 |
||||
* @param stationId 站点编码 |
||||
* @return |
||||
*/ |
||||
boolean deleteStationInfo(String stationId); |
||||
|
||||
/** |
||||
* 获取设备推送Fdp问题 |
||||
* @param deviceType 设备类型 |
||||
* @param stationType 站点类型 |
||||
* @return |
||||
*/ |
||||
List<DevsetQuestionResp> getDevsetQuestion(String deviceType, Integer stationType); |
||||
|
||||
/** |
||||
* 提交设备问题答案 |
||||
* @param submitAnswerReq 提交答案 |
||||
* @return |
||||
*/ |
||||
boolean submitDeviceAnswer(@RequestBody SubmitAnswerReq submitAnswerReq); |
||||
|
||||
} |
@ -0,0 +1,156 @@
|
||||
package com.hnac.hzims.fdp.service.impl; |
||||
|
||||
import cn.hutool.http.HttpRequest; |
||||
import cn.hutool.http.HttpResponse; |
||||
import com.alibaba.fastjson.JSON; |
||||
import com.alibaba.fastjson.JSONArray; |
||||
import com.alibaba.fastjson.JSONObject; |
||||
import com.google.common.collect.Lists; |
||||
import com.hnac.hzims.fdp.config.FdpUrlConfiguration; |
||||
import com.hnac.hzims.fdp.constants.FdpConstant; |
||||
import com.hnac.hzims.fdp.request.StationInfoReq; |
||||
import com.hnac.hzims.fdp.request.SubmitAnswerReq; |
||||
import com.hnac.hzims.fdp.response.DevsetQuestionResp; |
||||
import com.hnac.hzims.fdp.service.IFdpQuestionService; |
||||
import com.hnac.hzims.operational.station.StationConstants; |
||||
import com.hnac.hzims.operational.station.entity.StationEntity; |
||||
import com.hnac.hzims.operational.station.enume.StationEnumConstants; |
||||
import com.hnac.hzims.operational.station.feign.IStationClient; |
||||
import lombok.AllArgsConstructor; |
||||
import lombok.extern.slf4j.Slf4j; |
||||
import org.springblade.core.log.exception.ServiceException; |
||||
import org.springblade.core.log.logger.BladeLogger; |
||||
import org.springblade.core.tool.api.R; |
||||
import org.springblade.core.tool.utils.Func; |
||||
import org.springblade.core.tool.utils.StringUtil; |
||||
import org.springframework.stereotype.Service; |
||||
|
||||
import javax.servlet.http.HttpServletResponse; |
||||
import java.util.HashMap; |
||||
import java.util.List; |
||||
import java.util.Optional; |
||||
|
||||
/** |
||||
* @author hx |
||||
* @version 1.0 |
||||
* @date 2023/3/6 10:18 |
||||
*/ |
||||
@Service |
||||
@AllArgsConstructor |
||||
@Slf4j |
||||
public class FdpQuestionServiceImpl implements IFdpQuestionService { |
||||
|
||||
private final IStationClient stationClient; |
||||
private final FdpUrlConfiguration fdpUrlConfiguration; |
||||
private final BladeLogger logger; |
||||
|
||||
@Override |
||||
public boolean submitStationInfo(String stationCode, String stationDesc) { |
||||
R<StationEntity> stationResult = stationClient.getStationByCode(stationCode); |
||||
if(stationResult.isSuccess()) { |
||||
StationEntity stationEntity = stationResult.getData(); |
||||
StationInfoReq infoReq = StationInfoReq.builder() |
||||
.stationId(stationCode) |
||||
.stationName(stationEntity.getName()) |
||||
.type(this.getStationType(stationEntity.getType())) |
||||
.stationDesc(Optional.ofNullable(stationDesc).orElse("")) |
||||
.build(); |
||||
if(Func.isNotEmpty(infoReq.getType())) { |
||||
HttpResponse httpResponse = HttpRequest.post(fdpUrlConfiguration.getSubmitStationInfo()) |
||||
.body(JSON.toJSONString(infoReq)) |
||||
.execute(); |
||||
if(httpResponse.getStatus() == HttpServletResponse.SC_OK && |
||||
"1".equals(JSONObject.parseObject(httpResponse.body()).getString("success"))) { |
||||
return true; |
||||
} |
||||
else { |
||||
throw new ServiceException("Fdp信息站点信息失败!"); |
||||
} |
||||
} |
||||
} |
||||
return false; |
||||
} |
||||
|
||||
@Override |
||||
public boolean deleteStationInfo(String stationId) { |
||||
HttpResponse response = HttpRequest.post(fdpUrlConfiguration.getDeleteStationInfo()).body(JSON.toJSONString( |
||||
new HashMap<String, String>(1) {{ |
||||
put("stationId", stationId); |
||||
}} |
||||
)).execute(); |
||||
if(response.getStatus() == HttpServletResponse.SC_OK && |
||||
"1".equals(JSONObject.parseObject(response.body()).getString("success"))) { |
||||
return true; |
||||
} |
||||
else { |
||||
throw new ServiceException("Fdp删除站点信息失败!"); |
||||
} |
||||
} |
||||
|
||||
@Override |
||||
public List<DevsetQuestionResp> getDevsetQuestion(String deviceType, Integer stationType) { |
||||
List<DevsetQuestionResp> result = Lists.newArrayList(); |
||||
String getQuestionUrl = ""; |
||||
switch(deviceType) { |
||||
case FdpConstant.COMMON_DEVICE_TYPE: |
||||
getQuestionUrl = fdpUrlConfiguration.getGetStationQuestion(); |
||||
break; |
||||
case FdpConstant.SET_DEVICE_TYPE: |
||||
getQuestionUrl = fdpUrlConfiguration.getGetDevsetQuestion(); |
||||
break; |
||||
default: |
||||
break; |
||||
} |
||||
if(StringUtil.isNotBlank(getQuestionUrl)) { |
||||
HttpResponse response = HttpRequest.post(getQuestionUrl).body(JSON.toJSONString( |
||||
new HashMap<String, Integer>(1) {{ |
||||
put("type", getStationType(stationType)); |
||||
}} |
||||
)).execute(); |
||||
if(response.getStatus() == HttpServletResponse.SC_OK && |
||||
"1".equals(JSONObject.parseObject(response.body()).getString("success"))) { |
||||
JSONObject responseJSON = JSONObject.parseObject(response.body()); |
||||
result = JSONArray.parseArray(responseJSON.getString("data"), DevsetQuestionResp.class); |
||||
} |
||||
} |
||||
return result; |
||||
} |
||||
|
||||
@Override |
||||
public boolean submitDeviceAnswer(SubmitAnswerReq submitAnswerReq) { |
||||
logger.info("hzims:equipment:submitDeviceAnswer",JSON.toJSONString(submitAnswerReq)); |
||||
String submitAnswerUrl = ""; |
||||
switch(submitAnswerReq.getDeviceType()) { |
||||
case FdpConstant.COMMON_DEVICE_TYPE: |
||||
submitAnswerUrl = fdpUrlConfiguration.getSubmitStationAnswer(); |
||||
break; |
||||
case FdpConstant.SET_DEVICE_TYPE: |
||||
submitAnswerUrl = fdpUrlConfiguration.getSubmitDevsetAnswer(); |
||||
break; |
||||
default: |
||||
break; |
||||
} |
||||
if(StringUtil.isNotBlank(submitAnswerUrl)) { |
||||
submitAnswerReq.setType(this.getStationType(submitAnswerReq.getStationType())); |
||||
HttpResponse response = HttpRequest.post(submitAnswerUrl).body(JSON.toJSONString(submitAnswerReq)).execute(); |
||||
return response.getStatus() == HttpServletResponse.SC_OK && |
||||
"1".equals(JSONObject.parseObject(response.body()).getString("success")); |
||||
} |
||||
return false; |
||||
} |
||||
|
||||
/** |
||||
* 根据站点类型获取Fdp的站点类型 |
||||
* @param stationType 站点管理站点类型 |
||||
* @return Fdp站点类型 |
||||
*/ |
||||
private Integer getStationType(Integer stationType) { |
||||
if(StationEnumConstants.StationTypeEnum.STATION_TYPE_0.getStatus() == stationType) { |
||||
return FdpConstant.HYDROELECTRIC_STATION; |
||||
} |
||||
else if(StationEnumConstants.StationTypeEnum.STATION_TYPE_8.getStatus() == stationType) { |
||||
return FdpConstant.PUMP_STATION; |
||||
} |
||||
return null; |
||||
} |
||||
} |
Loading…
Reference in new issue