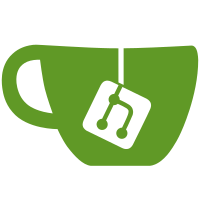
112 changed files with 5687 additions and 1 deletions
@ -0,0 +1,94 @@ |
|||||||
|
package com.hnac.hzims.cache; |
||||||
|
|
||||||
|
import com.github.benmanes.caffeine.cache.Cache; |
||||||
|
import com.github.benmanes.caffeine.cache.Caffeine; |
||||||
|
import com.hnac.hzims.entity.Report; |
||||||
|
import com.hnac.hzims.entity.YearReport; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
import java.util.concurrent.TimeUnit; |
||||||
|
|
||||||
|
//Caffeine采用了W-TinyLFU(LUR和LFU的优点结合)开源的缓存技术。缓存性能接近理论最优,属于是Guava Cache的增强版
|
||||||
|
public class CaffeineCacheUtil { |
||||||
|
|
||||||
|
//创建guava cache
|
||||||
|
private static Cache<String, Report> loadingCache = Caffeine.newBuilder() |
||||||
|
//cache的初始容量
|
||||||
|
.initialCapacity(5) |
||||||
|
//cache最大缓存数
|
||||||
|
.maximumSize(50) |
||||||
|
//设置写缓存后n秒钟过期
|
||||||
|
.expireAfterWrite(1, TimeUnit.HOURS) |
||||||
|
//设置读写缓存后n秒钟过期,实际很少用到,类似于expireAfterWrite
|
||||||
|
//.expireAfterAccess(17, TimeUnit.SECONDS)
|
||||||
|
.build(); |
||||||
|
|
||||||
|
private static Cache<String, YearReport> loadingYearCache = Caffeine.newBuilder() |
||||||
|
//cache的初始容量
|
||||||
|
.initialCapacity(5) |
||||||
|
//cache最大缓存数
|
||||||
|
.maximumSize(50) |
||||||
|
//设置写缓存后n秒钟过期
|
||||||
|
.expireAfterWrite(1, TimeUnit.HOURS) |
||||||
|
//设置读写缓存后n秒钟过期,实际很少用到,类似于expireAfterWrite
|
||||||
|
//.expireAfterAccess(17, TimeUnit.SECONDS)
|
||||||
|
.build(); |
||||||
|
|
||||||
|
private static Cache<String, List<Map<String,Object>>> loadingMapCache = Caffeine.newBuilder() |
||||||
|
//cache的初始容量
|
||||||
|
.initialCapacity(5) |
||||||
|
//cache最大缓存数
|
||||||
|
.maximumSize(50) |
||||||
|
//设置写缓存后n秒钟过期
|
||||||
|
.expireAfterWrite(1, TimeUnit.HOURS) |
||||||
|
//设置读写缓存后n秒钟过期,实际很少用到,类似于expireAfterWrite
|
||||||
|
//.expireAfterAccess(17, TimeUnit.SECONDS)
|
||||||
|
.build(); |
||||||
|
|
||||||
|
public static void add(String key, Report report){ |
||||||
|
// 往缓存写数据
|
||||||
|
if(report!=null) { |
||||||
|
loadingCache.put(key, report); |
||||||
|
} |
||||||
|
|
||||||
|
// // 获取value的值,如果key不存在,获取value后再返回
|
||||||
|
// String value = loadingCache.get(key, CaffeineCacheTest::getValueFromDB);
|
||||||
|
// System.out.println(value);
|
||||||
|
// // 删除key
|
||||||
|
// loadingCache.invalidate(key);
|
||||||
|
} |
||||||
|
|
||||||
|
public static void addYear(String key, YearReport report){ |
||||||
|
// 往缓存写数据
|
||||||
|
if(report!=null) { |
||||||
|
loadingYearCache.put(key, report); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public static void addMap(String key, List<Map<String,Object>> report){ |
||||||
|
// 往缓存写数据
|
||||||
|
if(report!=null && !report.isEmpty()) { |
||||||
|
loadingMapCache.put(key, report); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public static List<Map<String,Object>> getMap(String key){ |
||||||
|
// // 获取value的值,如果key不存在,获取value后再返回
|
||||||
|
List<Map<String,Object>> report = loadingMapCache.getIfPresent(key); |
||||||
|
return report; |
||||||
|
} |
||||||
|
|
||||||
|
public static YearReport getYear(String key){ |
||||||
|
// // 获取value的值,如果key不存在,获取value后再返回
|
||||||
|
YearReport report = loadingYearCache.getIfPresent(key); |
||||||
|
return report; |
||||||
|
} |
||||||
|
|
||||||
|
public static Report get(String key){ |
||||||
|
// // 获取value的值,如果key不存在,获取value后再返回
|
||||||
|
Report report = loadingCache.getIfPresent(key); |
||||||
|
return report; |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,273 @@ |
|||||||
|
package com.hnac.hzims.entity; |
||||||
|
|
||||||
|
import com.hnac.hzims.enums.QueryDateTypeEnum; |
||||||
|
import com.hnac.hzims.util.ReportDateUtil; |
||||||
|
import org.apache.commons.lang3.builder.ToStringBuilder; |
||||||
|
|
||||||
|
import java.text.SimpleDateFormat; |
||||||
|
import java.util.*; |
||||||
|
|
||||||
|
/** |
||||||
|
* 提供出去的报表 |
||||||
|
* 提供基础业务数据以及统计数据 |
||||||
|
* |
||||||
|
* @Author: liugang |
||||||
|
* @Date: 2019/7/8 15:03 |
||||||
|
*/ |
||||||
|
public class Report { |
||||||
|
|
||||||
|
/** |
||||||
|
* (唯一标识key,标题名称value), |
||||||
|
*/ |
||||||
|
private List<Map<String, String>> title; |
||||||
|
|
||||||
|
/** |
||||||
|
* 报表基础数据 |
||||||
|
*/ |
||||||
|
private List<ReportUnit> data; |
||||||
|
|
||||||
|
/** |
||||||
|
* 附加报表统计数据 |
||||||
|
*/ |
||||||
|
private List<ReportUnit> standardData; |
||||||
|
|
||||||
|
/** |
||||||
|
* 初始化基础报表数据 |
||||||
|
* 把数据全部置为初始状态("-") |
||||||
|
* |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
public void initBaseReport(int size, Date begin, Date end, QueryDateTypeEnum dtEnum) { |
||||||
|
data = new ArrayList<>(); |
||||||
|
Calendar cal = Calendar.getInstance(); |
||||||
|
cal.setTime(begin); |
||||||
|
SimpleDateFormat sdf = ReportDateUtil.getSimpleDateFormatByType(dtEnum); |
||||||
|
int step = ReportDateUtil.getCalendarType(dtEnum); |
||||||
|
Date temp = cal.getTime(); |
||||||
|
while (temp.before(end)) { |
||||||
|
String key = sdf.format(temp); |
||||||
|
ReportUnit unit = new ReportUnit(); |
||||||
|
unit.init(key, size); |
||||||
|
data.add(unit); |
||||||
|
cal.set(step, cal.get(step) + 1); |
||||||
|
temp = cal.getTime(); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public void initBaseReportMonth(int day,int month){ |
||||||
|
data = new ArrayList<>(); |
||||||
|
for(int i=1;i<=day;i++){ |
||||||
|
String key=i> 9 ? ""+i:"0"+i; |
||||||
|
ReportUnit unit = new ReportUnit(); |
||||||
|
unit.init(key, month); |
||||||
|
data.add(unit); |
||||||
|
} |
||||||
|
ReportUnit unit = new ReportUnit(); |
||||||
|
unit.init("max", month); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("maxDate", month); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("min", month); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("minDate", month); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("avg", month); |
||||||
|
data.add(unit); |
||||||
|
} |
||||||
|
|
||||||
|
public void initBaseReportMaxMin(int size, Date begin, Date end, QueryDateTypeEnum dtEnum) { |
||||||
|
initBaseReport(size, begin, end, dtEnum); |
||||||
|
ReportUnit unit = new ReportUnit(); |
||||||
|
unit.init("max", size); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("maxDate", size); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("min", size); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("minDate", size); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("avg", size); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("range", size);//极差
|
||||||
|
data.add(unit); |
||||||
|
} |
||||||
|
|
||||||
|
public void initBaseReportHistory(int size){ |
||||||
|
data = new ArrayList<>(); |
||||||
|
ReportUnit unit = new ReportUnit(); |
||||||
|
unit.init("max", size); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("maxDate", size); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("min", size); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("minDate", size); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("avg", size); |
||||||
|
data.add(unit); |
||||||
|
|
||||||
|
unit = new ReportUnit(); |
||||||
|
unit.init("range", size);//极差
|
||||||
|
data.add(unit); |
||||||
|
} |
||||||
|
|
||||||
|
public void initCompareBaseReport(int size, Date begin, Date end, QueryDateTypeEnum dtEnum) { |
||||||
|
data = new ArrayList<>(); |
||||||
|
Calendar cal = Calendar.getInstance(); |
||||||
|
cal.setTime(begin); |
||||||
|
SimpleDateFormat sdf = new SimpleDateFormat("MM-dd"); |
||||||
|
int step = ReportDateUtil.getCalendarType(dtEnum); |
||||||
|
Date temp = cal.getTime(); |
||||||
|
while (temp.before(end)) { |
||||||
|
String key = sdf.format(temp); |
||||||
|
ReportUnit unit = new ReportUnit(); |
||||||
|
unit.init(key, size); |
||||||
|
data.add(unit); |
||||||
|
cal.set(step, cal.get(step) + 1); |
||||||
|
temp = cal.getTime(); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public void initBaseRingReport(int size, Date begin, Date end, QueryDateTypeEnum dtEnum){ |
||||||
|
data = new ArrayList<>(); |
||||||
|
Calendar cal = Calendar.getInstance(); |
||||||
|
cal.setTime(begin); |
||||||
|
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH"); |
||||||
|
int step = ReportDateUtil.getCalendarType(dtEnum); |
||||||
|
Date temp = cal.getTime(); |
||||||
|
while (temp.before(end)) { |
||||||
|
String key = sdf.format(temp); |
||||||
|
ReportUnit unit = new ReportUnit(); |
||||||
|
unit.init(key, size); |
||||||
|
data.add(unit); |
||||||
|
cal.set(step, cal.get(step) + 1); |
||||||
|
temp = cal.getTime(); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public void initRingBaseReport(int size, Date begin, Date end, QueryDateTypeEnum dtEnum) { |
||||||
|
data = new ArrayList<>(); |
||||||
|
Calendar cal = Calendar.getInstance(); |
||||||
|
cal.setTime(begin); |
||||||
|
SimpleDateFormat sdf = new SimpleDateFormat("MM-dd"); |
||||||
|
int step = ReportDateUtil.getCalendarType(dtEnum); |
||||||
|
Date temp = cal.getTime(); |
||||||
|
while (temp.before(end)) { |
||||||
|
String key = sdf.format(temp); |
||||||
|
ReportUnit unit = new ReportUnit(); |
||||||
|
unit.init(key, size); |
||||||
|
data.add(unit); |
||||||
|
cal.set(step, cal.get(step) + 1); |
||||||
|
temp = cal.getTime(); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 初始化统计数据 |
||||||
|
* |
||||||
|
* @param size 大小 |
||||||
|
* @param keys 统计数据类型 |
||||||
|
*/ |
||||||
|
public void initStandardReport(int size, String[] keys) { |
||||||
|
standardData = new ArrayList<>(); |
||||||
|
for (String key : keys) { |
||||||
|
standardData.add(initSingleStandard(key, size)); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
private ReportUnit initSingleStandard(String key, int size) { |
||||||
|
ReportUnit unit = new ReportUnit(); |
||||||
|
unit.setKey(key); |
||||||
|
unit.setDataList(new ArrayList<>()); |
||||||
|
for (int i = 0; i < size; i++) { |
||||||
|
unit.getDataList().add("-"); |
||||||
|
} |
||||||
|
return unit; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改唯一标识标定的数据对象(基础数据) |
||||||
|
* |
||||||
|
* @param key 唯一标识 |
||||||
|
* @param index 修改的数据索引 |
||||||
|
* @param value 修改的值 |
||||||
|
*/ |
||||||
|
public void setBaseCell(String key, int index, String value) { |
||||||
|
for (ReportUnit unit : data) { |
||||||
|
if (unit.getKey().equals(key)) { |
||||||
|
unit.getDataList().set(index, value); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改唯一标识标定的数据对象(标准数据) |
||||||
|
* |
||||||
|
* @param key 唯一标识 |
||||||
|
* @param index 修改的数据索引 |
||||||
|
* @param value 修改的值 |
||||||
|
*/ |
||||||
|
public void setStandardCell(String key, int index, String value) { |
||||||
|
for (ReportUnit unit : standardData) { |
||||||
|
if (unit.getKey().equals(key)) { |
||||||
|
unit.getDataList().set(index, value); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public List<Map<String, String>> getTitle() { |
||||||
|
return title; |
||||||
|
} |
||||||
|
|
||||||
|
public void setTitle(List<Map<String, String>> title) { |
||||||
|
this.title = title; |
||||||
|
} |
||||||
|
|
||||||
|
public List<ReportUnit> getData() { |
||||||
|
return data; |
||||||
|
} |
||||||
|
|
||||||
|
public void setData(List<ReportUnit> data) { |
||||||
|
this.data = data; |
||||||
|
} |
||||||
|
|
||||||
|
public List<ReportUnit> getStandardData() { |
||||||
|
return standardData; |
||||||
|
} |
||||||
|
|
||||||
|
public void setStandardData(List<ReportUnit> standardData) { |
||||||
|
this.standardData = standardData; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public String toString() { |
||||||
|
return ToStringBuilder.reflectionToString(this); |
||||||
|
} |
||||||
|
} |
||||||
|
|
@ -0,0 +1,107 @@ |
|||||||
|
package com.hnac.hzims.entity; |
||||||
|
|
||||||
|
/** |
||||||
|
* 封装一个key,value键值对象 |
||||||
|
* 可能是数据库返回查询数据 |
||||||
|
* 也可能是使用的对象由于数据需要置为- |
||||||
|
* 所以采用String存储处理 |
||||||
|
* |
||||||
|
* @Author: liugang |
||||||
|
* @Date: 2019/7/8 16:02 |
||||||
|
*/ |
||||||
|
public class ReportData { |
||||||
|
|
||||||
|
private String keyStr; |
||||||
|
|
||||||
|
private String keyDate; |
||||||
|
|
||||||
|
private String val; |
||||||
|
//测站 或 属性
|
||||||
|
private String stcd; |
||||||
|
//属性名
|
||||||
|
private String name; |
||||||
|
|
||||||
|
private Double value; |
||||||
|
|
||||||
|
private int month=-1; |
||||||
|
|
||||||
|
private String day; |
||||||
|
|
||||||
|
public String getDay() { |
||||||
|
return day; |
||||||
|
} |
||||||
|
|
||||||
|
public void setDay(String day) { |
||||||
|
this.day = day; |
||||||
|
} |
||||||
|
|
||||||
|
public int getMonth() { |
||||||
|
return month; |
||||||
|
} |
||||||
|
|
||||||
|
public void setMonth(int month) { |
||||||
|
this.month = month; |
||||||
|
} |
||||||
|
|
||||||
|
public String getName() { |
||||||
|
return name; |
||||||
|
} |
||||||
|
|
||||||
|
public void setName(String name) { |
||||||
|
this.name = name; |
||||||
|
} |
||||||
|
|
||||||
|
public String getStcd() { |
||||||
|
return stcd; |
||||||
|
} |
||||||
|
|
||||||
|
public void setStcd(String stcd) { |
||||||
|
this.stcd = stcd; |
||||||
|
} |
||||||
|
|
||||||
|
public ReportData() { |
||||||
|
} |
||||||
|
|
||||||
|
public String getKeyDate() { |
||||||
|
return keyDate; |
||||||
|
} |
||||||
|
|
||||||
|
public void setKeyDate(String keyDate) { |
||||||
|
this.keyDate = keyDate; |
||||||
|
} |
||||||
|
|
||||||
|
public ReportData(String keyStr, String val) { |
||||||
|
this.keyStr = keyStr; |
||||||
|
this.val = val; |
||||||
|
} |
||||||
|
|
||||||
|
public String getKeyStr() { |
||||||
|
return keyStr; |
||||||
|
} |
||||||
|
|
||||||
|
public void setKeyStr(String keyStr) { |
||||||
|
this.keyStr = keyStr; |
||||||
|
} |
||||||
|
|
||||||
|
public String getVal() { |
||||||
|
return val; |
||||||
|
} |
||||||
|
|
||||||
|
public void setVal(String val) { |
||||||
|
this.val = val; |
||||||
|
} |
||||||
|
|
||||||
|
public Double getValue() { |
||||||
|
if(val!=null && !"".equals(val.trim())) { |
||||||
|
if(val.indexOf("-")==-1) { |
||||||
|
return Double.valueOf(val); |
||||||
|
} |
||||||
|
} |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
public void setValue(Double value) { |
||||||
|
this.value = value; |
||||||
|
} |
||||||
|
} |
||||||
|
|
@ -0,0 +1,34 @@ |
|||||||
|
package com.hnac.hzims.entity; |
||||||
|
|
||||||
|
import java.util.ArrayList; |
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
public class ReportUnit { |
||||||
|
private String key; |
||||||
|
|
||||||
|
private List<String> dataList; |
||||||
|
|
||||||
|
public void init(String key, int size) { |
||||||
|
this.key = key; |
||||||
|
dataList = new ArrayList<>(); |
||||||
|
for (int i = 0; i < size; i++) { |
||||||
|
dataList.add("-"); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public String getKey() { |
||||||
|
return key; |
||||||
|
} |
||||||
|
|
||||||
|
public void setKey(String key) { |
||||||
|
this.key = key; |
||||||
|
} |
||||||
|
|
||||||
|
public List<String> getDataList() { |
||||||
|
return dataList; |
||||||
|
} |
||||||
|
|
||||||
|
public void setDataList(List<String> dataList) { |
||||||
|
this.dataList = dataList; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,174 @@ |
|||||||
|
package com.hnac.hzims.entity; |
||||||
|
|
||||||
|
import org.apache.commons.lang3.StringUtils; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
import java.util.Objects; |
||||||
|
|
||||||
|
public class StandardData { |
||||||
|
public static final String STANDARD_AVG = "时刻平均值"; |
||||||
|
public static final String STANDARD_MAX = "时刻最大值"; |
||||||
|
public static final String STANDARD_MAX_DATE = "最大值出现的时间"; |
||||||
|
public static final String STANDARD_MIN = "时刻最小值"; |
||||||
|
public static final String STANDARD_MIN_DATE = "最小值出现的时间"; |
||||||
|
public static final String STANDARD_AMPLITUDE = "最大变幅"; |
||||||
|
public static final String STANDARD_SUM = "总量"; |
||||||
|
|
||||||
|
/** |
||||||
|
* 平均值 |
||||||
|
*/ |
||||||
|
private String dataAvg; |
||||||
|
/** |
||||||
|
* 最大值 |
||||||
|
*/ |
||||||
|
private String dataMax; |
||||||
|
/** |
||||||
|
* 最大值时间 |
||||||
|
*/ |
||||||
|
private String dataMaxDate; |
||||||
|
/** |
||||||
|
* 最小值 |
||||||
|
*/ |
||||||
|
private String dataMin; |
||||||
|
/** |
||||||
|
* 最小值出现时间 |
||||||
|
*/ |
||||||
|
private String dataMinDate; |
||||||
|
/** |
||||||
|
* 最大变幅 |
||||||
|
*/ |
||||||
|
private String amplitude; |
||||||
|
/** |
||||||
|
* 总量 |
||||||
|
*/ |
||||||
|
private String dataSum; |
||||||
|
|
||||||
|
/** |
||||||
|
* 比较并处理最大值和最大值日期 |
||||||
|
* |
||||||
|
* @param value 被比较值 |
||||||
|
* @param date 日期 |
||||||
|
*/ |
||||||
|
public void compareDealMax(String value, String date) { |
||||||
|
if (Objects.isNull(dataMax)) { |
||||||
|
dataMax = value; |
||||||
|
dataMaxDate = date; |
||||||
|
} else { |
||||||
|
if (Double.valueOf(dataMax) < Double.valueOf(value)) { |
||||||
|
dataMax = value; |
||||||
|
dataMaxDate = date; |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 比较并处理最小值和最小值日期 |
||||||
|
* |
||||||
|
* @param value 被比较值 |
||||||
|
* @param date 日期 |
||||||
|
*/ |
||||||
|
public void compareDealMin(String value, String date) { |
||||||
|
if (Objects.isNull(dataMin)) { |
||||||
|
dataMin = value; |
||||||
|
dataMinDate = date; |
||||||
|
} else { |
||||||
|
if (Double.valueOf(value) < Double.valueOf(dataMin)) { |
||||||
|
dataMin = value; |
||||||
|
dataMinDate = date; |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 默认计算总和,如有其他逻辑请继承后改写 |
||||||
|
* |
||||||
|
* @param value |
||||||
|
*/ |
||||||
|
public void countSum(String value) { |
||||||
|
if (Objects.isNull(dataSum)) { |
||||||
|
dataSum = value; |
||||||
|
} else { |
||||||
|
dataSum = new BigDecimal(value).add(new BigDecimal(dataSum)).toString(); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 处理空数据,如果数据为空则置- |
||||||
|
*/ |
||||||
|
public void dealNullData() { |
||||||
|
if (StringUtils.isEmpty(dataAvg)) { |
||||||
|
dataAvg = "-"; |
||||||
|
} |
||||||
|
if (StringUtils.isEmpty(dataMax)) { |
||||||
|
dataMax = "-"; |
||||||
|
} |
||||||
|
if (StringUtils.isEmpty(dataMaxDate)) { |
||||||
|
dataMaxDate = "-"; |
||||||
|
} |
||||||
|
if (StringUtils.isEmpty(dataMin)) { |
||||||
|
dataMin = "-"; |
||||||
|
} |
||||||
|
if (StringUtils.isEmpty(dataMinDate)) { |
||||||
|
dataMinDate = "-"; |
||||||
|
} |
||||||
|
if (StringUtils.isEmpty(dataSum)) { |
||||||
|
dataSum = "-"; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
public String getDataAvg() { |
||||||
|
return dataAvg; |
||||||
|
} |
||||||
|
|
||||||
|
public void setDataAvg(String dataAvg) { |
||||||
|
this.dataAvg = dataAvg; |
||||||
|
} |
||||||
|
|
||||||
|
public String getDataMax() { |
||||||
|
return dataMax; |
||||||
|
} |
||||||
|
|
||||||
|
public void setDataMax(String dataMax) { |
||||||
|
this.dataMax = dataMax; |
||||||
|
} |
||||||
|
|
||||||
|
public String getDataMaxDate() { |
||||||
|
return dataMaxDate; |
||||||
|
} |
||||||
|
|
||||||
|
public void setDataMaxDate(String dataMaxDate) { |
||||||
|
this.dataMaxDate = dataMaxDate; |
||||||
|
} |
||||||
|
|
||||||
|
public String getDataMin() { |
||||||
|
return dataMin; |
||||||
|
} |
||||||
|
|
||||||
|
public void setDataMin(String dataMin) { |
||||||
|
this.dataMin = dataMin; |
||||||
|
} |
||||||
|
|
||||||
|
public String getDataMinDate() { |
||||||
|
return dataMinDate; |
||||||
|
} |
||||||
|
|
||||||
|
public void setDataMinDate(String dataMinDate) { |
||||||
|
this.dataMinDate = dataMinDate; |
||||||
|
} |
||||||
|
|
||||||
|
public String getAmplitude() { |
||||||
|
return amplitude; |
||||||
|
} |
||||||
|
|
||||||
|
public void setAmplitude(String amplitude) { |
||||||
|
this.amplitude = amplitude; |
||||||
|
} |
||||||
|
|
||||||
|
public String getDataSum() { |
||||||
|
return dataSum; |
||||||
|
} |
||||||
|
|
||||||
|
public void setDataSum(String dataSum) { |
||||||
|
this.dataSum = dataSum; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,178 @@ |
|||||||
|
package com.hnac.hzims.entity; |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.util.ReportDateUtil; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
import java.util.ArrayList; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Objects; |
||||||
|
|
||||||
|
public class YearReport { |
||||||
|
private static final int MAX_DAY = 31; |
||||||
|
|
||||||
|
private int year; |
||||||
|
private String stcd; |
||||||
|
|
||||||
|
/** |
||||||
|
* 一个List<String>代表1个月 |
||||||
|
* 1个List<String>有31个Double对象代表每一天 |
||||||
|
* 如果没有数据或者天不存在就用-表示 |
||||||
|
*/ |
||||||
|
private List<List<String>> dayList; |
||||||
|
|
||||||
|
/** |
||||||
|
* 一个key代表统计数据类型,value代表每个月统计数据值 |
||||||
|
* 例如<最大值,12个月的数据> |
||||||
|
* 最大值,最小值,平均值等等组成一个List |
||||||
|
*/ |
||||||
|
private List<ReportUnit> monthList; |
||||||
|
|
||||||
|
/** |
||||||
|
* 全年统计数据 |
||||||
|
*/ |
||||||
|
private List<ReportData> yearList; |
||||||
|
|
||||||
|
public void init(String stcd, Integer year) { |
||||||
|
this.year = year; |
||||||
|
this.stcd = stcd; |
||||||
|
initDayList(); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 依据年份初始化年度报表,每个月的每天都加上- |
||||||
|
* 目前不存在的年份也加上了- |
||||||
|
* 如果有需求,则只把有日期的天上加上- |
||||||
|
*/ |
||||||
|
public void initDayList() { |
||||||
|
dayList = new ArrayList<>(); |
||||||
|
for (int month = 0; month <= ReportDateUtil.MAX_MONTH; month++) { |
||||||
|
dayList.add(new ArrayList<>()); |
||||||
|
for (int day = 0; day < MAX_DAY; day++) { |
||||||
|
dayList.get(month).add("-"); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 初始化月份统计数据 |
||||||
|
* |
||||||
|
* @param keys 最大值,最小值,平均值等 |
||||||
|
*/ |
||||||
|
public void initMonthList(String[] keys) { |
||||||
|
monthList = new ArrayList<>(); |
||||||
|
for (String key : keys) { |
||||||
|
ReportUnit reportUnit = new ReportUnit(); |
||||||
|
reportUnit.setKey(key); |
||||||
|
List<String> list = new ArrayList<>(); |
||||||
|
for (int month = 0; month <= ReportDateUtil.MAX_MONTH; month++) { |
||||||
|
list.add("-"); |
||||||
|
} |
||||||
|
reportUnit.setDataList(list); |
||||||
|
monthList.add(reportUnit); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 获取某类型每个月的数据 |
||||||
|
* |
||||||
|
* @param key |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
public ReportUnit getMonthKeyList(String key) { |
||||||
|
for (ReportUnit reportUnit : monthList) { |
||||||
|
if (reportUnit.getKey().equals(key)) { |
||||||
|
return reportUnit; |
||||||
|
} |
||||||
|
} |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 提供出去的设置每天数据 |
||||||
|
* |
||||||
|
* @param month 0-11月 |
||||||
|
* @param day 1-31日 |
||||||
|
* @param value 值 |
||||||
|
*/ |
||||||
|
public void setDayValue(int month, int day, String value) { |
||||||
|
dayList.get(month).set(day - 1, value); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 提供出去的设置每月的统计数据 |
||||||
|
* |
||||||
|
* @param key 类型(最大值,最小值等) |
||||||
|
* @param month 0-11月 |
||||||
|
* @param value 值 |
||||||
|
*/ |
||||||
|
public void setMonthValue(String key, int month, String value) { |
||||||
|
for (ReportUnit reportUnit : monthList) { |
||||||
|
if (reportUnit.getKey().equals(key)) { |
||||||
|
reportUnit.getDataList().set(month, value); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 针对数据进行求和计算 |
||||||
|
* |
||||||
|
* @param list |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
public String sum(List<String> list) { |
||||||
|
String temp = null; |
||||||
|
for (String data : list) { |
||||||
|
if ("-".equals(data) || Double.valueOf(data) <= 0) { |
||||||
|
continue; |
||||||
|
} |
||||||
|
if (Objects.isNull(temp)) { |
||||||
|
temp = data; |
||||||
|
} else { |
||||||
|
temp = new BigDecimal(temp).add(new BigDecimal(data)).toString(); |
||||||
|
} |
||||||
|
} |
||||||
|
return temp; |
||||||
|
} |
||||||
|
|
||||||
|
public int getYear() { |
||||||
|
return year; |
||||||
|
} |
||||||
|
|
||||||
|
public void setYear(int year) { |
||||||
|
this.year = year; |
||||||
|
} |
||||||
|
|
||||||
|
public String getStcd() { |
||||||
|
return stcd; |
||||||
|
} |
||||||
|
|
||||||
|
public void setStcd(String stcd) { |
||||||
|
this.stcd = stcd; |
||||||
|
} |
||||||
|
|
||||||
|
public List<List<String>> getDayList() { |
||||||
|
return dayList; |
||||||
|
} |
||||||
|
|
||||||
|
public void setDayList(List<List<String>> dayList) { |
||||||
|
this.dayList = dayList; |
||||||
|
} |
||||||
|
|
||||||
|
public List<ReportUnit> getMonthList() { |
||||||
|
return monthList; |
||||||
|
} |
||||||
|
|
||||||
|
public void setMonthList(List<ReportUnit> monthList) { |
||||||
|
this.monthList = monthList; |
||||||
|
} |
||||||
|
|
||||||
|
public List<ReportData> getYearList() { |
||||||
|
return yearList; |
||||||
|
} |
||||||
|
|
||||||
|
public void setYearList(List<ReportData> yearList) { |
||||||
|
this.yearList = yearList; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,35 @@ |
|||||||
|
package com.hnac.hzims.enums; |
||||||
|
|
||||||
|
public enum QueryDateTypeEnum { |
||||||
|
//时
|
||||||
|
HOUR("hour"), |
||||||
|
//日
|
||||||
|
DAY("day"), |
||||||
|
//月
|
||||||
|
MONTH("month"), |
||||||
|
//年
|
||||||
|
YEAR("year"); |
||||||
|
|
||||||
|
private String queryDateType; |
||||||
|
|
||||||
|
QueryDateTypeEnum(String queryDateType) { |
||||||
|
this.queryDateType = queryDateType; |
||||||
|
} |
||||||
|
|
||||||
|
public static QueryDateTypeEnum getInstance(String queryDateType) { |
||||||
|
for (QueryDateTypeEnum queryTypeEnum : QueryDateTypeEnum.values()) { |
||||||
|
if (queryTypeEnum.getQueryDateType().equals(queryDateType)) { |
||||||
|
return queryTypeEnum; |
||||||
|
} |
||||||
|
} |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
public String getQueryDateType() { |
||||||
|
return queryDateType; |
||||||
|
} |
||||||
|
|
||||||
|
public void setQueryDateType(String queryDateType) { |
||||||
|
this.queryDateType = queryDateType; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,148 @@ |
|||||||
|
package com.hnac.hzims.util; |
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.entity.ReportData; |
||||||
|
import com.hnac.hzims.enums.QueryDateTypeEnum; |
||||||
|
|
||||||
|
import java.text.SimpleDateFormat; |
||||||
|
import java.util.*; |
||||||
|
|
||||||
|
public class CommonUtil { |
||||||
|
/** |
||||||
|
* 检查查询类型是否符合 |
||||||
|
* |
||||||
|
* @param type |
||||||
|
*/ |
||||||
|
public static QueryDateTypeEnum checkType(String type) { |
||||||
|
QueryDateTypeEnum dtEnum = QueryDateTypeEnum.getInstance(type); |
||||||
|
if (Objects.isNull(dtEnum)) { |
||||||
|
return null; |
||||||
|
} |
||||||
|
return dtEnum; |
||||||
|
} |
||||||
|
|
||||||
|
public static Date getHistoryDate(Date now,int year){ |
||||||
|
Calendar calendar = Calendar.getInstance(); //创建Calendar 的实例
|
||||||
|
calendar.setTime(now); |
||||||
|
calendar.add(Calendar.YEAR, -year);//当前时间减去一年,即一年前的时间
|
||||||
|
return calendar.getTime(); |
||||||
|
} |
||||||
|
|
||||||
|
public static String getLastDayOfMonth(String yearMonth) |
||||||
|
{ |
||||||
|
String[] arr=yearMonth.split("-"); |
||||||
|
Calendar cal = Calendar.getInstance(); |
||||||
|
//设置年份
|
||||||
|
int year=Integer.valueOf(arr[0]); |
||||||
|
cal.set(Calendar.YEAR,year); |
||||||
|
//设置月份
|
||||||
|
int month=Integer.valueOf(arr[1]); |
||||||
|
cal.set(Calendar.MONTH, month-1); |
||||||
|
//获取某月最大天数
|
||||||
|
int lastDay = cal.getActualMaximum(Calendar.DAY_OF_MONTH); |
||||||
|
//设置日历中月份的最大天数
|
||||||
|
cal.set(Calendar.DAY_OF_MONTH, lastDay); |
||||||
|
//格式化日期
|
||||||
|
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); |
||||||
|
String lastDayOfMonth = sdf.format(cal.getTime()); |
||||||
|
|
||||||
|
return lastDayOfMonth; |
||||||
|
} |
||||||
|
|
||||||
|
public static int getYearGap(Date start,Date end){ |
||||||
|
Calendar calStartTime = Calendar.getInstance(); //获取日历实例
|
||||||
|
Calendar calEndTime = Calendar.getInstance(); |
||||||
|
calStartTime.setTime(start); |
||||||
|
calEndTime.setTime(end); |
||||||
|
return calEndTime.get(Calendar.YEAR) - calStartTime.get(Calendar.YEAR); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
public static Map<String, Date> getStartEnd(String beginSpt, String endSpt){ |
||||||
|
|
||||||
|
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); |
||||||
|
Date begin = null; |
||||||
|
Date end = null; |
||||||
|
try { |
||||||
|
begin = sdf.parse(beginSpt); |
||||||
|
end = sdf.parse(endSpt); |
||||||
|
} catch (Exception e) { |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
Map<String,Date> res=new HashMap<>(); |
||||||
|
res.put("begin",begin); |
||||||
|
res.put("end",end); |
||||||
|
return res; |
||||||
|
} |
||||||
|
|
||||||
|
public static String getKey(String str) { |
||||||
|
String[] arr=str.split(" "); |
||||||
|
String arr0=arr[0]; |
||||||
|
String[] keys=arr0.split("-"); |
||||||
|
String key=keys[1]+"-"+keys[2]; |
||||||
|
return key; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
public static List<ReportData> getReportDataBySignage(List<ReportData> timeDataList, String signage){ |
||||||
|
List<ReportData> list=new ArrayList<>(); |
||||||
|
for(ReportData data:timeDataList){ |
||||||
|
if(signage.equals(data.getStcd())){ |
||||||
|
list.add(data); |
||||||
|
} |
||||||
|
} |
||||||
|
return list; |
||||||
|
} |
||||||
|
|
||||||
|
public static int getMonth(String key) { |
||||||
|
String[] keys = key.split("-"); |
||||||
|
String month = keys[1]; |
||||||
|
return Integer.valueOf(month); |
||||||
|
} |
||||||
|
|
||||||
|
public static String getDay(String key) { |
||||||
|
String[] keys = key.split("-"); |
||||||
|
String dayHour = keys[2]; |
||||||
|
String[] dayHourArr=dayHour.split(" "); |
||||||
|
return dayHourArr[0]; |
||||||
|
} |
||||||
|
|
||||||
|
public static int getHour(String key) { |
||||||
|
String[] keys = key.split(" "); |
||||||
|
String dayHour = keys[1]; |
||||||
|
String[] dayHourArr=dayHour.split(":"); |
||||||
|
return Integer.valueOf(dayHourArr[0]); |
||||||
|
} |
||||||
|
|
||||||
|
//0-> s(秒) 1->、m(分)、2->h(小时)3->、d(天)4->、w(周)5->、n(自然月)、6->y(自然年)
|
||||||
|
public static String getKeyBySaveTimeType(String key,Integer saveTimeType){ |
||||||
|
if(saveTimeType.intValue() == 2){//小时
|
||||||
|
int index=key.indexOf(":"); |
||||||
|
if(index>0) { |
||||||
|
String tmp = key.substring(0, index); |
||||||
|
return tmp; |
||||||
|
} |
||||||
|
} |
||||||
|
if(saveTimeType.intValue() == 3){//天
|
||||||
|
String[] arr=key.split(" "); |
||||||
|
return arr[0]; |
||||||
|
} |
||||||
|
if(saveTimeType.intValue() == 5){//月
|
||||||
|
int index=key.lastIndexOf("-"); |
||||||
|
if(index>0) { |
||||||
|
String tmp = key.substring(0, index); |
||||||
|
return tmp; |
||||||
|
} |
||||||
|
} |
||||||
|
if(saveTimeType.intValue() == 6){//年
|
||||||
|
int index = key.indexOf("-"); |
||||||
|
if(index>0) { |
||||||
|
String tmp = key.substring(0, index); |
||||||
|
return tmp; |
||||||
|
} |
||||||
|
} |
||||||
|
return key; |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,116 @@ |
|||||||
|
package com.hnac.hzims.util; |
||||||
|
|
||||||
|
import com.hnac.hzims.enums.QueryDateTypeEnum; |
||||||
|
import org.apache.commons.lang3.StringUtils; |
||||||
|
|
||||||
|
import java.text.SimpleDateFormat; |
||||||
|
import java.util.Calendar; |
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
public class ReportDateUtil { |
||||||
|
public static final int MAX_MONTH = 11; |
||||||
|
|
||||||
|
public static int getDateDiff(Date begin, Date end, int step) { |
||||||
|
//初始化差距
|
||||||
|
int diff = 0; |
||||||
|
Calendar cal = Calendar.getInstance(); |
||||||
|
cal.setTime(begin); |
||||||
|
Date temp = cal.getTime(); |
||||||
|
while (temp.before(end)) { |
||||||
|
diff++; |
||||||
|
cal.set(step, cal.get(step) + 1); |
||||||
|
temp = cal.getTime(); |
||||||
|
} |
||||||
|
return diff; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 获取日期 |
||||||
|
* |
||||||
|
* @param year 年 |
||||||
|
* @param month 月(0-11) |
||||||
|
* @param day 日(1-31) |
||||||
|
* @param hour 时 |
||||||
|
* @param minute 分 |
||||||
|
* @param second 秒 |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
public static Date getDate(int year, int month, int day, int hour, int minute, int second) { |
||||||
|
Calendar calendar = Calendar.getInstance(); |
||||||
|
calendar.set(Calendar.YEAR, year); |
||||||
|
calendar.set(Calendar.MONTH, month); |
||||||
|
calendar.set(Calendar.DAY_OF_MONTH, day); |
||||||
|
calendar.set(Calendar.HOUR_OF_DAY, hour); |
||||||
|
calendar.set(Calendar.MINUTE, minute); |
||||||
|
calendar.set(Calendar.SECOND, second); |
||||||
|
calendar.set(Calendar.MILLISECOND, 0); |
||||||
|
Date date = calendar.getTime(); |
||||||
|
return date; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 获取下一个日期(下一年,下一月,下一日等) |
||||||
|
* |
||||||
|
* @param curDate |
||||||
|
* @param field |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
public static Date getNextDate(Date curDate, int field) { |
||||||
|
Calendar calendar = Calendar.getInstance(); |
||||||
|
calendar.setTime(curDate); |
||||||
|
calendar.set(field, calendar.get(field) + 1); |
||||||
|
return calendar.getTime(); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 依据查询类型获取时间格式类型 |
||||||
|
* |
||||||
|
* @param dtEnum |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
public static SimpleDateFormat getSimpleDateFormatByType(QueryDateTypeEnum dtEnum) { |
||||||
|
switch (dtEnum) { |
||||||
|
case HOUR: |
||||||
|
return new SimpleDateFormat("yyyy-MM-dd HH"); |
||||||
|
case DAY: |
||||||
|
return new SimpleDateFormat("yyyy-MM-dd"); |
||||||
|
case MONTH: |
||||||
|
return new SimpleDateFormat("yyyy-MM"); |
||||||
|
case YEAR: |
||||||
|
return new SimpleDateFormat("yyyy"); |
||||||
|
default: |
||||||
|
return new SimpleDateFormat("yyyy-MM-dd"); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 依据查询类型获取日历类型 |
||||||
|
* |
||||||
|
* @param dtEnum |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
public static int getCalendarType(QueryDateTypeEnum dtEnum) { |
||||||
|
switch (dtEnum) { |
||||||
|
case HOUR: |
||||||
|
return Calendar.HOUR_OF_DAY; |
||||||
|
case DAY: |
||||||
|
return Calendar.DAY_OF_MONTH; |
||||||
|
case MONTH: |
||||||
|
return Calendar.MONTH; |
||||||
|
case YEAR: |
||||||
|
return Calendar.YEAR; |
||||||
|
default: |
||||||
|
return Calendar.DAY_OF_MONTH; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/*** |
||||||
|
* 年月日时分秒 转年月日 |
||||||
|
*/ |
||||||
|
public static String getYMDStr(String ymd) { |
||||||
|
if(StringUtils.isNotEmpty(ymd)){ |
||||||
|
return ymd.substring(0,11); |
||||||
|
} |
||||||
|
return ""; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,20 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<project xmlns="http://maven.apache.org/POM/4.0.0" |
||||||
|
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" |
||||||
|
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> |
||||||
|
<modelVersion>4.0.0</modelVersion> |
||||||
|
<parent> |
||||||
|
<groupId>com.hnac.hzims</groupId> |
||||||
|
<artifactId>hzims-service-api</artifactId> |
||||||
|
<version>4.0.0-SNAPSHOT</version> |
||||||
|
</parent> |
||||||
|
|
||||||
|
<artifactId>dam-safety-api</artifactId> |
||||||
|
|
||||||
|
<properties> |
||||||
|
<maven.compiler.source>8</maven.compiler.source> |
||||||
|
<maven.compiler.target>8</maven.compiler.target> |
||||||
|
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> |
||||||
|
</properties> |
||||||
|
|
||||||
|
</project> |
@ -0,0 +1,37 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import org.springblade.core.mp.base.BaseEntity; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
|
||||||
|
/** |
||||||
|
* 大坝信息表 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("t_building") |
||||||
|
public class TBuilding extends BaseEntity { |
||||||
|
@ApiModelProperty("水库编号") |
||||||
|
private String rscd; |
||||||
|
@ApiModelProperty("建筑物名称") |
||||||
|
private String name; |
||||||
|
@ApiModelProperty("建筑物分级路径") |
||||||
|
private String ipath; |
||||||
|
@ApiModelProperty("简介") |
||||||
|
private String brief; |
||||||
|
@ApiModelProperty("图片地址") |
||||||
|
private String attach; |
||||||
|
@ApiModelProperty("文件地址") |
||||||
|
private String filePath; |
||||||
|
|
||||||
|
//大坝信息
|
||||||
|
@ApiModelProperty("坝类型") |
||||||
|
private String type;//坝类型
|
||||||
|
@ApiModelProperty("地址") |
||||||
|
private String address;//地址
|
||||||
|
@ApiModelProperty("坝高") |
||||||
|
private BigDecimal damHeight;//坝高
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,38 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
import org.springblade.core.mp.base.BaseEntity; |
||||||
|
|
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
/** |
||||||
|
* 工程物信息表 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("t_projinfo") |
||||||
|
@EqualsAndHashCode(callSuper = true) |
||||||
|
public class TProjInfo extends BaseEntity { |
||||||
|
@ApiModelProperty("水库编码") |
||||||
|
private String rscd; |
||||||
|
@ApiModelProperty("工程名称") |
||||||
|
private String projName; |
||||||
|
@ApiModelProperty("工程编号") |
||||||
|
private String projCode; |
||||||
|
@ApiModelProperty("开工日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date startDate; |
||||||
|
@ApiModelProperty("竣工日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date completeDate; |
||||||
|
@ApiModelProperty("蓄水日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date storeWaterDate; |
||||||
|
@ApiModelProperty("工程所在位置") |
||||||
|
private String location; |
||||||
|
@ApiModelProperty("备注") |
||||||
|
private String rm; |
||||||
|
} |
@ -0,0 +1,34 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.IdType; |
||||||
|
import com.baomidou.mybatisplus.annotation.TableId; |
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
|
||||||
|
import java.io.Serializable; |
||||||
|
import java.math.BigDecimal; |
||||||
|
|
||||||
|
/** |
||||||
|
* 大坝断面数据配置表 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("t_section_config") |
||||||
|
public class TSectionConfig implements Serializable { |
||||||
|
@ApiModelProperty("渗流量测点编号") |
||||||
|
@TableId(type = IdType.INPUT) |
||||||
|
private String stcd; |
||||||
|
@ApiModelProperty("断面编号") |
||||||
|
private String sectionNo; |
||||||
|
@ApiModelProperty("是否显示浸润线") |
||||||
|
private String opinion; |
||||||
|
@ApiModelProperty("x轴") |
||||||
|
private BigDecimal xzhou; |
||||||
|
@ApiModelProperty("y轴最大值") |
||||||
|
private BigDecimal ymax; |
||||||
|
@ApiModelProperty("y轴最小值") |
||||||
|
private BigDecimal ymin; |
||||||
|
@ApiModelProperty("警戒值") |
||||||
|
private BigDecimal warm; |
||||||
|
|
||||||
|
} |
@ -0,0 +1,31 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.IdType; |
||||||
|
import com.baomidou.mybatisplus.annotation.TableId; |
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
/** |
||||||
|
* 断面特征点信息表 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("wrp_dams_b") |
||||||
|
public class WrpDamsB{ |
||||||
|
@ApiModelProperty("断面编号") |
||||||
|
private String damcd; |
||||||
|
@ApiModelProperty("特征点编号") |
||||||
|
@TableId(type= IdType.INPUT) |
||||||
|
private String damscd; |
||||||
|
@ApiModelProperty("特征点名称") |
||||||
|
private String damsnm; |
||||||
|
@ApiModelProperty("起点距(m)") |
||||||
|
private BigDecimal redi; |
||||||
|
@ApiModelProperty("高程(m)") |
||||||
|
private BigDecimal poel; |
||||||
|
@ApiModelProperty("更新时间") |
||||||
|
private Date dtuptm; |
||||||
|
} |
@ -0,0 +1,56 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
import org.springblade.core.mp.base.BaseEntity; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
/** |
||||||
|
*水平位移监测测点 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("wrp_dfr_srhrdsmp") |
||||||
|
@EqualsAndHashCode(callSuper = true) |
||||||
|
public class WrpDfrSrhrdsmp extends BaseEntity { |
||||||
|
@ApiModelProperty("测站编码") |
||||||
|
private String stcd; |
||||||
|
@ApiModelProperty("断面编号") |
||||||
|
private String damcd; |
||||||
|
@ApiModelProperty("测点编号") |
||||||
|
private String mpcd; |
||||||
|
@ApiModelProperty("桩号") |
||||||
|
private String ch; |
||||||
|
@ApiModelProperty("轴距") |
||||||
|
private BigDecimal ofax; |
||||||
|
@ApiModelProperty("高程") |
||||||
|
private BigDecimal el; |
||||||
|
@ApiModelProperty("基准值 X") |
||||||
|
private BigDecimal stvlx; |
||||||
|
@ApiModelProperty("基准值 Y") |
||||||
|
private BigDecimal stvly; |
||||||
|
@ApiModelProperty("位移阈值") |
||||||
|
private BigDecimal xyhrds; |
||||||
|
@ApiModelProperty("型式") |
||||||
|
private String tp; |
||||||
|
@ApiModelProperty("基础情况") |
||||||
|
private String bsin; |
||||||
|
@ApiModelProperty("安装日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date indt; |
||||||
|
@ApiModelProperty("测定日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date dtdt; |
||||||
|
@ApiModelProperty("仪器编号") |
||||||
|
private String dvcd; |
||||||
|
@ApiModelProperty("经度") |
||||||
|
private BigDecimal eslg; |
||||||
|
@ApiModelProperty("纬度") |
||||||
|
private BigDecimal nrlt; |
||||||
|
@ApiModelProperty("备注") |
||||||
|
private String rm; |
||||||
|
} |
@ -0,0 +1,55 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
import org.springblade.core.mp.base.BaseEntity; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
/** |
||||||
|
* 监测基点表 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("wrp_dfr_srvrdsbp") |
||||||
|
@EqualsAndHashCode(callSuper = true) |
||||||
|
public class WrpDfrSrvrdsbp extends BaseEntity { |
||||||
|
@ApiModelProperty("水库代码") |
||||||
|
private String rscd; |
||||||
|
@ApiModelProperty("水工建筑物id") |
||||||
|
private Long hycncd; |
||||||
|
@ApiModelProperty("基点编号") |
||||||
|
private String bpcd; |
||||||
|
@ApiModelProperty("考证信息日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date txindt; |
||||||
|
@ApiModelProperty("基点类型") |
||||||
|
private String bptp; |
||||||
|
@ApiModelProperty("桩号") |
||||||
|
private String ch; |
||||||
|
@ApiModelProperty("轴距") |
||||||
|
private BigDecimal ofax; |
||||||
|
@ApiModelProperty("高程") |
||||||
|
private BigDecimal el; |
||||||
|
@ApiModelProperty("型式") |
||||||
|
private String tp; |
||||||
|
@ApiModelProperty("基础情况") |
||||||
|
private String bsin; |
||||||
|
@ApiModelProperty("安装日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date indt; |
||||||
|
@ApiModelProperty("测定日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date dtdt; |
||||||
|
@ApiModelProperty("经度") |
||||||
|
private BigDecimal lgtd; |
||||||
|
@ApiModelProperty("纬度") |
||||||
|
private BigDecimal lttd; |
||||||
|
@ApiModelProperty("工作状态") |
||||||
|
private String wkcn; |
||||||
|
@ApiModelProperty("备注") |
||||||
|
private String rm; |
||||||
|
} |
@ -0,0 +1,53 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
import org.springblade.core.mp.base.BaseEntity; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
/** |
||||||
|
* 垂直位移监测测点 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("wrp_dfr_srvrdsmp") |
||||||
|
@EqualsAndHashCode(callSuper = true) |
||||||
|
public class WrpDfrSrvrdsmp extends BaseEntity { |
||||||
|
@ApiModelProperty("测站编码") |
||||||
|
private String stcd; |
||||||
|
@ApiModelProperty("断面编号") |
||||||
|
private String damcd; |
||||||
|
@ApiModelProperty("测点编号") |
||||||
|
private String mpcd; |
||||||
|
@ApiModelProperty("桩号") |
||||||
|
private String ch; |
||||||
|
@ApiModelProperty("轴距") |
||||||
|
private BigDecimal ofax; |
||||||
|
@ApiModelProperty("初始高程") |
||||||
|
private BigDecimal inel; |
||||||
|
@ApiModelProperty("位移阈值") |
||||||
|
private BigDecimal vrds; |
||||||
|
@ApiModelProperty("型式") |
||||||
|
private String tp; |
||||||
|
@ApiModelProperty("基础情况") |
||||||
|
private String bsin; |
||||||
|
@ApiModelProperty("安装日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date indt; |
||||||
|
@ApiModelProperty("测定日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date dtdt; |
||||||
|
@ApiModelProperty("仪器编号") |
||||||
|
private String dvcd; |
||||||
|
@ApiModelProperty("经度") |
||||||
|
private BigDecimal eslg; |
||||||
|
@ApiModelProperty("纬度") |
||||||
|
private BigDecimal nrlt; |
||||||
|
@ApiModelProperty("备注") |
||||||
|
private String rm; |
||||||
|
|
||||||
|
} |
@ -0,0 +1,38 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
import org.springblade.core.mp.base.BaseEntity; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
|
||||||
|
/** |
||||||
|
* 大坝断面信息表 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("wrp_section_b") |
||||||
|
@EqualsAndHashCode(callSuper = true) |
||||||
|
public class WrpSectionB extends BaseEntity { |
||||||
|
@ApiModelProperty("水库编号") |
||||||
|
private String rscd; |
||||||
|
@ApiModelProperty("建筑物id") |
||||||
|
private Long buildingId; |
||||||
|
@ApiModelProperty("断面编码") |
||||||
|
private String damcd; |
||||||
|
@ApiModelProperty("断面名称") |
||||||
|
private String damnm; |
||||||
|
@ApiModelProperty("防渗墙类型") |
||||||
|
private String wallType; |
||||||
|
@ApiModelProperty("断面长度") |
||||||
|
private BigDecimal damlen; |
||||||
|
@ApiModelProperty("断面宽度") |
||||||
|
private BigDecimal damwd; |
||||||
|
@ApiModelProperty("备注") |
||||||
|
private String rm; |
||||||
|
@ApiModelProperty("预留字段") |
||||||
|
private String text; |
||||||
|
@ApiModelProperty("图片地址") |
||||||
|
private String attach; |
||||||
|
} |
@ -0,0 +1,57 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
import org.springblade.core.mp.base.BaseEntity; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
|
||||||
|
/** |
||||||
|
* 测压管测点 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("wrp_spg_pztb") |
||||||
|
@EqualsAndHashCode(callSuper = true) |
||||||
|
public class WrpSpgPztb extends BaseEntity { |
||||||
|
@ApiModelProperty("测站编码") |
||||||
|
private String stcd; |
||||||
|
@ApiModelProperty("测点编号") |
||||||
|
private String mpcd; |
||||||
|
@ApiModelProperty("断面编号") |
||||||
|
private String damcd; |
||||||
|
@ApiModelProperty("桩号") |
||||||
|
private String ch; |
||||||
|
@ApiModelProperty("坝轴距") |
||||||
|
private BigDecimal ofax; |
||||||
|
@ApiModelProperty("监测部位") |
||||||
|
private String msps; |
||||||
|
|
||||||
|
@ApiModelProperty("监测类型") |
||||||
|
private String mstp; |
||||||
|
@ApiModelProperty("安装高程") |
||||||
|
private BigDecimal el; |
||||||
|
@ApiModelProperty("进水段底高程") |
||||||
|
private BigDecimal ipbtel; |
||||||
|
@ApiModelProperty("进水段顶高程") |
||||||
|
private BigDecimal iptpel; |
||||||
|
@ApiModelProperty("管口高程") |
||||||
|
private BigDecimal tbtpel; |
||||||
|
@ApiModelProperty("管底高程") |
||||||
|
private BigDecimal tbbtel; |
||||||
|
@ApiModelProperty("水位阈值高程") |
||||||
|
private BigDecimal pztbtel; |
||||||
|
|
||||||
|
@ApiModelProperty("仪器编号") |
||||||
|
private String dvcd; |
||||||
|
@ApiModelProperty("经度") |
||||||
|
private BigDecimal eslg; |
||||||
|
@ApiModelProperty("纬度") |
||||||
|
private BigDecimal nrlt; |
||||||
|
@ApiModelProperty("备注") |
||||||
|
private String rm; |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,52 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
import org.springblade.core.mp.base.BaseEntity; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
/** |
||||||
|
* 渗流压力测点信息表 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("wrp_spg_spprmp") |
||||||
|
@EqualsAndHashCode(callSuper = true) |
||||||
|
public class WrpSpgSpprmp extends BaseEntity { |
||||||
|
@ApiModelProperty("测站编码") |
||||||
|
private String stcd; |
||||||
|
@ApiModelProperty("断面编码") |
||||||
|
private String damcd; |
||||||
|
@ApiModelProperty("测点编号") |
||||||
|
private String mpcd; |
||||||
|
@ApiModelProperty("考证信息日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date txindt; |
||||||
|
@ApiModelProperty("桩号") |
||||||
|
private String ch; |
||||||
|
@ApiModelProperty("轴距") |
||||||
|
private BigDecimal ofax; |
||||||
|
@ApiModelProperty("高程") |
||||||
|
private BigDecimal el; |
||||||
|
@ApiModelProperty("监测类型") |
||||||
|
private String mstp; |
||||||
|
@ApiModelProperty("透水段底高程") |
||||||
|
private BigDecimal pmbtel; |
||||||
|
@ApiModelProperty("透水段顶高程") |
||||||
|
private BigDecimal pmtpel; |
||||||
|
@ApiModelProperty("安装日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date indt; |
||||||
|
@ApiModelProperty("仪器出厂编号") |
||||||
|
private String dvfccd; |
||||||
|
@ApiModelProperty("工作状态") |
||||||
|
private String wkcn; |
||||||
|
@ApiModelProperty("备注") |
||||||
|
private String rm; |
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,45 @@ |
|||||||
|
package com.hnac.hzims.damsafety.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
import org.springblade.core.mp.base.BaseEntity; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
/** |
||||||
|
* 渗流量测点 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("wrp_spg_spqnmp") |
||||||
|
@EqualsAndHashCode(callSuper = true) |
||||||
|
public class WrpSpgSpqnmp extends BaseEntity { |
||||||
|
@ApiModelProperty("测站编码") |
||||||
|
private String stcd; |
||||||
|
@ApiModelProperty("测点编号") |
||||||
|
private String mpcd; |
||||||
|
@ApiModelProperty("断面编号") |
||||||
|
private String damcd; |
||||||
|
@ApiModelProperty("桩号") |
||||||
|
private String ch; |
||||||
|
@ApiModelProperty("轴距") |
||||||
|
private BigDecimal ofax; |
||||||
|
@ApiModelProperty("高程") |
||||||
|
private BigDecimal el; |
||||||
|
@ApiModelProperty("渗流阈值") |
||||||
|
private BigDecimal spprwl; |
||||||
|
@ApiModelProperty("安装日期") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd") |
||||||
|
private Date indt; |
||||||
|
@ApiModelProperty("仪器编号") |
||||||
|
private String dvcd; |
||||||
|
@ApiModelProperty("经度") |
||||||
|
private BigDecimal eslg; |
||||||
|
@ApiModelProperty("纬度") |
||||||
|
private BigDecimal nrlt; |
||||||
|
@ApiModelProperty("备注") |
||||||
|
private String rm; |
||||||
|
} |
@ -0,0 +1,26 @@ |
|||||||
|
package com.hnac.hzims.damsafety.feign; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.vo.WrpSectionBVO; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.cloud.openfeign.FeignClient; |
||||||
|
import org.springframework.web.bind.annotation.GetMapping; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@FeignClient( |
||||||
|
value = "dam-safety", |
||||||
|
url = "${feign.dam-safety:}" |
||||||
|
) |
||||||
|
public interface IWrpSectionBClient { |
||||||
|
String API_PREFIX = "/wrpSectionBClient"; |
||||||
|
String LIST = API_PREFIX + "/list"; |
||||||
|
|
||||||
|
/** |
||||||
|
* 获取断面列表 |
||||||
|
* |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@GetMapping(LIST) |
||||||
|
R<List<WrpSectionBVO>> list(Map<String, Object> param); |
||||||
|
} |
@ -0,0 +1,8 @@ |
|||||||
|
package com.hnac.hzims.damsafety.vo; |
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.TBuilding; |
||||||
|
|
||||||
|
public class TBuildingVo extends TBuilding { |
||||||
|
|
||||||
|
} |
@ -0,0 +1,8 @@ |
|||||||
|
package com.hnac.hzims.damsafety.vo; |
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.TProjInfo; |
||||||
|
|
||||||
|
public class TProjInfoVo extends TProjInfo { |
||||||
|
|
||||||
|
} |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.vo; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrhrdsmp; |
||||||
|
|
||||||
|
public class WrpDfrSrhrdsmpVo extends WrpDfrSrhrdsmp { |
||||||
|
|
||||||
|
} |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.vo; |
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsbp; |
||||||
|
|
||||||
|
public class WrpDfrSrvrdsbpVo extends WrpDfrSrvrdsbp { |
||||||
|
} |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.vo; |
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsmp; |
||||||
|
|
||||||
|
public class WrpDfrSrvrdsmpVo extends WrpDfrSrvrdsmp { |
||||||
|
} |
@ -0,0 +1,16 @@ |
|||||||
|
package com.hnac.hzims.damsafety.vo; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsbp; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSectionB; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
|
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
@Data |
||||||
|
@EqualsAndHashCode(callSuper = true) |
||||||
|
public class WrpSectionBVO extends WrpSectionB { |
||||||
|
//测点集合
|
||||||
|
private List<WrpDfrSrvrdsbp> points; |
||||||
|
} |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.vo; |
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgPztb; |
||||||
|
|
||||||
|
public class WrpSpgPztbVo extends WrpSpgPztb { |
||||||
|
} |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.vo; |
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgSpprmp; |
||||||
|
|
||||||
|
public class WrpSpgSpprmpVo extends WrpSpgSpprmp { |
||||||
|
} |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.vo; |
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgSpqnmp; |
||||||
|
|
||||||
|
public class WrpSpgSpqnmpVo extends WrpSpgSpqnmp { |
||||||
|
} |
@ -0,0 +1,67 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<project xmlns="http://maven.apache.org/POM/4.0.0" |
||||||
|
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" |
||||||
|
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> |
||||||
|
<modelVersion>4.0.0</modelVersion> |
||||||
|
<parent> |
||||||
|
<groupId>com.hnac.hzims</groupId> |
||||||
|
<artifactId>hzims-service</artifactId> |
||||||
|
<version>4.0.0-SNAPSHOT</version> |
||||||
|
</parent> |
||||||
|
|
||||||
|
<artifactId>dam-safety</artifactId> |
||||||
|
|
||||||
|
<properties> |
||||||
|
<maven.compiler.source>8</maven.compiler.source> |
||||||
|
<maven.compiler.target>8</maven.compiler.target> |
||||||
|
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding> |
||||||
|
</properties> |
||||||
|
|
||||||
|
|
||||||
|
<dependencies> |
||||||
|
<dependency> |
||||||
|
<groupId>com.hnac.hzims</groupId> |
||||||
|
<artifactId>dam-safety-api</artifactId> |
||||||
|
<version>${hzims.project.version}</version> |
||||||
|
</dependency> |
||||||
|
<dependency> |
||||||
|
<groupId>org.springblade</groupId> |
||||||
|
<artifactId>blade-core-boot</artifactId> |
||||||
|
</dependency> |
||||||
|
<dependency> |
||||||
|
<groupId>org.springblade</groupId> |
||||||
|
<artifactId>blade-starter-swagger</artifactId> |
||||||
|
</dependency> |
||||||
|
<dependency> |
||||||
|
<groupId>org.springblade</groupId> |
||||||
|
<artifactId>blade-starter-oss-all</artifactId> |
||||||
|
</dependency> |
||||||
|
<dependency> |
||||||
|
<groupId>com.baomidou</groupId> |
||||||
|
<artifactId>dynamic-datasource-spring-boot-starter</artifactId> |
||||||
|
</dependency> |
||||||
|
<dependency> |
||||||
|
<groupId>org.springblade</groupId> |
||||||
|
<artifactId>blade-core-test</artifactId> |
||||||
|
<scope>test</scope> |
||||||
|
</dependency> |
||||||
|
<dependency> |
||||||
|
<groupId>org.springblade</groupId> |
||||||
|
<artifactId>blade-core-auto</artifactId> |
||||||
|
<scope>compile</scope> |
||||||
|
</dependency> |
||||||
|
<dependency> |
||||||
|
<groupId>com.hnac.hzinfo.data</groupId> |
||||||
|
<artifactId>hzinfo-data-sdk</artifactId> |
||||||
|
</dependency> |
||||||
|
<dependency> |
||||||
|
<groupId>org.springblade</groupId> |
||||||
|
<artifactId>blade-common</artifactId> |
||||||
|
</dependency> |
||||||
|
<dependency> |
||||||
|
<groupId>com.hnac.hzims</groupId> |
||||||
|
<artifactId>common-api</artifactId> |
||||||
|
</dependency> |
||||||
|
</dependencies> |
||||||
|
|
||||||
|
</project> |
@ -0,0 +1,40 @@ |
|||||||
|
package com.hnac.hzims.damsafety; |
||||||
|
|
||||||
|
import org.mybatis.spring.annotation.MapperScan; |
||||||
|
import org.springblade.core.cloud.feign.EnableBladeFeign; |
||||||
|
import org.springblade.core.launch.BladeApplication; |
||||||
|
import org.springframework.boot.builder.SpringApplicationBuilder; |
||||||
|
import org.springframework.boot.web.servlet.support.SpringBootServletInitializer; |
||||||
|
import org.springframework.cloud.client.SpringCloudApplication; |
||||||
|
import org.springframework.context.annotation.ComponentScan; |
||||||
|
import org.springframework.scheduling.annotation.EnableScheduling; |
||||||
|
|
||||||
|
//大坝安全模块
|
||||||
|
@EnableBladeFeign(basePackages = {"org.springblade","com.hnac"}) |
||||||
|
@SpringCloudApplication |
||||||
|
@MapperScan("com.hnac.hzims.**.mapper.**") |
||||||
|
@EnableScheduling |
||||||
|
@ComponentScan(basePackages = {"com.hnac.hzims.*"}) |
||||||
|
public class DamSafetyApplication extends SpringBootServletInitializer { |
||||||
|
public final static String APP_NAME = "dam-safety"; |
||||||
|
|
||||||
|
|
||||||
|
static { |
||||||
|
System.setProperty("spring.cloud.nacos.discovery.server-addr", "http://192.168.65.105:8848"); |
||||||
|
System.setProperty("spring.cloud.nacos.config.server-addr", "http://192.168.65.105:8848"); |
||||||
|
System.setProperty("spring.cloud.nacos.username", "nacos"); |
||||||
|
System.setProperty("spring.cloud.nacos.password", "nacos"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
public static void main(String[] args) { |
||||||
|
// System.setProperty("nacos.standalone", "true");
|
||||||
|
BladeApplication.run(APP_NAME, DamSafetyApplication.class, args); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
protected SpringApplicationBuilder configure(SpringApplicationBuilder builder) { |
||||||
|
return BladeApplication.createSpringApplicationBuilder(builder, APP_NAME, DamSafetyApplication.class); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,211 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.TProjInfo; |
||||||
|
import com.hnac.hzims.damsafety.service.IDamSafetyDataService; |
||||||
|
import com.hnac.hzims.damsafety.service.ITProjInfoService; |
||||||
|
import com.hnac.hzims.entity.Report; |
||||||
|
import com.hnac.hzims.enums.QueryDateTypeEnum; |
||||||
|
import com.hnac.hzims.util.CommonUtil; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.domain.FieldsData; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.vo.AnalyzeInstanceFieldVO; |
||||||
|
import com.hnac.hzinfo.sdk.analyse.vo.DeviceSinglePropsValueVO; |
||||||
|
import io.swagger.annotations.Api; |
||||||
|
import io.swagger.annotations.ApiImplicitParam; |
||||||
|
import io.swagger.annotations.ApiImplicitParams; |
||||||
|
import io.swagger.annotations.ApiOperation; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.web.bind.annotation.RequestMapping; |
||||||
|
import org.springframework.web.bind.annotation.RequestMethod; |
||||||
|
import org.springframework.web.bind.annotation.RestController; |
||||||
|
|
||||||
|
import java.util.*; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/data") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "大坝安全数据查询接口", tags = "大坝安全数据查询接口") |
||||||
|
public class DamSafetyDataController extends BladeController implements CacheNames { |
||||||
|
private final IDamSafetyDataService damSafetyDataService; |
||||||
|
|
||||||
|
|
||||||
|
@ApiOperation("实时数据接口") |
||||||
|
@RequestMapping(value = "/getRealData", method = RequestMethod.GET) |
||||||
|
public R getRealData(String deviceCode){ |
||||||
|
List<FieldsData> data = damSafetyDataService.getRealData(deviceCode); |
||||||
|
return R.data(data,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
//过程线
|
||||||
|
@ApiOperation("基础数据报表,数据查询和统计分析使用接口") |
||||||
|
@RequestMapping(value = "/getReportData", method = RequestMethod.GET) |
||||||
|
public R getReportData(String deviceCode, String beginSpt, String endSpt, String type, Integer accessRules,String col){ |
||||||
|
Map<String, Date> res= CommonUtil.getStartEnd(beginSpt,endSpt); |
||||||
|
if(res == null){ |
||||||
|
return R.data(500,false, "日期格式错误"); |
||||||
|
} |
||||||
|
//查询基础数据
|
||||||
|
String[] deviceCodes=deviceCode.split(","); |
||||||
|
Report report = damSafetyDataService.getReportData(Arrays.asList(deviceCodes),type,accessRules, res.get("begin"), res.get("end"),col); |
||||||
|
return R.data(report,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
@ApiOperation("大坝浸润线") |
||||||
|
@RequestMapping(value = "/getSeepageLine", method = RequestMethod.GET) |
||||||
|
public R getSeepageLine(String deviceCode, String beginSpt, String endSpt,String col){ |
||||||
|
Map<String, Date> res= CommonUtil.getStartEnd(beginSpt,endSpt); |
||||||
|
if(res == null){ |
||||||
|
return R.data(500,false, "日期格式错误"); |
||||||
|
} |
||||||
|
//查询基础数据
|
||||||
|
String[] deviceCodes=deviceCode.split(","); |
||||||
|
List<DeviceSinglePropsValueVO> data = damSafetyDataService.getSeepageLine(Arrays.asList(deviceCodes), res.get("begin"), res.get("end"),col); |
||||||
|
return R.data(data,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
@ApiOperation("用于渗压水位过程线,相同业务场景也可用此接口") |
||||||
|
@RequestMapping(value = "/getReportDataByDeviceCode", method = RequestMethod.GET) |
||||||
|
public R getReportData(String deviceCode, String beginSpt, String endSpt, String type, Integer accessRules){ |
||||||
|
Map<String, Date> res= CommonUtil.getStartEnd(beginSpt,endSpt); |
||||||
|
if(res == null){ |
||||||
|
return R.data(500,false, "日期格式错误"); |
||||||
|
} |
||||||
|
//查询基础数据
|
||||||
|
Report report = damSafetyDataService.getReportDataByDeviceCode(deviceCode,type,accessRules, res.get("begin"), res.get("end")); |
||||||
|
return R.data(report,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
@ApiOperation("表头列") |
||||||
|
@RequestMapping(value = "/getTableHead", method = RequestMethod.GET) |
||||||
|
public R getTableHead(String stcd) { |
||||||
|
//查询基础数据
|
||||||
|
List<AnalyzeInstanceFieldVO> report = damSafetyDataService.getSignages(stcd); |
||||||
|
return R.data(report,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
//==============================报表接口==========================
|
||||||
|
@ApiOperation("渗压年报表") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "deviceCode", value = "仪器模型code", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "year", value = "年份", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "col", value = "传渗压属性字段", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@RequestMapping(value = "/getYearSpprReport", method = RequestMethod.GET) |
||||||
|
public R getYearSpprReport(String rscd,String deviceCode,String year,String col) { |
||||||
|
Map<String, Date> res= CommonUtil.getStartEnd(year+"-01-01 00:00:00",year+"-12-31 23:59:59"); |
||||||
|
//查询基础数据
|
||||||
|
String[] deviceCodes=deviceCode.split(","); |
||||||
|
Map<String,Object> data = damSafetyDataService.getYearSpprReport(rscd,Arrays.asList(deviceCodes),res.get("begin"), res.get("end"),col); |
||||||
|
return R.data(data,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
//==============================报表接口==========================
|
||||||
|
@ApiOperation("渗流年报表") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "deviceCode", value = "仪器模型code", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "year", value = "年份", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "col", value = "传渗流属性字段", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@RequestMapping(value = "/getYearSpqnReport", method = RequestMethod.GET) |
||||||
|
public R getYearSpqnReport(String rscd,String deviceCode,String year,String col) { |
||||||
|
Map<String, Date> res= CommonUtil.getStartEnd(year+"-01-01 00:00:00",year+"-12-31 23:59:59"); |
||||||
|
//查询基础数据
|
||||||
|
String[] deviceCodes=deviceCode.split(","); |
||||||
|
Map<String,Object> data = damSafetyDataService.getYearSpprReport(rscd,Arrays.asList(deviceCodes),res.get("begin"), res.get("end"),col); |
||||||
|
return R.data(data,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
@ApiOperation("水平位移年报表") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "deviceCode", value = "仪器模型code", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "year", value = "年份", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@RequestMapping(value = "/getYearHrdsReport", method = RequestMethod.GET) |
||||||
|
public R getYearHrdsReport(String deviceCode,String year) { |
||||||
|
Map<String, Date> res= CommonUtil.getStartEnd(year+"-01-01 00:00:00",year+"-12-31 23:59:59"); |
||||||
|
//查询基础数据
|
||||||
|
String[] deviceCodes=deviceCode.split(","); |
||||||
|
Map<String,Object> data = damSafetyDataService.getYearHrdsReport(Arrays.asList(deviceCodes),res.get("begin"), res.get("end")); |
||||||
|
return R.data(data,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
@ApiOperation("垂直位移年报表") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "deviceCode", value = "仪器模型code", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "year", value = "年份", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "col", value = "传垂直位移属性字段", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@RequestMapping(value = "/getYearVrdsReport", method = RequestMethod.GET) |
||||||
|
public R getYearVrdsReport(String deviceCode,String year,String col) { |
||||||
|
Map<String, Date> res= CommonUtil.getStartEnd(year+"-01-01 00:00:00",year+"-12-31 23:59:59"); |
||||||
|
//查询基础数据
|
||||||
|
String[] deviceCodes=deviceCode.split(","); |
||||||
|
Report data = damSafetyDataService.getYearVrdsReport(Arrays.asList(deviceCodes),res.get("begin"), res.get("end"),col); |
||||||
|
return R.data(data,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
@ApiOperation("压力(应力)年报表") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "deviceCode", value = "仪器模型code", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "year", value = "年份", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "col", value = "传垂直位移属性字段", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@RequestMapping(value = "/getYearStressReport", method = RequestMethod.GET) |
||||||
|
public R getYearStressReport(String deviceCode,String year,String col) { |
||||||
|
Map<String, Date> res= CommonUtil.getStartEnd(year+"-01-01 00:00:00",year+"-12-31 23:59:59"); |
||||||
|
//查询基础数据
|
||||||
|
String[] deviceCodes=deviceCode.split(","); |
||||||
|
Report data = damSafetyDataService.getYearVrdsReport(Arrays.asList(deviceCodes),res.get("begin"), res.get("end"),col); |
||||||
|
return R.data(data,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
private final ITProjInfoService tProjInfoService; |
||||||
|
@ApiOperation("大坝报表 无工程竣工日期将默认查询三年的历史数据") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "deviceCode", value = "仪器模型code", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "queryType", value = "year 或 month", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "yearMonth", value = "年份或者年月", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "col", value = "属性字段", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@RequestMapping(value = "/getDamReport", method = RequestMethod.GET) |
||||||
|
public R getDamReport(String deviceCode,String queryType,String yearMonth,String col) { |
||||||
|
Map<String, Date> res = CommonUtil.getStartEnd(yearMonth + "-01-01 00:00:00", yearMonth + "-12-31 23:59:59"); |
||||||
|
String type="month"; |
||||||
|
if(QueryDateTypeEnum.MONTH.getQueryDateType().equals(queryType)) { |
||||||
|
String yearMonthDay = CommonUtil.getLastDayOfMonth(yearMonth); |
||||||
|
type="day"; |
||||||
|
res = CommonUtil.getStartEnd(yearMonth + "-01 00:00:00", yearMonthDay + " 23:59:59"); |
||||||
|
} |
||||||
|
|
||||||
|
List<TProjInfo> dd=tProjInfoService.list(); |
||||||
|
int yearGap=3; |
||||||
|
if(dd!=null && !dd.isEmpty()){ |
||||||
|
Date completeDate=dd.get(0).getCompleteDate(); |
||||||
|
if(completeDate!=null) { |
||||||
|
yearGap = CommonUtil.getYearGap(completeDate, res.get("begin")); |
||||||
|
} |
||||||
|
} |
||||||
|
//查询基础数据
|
||||||
|
String[] deviceCodes=deviceCode.split(","); |
||||||
|
String[] cols=col.split(","); |
||||||
|
if(cols.length == 1) {//渗压、垂直位移
|
||||||
|
Map<String,Object> data = damSafetyDataService.getDamReport(Arrays.asList(deviceCodes),type, res.get("begin"), res.get("end"), cols[0],yearGap); |
||||||
|
return R.data(data,"查询成功"); |
||||||
|
} |
||||||
|
|
||||||
|
if(cols.length == 2) {//水平位移
|
||||||
|
Map<String,Object> xData = damSafetyDataService.getDamReport(Arrays.asList(deviceCodes),type, res.get("begin"), res.get("end"), cols[0],yearGap); |
||||||
|
Map<String,Object> yData = damSafetyDataService.getDamReport(Arrays.asList(deviceCodes),type, res.get("begin"), res.get("end"), cols[1],yearGap); |
||||||
|
Map<String,Object> data=new HashMap<>(); |
||||||
|
data.put("xData",xData); |
||||||
|
data.put("yData",yData); |
||||||
|
return R.data(data,"查询成功"); |
||||||
|
} |
||||||
|
return null; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,57 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.service.IFileUploadService; |
||||||
|
import io.swagger.annotations.Api; |
||||||
|
import io.swagger.annotations.ApiOperation; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||||
|
import org.springframework.web.bind.annotation.RequestMapping; |
||||||
|
import org.springframework.web.bind.annotation.RequestMethod; |
||||||
|
import org.springframework.web.bind.annotation.RequestParam; |
||||||
|
import org.springframework.web.bind.annotation.RestController; |
||||||
|
import org.springframework.web.multipart.MultipartFile; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/fileUpload") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "大坝文件上传", tags = "大坝文件上传接口") |
||||||
|
public class FileUploadController { |
||||||
|
|
||||||
|
@Autowired |
||||||
|
IFileUploadService iImageUploadService; |
||||||
|
|
||||||
|
@RequestMapping(value="/uploadFile", method = {RequestMethod.POST}) |
||||||
|
@ApiOperation(notes ="上传单个文件", value = "上传单个文件") |
||||||
|
public R addImage(@RequestParam("file") MultipartFile file){ |
||||||
|
try { |
||||||
|
Object path = iImageUploadService.addFile(file,"fileInfo"); |
||||||
|
if (path!=null) { |
||||||
|
return R.data(path); |
||||||
|
} else { |
||||||
|
return R.fail("操作失败"); |
||||||
|
} |
||||||
|
}catch (Exception e) { |
||||||
|
return R.fail("操作失败"); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
@RequestMapping(value="/uploadFiles", method = {RequestMethod.POST}) |
||||||
|
@ApiOperation(notes ="上传多文件", value = "上传多文件") |
||||||
|
public R addFiles(@RequestParam("files") MultipartFile[] files){ |
||||||
|
try { |
||||||
|
List<String> paths = iImageUploadService.addFiles(files,"fileInfo"); |
||||||
|
if (paths!=null) { |
||||||
|
return R.data(paths); |
||||||
|
} else { |
||||||
|
return R.fail("操作失败"); |
||||||
|
} |
||||||
|
}catch (Exception e) { |
||||||
|
return R.fail("操作失败"); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,124 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.damsafety.entity.TBuilding; |
||||||
|
import com.hnac.hzims.damsafety.service.ITBuildingService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import com.hnac.hzims.damsafety.vo.TBuildingVo; |
||||||
|
import com.hnac.hzims.damsafety.wrapper.TBuildingWrapper; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/tBuilding") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "建筑物", tags = "建筑物接口") |
||||||
|
public class TBuildingController extends BladeController implements CacheNames { |
||||||
|
private final ITBuildingService tBuildingService; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "name", value = "建筑物名称", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入tBuilding") |
||||||
|
public R<IPage<TBuildingVo>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<TBuilding> wrapper= ParamUtil.conditionTBuilding(param); |
||||||
|
IPage<TBuilding> pages = tBuildingService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(TBuildingWrapper.build().pageVO(pages)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "name", value = "建筑物名称", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入tBuilding") |
||||||
|
public R<List<TBuildingVo>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<TBuilding> wrapper=ParamUtil.conditionTBuilding(param); |
||||||
|
List<TBuilding> list = tBuildingService.list(wrapper); |
||||||
|
return R.data(TBuildingWrapper.build().listVO(list)); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入tBuilding") |
||||||
|
public R<TBuildingVo> detail(TBuilding tBuilding) { |
||||||
|
TBuilding detail = tBuildingService.getOne(Condition.getQueryWrapper(tBuilding)); |
||||||
|
return R.data(TBuildingWrapper.build().entityVO(detail)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入tBuilding") |
||||||
|
public R save(@RequestBody TBuilding tBuilding) { |
||||||
|
LambdaQueryWrapper<TBuilding> wrapper=new LambdaQueryWrapper(); |
||||||
|
|
||||||
|
wrapper.eq(TBuilding::getRscd,tBuilding.getRscd()); |
||||||
|
wrapper.eq(TBuilding::getName,tBuilding.getName()); |
||||||
|
List<TBuilding> list = tBuildingService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()){ |
||||||
|
return R.fail("此水库中建筑物名称已存在"); |
||||||
|
} |
||||||
|
return R.status(tBuildingService.save(tBuilding)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入tBuilding") |
||||||
|
public R update(@RequestBody TBuilding tBuilding) { |
||||||
|
LambdaQueryWrapper<TBuilding> wrapper=new LambdaQueryWrapper(); |
||||||
|
wrapper.eq(TBuilding::getRscd,tBuilding.getRscd()); |
||||||
|
wrapper.eq(TBuilding::getName,tBuilding.getName()); |
||||||
|
wrapper.ne(TBuilding::getId,tBuilding.getId()); |
||||||
|
List<TBuilding> list = tBuildingService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()){ |
||||||
|
return R.fail("此水库中建筑物名称已存在"); |
||||||
|
} |
||||||
|
return R.status(tBuildingService.updateById(tBuilding)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "ids") |
||||||
|
public R remove(@ApiParam(value = "主键集合") @RequestParam String ids) { |
||||||
|
boolean temp = tBuildingService.deleteLogic(Func.toLongList(ids)); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,131 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.damsafety.entity.TProjInfo; |
||||||
|
import com.hnac.hzims.damsafety.service.ITProjInfoService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import com.hnac.hzims.damsafety.vo.TProjInfoVo; |
||||||
|
import com.hnac.hzims.damsafety.wrapper.TProjInfoWrapper; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/tProjInfo") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "工程信息", tags = "工程信息接口") |
||||||
|
public class TProjInfoController extends BladeController implements CacheNames { |
||||||
|
|
||||||
|
private final ITProjInfoService tProjInfoService; |
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "projName", value = "工程名称", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "projCode", value = "工程编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入tProjInfo") |
||||||
|
public R<IPage<TProjInfoVo>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<TProjInfo> wrapper= ParamUtil.conditionTProjInfo(param); |
||||||
|
IPage<TProjInfo> pages = tProjInfoService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(TProjInfoWrapper.build().pageVO(pages)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "projName", value = "工程名称", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "projCode", value = "工程编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入tProjInfo") |
||||||
|
public R<List<TProjInfoVo>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<TProjInfo> wrapper=ParamUtil.conditionTProjInfo(param); |
||||||
|
List<TProjInfo> list = tProjInfoService.list(wrapper); |
||||||
|
return R.data(TProjInfoWrapper.build().listVO(list)); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入tProjInfo") |
||||||
|
public R<TProjInfoVo> detail(TProjInfo tProjInfo) { |
||||||
|
TProjInfo detail = tProjInfoService.getOne(Condition.getQueryWrapper(tProjInfo)); |
||||||
|
return R.data(TProjInfoWrapper.build().entityVO(detail)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入tProjInfo") |
||||||
|
public R save(@RequestBody TProjInfo tProjInfo) { |
||||||
|
LambdaQueryWrapper<TProjInfo> wrapper=new LambdaQueryWrapper(); |
||||||
|
wrapper.eq(TProjInfo::getProjCode,tProjInfo.getProjCode()); |
||||||
|
List<TProjInfo> list = tProjInfoService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()){ |
||||||
|
return R.fail("工程编码已存在"); |
||||||
|
} |
||||||
|
|
||||||
|
wrapper=new LambdaQueryWrapper(); |
||||||
|
wrapper.eq(TProjInfo::getProjName,tProjInfo.getProjName()); |
||||||
|
list = tProjInfoService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()){ |
||||||
|
return R.fail("工程名称已存在"); |
||||||
|
} |
||||||
|
|
||||||
|
return R.status(tProjInfoService.save(tProjInfo)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入tProjInfo") |
||||||
|
public R update(@RequestBody TProjInfo tProjInfo) { |
||||||
|
LambdaQueryWrapper<TProjInfo> wrapper=new LambdaQueryWrapper(); |
||||||
|
wrapper.eq(TProjInfo::getProjCode,tProjInfo.getProjCode()); |
||||||
|
wrapper.ne(TProjInfo::getId,tProjInfo.getId()); |
||||||
|
List<TProjInfo> list = tProjInfoService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()){ |
||||||
|
return R.fail("工程编码已存在"); |
||||||
|
} |
||||||
|
return R.status(tProjInfoService.updateById(tProjInfo)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "ids") |
||||||
|
public R remove(@ApiParam(value = "主键集合") @RequestParam String ids) { |
||||||
|
boolean temp = tProjInfoService.deleteLogic(Func.toLongList(ids)); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,114 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.damsafety.entity.TSectionConfig; |
||||||
|
import com.hnac.hzims.damsafety.service.ITSectionConfigService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/tSectionConfig") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "断面配置", tags = "断面配置接口") |
||||||
|
public class TSectionConfigController extends BladeController implements CacheNames { |
||||||
|
private final ITSectionConfigService tSectionConfigService; |
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测点编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "sectionNo", value = "断面编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入TSectionConfig") |
||||||
|
public R<IPage<TSectionConfig>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<TSectionConfig> wrapper= ParamUtil.conditionTSectionConfig(param); |
||||||
|
IPage<TSectionConfig> pages = tSectionConfigService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(pages); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测点编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "sectionNo", value = "断面编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入TSectionConfig") |
||||||
|
public R<List<TSectionConfig>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<TSectionConfig> wrapper=ParamUtil.conditionTSectionConfig(param); |
||||||
|
List<TSectionConfig> list = tSectionConfigService.list(wrapper); |
||||||
|
return R.data(list); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入tSectionConfig") |
||||||
|
public R<TSectionConfig> detail(TSectionConfig tSectionConfig) { |
||||||
|
TSectionConfig detail = tSectionConfigService.getOne(Condition.getQueryWrapper(tSectionConfig)); |
||||||
|
return R.data(detail); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入tSectionConfig") |
||||||
|
public R save(@RequestBody TSectionConfig tSectionConfig) { |
||||||
|
LambdaQueryWrapper<TSectionConfig> wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(TSectionConfig::getStcd,tSectionConfig.getStcd()); |
||||||
|
List<TSectionConfig> list = tSectionConfigService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()){ |
||||||
|
return R.fail("该测点编号配置已存在"); |
||||||
|
} |
||||||
|
|
||||||
|
return R.status(tSectionConfigService.save(tSectionConfig)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入tSectionConfig") |
||||||
|
public R update(@RequestBody TSectionConfig tSectionConfig) { |
||||||
|
return R.status(tSectionConfigService.updateById(tSectionConfig)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "stcd") |
||||||
|
public R remove(@ApiParam(value = "测点编号集合") @RequestParam String stcd) { |
||||||
|
List<String> list=Func.toStrList(stcd); |
||||||
|
LambdaQueryWrapper<TSectionConfig> wrapper=new LambdaQueryWrapper(); |
||||||
|
wrapper.in(TSectionConfig::getStcd,list); |
||||||
|
boolean temp = tSectionConfigService.delete(wrapper); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,123 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDamsB; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpDamsBService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/wrpDamsB") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "断面特征点信息", tags = "断面特征点信息接口") |
||||||
|
public class WrpDamsBController extends BladeController implements CacheNames { |
||||||
|
private final IWrpDamsBService wrpDamsBService; |
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damscd", value = "特征点编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damsnm", value = "特征点名称", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入wrpDamsB") |
||||||
|
public R<IPage<WrpDamsB>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<WrpDamsB> wrapper= ParamUtil.conditionWrpDamsB(param); |
||||||
|
IPage<WrpDamsB> pages = wrpDamsBService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(pages); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damscd", value = "特征点编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damsnm", value = "特征点名称", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入wrpDamsB") |
||||||
|
public R<List<WrpDamsB>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<WrpDamsB> wrapper=ParamUtil.conditionWrpDamsB(param); |
||||||
|
List<WrpDamsB> list = wrpDamsBService.list(wrapper); |
||||||
|
return R.data(list); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入wrpDamsB") |
||||||
|
public R<WrpDamsB> detail(WrpDamsB wrpDamsB) { |
||||||
|
WrpDamsB detail = wrpDamsBService.getOne(Condition.getQueryWrapper(wrpDamsB)); |
||||||
|
return R.data(detail); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入wrpDamsB") |
||||||
|
public R save(@RequestBody WrpDamsB wrpDamsB) { |
||||||
|
LambdaQueryWrapper<WrpDamsB> wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(WrpDamsB::getDamscd,wrpDamsB.getDamscd()); |
||||||
|
List<WrpDamsB> list = wrpDamsBService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()) { |
||||||
|
return R.data(500,false,"特征点编号已存在"); |
||||||
|
} |
||||||
|
|
||||||
|
wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(WrpDamsB::getDamsnm,wrpDamsB.getDamsnm()); |
||||||
|
list = wrpDamsBService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()) { |
||||||
|
return R.data(500,false,"特征点名称已存在"); |
||||||
|
} |
||||||
|
|
||||||
|
return R.status(wrpDamsBService.save(wrpDamsB)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入wrpDamsB") |
||||||
|
public R update(@RequestBody WrpDamsB wrpDamsB) { |
||||||
|
return R.status(wrpDamsBService.updateById(wrpDamsB)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "damscd") |
||||||
|
public R remove(@ApiParam(value = "特征点编号集合") @RequestParam String damscd) { |
||||||
|
List<String> list=Func.toStrList(damscd); |
||||||
|
LambdaQueryWrapper<WrpDamsB> wrapper=new LambdaQueryWrapper(); |
||||||
|
wrapper.in(WrpDamsB::getDamscd,list); |
||||||
|
boolean temp = wrpDamsBService.delete(wrapper); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,135 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrhrdsmp; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpDfrSrhrdsmpService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import com.hnac.hzims.damsafety.vo.WrpDfrSrhrdsmpVo; |
||||||
|
import com.hnac.hzims.damsafety.wrapper.WrpDfrSrhrdsmpWrapper; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.apache.commons.lang3.StringUtils; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.secure.utils.AuthUtil; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import javax.servlet.http.HttpServletRequest; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/wrpDfrSrhrdsmp") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "水平位移监测测点", tags = "水平位移监测测点接口") |
||||||
|
public class WrpDfrSrhrdsmpController extends BladeController implements CacheNames { |
||||||
|
private final IWrpDfrSrhrdsmpService wrpDfrSrhrdsmpService; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测站编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "mpcd", value = "测点编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入wrpDfrSrhrdsmp") |
||||||
|
public R<IPage<WrpDfrSrhrdsmpVo>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<WrpDfrSrhrdsmp> wrapper= ParamUtil.conditionWrpDfrSrhrdsmp(param); |
||||||
|
IPage<WrpDfrSrhrdsmp> pages = wrpDfrSrhrdsmpService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(WrpDfrSrhrdsmpWrapper.build().pageVO(pages)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测站编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "mpcd", value = "测点编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入wrpDfrSrhrdsmp") |
||||||
|
public R<List<WrpDfrSrhrdsmpVo>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<WrpDfrSrhrdsmp> wrapper=ParamUtil.conditionWrpDfrSrhrdsmp(param); |
||||||
|
List<WrpDfrSrhrdsmp> list = wrpDfrSrhrdsmpService.list(wrapper); |
||||||
|
return R.data(WrpDfrSrhrdsmpWrapper.build().listVO(list)); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 查询水平位移断面 |
||||||
|
*/ |
||||||
|
@GetMapping("/section") |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "查询水平位移断面") |
||||||
|
public R<List<Map<String,Object>>> section(HttpServletRequest request,String rscd) { |
||||||
|
String deptId=request.getHeader("dept-id"); |
||||||
|
if(StringUtils.isBlank(deptId)){ |
||||||
|
deptId = AuthUtil.getDeptId(); |
||||||
|
} |
||||||
|
List<Map<String,Object>> list = wrpDfrSrhrdsmpService.section(deptId,rscd); |
||||||
|
//返回断面加仪器数据
|
||||||
|
return R.data(list,"查询断面成功"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入wrpDfrSrhrdsmp") |
||||||
|
public R<WrpDfrSrhrdsmpVo> detail(WrpDfrSrhrdsmp wrpDfrSrhrdsmp) { |
||||||
|
WrpDfrSrhrdsmp detail = wrpDfrSrhrdsmpService.getOne(Condition.getQueryWrapper(wrpDfrSrhrdsmp)); |
||||||
|
return R.data(WrpDfrSrhrdsmpWrapper.build().entityVO(detail)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入wrpDfrSrhrdsmp") |
||||||
|
public R save(@RequestBody WrpDfrSrhrdsmp wrpDfrSrhrdsmp) { |
||||||
|
LambdaQueryWrapper<WrpDfrSrhrdsmp> wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(WrpDfrSrhrdsmp::getMpcd,wrpDfrSrhrdsmp.getMpcd()); |
||||||
|
List<WrpDfrSrhrdsmp> list = wrpDfrSrhrdsmpService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()) { |
||||||
|
return R.data(500,false,"测点编号已存在"); |
||||||
|
} |
||||||
|
return R.status(wrpDfrSrhrdsmpService.save(wrpDfrSrhrdsmp)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入wrpDfrSrhrdsmp") |
||||||
|
public R update(@RequestBody WrpDfrSrhrdsmp wrpDfrSrhrdsmp) { |
||||||
|
return R.status(wrpDfrSrhrdsmpService.updateById(wrpDfrSrhrdsmp)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "ids") |
||||||
|
public R remove(@ApiParam(value = "主键集合") @RequestParam String ids) { |
||||||
|
boolean temp = wrpDfrSrhrdsmpService.deleteLogic(Func.toLongList(ids)); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,117 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsbp; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpDfrSrvrdsbpService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import com.hnac.hzims.damsafety.vo.WrpDfrSrvrdsbpVo; |
||||||
|
import com.hnac.hzims.damsafety.wrapper.WrpDfrSrvrdsbpWrapper; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/wrpDfrSrvrdsbp") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "监测基点表", tags = "监测基点接口") |
||||||
|
public class WrpDfrSrvrdsbpController extends BladeController implements CacheNames { |
||||||
|
private final IWrpDfrSrvrdsbpService wrpDfrSrvrdsbpService; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库代码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "hycncd", value = "水工建筑物id", paramType = "query", dataType = "long"), |
||||||
|
@ApiImplicitParam(name = "bpcd", value = "基点编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "bptp", value = "基点类型", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入WrpDfrSrvrdsbp") |
||||||
|
public R<IPage<WrpDfrSrvrdsbpVo>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<WrpDfrSrvrdsbp> wrapper= ParamUtil.conditionWrpDfrSrvrdsbp(param); |
||||||
|
IPage<WrpDfrSrvrdsbp> pages = wrpDfrSrvrdsbpService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(WrpDfrSrvrdsbpWrapper.build().pageVO(pages)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库代码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "hycncd", value = "水工建筑物id", paramType = "query", dataType = "long"), |
||||||
|
@ApiImplicitParam(name = "bpcd", value = "基点编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "bptp", value = "基点类型", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入WrpDfrSrvrdsbp") |
||||||
|
public R<List<WrpDfrSrvrdsbpVo>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<WrpDfrSrvrdsbp> wrapper=ParamUtil.conditionWrpDfrSrvrdsbp(param); |
||||||
|
List<WrpDfrSrvrdsbp> list = wrpDfrSrvrdsbpService.list(wrapper); |
||||||
|
return R.data(WrpDfrSrvrdsbpWrapper.build().listVO(list)); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入wrpDfrSrvrdsbp") |
||||||
|
public R<WrpDfrSrvrdsbpVo> detail(WrpDfrSrvrdsbp wrpDfrSrvrdsbp) { |
||||||
|
WrpDfrSrvrdsbp detail = wrpDfrSrvrdsbpService.getOne(Condition.getQueryWrapper(wrpDfrSrvrdsbp)); |
||||||
|
return R.data(WrpDfrSrvrdsbpWrapper.build().entityVO(detail)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入wrpDfrSrvrdsbp") |
||||||
|
public R save(@RequestBody WrpDfrSrvrdsbp wrpDfrSrvrdsbp) { |
||||||
|
LambdaQueryWrapper<WrpDfrSrvrdsbp> wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(WrpDfrSrvrdsbp::getBpcd,wrpDfrSrvrdsbp.getBpcd()); |
||||||
|
List<WrpDfrSrvrdsbp> list = wrpDfrSrvrdsbpService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()) { |
||||||
|
return R.data(500,false,"基点编号已存在"); |
||||||
|
} |
||||||
|
return R.status(wrpDfrSrvrdsbpService.save(wrpDfrSrvrdsbp)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入wrpDfrSrvrdsbp") |
||||||
|
public R update(@RequestBody WrpDfrSrvrdsbp wrpDfrSrvrdsbp) { |
||||||
|
return R.status(wrpDfrSrvrdsbpService.updateById(wrpDfrSrvrdsbp)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "ids") |
||||||
|
public R remove(@ApiParam(value = "主键集合") @RequestParam String ids) { |
||||||
|
boolean temp = wrpDfrSrvrdsbpService.deleteLogic(Func.toLongList(ids)); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,135 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsmp; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpDfrSrvrdsmpService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import com.hnac.hzims.damsafety.vo.WrpDfrSrvrdsmpVo; |
||||||
|
import com.hnac.hzims.damsafety.wrapper.WrpDfrSrvrdsmpWrapper; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.apache.commons.lang3.StringUtils; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.secure.utils.AuthUtil; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import javax.servlet.http.HttpServletRequest; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/wrpDfrSrvrdsmp") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "垂直位移监测测点", tags = "垂直位移监测测点接口") |
||||||
|
public class WrpDfrSrvrdsmpController extends BladeController implements CacheNames { |
||||||
|
private final IWrpDfrSrvrdsmpService wrpDfrSrvrdsmpService; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测站编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "mpcd", value = "测点编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入wrpDfrSrvrdsmp") |
||||||
|
public R<IPage<WrpDfrSrvrdsmpVo>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<WrpDfrSrvrdsmp> wrapper= ParamUtil.conditionWrpDfrSrvrdsmp(param); |
||||||
|
IPage<WrpDfrSrvrdsmp> pages = wrpDfrSrvrdsmpService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(WrpDfrSrvrdsmpWrapper.build().pageVO(pages)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测站编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "mpcd", value = "测点编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入wrpDfrSrvrdsmp") |
||||||
|
public R<List<WrpDfrSrvrdsmpVo>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<WrpDfrSrvrdsmp> wrapper=ParamUtil.conditionWrpDfrSrvrdsmp(param); |
||||||
|
List<WrpDfrSrvrdsmp> list = wrpDfrSrvrdsmpService.list(wrapper); |
||||||
|
return R.data(WrpDfrSrvrdsmpWrapper.build().listVO(list)); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 查询垂直位移断面 |
||||||
|
*/ |
||||||
|
@GetMapping("/section") |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "查询垂直位移断面") |
||||||
|
public R<List<Map<String,Object>>> section(HttpServletRequest request,String rscd) { |
||||||
|
String deptId=request.getHeader("dept-id"); |
||||||
|
if(StringUtils.isBlank(deptId)){ |
||||||
|
deptId = AuthUtil.getDeptId(); |
||||||
|
} |
||||||
|
List<Map<String,Object>> list = wrpDfrSrvrdsmpService.section(deptId,rscd); |
||||||
|
//返回断面加仪器数据
|
||||||
|
return R.data(list,"查询断面成功"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入wrpDfrSrvrdsmp") |
||||||
|
public R<WrpDfrSrvrdsmpVo> detail(WrpDfrSrvrdsmp wrpDfrSrvrdsmp) { |
||||||
|
WrpDfrSrvrdsmp detail = wrpDfrSrvrdsmpService.getOne(Condition.getQueryWrapper(wrpDfrSrvrdsmp)); |
||||||
|
return R.data(WrpDfrSrvrdsmpWrapper.build().entityVO(detail)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入wrpDfrSrvrdsmp") |
||||||
|
public R save(@RequestBody WrpDfrSrvrdsmp wrpDfrSrvrdsmp) { |
||||||
|
LambdaQueryWrapper<WrpDfrSrvrdsmp> wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(WrpDfrSrvrdsmp::getMpcd,wrpDfrSrvrdsmp.getMpcd()); |
||||||
|
List<WrpDfrSrvrdsmp> list = wrpDfrSrvrdsmpService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()) { |
||||||
|
return R.data(500,false,"测点编号已存在"); |
||||||
|
} |
||||||
|
return R.status(wrpDfrSrvrdsmpService.save(wrpDfrSrvrdsmp)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入wrpDfrSrvrdsmp") |
||||||
|
public R update(@RequestBody WrpDfrSrvrdsmp wrpDfrSrvrdsmp) { |
||||||
|
return R.status(wrpDfrSrvrdsmpService.updateById(wrpDfrSrvrdsmp)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "ids") |
||||||
|
public R remove(@ApiParam(value = "主键集合") @RequestParam String ids) { |
||||||
|
boolean temp = wrpDfrSrvrdsmpService.deleteLogic(Func.toLongList(ids)); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,149 @@ |
|||||||
|
/* |
||||||
|
* Copyright (c) 2018-2028, Chill Zhuang All rights reserved. |
||||||
|
* |
||||||
|
* Redistribution and use in source and binary forms, with or without |
||||||
|
* modification, are permitted provided that the following conditions are met: |
||||||
|
* |
||||||
|
* Redistributions of source code must retain the above copyright Example, |
||||||
|
* this list of conditions and the following disclaimer. |
||||||
|
* Redistributions in binary form must reproduce the above copyright |
||||||
|
* Example, this list of conditions and the following disclaimer in the |
||||||
|
* documentation and/or other materials provided with the distribution. |
||||||
|
* Neither the name of the dreamlu.net developer nor the names of its |
||||||
|
* contributors may be used to endorse or promote products derived from |
||||||
|
* this software without specific prior written permission. |
||||||
|
* Author: Chill 庄骞 (smallchill@163.com) |
||||||
|
*/ |
||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSectionB; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpSectionBService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import com.hnac.hzims.damsafety.vo.WrpSectionBVO; |
||||||
|
import com.hnac.hzims.damsafety.wrapper.WrpSectionBWrapper; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
/** |
||||||
|
* 大坝断面控制器 |
||||||
|
* |
||||||
|
* @author Chill |
||||||
|
*/ |
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/wrpSectionB") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "大坝断面", tags = "大坝断面接口") |
||||||
|
public class WrpSectionBController extends BladeController implements CacheNames { |
||||||
|
|
||||||
|
private final IWrpSectionBService wrpSectionBService; |
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "buildingId", value = "建筑物id", paramType = "query", dataType = "long"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damnm", value = "断面名称", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "wallType", value = "防渗墙类型", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入wrpSectionB") |
||||||
|
public R<IPage<WrpSectionBVO>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<WrpSectionB> wrapper= ParamUtil.conditionWrpSectionB(param); |
||||||
|
IPage<WrpSectionB> pages = wrpSectionBService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(WrpSectionBWrapper.build().pageVO(pages)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "buildingId", value = "建筑物id", paramType = "query", dataType = "long"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damnm", value = "断面名称", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "wallType", value = "防渗墙类型", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入wrpSectionB") |
||||||
|
public R<List<WrpSectionBVO>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<WrpSectionB> wrapper=ParamUtil.conditionWrpSectionB(param); |
||||||
|
List<WrpSectionB> list = wrpSectionBService.list(wrapper); |
||||||
|
return R.data(WrpSectionBWrapper.build().listVO(list)); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入wrpSectionB") |
||||||
|
public R<WrpSectionBVO> detail(WrpSectionB WrpSectionB) { |
||||||
|
WrpSectionB detail = wrpSectionBService.getOne(Condition.getQueryWrapper(WrpSectionB)); |
||||||
|
return R.data(WrpSectionBWrapper.build().entityVO(detail)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入wrpSectionB") |
||||||
|
public R save(@RequestBody WrpSectionB wrpSectionB) { |
||||||
|
LambdaQueryWrapper<WrpSectionB> wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(WrpSectionB::getDamcd,wrpSectionB.getDamcd()); |
||||||
|
List<WrpSectionB> list = wrpSectionBService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()) { |
||||||
|
return R.data(500,false,"断面编码已存在"); |
||||||
|
} |
||||||
|
|
||||||
|
wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(WrpSectionB::getDamnm,wrpSectionB.getDamnm()); |
||||||
|
list = wrpSectionBService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()) { |
||||||
|
return R.data(500,false,"断面名称已存在"); |
||||||
|
} |
||||||
|
|
||||||
|
return R.status(wrpSectionBService.save(wrpSectionB)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入wrpSectionB") |
||||||
|
public R update(@RequestBody WrpSectionB wrpSectionB) { |
||||||
|
return R.status(wrpSectionBService.updateById(wrpSectionB)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "ids") |
||||||
|
public R remove(@ApiParam(value = "主键集合") @RequestParam String ids) { |
||||||
|
boolean temp = wrpSectionBService.deleteLogic(Func.toLongList(ids)); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,133 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgPztb; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpSpgPztbService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import com.hnac.hzims.damsafety.vo.WrpSpgPztbVo; |
||||||
|
import com.hnac.hzims.damsafety.wrapper.WrpSpgPztbWrapper; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.apache.commons.lang3.StringUtils; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.secure.utils.AuthUtil; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import javax.servlet.http.HttpServletRequest; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/wrpSpgPztb") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "测压管测点", tags = "测压管测点接口") |
||||||
|
public class WrpSpgPztbController extends BladeController implements CacheNames { |
||||||
|
private final IWrpSpgPztbService wrpSpgPztbService; |
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测站编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "mpcd", value = "测点编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入wrpSpgPztb") |
||||||
|
public R<IPage<WrpSpgPztbVo>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<WrpSpgPztb> wrapper= ParamUtil.conditionWrpSpgPztb(param); |
||||||
|
IPage<WrpSpgPztb> pages = wrpSpgPztbService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(WrpSpgPztbWrapper.build().pageVO(pages)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测站编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "mpcd", value = "测点编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入wrpSpgPztb") |
||||||
|
public R<List<WrpSpgPztbVo>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<WrpSpgPztb> wrapper=ParamUtil.conditionWrpSpgPztb(param); |
||||||
|
List<WrpSpgPztb> list = wrpSpgPztbService.list(wrapper); |
||||||
|
return R.data(WrpSpgPztbWrapper.build().listVO(list)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 查询测压管断面 |
||||||
|
*/ |
||||||
|
@GetMapping("/section") |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "查询测压管断面") |
||||||
|
public R<List<Map<String,Object>>> section(HttpServletRequest request,String rscd) { |
||||||
|
String deptId=request.getHeader("dept-id"); |
||||||
|
if(StringUtils.isBlank(deptId)){ |
||||||
|
deptId = AuthUtil.getDeptId(); |
||||||
|
} |
||||||
|
List<Map<String,Object>> list = wrpSpgPztbService.section(deptId,rscd); |
||||||
|
//返回断面加仪器数据
|
||||||
|
return R.data(list,"查询断面成功"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入wrpSpgPztb") |
||||||
|
public R<WrpSpgPztbVo> detail(WrpSpgPztb wrpSpgPztb) { |
||||||
|
WrpSpgPztb detail = wrpSpgPztbService.getOne(Condition.getQueryWrapper(wrpSpgPztb)); |
||||||
|
return R.data(WrpSpgPztbWrapper.build().entityVO(detail)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入wrpSpgPztb") |
||||||
|
public R save(@RequestBody WrpSpgPztb wrpSpgPztb) { |
||||||
|
LambdaQueryWrapper<WrpSpgPztb> wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(WrpSpgPztb::getMpcd,wrpSpgPztb.getMpcd()); |
||||||
|
List<WrpSpgPztb> list = wrpSpgPztbService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()) { |
||||||
|
return R.data(500,false,"测点编号已存在"); |
||||||
|
} |
||||||
|
return R.status(wrpSpgPztbService.save(wrpSpgPztb)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入wrpSpgPztb") |
||||||
|
public R update(@RequestBody WrpSpgPztb wrpSpgPztb) { |
||||||
|
return R.status(wrpSpgPztbService.updateById(wrpSpgPztb)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "ids") |
||||||
|
public R remove(@ApiParam(value = "主键集合") @RequestParam String ids) { |
||||||
|
boolean temp = wrpSpgPztbService.deleteLogic(Func.toLongList(ids)); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,139 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgSpprmp; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpSpgSpprmpService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import com.hnac.hzims.damsafety.vo.WrpSpgSpprmpVo; |
||||||
|
import com.hnac.hzims.damsafety.wrapper.WrpSpgSpprmpWrapper; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.apache.commons.lang3.StringUtils; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.secure.utils.AuthUtil; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import javax.servlet.http.HttpServletRequest; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/wrpSpgSpprmp") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "渗流压力测点信息", tags = "渗流压力测点信息接口") |
||||||
|
public class WrpSpgSpprmpController extends BladeController implements CacheNames { |
||||||
|
private final IWrpSpgSpprmpService wrpSpgSpprmpService; |
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测站编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "mpcd", value = "测点编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "ch", value = "桩号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入wrpSpgSpprmp") |
||||||
|
public R<IPage<WrpSpgSpprmpVo>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<WrpSpgSpprmp> wrapper= ParamUtil.conditionWrpSpgSpprmp(param); |
||||||
|
IPage<WrpSpgSpprmp> pages = wrpSpgSpprmpService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(WrpSpgSpprmpWrapper.build().pageVO(pages)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测站编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "mpcd", value = "测点编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "ch", value = "桩号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入wrpSpgSpprmp") |
||||||
|
public R<List<WrpSpgSpprmpVo>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<WrpSpgSpprmp> wrapper=ParamUtil.conditionWrpSpgSpprmp(param); |
||||||
|
List<WrpSpgSpprmp> list = wrpSpgSpprmpService.list(wrapper); |
||||||
|
return R.data(WrpSpgSpprmpWrapper.build().listVO(list)); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 查询渗压断面 |
||||||
|
*/ |
||||||
|
@GetMapping("/section") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "rscd", value = "水库编码", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "查询渗压断面") |
||||||
|
public R<List<Map<String,Object>>> section(HttpServletRequest request,String rscd) { |
||||||
|
String deptId=request.getHeader("dept-id"); |
||||||
|
if(StringUtils.isBlank(deptId)){ |
||||||
|
deptId = AuthUtil.getDeptId(); |
||||||
|
} |
||||||
|
List<Map<String,Object>> list = wrpSpgSpprmpService.section(deptId,rscd); |
||||||
|
//返回断面加仪器数据
|
||||||
|
return R.data(list,"查询断面成功"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入wrpSpgSpprmp") |
||||||
|
public R<WrpSpgSpprmpVo> detail(WrpSpgSpprmp wrpSpgSpprmp) { |
||||||
|
WrpSpgSpprmp detail = wrpSpgSpprmpService.getOne(Condition.getQueryWrapper(wrpSpgSpprmp)); |
||||||
|
return R.data(WrpSpgSpprmpWrapper.build().entityVO(detail)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入wrpSpgSpprmp") |
||||||
|
public R save(@RequestBody WrpSpgSpprmp wrpSpgSpprmp) { |
||||||
|
LambdaQueryWrapper<WrpSpgSpprmp> wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(WrpSpgSpprmp::getMpcd,wrpSpgSpprmp.getMpcd()); |
||||||
|
List<WrpSpgSpprmp> list = wrpSpgSpprmpService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()) { |
||||||
|
return R.data(500,false,"测点编号已存在"); |
||||||
|
} |
||||||
|
return R.status(wrpSpgSpprmpService.save(wrpSpgSpprmp)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入wrpSpgSpprmp") |
||||||
|
public R update(@RequestBody WrpSpgSpprmp wrpSpgSpprmp) { |
||||||
|
return R.status(wrpSpgSpprmpService.updateById(wrpSpgSpprmp)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "ids") |
||||||
|
public R remove(@ApiParam(value = "主键集合") @RequestParam String ids) { |
||||||
|
boolean temp = wrpSpgSpprmpService.deleteLogic(Func.toLongList(ids)); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,133 @@ |
|||||||
|
package com.hnac.hzims.damsafety.controller; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.common.logs.annotation.OperationAnnotation; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgSpqnmp; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpSpgSpqnmpService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import com.hnac.hzims.damsafety.vo.WrpSpgSpqnmpVo; |
||||||
|
import com.hnac.hzims.damsafety.wrapper.WrpSpgSpqnmpWrapper; |
||||||
|
import io.swagger.annotations.*; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.apache.commons.lang3.StringUtils; |
||||||
|
import org.springblade.common.cache.CacheNames; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.mp.support.Condition; |
||||||
|
import org.springblade.core.mp.support.Query; |
||||||
|
import org.springblade.core.secure.utils.AuthUtil; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
import springfox.documentation.annotations.ApiIgnore; |
||||||
|
|
||||||
|
import javax.servlet.http.HttpServletRequest; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
@RequestMapping("/damSafety/wrpSpgSpqnmp") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "渗流量测点", tags = "渗流量测点接口") |
||||||
|
public class WrpSpgSpqnmpController extends BladeController implements CacheNames { |
||||||
|
private final IWrpSpgSpqnmpService wrpSpgSpqnmpService; |
||||||
|
|
||||||
|
/** |
||||||
|
* 分页 |
||||||
|
*/ |
||||||
|
@GetMapping("/pageList") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测站编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "mpcd", value = "测点编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
@ApiOperation(value = "分页", notes = "传入wrpSpgSpqnmp") |
||||||
|
public R<IPage<WrpSpgSpqnmpVo>> pageList(@ApiIgnore @RequestParam Map<String, Object> param, Query query) { |
||||||
|
LambdaQueryWrapper<WrpSpgSpqnmp> wrapper= ParamUtil.conditionWrpSpgSpqnmp(param); |
||||||
|
IPage<WrpSpgSpqnmp> pages = wrpSpgSpqnmpService.page(Condition.getPage(query), wrapper); |
||||||
|
return R.data(WrpSpgSpqnmpWrapper.build().pageVO(pages)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 列表 |
||||||
|
*/ |
||||||
|
@GetMapping("/list") |
||||||
|
@ApiImplicitParams({ |
||||||
|
@ApiImplicitParam(name = "stcd", value = "测站编码", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "damcd", value = "断面编号", paramType = "query", dataType = "string"), |
||||||
|
@ApiImplicitParam(name = "mpcd", value = "测点编号", paramType = "query", dataType = "string") |
||||||
|
}) |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "列表", notes = "传入wrpSpgSpqnmp") |
||||||
|
public R<List<WrpSpgSpqnmpVo>> list(@ApiIgnore @RequestParam Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<WrpSpgSpqnmp> wrapper=ParamUtil.conditionWrpSpgSpqnmp(param); |
||||||
|
List<WrpSpgSpqnmp> list = wrpSpgSpqnmpService.list(wrapper); |
||||||
|
return R.data(WrpSpgSpqnmpWrapper.build().listVO(list)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 查询渗流断面 |
||||||
|
*/ |
||||||
|
@GetMapping("/section") |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
@ApiOperation(value = "查询渗流断面") |
||||||
|
public R<List<Map<String,Object>>> section(HttpServletRequest request,String rscd) { |
||||||
|
String deptId=request.getHeader("dept-id"); |
||||||
|
if(StringUtils.isBlank(deptId)){ |
||||||
|
deptId = AuthUtil.getDeptId(); |
||||||
|
} |
||||||
|
List<Map<String,Object>> list = wrpSpgSpqnmpService.section(deptId,rscd); |
||||||
|
//返回断面加仪器数据
|
||||||
|
return R.data(list,"查询断面成功"); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 详情 |
||||||
|
*/ |
||||||
|
@GetMapping("/detail") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
@ApiOperation(value = "详情", notes = "传入wrpSpgSpqnmp") |
||||||
|
public R<WrpSpgSpqnmpVo> detail(WrpSpgSpqnmp wrpSpgSpqnmp) { |
||||||
|
WrpSpgSpqnmp detail = wrpSpgSpqnmpService.getOne(Condition.getQueryWrapper(wrpSpgSpqnmp)); |
||||||
|
return R.data(WrpSpgSpqnmpWrapper.build().entityVO(detail)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 新增 |
||||||
|
*/ |
||||||
|
@PostMapping("/save") |
||||||
|
@ApiOperationSupport(order = 4) |
||||||
|
@ApiOperation(value = "新增", notes = "传入wrpSpgSpqnmp") |
||||||
|
public R save(@RequestBody WrpSpgSpqnmp wrpSpgSpqnmp) { |
||||||
|
LambdaQueryWrapper<WrpSpgSpqnmp> wrapper=new LambdaQueryWrapper<>(); |
||||||
|
wrapper.eq(WrpSpgSpqnmp::getMpcd,wrpSpgSpqnmp.getMpcd()); |
||||||
|
List<WrpSpgSpqnmp> list = wrpSpgSpqnmpService.list(wrapper); |
||||||
|
if(list!=null && !list.isEmpty()) { |
||||||
|
return R.data(500,false,"测点编号已存在"); |
||||||
|
} |
||||||
|
return R.status(wrpSpgSpqnmpService.save(wrpSpgSpqnmp)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 修改 |
||||||
|
*/ |
||||||
|
@PostMapping("/update") |
||||||
|
@ApiOperationSupport(order = 5) |
||||||
|
@ApiOperation(value = "修改", notes = "传入wrpSpgSpqnmp") |
||||||
|
public R update(@RequestBody WrpSpgSpqnmp wrpSpgSpqnmp) { |
||||||
|
return R.status(wrpSpgSpqnmpService.updateById(wrpSpgSpqnmp)); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 删除 |
||||||
|
*/ |
||||||
|
@PostMapping("/remove") |
||||||
|
@ApiOperationSupport(order = 6) |
||||||
|
@ApiOperation(value = "逻辑删除", notes = "ids") |
||||||
|
public R remove(@ApiParam(value = "主键集合") @RequestParam String ids) { |
||||||
|
boolean temp = wrpSpgSpqnmpService.deleteLogic(Func.toLongList(ids)); |
||||||
|
return R.status(temp); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,28 @@ |
|||||||
|
package com.hnac.hzims.damsafety.feign; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSectionB; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpSectionBService; |
||||||
|
import com.hnac.hzims.damsafety.util.ParamUtil; |
||||||
|
import com.hnac.hzims.damsafety.vo.WrpSectionBVO; |
||||||
|
import com.hnac.hzims.damsafety.wrapper.WrpSectionBWrapper; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||||
|
import org.springframework.web.bind.annotation.RestController; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@RestController |
||||||
|
public class WrpSectionBClient implements IWrpSectionBClient { |
||||||
|
|
||||||
|
@Autowired |
||||||
|
private IWrpSectionBService wrpSectionBService; |
||||||
|
|
||||||
|
@Override |
||||||
|
public R<List<WrpSectionBVO>> list(Map<String, Object> param) { |
||||||
|
LambdaQueryWrapper<WrpSectionB> wrapper= ParamUtil.conditionWrpSectionB(param); |
||||||
|
List<WrpSectionB> list = wrpSectionBService.list(wrapper); |
||||||
|
return R.data(WrpSectionBWrapper.build().listVO(list)); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.TBuilding; |
||||||
|
import org.springblade.core.datascope.mapper.UserDataScopeBaseMapper; |
||||||
|
|
||||||
|
public interface TbBuildingMapper extends UserDataScopeBaseMapper<TBuilding> { |
||||||
|
} |
@ -0,0 +1,5 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.TbBuildingMapper"> |
||||||
|
|
||||||
|
</mapper> |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.TProjInfo; |
||||||
|
import org.springblade.core.datascope.mapper.UserDataScopeBaseMapper; |
||||||
|
|
||||||
|
public interface TbProjInfoMapper extends UserDataScopeBaseMapper<TProjInfo> { |
||||||
|
} |
@ -0,0 +1,5 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.TbProjInfoMapper"> |
||||||
|
|
||||||
|
</mapper> |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
||||||
|
import com.hnac.hzims.damsafety.entity.TSectionConfig; |
||||||
|
|
||||||
|
public interface TbSectionConfigMapper extends BaseMapper<TSectionConfig> { |
||||||
|
} |
@ -0,0 +1,5 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.TbSectionConfigMapper"> |
||||||
|
|
||||||
|
</mapper> |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.mapper.BaseMapper; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDamsB; |
||||||
|
|
||||||
|
public interface WrpDamsBMapper extends BaseMapper<WrpDamsB> { |
||||||
|
} |
@ -0,0 +1,5 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.WrpDamsBMapper"> |
||||||
|
|
||||||
|
</mapper> |
@ -0,0 +1,12 @@ |
|||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrhrdsmp; |
||||||
|
import org.apache.ibatis.annotations.Param; |
||||||
|
import org.springblade.core.datascope.mapper.UserDataScopeBaseMapper; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface WrpDfrSrhrdsmpMapper extends UserDataScopeBaseMapper<WrpDfrSrhrdsmp> { |
||||||
|
List<Map<String, Object>> section(@Param("deptId") String deptId, @Param("rscd") String rscd); |
||||||
|
} |
@ -0,0 +1,23 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.WrpDfrSrhrdsmpMapper"> |
||||||
|
<select id="section" resultType="java.util.Map"> |
||||||
|
SELECT |
||||||
|
srhrdsmp.STCD as stcd, |
||||||
|
srhrdsmp.MPCD as mpcd, |
||||||
|
sec.DAMCD as damcd, |
||||||
|
sec.DAMNM as damnm, |
||||||
|
sec.RSCD as rscd |
||||||
|
FROM |
||||||
|
wrp_dfr_srhrdsmp srhrdsmp |
||||||
|
LEFT JOIN wrp_section_b sec ON srhrdsmp.DAMCD = sec.DAMCD |
||||||
|
WHERE |
||||||
|
srhrdsmp.IS_DELETED =0 |
||||||
|
<if test=" deptId!=null and deptId!='' "> |
||||||
|
and srhrdsmp.create_dept like concat('',#{deptId},'%') |
||||||
|
</if> |
||||||
|
<if test=" rscd!=null and rscd!='' "> |
||||||
|
and sec.RSCD = #{rscd} |
||||||
|
</if> |
||||||
|
</select> |
||||||
|
</mapper> |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsbp; |
||||||
|
import org.springblade.core.datascope.mapper.UserDataScopeBaseMapper; |
||||||
|
|
||||||
|
public interface WrpDfrSrvrdsbpMapper extends UserDataScopeBaseMapper<WrpDfrSrvrdsbp> { |
||||||
|
} |
@ -0,0 +1,5 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.WrpDfrSrvrdsbpMapper"> |
||||||
|
|
||||||
|
</mapper> |
@ -0,0 +1,13 @@ |
|||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsmp; |
||||||
|
import org.apache.ibatis.annotations.Param; |
||||||
|
import org.springblade.core.datascope.mapper.UserDataScopeBaseMapper; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface WrpDfrSrvrdsmpMapper extends UserDataScopeBaseMapper<WrpDfrSrvrdsmp> { |
||||||
|
List<Map<String, Object>> section(@Param("deptId") String deptId,@Param("rscd") String rscd); |
||||||
|
} |
||||||
|
|
@ -0,0 +1,23 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.WrpDfrSrvrdsmpMapper"> |
||||||
|
<select id="section" resultType="java.util.Map"> |
||||||
|
SELECT |
||||||
|
srvrdsmp.STCD as stcd, |
||||||
|
srvrdsmp.MPCD as mpcd, |
||||||
|
sec.DAMCD as damcd, |
||||||
|
sec.DAMNM as damnm, |
||||||
|
sec.RSCD as rscd |
||||||
|
FROM |
||||||
|
wrp_dfr_srvrdsmp srvrdsmp |
||||||
|
LEFT JOIN wrp_section_b sec ON srvrdsmp.DAMCD = sec.DAMCD |
||||||
|
WHERE |
||||||
|
srvrdsmp.IS_DELETED =0 |
||||||
|
<if test=" deptId!=null and deptId!='' "> |
||||||
|
and srvrdsmp.create_dept like concat('',#{deptId},'%') |
||||||
|
</if> |
||||||
|
<if test=" rscd!=null and rscd!='' "> |
||||||
|
and sec.RSCD = #{rscd} |
||||||
|
</if> |
||||||
|
</select> |
||||||
|
</mapper> |
@ -0,0 +1,27 @@ |
|||||||
|
/* |
||||||
|
* Copyright (c) 2018-2028, Chill Zhuang All rights reserved. |
||||||
|
* |
||||||
|
* Redistribution and use in source and binary forms, with or without |
||||||
|
* modification, are permitted provided that the following conditions are met: |
||||||
|
* |
||||||
|
* Redistributions of source code must retain the above copyright Example, |
||||||
|
* this list of conditions and the following disclaimer. |
||||||
|
* Redistributions in binary form must reproduce the above copyright |
||||||
|
* Example, this list of conditions and the following disclaimer in the |
||||||
|
* documentation and/or other materials provided with the distribution. |
||||||
|
* Neither the name of the dreamlu.net developer nor the names of its |
||||||
|
* contributors may be used to endorse or promote products derived from |
||||||
|
* this software without specific prior written permission. |
||||||
|
* Author: Chill 庄骞 (smallchill@163.com) |
||||||
|
*/ |
||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSectionB; |
||||||
|
import org.springblade.core.datascope.mapper.UserDataScopeBaseMapper; |
||||||
|
|
||||||
|
/** |
||||||
|
* Mapper 接口 请不要使用TSectionMapper,否则扫描到的是TSectionMapper 而不是 tSectionMapper 导致找不到mapper |
||||||
|
* @author Chill |
||||||
|
*/ |
||||||
|
public interface WrpSectionBMapper extends UserDataScopeBaseMapper<WrpSectionB> { |
||||||
|
} |
@ -0,0 +1,42 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.WrpSectionBMapper"> |
||||||
|
|
||||||
|
<!-- 通用查询映射结果 |
||||||
|
<resultMap id="TSectionResultMap" type="com.hnac.hzims.damsafety.entity.WrpSectionB"> |
||||||
|
<result column="ID" property="id"/> |
||||||
|
<result column="CREATE_USER" property="createUser"/> |
||||||
|
<result column="CREATE_TIME" property="createTime"/> |
||||||
|
<result column="UPDATE_USER" property="updateUser"/> |
||||||
|
<result column="UPDATE_TIME" property="updateTime"/> |
||||||
|
<result column="STATUS" property="status"/> |
||||||
|
<result column="IS_DELETED" property="isDeleted"/> |
||||||
|
|
||||||
|
<result column="SECTION_NAME" property="sectionName"/> |
||||||
|
<result column="RZ" property="rz"/> |
||||||
|
<result column="BLRZ" property="blrz"/> |
||||||
|
<result column="DRP" property="drp"/> |
||||||
|
<result column="XZHOU" property="xzhou"/> |
||||||
|
<result column="ATTACH" property="attach"/> |
||||||
|
</resultMap>--> |
||||||
|
|
||||||
|
<!-- 通用查询结果列 |
||||||
|
<sql id="baseColumnList"> |
||||||
|
ID, |
||||||
|
SECTION_NAME, |
||||||
|
RZ, |
||||||
|
BLRZ, |
||||||
|
DRP, |
||||||
|
XZHOU, |
||||||
|
ATTACH, |
||||||
|
CREATE_USER, |
||||||
|
CREATE_DEPT, |
||||||
|
CREATE_TIME, |
||||||
|
UPDATE_USER, |
||||||
|
UPDATE_TIME, |
||||||
|
STATUS, |
||||||
|
IS_DELETED |
||||||
|
</sql>--> |
||||||
|
|
||||||
|
|
||||||
|
</mapper> |
@ -0,0 +1,12 @@ |
|||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgPztb; |
||||||
|
import org.apache.ibatis.annotations.Param; |
||||||
|
import org.springblade.core.datascope.mapper.UserDataScopeBaseMapper; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface WrpSpgPztbMapper extends UserDataScopeBaseMapper<WrpSpgPztb> { |
||||||
|
List<Map<String, Object>> section(@Param("deptId") String deptId,@Param("rscd") String rscd); |
||||||
|
} |
@ -0,0 +1,23 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.WrpSpgPztbMapper"> |
||||||
|
<select id="section" resultType="java.util.Map"> |
||||||
|
SELECT |
||||||
|
pztb.STCD as stcd, |
||||||
|
pztb.MPCD as mpcd, |
||||||
|
sec.DAMCD as damcd, |
||||||
|
sec.DAMNM as damnm, |
||||||
|
sec.RSCD as rscd |
||||||
|
FROM |
||||||
|
wrp_spg_pztb pztb |
||||||
|
LEFT JOIN wrp_section_b sec ON pztb.DAMCD = sec.DAMCD |
||||||
|
WHERE |
||||||
|
pztb.IS_DELETED =0 |
||||||
|
<if test=" deptId!=null and deptId!='' "> |
||||||
|
and pztb.create_dept like concat('',#{deptId},'%') |
||||||
|
</if> |
||||||
|
<if test=" rscd!=null and rscd!='' "> |
||||||
|
and sec.RSCD = #{rscd} |
||||||
|
</if> |
||||||
|
</select> |
||||||
|
</mapper> |
@ -0,0 +1,12 @@ |
|||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgSpprmp; |
||||||
|
import org.apache.ibatis.annotations.Param; |
||||||
|
import org.springblade.core.datascope.mapper.UserDataScopeBaseMapper; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface WrpSpgSpprmpMapper extends UserDataScopeBaseMapper<WrpSpgSpprmp> { |
||||||
|
List<Map<String, Object>> section(@Param("deptId") String deptId,@Param("rscd") String rscd); |
||||||
|
} |
@ -0,0 +1,23 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.WrpSpgSpprmpMapper"> |
||||||
|
<select id="section" resultType="java.util.HashMap"> |
||||||
|
SELECT |
||||||
|
spprmp.STCD as stcd, |
||||||
|
spprmp.MPCD as mpcd, |
||||||
|
sec.DAMCD as damcd, |
||||||
|
sec.DAMNM as damnm, |
||||||
|
sec.RSCD as rscd |
||||||
|
FROM |
||||||
|
wrp_spg_spprmp spprmp |
||||||
|
LEFT JOIN wrp_section_b sec ON spprmp.DAMCD = sec.DAMCD |
||||||
|
WHERE |
||||||
|
spprmp.IS_DELETED =0 |
||||||
|
<if test=" deptId!=null and deptId!='' "> |
||||||
|
and spprmp.create_dept like concat('',#{deptId},'%') |
||||||
|
</if> |
||||||
|
<if test=" rscd!=null and rscd!='' "> |
||||||
|
and sec.RSCD = #{rscd} |
||||||
|
</if> |
||||||
|
</select> |
||||||
|
</mapper> |
@ -0,0 +1,13 @@ |
|||||||
|
package com.hnac.hzims.damsafety.mapper; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgSpqnmp; |
||||||
|
import org.apache.ibatis.annotations.Param; |
||||||
|
import org.springblade.core.datascope.mapper.UserDataScopeBaseMapper; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface WrpSpgSpqnmpMapper extends UserDataScopeBaseMapper<WrpSpgSpqnmp> { |
||||||
|
List<Map<String, Object>> section(@Param("deptId") String deptId,@Param("rscd") String rscd); |
||||||
|
} |
||||||
|
|
@ -0,0 +1,23 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.damsafety.mapper.WrpSpgSpqnmpMapper"> |
||||||
|
<select id="section" resultType="java.util.Map"> |
||||||
|
SELECT |
||||||
|
spqnmp.STCD as stcd, |
||||||
|
spqnmp.MPCD as mpcd, |
||||||
|
sec.DAMCD as damcd, |
||||||
|
sec.DAMNM as damnm, |
||||||
|
sec.RSCD as rscd |
||||||
|
FROM |
||||||
|
wrp_spg_spqnmp spqnmp |
||||||
|
LEFT JOIN wrp_section_b sec ON spqnmp.DAMCD = sec.DAMCD |
||||||
|
WHERE |
||||||
|
spqnmp.IS_DELETED =0 |
||||||
|
<if test=" deptId!=null and deptId!='' "> |
||||||
|
and spqnmp.create_dept like concat('',#{deptId},'%') |
||||||
|
</if> |
||||||
|
<if test=" rscd!=null and rscd!='' "> |
||||||
|
and sec.RSCD = #{rscd} |
||||||
|
</if> |
||||||
|
</select> |
||||||
|
</mapper> |
@ -0,0 +1,25 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.entity.Report; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.domain.FieldsData; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.vo.AnalyzeInstanceFieldVO; |
||||||
|
import com.hnac.hzinfo.sdk.analyse.vo.DeviceSinglePropsValueVO; |
||||||
|
|
||||||
|
import java.util.Date; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface IDamSafetyDataService { |
||||||
|
Report getReportData(List<String> deviceCodes, String type, Integer accessRules, Date begin, Date end, String col); |
||||||
|
List<DeviceSinglePropsValueVO> getSeepageLine(List<String> deviceCodes, Date begin, Date end, String col); |
||||||
|
Report getReportDataByDeviceCode(String deviceCode, String type, Integer accessRules, Date begin, Date end); |
||||||
|
List<AnalyzeInstanceFieldVO> getSignages(String stcd); |
||||||
|
|
||||||
|
Map<String,Object> getYearSpprReport(String rscd,List<String> deviceCodes, Date begin, Date end,String col); |
||||||
|
Map<String,Object> getYearHrdsReport(List<String> deviceCodes,Date begin, Date end); |
||||||
|
Report getYearVrdsReport(List<String> deviceCodes,Date begin, Date end,String col); |
||||||
|
|
||||||
|
Map<String,Object> getDamReport(List<String> deviceCodes,String type, Date begin, Date end,String col,int yearGap); |
||||||
|
|
||||||
|
List<FieldsData> getRealData(String deviceCode); |
||||||
|
} |
@ -0,0 +1,10 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import org.springframework.web.multipart.MultipartFile; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
public interface IFileUploadService { |
||||||
|
String addFile(MultipartFile file,String childPath); |
||||||
|
List<String> addFiles(MultipartFile[] files,String childPath); |
||||||
|
} |
@ -0,0 +1,8 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.TBuilding; |
||||||
|
import org.springblade.core.mp.base.BaseService; |
||||||
|
|
||||||
|
|
||||||
|
public interface ITBuildingService extends BaseService<TBuilding> { |
||||||
|
} |
@ -0,0 +1,7 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.TProjInfo; |
||||||
|
import org.springblade.core.mp.base.BaseService; |
||||||
|
|
||||||
|
public interface ITProjInfoService extends BaseService<TProjInfo> { |
||||||
|
} |
@ -0,0 +1,23 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.hnac.hzims.damsafety.entity.TSectionConfig; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
public interface ITSectionConfigService{ |
||||||
|
IPage<TSectionConfig> page(IPage<TSectionConfig> page, LambdaQueryWrapper<TSectionConfig> wrapper); |
||||||
|
|
||||||
|
List<TSectionConfig> list(LambdaQueryWrapper<TSectionConfig> wrapper); |
||||||
|
|
||||||
|
TSectionConfig getOne(QueryWrapper<TSectionConfig> wrapper); |
||||||
|
|
||||||
|
boolean save(TSectionConfig entity); |
||||||
|
|
||||||
|
boolean updateById(TSectionConfig entity); |
||||||
|
|
||||||
|
boolean delete(Wrapper<TSectionConfig> wrapper); |
||||||
|
} |
@ -0,0 +1,23 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDamsB; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
public interface IWrpDamsBService { |
||||||
|
IPage<WrpDamsB> page(IPage<WrpDamsB> page, LambdaQueryWrapper<WrpDamsB> wrapper); |
||||||
|
|
||||||
|
List<WrpDamsB> list(LambdaQueryWrapper<WrpDamsB> wrapper); |
||||||
|
|
||||||
|
WrpDamsB getOne(QueryWrapper<WrpDamsB> wrapper); |
||||||
|
|
||||||
|
boolean save(WrpDamsB entity); |
||||||
|
|
||||||
|
boolean updateById(WrpDamsB entity); |
||||||
|
|
||||||
|
boolean delete(Wrapper<WrpDamsB> wrapper); |
||||||
|
} |
@ -0,0 +1,12 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrhrdsmp; |
||||||
|
import org.springblade.core.mp.base.BaseService; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface IWrpDfrSrhrdsmpService extends BaseService<WrpDfrSrhrdsmp> { |
||||||
|
List<Map<String,Object>> section(String deptId,String rscd); |
||||||
|
List<Map<String, Object>> getSection(String deptId,String rscd); |
||||||
|
} |
@ -0,0 +1,8 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsbp; |
||||||
|
import org.springblade.core.mp.base.BaseService; |
||||||
|
|
||||||
|
public interface IWrpDfrSrvrdsbpService extends BaseService<WrpDfrSrvrdsbp> { |
||||||
|
|
||||||
|
} |
@ -0,0 +1,12 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsmp; |
||||||
|
import org.springblade.core.mp.base.BaseService; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface IWrpDfrSrvrdsmpService extends BaseService<WrpDfrSrvrdsmp> { |
||||||
|
List<Map<String,Object>> section(String deptId,String rscd); |
||||||
|
List<Map<String,Object>> getSection(String deptId,String rscd); |
||||||
|
} |
@ -0,0 +1,28 @@ |
|||||||
|
/* |
||||||
|
* Copyright (c) 2018-2028, Chill Zhuang All rights reserved. |
||||||
|
* |
||||||
|
* Redistribution and use in source and binary forms, with or without |
||||||
|
* modification, are permitted provided that the following conditions are met: |
||||||
|
* |
||||||
|
* Redistributions of source code must retain the above copyright Example, |
||||||
|
* this list of conditions and the following disclaimer. |
||||||
|
* Redistributions in binary form must reproduce the above copyright |
||||||
|
* Example, this list of conditions and the following disclaimer in the |
||||||
|
* documentation and/or other materials provided with the distribution. |
||||||
|
* Neither the name of the dreamlu.net developer nor the names of its |
||||||
|
* contributors may be used to endorse or promote products derived from |
||||||
|
* this software without specific prior written permission. |
||||||
|
* Author: Chill 庄骞 (smallchill@163.com) |
||||||
|
*/ |
||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSectionB; |
||||||
|
import org.springblade.core.mp.base.BaseService; |
||||||
|
|
||||||
|
/** |
||||||
|
* 服务类 |
||||||
|
* |
||||||
|
* @author Chill |
||||||
|
*/ |
||||||
|
public interface IWrpSectionBService extends BaseService<WrpSectionB> { |
||||||
|
} |
@ -0,0 +1,12 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgPztb; |
||||||
|
import org.springblade.core.mp.base.BaseService; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface IWrpSpgPztbService extends BaseService<WrpSpgPztb> { |
||||||
|
List<Map<String,Object>> section(String deptId,String rscd); |
||||||
|
List<Map<String,Object>> getSection(String deptId,String rscd); |
||||||
|
} |
@ -0,0 +1,12 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgSpprmp; |
||||||
|
import org.springblade.core.mp.base.BaseService; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface IWrpSpgSpprmpService extends BaseService<WrpSpgSpprmp> { |
||||||
|
List<Map<String,Object>> section(String deptId,String rscd); |
||||||
|
List<Map<String,Object>> getSection(String deptId,String rscd); |
||||||
|
} |
@ -0,0 +1,13 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgSpqnmp; |
||||||
|
import org.springblade.core.mp.base.BaseService; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public interface IWrpSpgSpqnmpService extends BaseService<WrpSpgSpqnmp> { |
||||||
|
List<Map<String,Object>> section(String deptId,String rscd); |
||||||
|
List<Map<String, Object>> getSection(String deptId,String rscd); |
||||||
|
} |
||||||
|
|
@ -0,0 +1,647 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.service.IDamSafetyDataService; |
||||||
|
import com.hnac.hzims.entity.Report; |
||||||
|
import com.hnac.hzims.entity.ReportData; |
||||||
|
import com.hnac.hzims.enums.QueryDateTypeEnum; |
||||||
|
import com.hnac.hzims.util.CommonUtil; |
||||||
|
import com.hnac.hzims.util.ReportDateUtil; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.domain.FieldsData; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.po.AnalyseCodeByAnalyseDataPO; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.po.AnalyzeDataConditionPO; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.vo.AnalyseDataTaosVO; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.vo.AnalyzeDataConditionVO; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.vo.AnalyzeInstanceFieldVO; |
||||||
|
import com.hnac.hzinfo.datasearch.real.po.RealDataSearchPO; |
||||||
|
import com.hnac.hzinfo.sdk.analyse.AnalyseDataSearchClient; |
||||||
|
import com.hnac.hzinfo.sdk.analyse.po.ComputeBaseStrategyPO; |
||||||
|
import com.hnac.hzinfo.sdk.analyse.vo.DeviceSinglePropsValueQuery; |
||||||
|
import com.hnac.hzinfo.sdk.analyse.vo.DeviceSinglePropsValueVO; |
||||||
|
import com.hnac.hzinfo.sdk.core.response.Result; |
||||||
|
import com.hnac.hzinfo.sdk.real.RealDataSearchClient; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import java.math.BigDecimal; |
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.time.ZoneId; |
||||||
|
import java.util.*; |
||||||
|
|
||||||
|
@Service |
||||||
|
@Slf4j |
||||||
|
public class DamSafetyDataServiceImpl implements IDamSafetyDataService { |
||||||
|
|
||||||
|
@Autowired |
||||||
|
AnalyseDataSearchClient analyseDataSearchClient; |
||||||
|
|
||||||
|
@Autowired |
||||||
|
RealDataSearchClient realDataSearchClient; |
||||||
|
|
||||||
|
@Override |
||||||
|
public Report getReportData(List<String> deviceCodes, String type, Integer accessRules, Date begin, Date end, String col) { |
||||||
|
//检查数据是否正常并转化
|
||||||
|
final QueryDateTypeEnum dtEnum = CommonUtil.checkType(type); |
||||||
|
if(deviceCodes==null || deviceCodes.size()<=0){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
if(dtEnum ==null){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
//初始化报表
|
||||||
|
Report report = new Report(); |
||||||
|
report.initBaseReport(deviceCodes.size(), begin, end, dtEnum); |
||||||
|
//设置基础数据
|
||||||
|
for (int i = 0; i < deviceCodes.size(); i++) { |
||||||
|
String deviceCode = deviceCodes.get(i); |
||||||
|
//获取数据库某个站点不同时间要求的数据
|
||||||
|
List<ReportData> timeDataList = listDataReport(begin,end,dtEnum,deviceCode,accessRules,col); |
||||||
|
|
||||||
|
for (ReportData reportData : timeDataList) { |
||||||
|
String value=reportData.getVal(); |
||||||
|
// if(value!=null && !"-".equals(value) && !"".equals(value.trim())){
|
||||||
|
// value = new BigDecimal(value).setScale(3,BigDecimal.ROUND_DOWN).toString();
|
||||||
|
// }
|
||||||
|
if(value == null){ |
||||||
|
value = "-"; |
||||||
|
} |
||||||
|
report.setBaseCell(reportData.getKeyStr(), i, value); |
||||||
|
} |
||||||
|
} |
||||||
|
return report; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<DeviceSinglePropsValueVO> getSeepageLine(List<String> deviceCodes, Date begin, Date end, String col) { |
||||||
|
return getEightHourData(deviceCodes,"0",begin,end,col); |
||||||
|
} |
||||||
|
|
||||||
|
List<DeviceSinglePropsValueVO> getEightHourData(List<String> deviceCodes, String accessRules,Date begin, Date end,String col){ |
||||||
|
DeviceSinglePropsValueQuery query=new DeviceSinglePropsValueQuery(); |
||||||
|
query.setDeviceCodes(deviceCodes); |
||||||
|
query.setSignage(col); |
||||||
|
query.setAccessRule(accessRules); |
||||||
|
query.setBeginTime(begin); |
||||||
|
query.setEndTime(end); |
||||||
|
Result<List<DeviceSinglePropsValueVO>> result=analyseDataSearchClient.getValueOfBacthDeviceCode(query); |
||||||
|
if(result==null || !result.isSuccess()){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
List<DeviceSinglePropsValueVO> list=result.getData(); |
||||||
|
return list; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<AnalyzeInstanceFieldVO> getSignages(String stcd){ |
||||||
|
//查询列
|
||||||
|
Result<List<AnalyzeInstanceFieldVO>> result=analyseDataSearchClient.getInstanceFieldByAnalyseCode(stcd,1,""); |
||||||
|
if(result==null || !result.isSuccess()){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
List<AnalyzeInstanceFieldVO> analyzeInstanceFieldVOS=result.getData(); |
||||||
|
return analyzeInstanceFieldVOS; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public Map<String,Object> getYearSpprReport(String rscd,List<String> deviceCodes, Date begin, Date end,String col) { |
||||||
|
String type="day"; |
||||||
|
Report report=getYearDataReport(deviceCodes,type,begin,end,col); |
||||||
|
|
||||||
|
//根据水库模型获取数据
|
||||||
|
Report data=getMaxMinByDeviceCode(rscd,type,6,begin,end); |
||||||
|
Map<String,Object> res=new HashMap<>(); |
||||||
|
res.put("spprData",report); |
||||||
|
res.put("reservoirData",data); |
||||||
|
return res; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public Map<String,Object> getYearHrdsReport(List<String> deviceCodes, Date begin, Date end) { |
||||||
|
Report xData=getYearDataReport(deviceCodes,"day",begin,end,"xhrds"); |
||||||
|
Report yData=getYearDataReport(deviceCodes,"day",begin,end,"yhrds"); |
||||||
|
Map<String,Object> res=new HashMap<>(); |
||||||
|
res.put("xData",xData); |
||||||
|
res.put("yData",yData); |
||||||
|
return res; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public Report getYearVrdsReport(List<String> deviceCodes, Date begin, Date end, String col) { |
||||||
|
return getYearDataReport(deviceCodes,"day",begin,end,col); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public Map<String,Object> getDamReport(List<String> deviceCodes,String type, Date begin, Date end, String col,int yearGap) { |
||||||
|
Map<String,Object> res=new HashMap<>(); |
||||||
|
Report report=getYearDataReport(deviceCodes,type,begin,end,col); |
||||||
|
res.put("nowData",report); |
||||||
|
//历史时间对应的历史数据
|
||||||
|
Date historyDate=CommonUtil.getHistoryDate(begin,yearGap); |
||||||
|
Report historyData=getHistoryData(deviceCodes,"year", historyDate, end, col); |
||||||
|
res.put("historyData",historyData); |
||||||
|
return res; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<FieldsData> getRealData(String stcd) { |
||||||
|
RealDataSearchPO po=new RealDataSearchPO(); |
||||||
|
po.setAnalyzeCode(stcd); |
||||||
|
List<AnalyzeInstanceFieldVO> list=getSignages(stcd); |
||||||
|
if(list==null && list.isEmpty()){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
List<String> signages=new ArrayList<>(); |
||||||
|
for(AnalyzeInstanceFieldVO vo:list){ |
||||||
|
signages.add(vo.getSignage()); |
||||||
|
} |
||||||
|
po.setSignages(signages); |
||||||
|
Result<List<FieldsData>> result=realDataSearchClient.getRealDataByDeviceCode(po); |
||||||
|
return result.getData(); |
||||||
|
} |
||||||
|
|
||||||
|
public Report getHistoryData(List<String> deviceCodes,String type, Date begin, Date end, String col){ |
||||||
|
//检查数据是否正常并转化
|
||||||
|
final QueryDateTypeEnum dtEnum = CommonUtil.checkType(type); |
||||||
|
if(deviceCodes==null || deviceCodes.size()<=0){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
int count=deviceCodes.size(); |
||||||
|
//初始化报表
|
||||||
|
Report report = new Report(); |
||||||
|
report.initBaseReportHistory(count); |
||||||
|
//设置基础数据
|
||||||
|
for (int i = 0; i < count; i++) { |
||||||
|
String deviceCode = deviceCodes.get(i); |
||||||
|
// List<ReportData> dataList = listDataReport(param,dtEnum,deviceCode,1,col);
|
||||||
|
// dealMaxMin(report,i,dataList);
|
||||||
|
//获取数据库某个站点不同时间要求的数据
|
||||||
|
List<ReportData> maxList = listDataReport(begin, end,dtEnum,deviceCode,1,col); |
||||||
|
List<ReportData> minList = listDataReport(begin, end,dtEnum,deviceCode,2,col); |
||||||
|
List<ReportData> avgList = listDataReport(begin, end,dtEnum,deviceCode,3,col); |
||||||
|
dealHistoryMaxMin(report,i,maxList,minList,avgList); |
||||||
|
} |
||||||
|
return report; |
||||||
|
} |
||||||
|
|
||||||
|
private void dealHistoryMaxMin(Report report ,int i,List<ReportData> maxList,List<ReportData> minList,List<ReportData> avgList){ |
||||||
|
BigDecimal maxValue=null; |
||||||
|
BigDecimal minValue=null; |
||||||
|
BigDecimal avg=null; |
||||||
|
if(maxList!=null && !maxList.isEmpty()){ |
||||||
|
ReportData maxObj=maxList.get(0); |
||||||
|
if(maxObj!=null && maxObj.getValue()!=null) { |
||||||
|
maxValue = new BigDecimal(maxObj.getValue()).setScale(3, BigDecimal.ROUND_HALF_UP); |
||||||
|
report.setBaseCell("maxDate", i, ReportDateUtil.getYMDStr(maxObj.getKeyDate())); |
||||||
|
}else{ |
||||||
|
report.setBaseCell("maxDate", i, "-"); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
if(minList!=null && !minList.isEmpty()){ |
||||||
|
ReportData minObj=minList.get(0); |
||||||
|
if(minObj!=null && minObj.getValue()!=null) { |
||||||
|
minValue = new BigDecimal(minObj.getValue()).setScale(3, BigDecimal.ROUND_HALF_UP); |
||||||
|
report.setBaseCell("minDate", i, ReportDateUtil.getYMDStr(minObj.getKeyDate())); |
||||||
|
}else{ |
||||||
|
report.setBaseCell("minDate", i, "-"); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
if(avgList!=null && !avgList.isEmpty()){ |
||||||
|
ReportData avgObj=avgList.get(0); |
||||||
|
if(avgObj!=null && avgObj.getValue()!=null) { |
||||||
|
avg = new BigDecimal(avgObj.getValue()).setScale(3, BigDecimal.ROUND_HALF_UP); |
||||||
|
} |
||||||
|
} |
||||||
|
if(maxValue!=null) { |
||||||
|
report.setBaseCell("max", i, String.valueOf(maxValue)); |
||||||
|
}else{ |
||||||
|
report.setBaseCell("max", i, "-"); |
||||||
|
} |
||||||
|
|
||||||
|
if(minValue!=null) { |
||||||
|
report.setBaseCell("min", i, String.valueOf(minValue)); |
||||||
|
}else{ |
||||||
|
report.setBaseCell("min", i, "-"); |
||||||
|
} |
||||||
|
|
||||||
|
if(avg!=null){ |
||||||
|
report.setBaseCell("avg", i, String.valueOf(avg)); |
||||||
|
}else{ |
||||||
|
report.setBaseCell("avg", i, "-"); |
||||||
|
} |
||||||
|
|
||||||
|
if(maxValue!=null && minValue!=null) { |
||||||
|
BigDecimal range = maxValue.subtract(minValue); |
||||||
|
BigDecimal tmpRang = range.setScale(3, BigDecimal.ROUND_HALF_UP); |
||||||
|
report.setBaseCell("range", i, String.valueOf(tmpRang));//极差
|
||||||
|
}else{ |
||||||
|
report.setBaseCell("range", i, "-");//极差
|
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
private Report getYearDataReport(List<String> deviceCodes,String type, Date begin, Date end, String col){ |
||||||
|
//检查数据是否正常并转化
|
||||||
|
final QueryDateTypeEnum dtEnum = CommonUtil.checkType(type); |
||||||
|
if(deviceCodes==null || deviceCodes.size()<=0){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
int count=deviceCodes.size(); |
||||||
|
//初始化报表
|
||||||
|
Report report = new Report(); |
||||||
|
report.initBaseReportMaxMin(count, begin, end, dtEnum); |
||||||
|
//设置基础数据
|
||||||
|
for (int i = 0; i < count; i++) { |
||||||
|
String deviceCode = deviceCodes.get(i); |
||||||
|
//获取数据库某个站点不同时间要求的数据
|
||||||
|
List<ReportData> timeDataList = listDataReport(begin, end,dtEnum,deviceCode,6,col); |
||||||
|
|
||||||
|
for (ReportData reportData : timeDataList) { |
||||||
|
String value=reportData.getVal(); |
||||||
|
// if(value!=null && !"-".equals(value) && !"".equals(value.trim())){
|
||||||
|
// value = new BigDecimal(value).setScale(3,BigDecimal.ROUND_DOWN).toString();
|
||||||
|
// }
|
||||||
|
if(value == null){ |
||||||
|
value = "-"; |
||||||
|
} |
||||||
|
report.setBaseCell(reportData.getKeyStr(), i, value); |
||||||
|
} |
||||||
|
dealMaxMin(report,i,timeDataList); |
||||||
|
} |
||||||
|
return report; |
||||||
|
} |
||||||
|
|
||||||
|
// private void dealMaxMin(Report report ,int i,List<ReportData> timeDataList){
|
||||||
|
// if(timeDataList==null || timeDataList.isEmpty()){
|
||||||
|
// return;
|
||||||
|
// }
|
||||||
|
//
|
||||||
|
// Optional<ReportData> max = timeDataList.stream().filter(reportData -> reportData.getValue()!=null).max(Comparator.comparingDouble(ReportData::getValue));
|
||||||
|
// ReportData maxObj = max.get();
|
||||||
|
// double maxValue=maxObj.getValue();
|
||||||
|
//
|
||||||
|
// report.setBaseCell("max", i, String.valueOf(maxValue));
|
||||||
|
// report.setBaseCell("maxDate", i, ReportDateUtil.getYMDStr(maxObj.getKeyDate()));
|
||||||
|
//
|
||||||
|
// Optional<ReportData> min = timeDataList.stream().filter(reportData -> reportData.getValue()!=null).min(Comparator.comparingDouble(ReportData::getValue));
|
||||||
|
// ReportData minObj = min.get();
|
||||||
|
// double minValue=minObj.getValue();
|
||||||
|
//
|
||||||
|
// report.setBaseCell("min", i, String.valueOf(minValue));
|
||||||
|
// report.setBaseCell("minDate", i, ReportDateUtil.getYMDStr(minObj.getKeyDate()));
|
||||||
|
//
|
||||||
|
//
|
||||||
|
// Double avgs = timeDataList.stream().filter(reportData -> reportData.getValue()!=null).mapToDouble(ReportData::getValue).average().orElse(0D);
|
||||||
|
// BigDecimal avg=new BigDecimal(String.valueOf(avgs)).setScale(3,BigDecimal.ROUND_HALF_UP);
|
||||||
|
// report.setBaseCell("avg", i, String.valueOf(avg));
|
||||||
|
//
|
||||||
|
// BigDecimal range=new BigDecimal(maxValue).subtract(new BigDecimal(minValue));
|
||||||
|
// BigDecimal tmpRang=range.setScale(3,BigDecimal.ROUND_HALF_UP);
|
||||||
|
// report.setBaseCell("range", i, String.valueOf(tmpRang.abs()));//极差 0或正值
|
||||||
|
//
|
||||||
|
// }
|
||||||
|
|
||||||
|
private void dealMaxMin(Report report ,int i,List<ReportData> timeDataList){ |
||||||
|
if(timeDataList==null || timeDataList.isEmpty()){ |
||||||
|
return; |
||||||
|
} |
||||||
|
|
||||||
|
Optional<ReportData> max = timeDataList.stream().filter(reportData -> reportData.getValue()!=null).max(Comparator.comparingDouble(ReportData::getValue)); |
||||||
|
|
||||||
|
Double maxValue =0.0; |
||||||
|
if(max!=null && max.isPresent()) { |
||||||
|
ReportData maxObj = max.get(); |
||||||
|
maxValue = maxObj.getValue(); |
||||||
|
report.setBaseCell("max", i, String.valueOf(maxValue)); |
||||||
|
report.setBaseCell("maxDate", i, ReportDateUtil.getYMDStr(maxObj.getKeyDate())); |
||||||
|
}else{ |
||||||
|
report.setBaseCell("max", i, "-"); |
||||||
|
report.setBaseCell("maxDate", i, "-"); |
||||||
|
} |
||||||
|
|
||||||
|
Optional<ReportData> min = timeDataList.stream().filter(reportData -> reportData.getValue()!=null).min(Comparator.comparingDouble(ReportData::getValue)); |
||||||
|
|
||||||
|
Double minValue=0.0; |
||||||
|
if(min!=null && min.isPresent()) { |
||||||
|
ReportData minObj = min.get(); |
||||||
|
minValue = minObj.getValue(); |
||||||
|
report.setBaseCell("min", i, String.valueOf(minValue)); |
||||||
|
report.setBaseCell("minDate", i, ReportDateUtil.getYMDStr(minObj.getKeyDate())); |
||||||
|
}else{ |
||||||
|
report.setBaseCell("min", i, "-"); |
||||||
|
report.setBaseCell("minDate", i, "-"); |
||||||
|
} |
||||||
|
|
||||||
|
Double avgs = timeDataList.stream().filter(reportData -> reportData.getValue()!=null).mapToDouble(ReportData::getValue).average().orElse(0D); |
||||||
|
BigDecimal avg=new BigDecimal(String.valueOf(avgs)).setScale(3,BigDecimal.ROUND_HALF_UP); |
||||||
|
report.setBaseCell("avg", i, String.valueOf(avg)); |
||||||
|
|
||||||
|
BigDecimal range=new BigDecimal(maxValue).subtract(new BigDecimal(minValue)); |
||||||
|
BigDecimal tmpRange=range.setScale(3,BigDecimal.ROUND_HALF_UP); |
||||||
|
report.setBaseCell("range", i, String.valueOf(tmpRange));//极差
|
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
// public static void main(String[] args) {
|
||||||
|
// List<Double> dd=new ArrayList<>();
|
||||||
|
// dd.add(null);
|
||||||
|
// dd.add(-18.924);
|
||||||
|
// dd.add(-444.924);
|
||||||
|
//
|
||||||
|
// Optional<Double> max = dd.stream().filter(aDouble -> aDouble!=null).max(Comparator.comparingDouble(Double::doubleValue));
|
||||||
|
// Double maxObj = max.get();
|
||||||
|
// System.out.println(maxObj);
|
||||||
|
//
|
||||||
|
// Optional<Double> min = dd.stream().filter(aDouble -> aDouble!=null).min(Comparator.comparingDouble(Double::doubleValue));
|
||||||
|
// Double minObj = min.get();
|
||||||
|
// System.out.println(minObj);
|
||||||
|
//
|
||||||
|
// Double avgs = dd.stream().filter(reportData -> reportData!=null).mapToDouble(Double::doubleValue).average().orElse(0D);
|
||||||
|
// BigDecimal avg=new BigDecimal(String.valueOf(avgs)).setScale(3,BigDecimal.ROUND_HALF_UP);
|
||||||
|
//
|
||||||
|
// System.out.println(avg);
|
||||||
|
// }
|
||||||
|
|
||||||
|
|
||||||
|
public Report getMaxMinByDeviceCode(String deviceCode, String type, Integer accessRules, Date start, Date end) { |
||||||
|
//检查数据是否正常并转化
|
||||||
|
final QueryDateTypeEnum dtEnum = CommonUtil.checkType(type); |
||||||
|
if(deviceCode==null || "".equals(deviceCode)){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
if(dtEnum ==null){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
//设置基础数据
|
||||||
|
List<AnalyzeInstanceFieldVO> signages=getSignages(deviceCode); |
||||||
|
if(signages==null || signages.isEmpty()){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
//初始化报表
|
||||||
|
Report report = new Report(); |
||||||
|
report.initBaseReportMaxMin(signages.size(), start, end, dtEnum); |
||||||
|
|
||||||
|
//获取数据库某个站点不同时间要求的数据
|
||||||
|
List<ReportData> timeDataList = listDataReport(start, end,dtEnum,deviceCode,accessRules,signages); |
||||||
|
for(int i=0;i<signages.size();i++) { |
||||||
|
String signage=signages.get(i).getSignage(); |
||||||
|
List<ReportData> tmpList=CommonUtil.getReportDataBySignage(timeDataList,signage); |
||||||
|
|
||||||
|
dealMaxMin(report,i,tmpList); |
||||||
|
|
||||||
|
for (ReportData reportData : tmpList) { |
||||||
|
String value = reportData.getVal(); |
||||||
|
// if (value != null && !"-".equals(value) && !"".equals(value.trim())) {
|
||||||
|
// value = new BigDecimal(value).setScale(3, BigDecimal.ROUND_DOWN).toString();
|
||||||
|
// }
|
||||||
|
|
||||||
|
if(value == null){ |
||||||
|
value = "-"; |
||||||
|
} |
||||||
|
|
||||||
|
report.setBaseCell(reportData.getKeyStr(), i, value); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
return report; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public Report getReportDataByDeviceCode(String deviceCode, String type, Integer accessRules, Date start, Date end) { |
||||||
|
//检查数据是否正常并转化
|
||||||
|
final QueryDateTypeEnum dtEnum = CommonUtil.checkType(type); |
||||||
|
if(deviceCode==null || "".equals(deviceCode)){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
if(dtEnum ==null){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
//设置基础数据
|
||||||
|
List<AnalyzeInstanceFieldVO> signages=getSignages(deviceCode); |
||||||
|
if(signages==null || signages.isEmpty()){ |
||||||
|
return null; |
||||||
|
} |
||||||
|
//初始化报表
|
||||||
|
Report report = new Report(); |
||||||
|
// List<Map<String,String>> titles=new ArrayList<>();
|
||||||
|
// for(AnalyzeInstanceFieldVO vo:signages){
|
||||||
|
// Map<String,String> title=new HashMap<>();
|
||||||
|
// title.put(vo.getSignage(),vo.getFieldName());
|
||||||
|
// titles.add(title);
|
||||||
|
// }
|
||||||
|
// report.setTitle(titles);
|
||||||
|
report.initBaseReport(signages.size(), start, end, dtEnum); |
||||||
|
|
||||||
|
//获取数据库某个站点不同时间要求的数据
|
||||||
|
List<ReportData> timeDataList = listDataReport(start, end,dtEnum,deviceCode,accessRules,signages); |
||||||
|
for(int i=0;i<signages.size();i++) { |
||||||
|
String signage=signages.get(i).getSignage(); |
||||||
|
List<ReportData> tmpList=CommonUtil.getReportDataBySignage(timeDataList,signage); |
||||||
|
for (ReportData reportData : tmpList) { |
||||||
|
String value = reportData.getVal(); |
||||||
|
// if (value != null && !"-".equals(value) && !"".equals(value.trim())) {
|
||||||
|
// value = new BigDecimal(value).setScale(3, BigDecimal.ROUND_DOWN).toString();
|
||||||
|
// }
|
||||||
|
|
||||||
|
if(value == null){ |
||||||
|
value = "-"; |
||||||
|
} |
||||||
|
|
||||||
|
report.setBaseCell(reportData.getKeyStr(), i, value); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
return report; |
||||||
|
} |
||||||
|
|
||||||
|
//查询多个code对应的同一个值
|
||||||
|
List<DeviceSinglePropsValueVO> getDataByDeviceCodes(List<String> deviceCodes,Date begin,Date end,String accessRules,String col){ |
||||||
|
DeviceSinglePropsValueQuery query=new DeviceSinglePropsValueQuery(); |
||||||
|
query.setBeginTime(begin); |
||||||
|
query.setEndTime(end); |
||||||
|
query.setDeviceCodes(deviceCodes); |
||||||
|
query.setSignage(col); |
||||||
|
query.setAccessRule(accessRules); |
||||||
|
Result<List<DeviceSinglePropsValueVO>> result=analyseDataSearchClient.getValueOfBacthDeviceCode(query); |
||||||
|
List<DeviceSinglePropsValueVO> datas=result.getData(); |
||||||
|
return datas; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
private List<ReportData> listDataReport(Date begin,Date endSt, QueryDateTypeEnum dtEnum, String deviceCode, Integer accessRules, List<AnalyzeInstanceFieldVO> signages) { |
||||||
|
Integer saveTimeType=2; |
||||||
|
if(QueryDateTypeEnum.DAY.equals(dtEnum)){ |
||||||
|
saveTimeType = 3; |
||||||
|
} |
||||||
|
if(QueryDateTypeEnum.MONTH.equals(dtEnum)){ |
||||||
|
saveTimeType = 5; |
||||||
|
} |
||||||
|
if(QueryDateTypeEnum.YEAR.equals(dtEnum)){ |
||||||
|
saveTimeType = 6; |
||||||
|
} |
||||||
|
|
||||||
|
LocalDateTime start = LocalDateTime.ofInstant(begin.toInstant(), ZoneId.systemDefault()); |
||||||
|
LocalDateTime end = LocalDateTime.ofInstant(endSt.toInstant(), ZoneId.systemDefault()); |
||||||
|
|
||||||
|
return getDataByDeviceCode(deviceCode,accessRules,saveTimeType,start,end,signages); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
private List<ReportData> listDataReport(Date begin,Date endSt,QueryDateTypeEnum dtEnum,String deviceCode,Integer accessRules,String col) { |
||||||
|
|
||||||
|
|
||||||
|
Integer saveTimeType=2; |
||||||
|
if(QueryDateTypeEnum.DAY.equals(dtEnum)){ |
||||||
|
saveTimeType = 3; |
||||||
|
} |
||||||
|
if(QueryDateTypeEnum.MONTH.equals(dtEnum)){ |
||||||
|
saveTimeType = 5; |
||||||
|
} |
||||||
|
if(QueryDateTypeEnum.YEAR.equals(dtEnum)){ |
||||||
|
saveTimeType = 6; |
||||||
|
} |
||||||
|
|
||||||
|
LocalDateTime start = LocalDateTime.ofInstant(begin.toInstant(), ZoneId.systemDefault()); |
||||||
|
LocalDateTime end = LocalDateTime.ofInstant(endSt.toInstant(), ZoneId.systemDefault()); |
||||||
|
//saveTimeType 周期类型: 0-> s(秒) 1->、m(分)、2->h(小时)3->、d(天)4->、w(周)5->、n(自然月)、6->y(自然年)
|
||||||
|
//accessRules 取数规则0=最早值、1=最大值、2=最小值、3=平均值、4=(累计值/和值)、5=(变化值/差值) 6=最新值
|
||||||
|
return getDataByDeviceCode(deviceCode, accessRules, saveTimeType, start, end, col); |
||||||
|
} |
||||||
|
|
||||||
|
List<ReportData> getDataByDeviceCode(String deviceCode,Integer accessRules,Integer saveTimeType, |
||||||
|
LocalDateTime start,LocalDateTime end,List<AnalyzeInstanceFieldVO> signages){ |
||||||
|
List<ReportData> reportData=new ArrayList<>(); |
||||||
|
AnalyseCodeByAnalyseDataPO po=new AnalyseCodeByAnalyseDataPO(); |
||||||
|
po.setDeviceCode(deviceCode); |
||||||
|
|
||||||
|
List<AnalyzeDataConditionPO> signboardConditions=new ArrayList<>(); |
||||||
|
|
||||||
|
for(AnalyzeInstanceFieldVO vo:signages) { |
||||||
|
AnalyzeDataConditionPO conditionPO = new AnalyzeDataConditionPO(); |
||||||
|
conditionPO.setBeginTime(start); |
||||||
|
conditionPO.setEndTime(end); |
||||||
|
conditionPO.setSignages(vo.getSignage()); |
||||||
|
// conditionPO.setKeepFigures(3);
|
||||||
|
conditionPO.setAccessRules(accessRules); |
||||||
|
conditionPO.setSaveTimeType(saveTimeType); |
||||||
|
conditionPO.setTimeInterval(1); |
||||||
|
conditionPO.setFull(1); |
||||||
|
signboardConditions.add(conditionPO); |
||||||
|
} |
||||||
|
|
||||||
|
po.setSignboardConditions(signboardConditions); |
||||||
|
|
||||||
|
Result<List<AnalyzeDataConditionVO>> result=analyseDataSearchClient.getAnalyzeDataByAnalyzeCodeAndSignages(po); |
||||||
|
if(result==null){ |
||||||
|
return reportData; |
||||||
|
} |
||||||
|
List<AnalyzeDataConditionVO> datas=result.getData(); |
||||||
|
if(datas==null || datas.isEmpty()){ |
||||||
|
return reportData; |
||||||
|
} |
||||||
|
for(AnalyzeDataConditionVO vo:datas){ |
||||||
|
List<AnalyseDataTaosVO> dataTaosVOs=vo.getList(); |
||||||
|
for(AnalyseDataTaosVO vv:dataTaosVOs) { |
||||||
|
ReportData data=new ReportData(); |
||||||
|
data.setKeyStr(CommonUtil.getKeyBySaveTimeType(vv.getTs(),saveTimeType)); |
||||||
|
data.setKeyDate(vv.getTs()); |
||||||
|
data.setStcd(vo.getSignage());//测站变成属性值
|
||||||
|
data.setVal(vv.getVal()); |
||||||
|
data.setName(vo.getName()); |
||||||
|
reportData.add(data); |
||||||
|
} |
||||||
|
} |
||||||
|
return reportData; |
||||||
|
} |
||||||
|
|
||||||
|
List<ReportData> getDataByDeviceCode(String deviceCode,Integer accessRules,Integer saveTimeType, |
||||||
|
LocalDateTime start,LocalDateTime end,String col){ |
||||||
|
List<ReportData> reportData=new ArrayList<>(); |
||||||
|
AnalyseCodeByAnalyseDataPO po=new AnalyseCodeByAnalyseDataPO(); |
||||||
|
po.setDeviceCode(deviceCode); |
||||||
|
|
||||||
|
List<AnalyzeDataConditionPO> signboardConditions=new ArrayList<>(); |
||||||
|
AnalyzeDataConditionPO conditionPO=new AnalyzeDataConditionPO(); |
||||||
|
conditionPO.setBeginTime(start); |
||||||
|
conditionPO.setEndTime(end); |
||||||
|
conditionPO.setSignages(col); |
||||||
|
// conditionPO.setKeepFigures(3);
|
||||||
|
conditionPO.setAccessRules(accessRules); |
||||||
|
conditionPO.setSaveTimeType(saveTimeType); |
||||||
|
conditionPO.setTimeInterval(1); |
||||||
|
conditionPO.setFull(1); |
||||||
|
signboardConditions.add(conditionPO); |
||||||
|
po.setSignboardConditions(signboardConditions); |
||||||
|
Result<List<AnalyzeDataConditionVO>> result=analyseDataSearchClient.getAnalyzeDataByAnalyzeCodeAndSignages(po); |
||||||
|
if(result==null){ |
||||||
|
return reportData; |
||||||
|
} |
||||||
|
List<AnalyzeDataConditionVO> datas=result.getData(); |
||||||
|
if(datas==null || datas.isEmpty()){ |
||||||
|
return reportData; |
||||||
|
} |
||||||
|
for(AnalyzeDataConditionVO vo:datas){ |
||||||
|
List<AnalyseDataTaosVO> dataTaosVOs=vo.getList(); |
||||||
|
for (AnalyseDataTaosVO vv : dataTaosVOs) { |
||||||
|
if(vo.getSignage().equals(col)) { |
||||||
|
ReportData data = new ReportData(); |
||||||
|
data.setKeyStr(CommonUtil.getKeyBySaveTimeType(vv.getTs(), saveTimeType)); |
||||||
|
data.setKeyDate(vv.getTs()); |
||||||
|
data.setStcd(deviceCode); |
||||||
|
data.setVal(vv.getVal()); |
||||||
|
data.setName(vo.getName()); |
||||||
|
reportData.add(data); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
return reportData; |
||||||
|
} |
||||||
|
|
||||||
|
//按时间范围求最大值、最小值
|
||||||
|
public List<ReportData> queryMaxOrMin(String deviceCode, Date startTime, Date endTime, |
||||||
|
Integer calcType, String col) { |
||||||
|
List<ReportData> res=new ArrayList<>(); |
||||||
|
|
||||||
|
List<String> signages=new ArrayList<>(); |
||||||
|
signages.add(col); |
||||||
|
ComputeBaseStrategyPO po=new ComputeBaseStrategyPO(); |
||||||
|
LocalDateTime start = LocalDateTime.ofInstant(startTime.toInstant(), ZoneId.systemDefault()); |
||||||
|
po.setBeginTime(start); |
||||||
|
LocalDateTime end = LocalDateTime.ofInstant(endTime.toInstant(), ZoneId.systemDefault()); |
||||||
|
po.setEndTime(end); |
||||||
|
po.setAccessRules(calcType); |
||||||
|
po.setDeviceCode(deviceCode); |
||||||
|
po.setSignages(signages); |
||||||
|
Result<List<AnalyseDataTaosVO>> result=analyseDataSearchClient.getComputeBaseStrategy(po); |
||||||
|
if(result==null){ |
||||||
|
return res; |
||||||
|
} |
||||||
|
List<AnalyseDataTaosVO> datas =result.getData(); |
||||||
|
|
||||||
|
if(datas!=null && !datas.isEmpty()){ |
||||||
|
for(AnalyseDataTaosVO vo:datas) { |
||||||
|
if(col.equals(vo.getSignage())) { |
||||||
|
ReportData reportData=new ReportData(); |
||||||
|
String val=vo.getVal(); |
||||||
|
String tm=vo.getTs(); |
||||||
|
reportData.setKeyStr(tm); |
||||||
|
reportData.setStcd(deviceCode); |
||||||
|
reportData.setVal(val); |
||||||
|
res.add(reportData); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
return res; |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,63 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.service.IFileUploadService; |
||||||
|
import org.apache.commons.io.FileUtils; |
||||||
|
import org.springframework.beans.factory.annotation.Value; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
import org.springframework.web.multipart.MultipartFile; |
||||||
|
|
||||||
|
import java.io.File; |
||||||
|
import java.io.IOException; |
||||||
|
import java.util.ArrayList; |
||||||
|
import java.util.List; |
||||||
|
import java.util.UUID; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class FileUploadServiceImpl implements IFileUploadService { |
||||||
|
@Value("${file.upload.path}") |
||||||
|
String fileUploadPath; |
||||||
|
|
||||||
|
@Override |
||||||
|
public String addFile(MultipartFile file,String childPath) { |
||||||
|
MultipartFile[] files=new MultipartFile[1]; |
||||||
|
files[0] = file; |
||||||
|
return addFiles(files,childPath).get(0); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<String> addFiles(MultipartFile[] files,String childPath){ |
||||||
|
List<String> list=new ArrayList<>(); |
||||||
|
String dirName = UUID.randomUUID().toString().replace("-", ""); |
||||||
|
File uploadPath = new File(fileUploadPath, childPath); |
||||||
|
uploadPath = new File(uploadPath, dirName); |
||||||
|
try { |
||||||
|
if(!uploadPath.exists()){ |
||||||
|
uploadPath.mkdirs(); |
||||||
|
} |
||||||
|
for(MultipartFile file:files){ |
||||||
|
saveFile(file, uploadPath); |
||||||
|
list.add(File.separator+childPath+File.separator+dirName+File.separator+file.getOriginalFilename()); |
||||||
|
} |
||||||
|
return list; |
||||||
|
}catch (IOException e){ |
||||||
|
return list; |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 保存文件,如果后期有要求需要处理重名情况 |
||||||
|
* |
||||||
|
* @param file 待保存的文件 |
||||||
|
* @param uploadPath 上传的路径 |
||||||
|
* @throws IOException |
||||||
|
*/ |
||||||
|
private void saveFile(MultipartFile file, File uploadPath) throws IOException { |
||||||
|
String originalName = file.getOriginalFilename(); |
||||||
|
File path = new File(uploadPath, originalName); |
||||||
|
if(!path.exists()){ |
||||||
|
path.createNewFile(); |
||||||
|
} |
||||||
|
FileUtils.copyInputStreamToFile(file.getInputStream(), path); |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,11 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.TBuilding; |
||||||
|
import com.hnac.hzims.damsafety.mapper.TbBuildingMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.ITBuildingService; |
||||||
|
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class TBuildingServiceImpl extends BaseServiceImpl<TbBuildingMapper, TBuilding> implements ITBuildingService { |
||||||
|
} |
@ -0,0 +1,11 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.TProjInfo; |
||||||
|
import com.hnac.hzims.damsafety.mapper.TbProjInfoMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.ITProjInfoService; |
||||||
|
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class TProjInfoServiceImpl extends BaseServiceImpl<TbProjInfoMapper, TProjInfo> implements ITProjInfoService { |
||||||
|
} |
@ -0,0 +1,53 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.hnac.hzims.damsafety.entity.TSectionConfig; |
||||||
|
import com.hnac.hzims.damsafety.mapper.TbSectionConfigMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.ITSectionConfigService; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import javax.annotation.Resource; |
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class TSectionConfigServiceImpl implements ITSectionConfigService { |
||||||
|
|
||||||
|
@Resource |
||||||
|
TbSectionConfigMapper tbSectionConfigMapper; |
||||||
|
|
||||||
|
@Override |
||||||
|
public IPage<TSectionConfig> page(IPage<TSectionConfig> page, LambdaQueryWrapper<TSectionConfig> wrapper) { |
||||||
|
return tbSectionConfigMapper.selectPage(page,wrapper); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<TSectionConfig> list(LambdaQueryWrapper<TSectionConfig> wrapper) { |
||||||
|
return tbSectionConfigMapper.selectList(wrapper); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public TSectionConfig getOne(QueryWrapper<TSectionConfig> wrapper) { |
||||||
|
return tbSectionConfigMapper.selectOne(wrapper); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public boolean save(TSectionConfig entity) { |
||||||
|
int count=tbSectionConfigMapper.insert(entity); |
||||||
|
return count>0; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public boolean updateById(TSectionConfig entity) { |
||||||
|
int count=tbSectionConfigMapper.updateById(entity); |
||||||
|
return count>0; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public boolean delete(Wrapper<TSectionConfig> wrapper) { |
||||||
|
int count=tbSectionConfigMapper.delete(wrapper); |
||||||
|
return count>0; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,57 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.Wrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.update.LambdaUpdateWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDamsB; |
||||||
|
import com.hnac.hzims.damsafety.mapper.WrpDamsBMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpDamsBService; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import javax.annotation.Resource; |
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class WrpDamsBServiceImpl implements IWrpDamsBService { |
||||||
|
|
||||||
|
@Resource |
||||||
|
WrpDamsBMapper wrpDamsBMapper; |
||||||
|
|
||||||
|
@Override |
||||||
|
public IPage<WrpDamsB> page(IPage<WrpDamsB> page, LambdaQueryWrapper<WrpDamsB> wrapper) { |
||||||
|
return wrpDamsBMapper.selectPage(page,wrapper); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<WrpDamsB> list(LambdaQueryWrapper<WrpDamsB> wrapper) { |
||||||
|
return wrpDamsBMapper.selectList(wrapper); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public WrpDamsB getOne(QueryWrapper<WrpDamsB> wrapper) { |
||||||
|
return wrpDamsBMapper.selectOne(wrapper); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public boolean save(WrpDamsB entity) { |
||||||
|
int count=wrpDamsBMapper.insert(entity); |
||||||
|
return count>0; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public boolean updateById(WrpDamsB entity) { |
||||||
|
LambdaUpdateWrapper<WrpDamsB> wrapper=new LambdaUpdateWrapper(); |
||||||
|
wrapper.eq(WrpDamsB::getDamscd,entity.getDamscd()); |
||||||
|
int count=wrpDamsBMapper.update(entity,wrapper); |
||||||
|
return count>0; |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public boolean delete(Wrapper<WrpDamsB> wrapper) { |
||||||
|
int count=wrpDamsBMapper.delete(wrapper); |
||||||
|
return count>0; |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,30 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrhrdsmp; |
||||||
|
import com.hnac.hzims.damsafety.mapper.WrpDfrSrhrdsmpMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpDfrSrhrdsmpService; |
||||||
|
import com.hnac.hzims.damsafety.util.DealSectionUtil; |
||||||
|
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import javax.annotation.Resource; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class WrpDfrSrhrdsmpServiceImpl extends BaseServiceImpl<WrpDfrSrhrdsmpMapper, WrpDfrSrhrdsmp> implements IWrpDfrSrhrdsmpService { |
||||||
|
@Resource |
||||||
|
WrpDfrSrhrdsmpMapper wrpDfrSrhrdsmpMapper; |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<Map<String, Object>> section(String deptId,String rscd) { |
||||||
|
List<Map<String, Object>> list=wrpDfrSrhrdsmpMapper.section(deptId,rscd); |
||||||
|
return DealSectionUtil.getSection(list); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<Map<String, Object>> getSection(String deptId,String rscd) { |
||||||
|
List<Map<String, Object>> list=wrpDfrSrhrdsmpMapper.section(deptId,rscd); |
||||||
|
return list; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,11 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsbp; |
||||||
|
import com.hnac.hzims.damsafety.mapper.WrpDfrSrvrdsbpMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpDfrSrvrdsbpService; |
||||||
|
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class WrpDfrSrvrdsbpServiceImpl extends BaseServiceImpl<WrpDfrSrvrdsbpMapper, WrpDfrSrvrdsbp> implements IWrpDfrSrvrdsbpService { |
||||||
|
} |
@ -0,0 +1,28 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpDfrSrvrdsmp; |
||||||
|
import com.hnac.hzims.damsafety.mapper.WrpDfrSrvrdsmpMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpDfrSrvrdsmpService; |
||||||
|
import com.hnac.hzims.damsafety.util.DealSectionUtil; |
||||||
|
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import javax.annotation.Resource; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class WrpDfrSrvrdsmpServiceImpl extends BaseServiceImpl<WrpDfrSrvrdsmpMapper, WrpDfrSrvrdsmp> implements IWrpDfrSrvrdsmpService { |
||||||
|
@Resource |
||||||
|
WrpDfrSrvrdsmpMapper wrpDfrSrvrdsmpMapper; |
||||||
|
@Override |
||||||
|
public List<Map<String, Object>> section(String deptId,String rscd) { |
||||||
|
List<Map<String, Object>> list=wrpDfrSrvrdsmpMapper.section(deptId,rscd); |
||||||
|
return DealSectionUtil.getSection(list); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<Map<String, Object>> getSection(String deptId, String rscd) { |
||||||
|
return wrpDfrSrvrdsmpMapper.section(deptId,rscd); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,31 @@ |
|||||||
|
/* |
||||||
|
* Copyright (c) 2018-2028, Chill Zhuang All rights reserved. |
||||||
|
* |
||||||
|
* Redistribution and use in source and binary forms, with or without |
||||||
|
* modification, are permitted provided that the following conditions are met: |
||||||
|
* |
||||||
|
* Redistributions of source code must retain the above copyright Example, |
||||||
|
* this list of conditions and the following disclaimer. |
||||||
|
* Redistributions in binary form must reproduce the above copyright |
||||||
|
* Example, this list of conditions and the following disclaimer in the |
||||||
|
* documentation and/or other materials provided with the distribution. |
||||||
|
* Neither the name of the dreamlu.net developer nor the names of its |
||||||
|
* contributors may be used to endorse or promote products derived from |
||||||
|
* this software without specific prior written permission. |
||||||
|
* Author: Chill 庄骞 (smallchill@163.com) |
||||||
|
*/ |
||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSectionB; |
||||||
|
import com.hnac.hzims.damsafety.mapper.WrpSectionBMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpSectionBService; |
||||||
|
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
/** |
||||||
|
* 服务实现类 |
||||||
|
* @author Chill |
||||||
|
*/ |
||||||
|
@Service |
||||||
|
public class WrpSectionBServiceImpl extends BaseServiceImpl<WrpSectionBMapper, WrpSectionB> implements IWrpSectionBService { |
||||||
|
} |
@ -0,0 +1,30 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgPztb; |
||||||
|
import com.hnac.hzims.damsafety.mapper.WrpSpgPztbMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpSpgPztbService; |
||||||
|
import com.hnac.hzims.damsafety.util.DealSectionUtil; |
||||||
|
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import javax.annotation.Resource; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class WrpSpgPztbServiceImpl extends BaseServiceImpl<WrpSpgPztbMapper, WrpSpgPztb> implements IWrpSpgPztbService { |
||||||
|
|
||||||
|
@Resource |
||||||
|
WrpSpgPztbMapper wrpSpgPztbMapper; |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<Map<String, Object>> section(String deptId,String rscd) { |
||||||
|
List<Map<String, Object>> list=wrpSpgPztbMapper.section(deptId,rscd); |
||||||
|
return DealSectionUtil.getSection(list); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<Map<String, Object>> getSection(String deptId, String rscd) { |
||||||
|
return wrpSpgPztbMapper.section(deptId,rscd); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,32 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgSpprmp; |
||||||
|
import com.hnac.hzims.damsafety.mapper.WrpSpgSpprmpMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpSpgSpprmpService; |
||||||
|
import com.hnac.hzims.damsafety.util.DealSectionUtil; |
||||||
|
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import javax.annotation.Resource; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class WrpSpgSpprmpServiceImpl extends BaseServiceImpl<WrpSpgSpprmpMapper, WrpSpgSpprmp> implements IWrpSpgSpprmpService { |
||||||
|
|
||||||
|
@Resource |
||||||
|
WrpSpgSpprmpMapper wrpSpgSpprmpMapper; |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<Map<String, Object>> section(String deptId,String rscd) { |
||||||
|
List<Map<String, Object>> list=wrpSpgSpprmpMapper.section(deptId,rscd); |
||||||
|
return DealSectionUtil.getSection(list); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<Map<String, Object>> getSection(String deptId, String rscd) { |
||||||
|
return wrpSpgSpprmpMapper.section(deptId,rscd); |
||||||
|
} |
||||||
|
|
||||||
|
} |
||||||
|
|
@ -0,0 +1,30 @@ |
|||||||
|
package com.hnac.hzims.damsafety.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.WrpSpgSpqnmp; |
||||||
|
import com.hnac.hzims.damsafety.mapper.WrpSpgSpqnmpMapper; |
||||||
|
import com.hnac.hzims.damsafety.service.IWrpSpgSpqnmpService; |
||||||
|
import com.hnac.hzims.damsafety.util.DealSectionUtil; |
||||||
|
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import javax.annotation.Resource; |
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
@Service |
||||||
|
public class WrpSpgSpqnmpServiceImpl extends BaseServiceImpl<WrpSpgSpqnmpMapper, WrpSpgSpqnmp> implements IWrpSpgSpqnmpService { |
||||||
|
@Resource |
||||||
|
WrpSpgSpqnmpMapper wrpSpgSpqnmpMapper; |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<Map<String, Object>> section(String deptId,String rscd) { |
||||||
|
List<Map<String, Object>> list=wrpSpgSpqnmpMapper.section(deptId,rscd); |
||||||
|
return DealSectionUtil.getSection(list); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<Map<String, Object>> getSection(String deptId,String rscd) { |
||||||
|
List<Map<String, Object>> list=wrpSpgSpqnmpMapper.section(deptId,rscd); |
||||||
|
return DealSectionUtil.getSection(list); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,48 @@ |
|||||||
|
package com.hnac.hzims.damsafety.util; |
||||||
|
|
||||||
|
import java.util.*; |
||||||
|
|
||||||
|
public class DealSectionUtil { |
||||||
|
public static String getDeviceCode(List<Map<String, Object>> data){ |
||||||
|
StringBuilder sb=new StringBuilder(); |
||||||
|
for(Map<String, Object> dd:data){ |
||||||
|
String stcd=String.valueOf(dd.get("stcd")); |
||||||
|
if("".equals(sb.toString())){ |
||||||
|
sb.append(stcd); |
||||||
|
}else{ |
||||||
|
sb.append(","+stcd); |
||||||
|
} |
||||||
|
} |
||||||
|
return sb.toString(); |
||||||
|
} |
||||||
|
|
||||||
|
public static List<Map<String, Object>> getSection(List<Map<String, Object>> list){ |
||||||
|
List<Map<String, Object>> res=new ArrayList<>(); |
||||||
|
Map<String, List<Map<String, Object>>> map = new LinkedHashMap<>(); |
||||||
|
for(Map<String, Object> ll:list) { |
||||||
|
String damcd=String.valueOf(ll.get("damcd")); |
||||||
|
List<Map<String, Object>> tmpList=map.get(damcd); |
||||||
|
if(tmpList!=null && !tmpList.isEmpty()) { |
||||||
|
tmpList.add(ll); |
||||||
|
map.put(damcd, tmpList); |
||||||
|
}else{ |
||||||
|
tmpList = new ArrayList<>(); |
||||||
|
tmpList.add(ll); |
||||||
|
map.put(damcd, tmpList); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
for (Map.Entry<String, List<Map<String, Object>>> m : map.entrySet()) { |
||||||
|
List<Map<String, Object>> data= m.getValue(); |
||||||
|
Map<String, Object> sj=new HashMap<>(); |
||||||
|
sj.put("damnm",data.get(0).get("damnm")); |
||||||
|
sj.put("rscd",data.get(0).get("rscd")); |
||||||
|
sj.put("damcd",m.getKey()); |
||||||
|
sj.put("deviceCode",getDeviceCode(data)); |
||||||
|
res.add(sj); |
||||||
|
} |
||||||
|
|
||||||
|
return res; |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,177 @@ |
|||||||
|
package com.hnac.hzims.damsafety.util; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.hnac.hzims.damsafety.entity.*; |
||||||
|
|
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
public class ParamUtil { |
||||||
|
public static LambdaQueryWrapper<WrpSectionB> conditionWrpSectionB(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<WrpSectionB> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("rscd")!=null) { |
||||||
|
wrapper.like(WrpSectionB::getRscd,param.get("rscd")); |
||||||
|
} |
||||||
|
if(param.get("buildingId")!=null) { |
||||||
|
wrapper.eq(WrpSectionB::getBuildingId,param.get("buildingId")); |
||||||
|
} |
||||||
|
if(param.get("damcd")!=null) { |
||||||
|
wrapper.like(WrpSectionB::getDamcd,param.get("damcd")); |
||||||
|
} |
||||||
|
|
||||||
|
if(param.get("damnm")!=null) { |
||||||
|
wrapper.like(WrpSectionB::getDamnm,param.get("damnm")); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
if(param.get("wallType")!=null) { |
||||||
|
wrapper.eq(WrpSectionB::getWallType,param.get("wallType")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
public static LambdaQueryWrapper<TBuilding> conditionTBuilding(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<TBuilding> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("rscd")!=null) { |
||||||
|
wrapper.like(TBuilding::getRscd,param.get("rscd")); |
||||||
|
} |
||||||
|
if(param.get("name")!=null) { |
||||||
|
wrapper.like(TBuilding::getName,param.get("name")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
public static LambdaQueryWrapper<TProjInfo> conditionTProjInfo(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<TProjInfo> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("rscd")!=null) { |
||||||
|
wrapper.like(TProjInfo::getRscd,param.get("rscd")); |
||||||
|
} |
||||||
|
if(param.get("projName")!=null) { |
||||||
|
wrapper.like(TProjInfo::getProjName,param.get("projName")); |
||||||
|
} |
||||||
|
if(param.get("projCode")!=null) { |
||||||
|
wrapper.like(TProjInfo::getProjCode,param.get("projCode")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
public static LambdaQueryWrapper<TSectionConfig> conditionTSectionConfig(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<TSectionConfig> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("stcd")!=null) { |
||||||
|
wrapper.like(TSectionConfig::getStcd,param.get("stcd")); |
||||||
|
} |
||||||
|
if(param.get("sectionNo")!=null) { |
||||||
|
wrapper.like(TSectionConfig::getSectionNo,param.get("sectionNo")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
public static LambdaQueryWrapper<WrpDamsB> conditionWrpDamsB(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<WrpDamsB> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("damcd")!=null) { |
||||||
|
wrapper.like(WrpDamsB::getDamcd,param.get("damcd")); |
||||||
|
} |
||||||
|
if(param.get("damscd")!=null) { |
||||||
|
wrapper.like(WrpDamsB::getDamscd,param.get("damscd")); |
||||||
|
} |
||||||
|
if(param.get("damsnm")!=null) { |
||||||
|
wrapper.like(WrpDamsB::getDamscd,param.get("damsnm")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
public static LambdaQueryWrapper<WrpDfrSrhrdsmp> conditionWrpDfrSrhrdsmp(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<WrpDfrSrhrdsmp> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("damcd")!=null) { |
||||||
|
wrapper.like(WrpDfrSrhrdsmp::getDamcd,param.get("damcd")); |
||||||
|
} |
||||||
|
if(param.get("stcd")!=null) { |
||||||
|
wrapper.like(WrpDfrSrhrdsmp::getStcd,param.get("stcd")); |
||||||
|
} |
||||||
|
if(param.get("mpcd")!=null) { |
||||||
|
wrapper.like(WrpDfrSrhrdsmp::getMpcd,param.get("mpcd")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
public static LambdaQueryWrapper<WrpDfrSrvrdsbp> conditionWrpDfrSrvrdsbp(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<WrpDfrSrvrdsbp> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("rscd")!=null) { |
||||||
|
wrapper.like(WrpDfrSrvrdsbp::getRscd,param.get("rscd")); |
||||||
|
} |
||||||
|
if(param.get("hycncd")!=null) { |
||||||
|
wrapper.eq(WrpDfrSrvrdsbp::getHycncd,param.get("hycncd")); |
||||||
|
} |
||||||
|
if(param.get("bpcd")!=null) { |
||||||
|
wrapper.like(WrpDfrSrvrdsbp::getBpcd,param.get("bpcd")); |
||||||
|
} |
||||||
|
if(param.get("bptp")!=null) { |
||||||
|
wrapper.like(WrpDfrSrvrdsbp::getBptp,param.get("bptp")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
public static LambdaQueryWrapper<WrpDfrSrvrdsmp> conditionWrpDfrSrvrdsmp(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<WrpDfrSrvrdsmp> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("damcd")!=null) { |
||||||
|
wrapper.like(WrpDfrSrvrdsmp::getDamcd,param.get("damcd")); |
||||||
|
} |
||||||
|
if(param.get("stcd")!=null) { |
||||||
|
wrapper.like(WrpDfrSrvrdsmp::getStcd,param.get("stcd")); |
||||||
|
} |
||||||
|
if(param.get("mpcd")!=null) { |
||||||
|
wrapper.like(WrpDfrSrvrdsmp::getMpcd,param.get("mpcd")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
public static LambdaQueryWrapper<WrpSpgPztb> conditionWrpSpgPztb(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<WrpSpgPztb> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("damcd")!=null) { |
||||||
|
wrapper.like(WrpSpgPztb::getDamcd,param.get("damcd")); |
||||||
|
} |
||||||
|
if(param.get("stcd")!=null) { |
||||||
|
wrapper.like(WrpSpgPztb::getStcd,param.get("stcd")); |
||||||
|
} |
||||||
|
if(param.get("mpcd")!=null) { |
||||||
|
wrapper.like(WrpSpgPztb::getMpcd,param.get("mpcd")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
public static LambdaQueryWrapper<WrpSpgSpprmp> conditionWrpSpgSpprmp(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<WrpSpgSpprmp> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("damcd")!=null) { |
||||||
|
wrapper.like(WrpSpgSpprmp::getDamcd,param.get("damcd")); |
||||||
|
} |
||||||
|
if(param.get("stcd")!=null) { |
||||||
|
wrapper.like(WrpSpgSpprmp::getStcd,param.get("stcd")); |
||||||
|
} |
||||||
|
if(param.get("mpcd")!=null) { |
||||||
|
wrapper.like(WrpSpgSpprmp::getMpcd,param.get("mpcd")); |
||||||
|
} |
||||||
|
if(param.get("ch")!=null) { |
||||||
|
wrapper.like(WrpSpgSpprmp::getCh,param.get("ch")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
public static LambdaQueryWrapper<WrpSpgSpqnmp> conditionWrpSpgSpqnmp(Map<String, Object> param){ |
||||||
|
LambdaQueryWrapper<WrpSpgSpqnmp> wrapper=new LambdaQueryWrapper(); |
||||||
|
if(param.get("damcd")!=null) { |
||||||
|
wrapper.like(WrpSpgSpqnmp::getDamcd,param.get("damcd")); |
||||||
|
} |
||||||
|
if(param.get("stcd")!=null) { |
||||||
|
wrapper.like(WrpSpgSpqnmp::getStcd,param.get("stcd")); |
||||||
|
} |
||||||
|
if(param.get("mpcd")!=null) { |
||||||
|
wrapper.like(WrpSpgSpqnmp::getMpcd,param.get("mpcd")); |
||||||
|
} |
||||||
|
return wrapper; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,24 @@ |
|||||||
|
package com.hnac.hzims.damsafety.wrapper; |
||||||
|
|
||||||
|
import com.hnac.hzims.damsafety.entity.TBuilding; |
||||||
|
import com.hnac.hzims.damsafety.vo.TBuildingVo; |
||||||
|
import org.springblade.core.mp.support.BaseEntityWrapper; |
||||||
|
import org.springblade.core.tool.utils.BeanUtil; |
||||||
|
|
||||||
|
|
||||||
|
import java.util.Objects; |
||||||
|
|
||||||
|
public class TBuildingWrapper extends BaseEntityWrapper<TBuilding, TBuildingVo> { |
||||||
|
public TBuildingWrapper() { |
||||||
|
} |
||||||
|
|
||||||
|
public static TBuildingWrapper build() { |
||||||
|
return new TBuildingWrapper(); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public TBuildingVo entityVO(TBuilding entity) { |
||||||
|
TBuildingVo tBuildingVo = Objects.requireNonNull(BeanUtil.copy(entity, TBuildingVo.class)); |
||||||
|
return tBuildingVo; |
||||||
|
} |
||||||
|
} |
Some files were not shown because too many files have changed in this diff Show More
Loading…
Reference in new issue