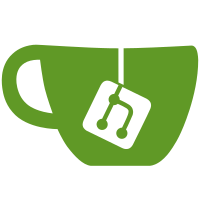
4 changed files with 143 additions and 0 deletions
@ -0,0 +1,123 @@
|
||||
package com.hnac.hzims.scheduled.scheduled; |
||||
|
||||
import com.hnac.hzims.common.logs.utils.StringUtils; |
||||
import com.hnac.hzims.message.MessageConstants; |
||||
import com.hnac.hzims.message.dto.BusinessMessageDTO; |
||||
import com.hnac.hzims.message.fegin.IMessageClient; |
||||
import com.hnac.hzims.scheduled.service.operation.business.impl.DutyServiceImpl; |
||||
import com.xxl.job.core.biz.model.ReturnT; |
||||
import com.xxl.job.core.handler.annotation.XxlJob; |
||||
import lombok.extern.slf4j.Slf4j; |
||||
import org.springblade.core.log.exception.ServiceException; |
||||
import org.springblade.core.tool.api.R; |
||||
import org.springblade.core.tool.utils.DateUtil; |
||||
import org.springblade.core.tool.utils.Func; |
||||
import org.springblade.system.feign.ISysClient; |
||||
import org.springblade.system.user.entity.User; |
||||
import org.springblade.system.user.feign.IUserClient; |
||||
import org.springframework.beans.factory.annotation.Autowired; |
||||
import org.springframework.stereotype.Component; |
||||
|
||||
import java.text.DateFormat; |
||||
import java.text.ParseException; |
||||
import java.text.SimpleDateFormat; |
||||
import java.util.Date; |
||||
import java.util.List; |
||||
import java.util.stream.Collectors; |
||||
|
||||
import static com.hnac.hzims.operational.main.constant.MainConstants.DUTY_NOTICE; |
||||
import static com.hnac.hzims.operational.main.constant.MainConstants.PROJECT_MANAGER; |
||||
|
||||
|
||||
/** |
||||
* 排班到期定时任务通知 |
||||
* |
||||
* @author ty |
||||
*/ |
||||
@Slf4j |
||||
@Component |
||||
public class DutyNoticeScheduledTask { |
||||
|
||||
@Autowired |
||||
private DutyServiceImpl service; |
||||
@Autowired |
||||
private ISysClient sysClient; |
||||
@Autowired |
||||
private IUserClient userClient; |
||||
@Autowired |
||||
private IMessageClient messageClient; |
||||
|
||||
/** |
||||
* realId刷新 |
||||
*/ |
||||
@XxlJob(DUTY_NOTICE) |
||||
// @Scheduled(cron = "0 */1 * * * ? ")
|
||||
public ReturnT<String> dutyNotice(String param) throws ParseException { |
||||
if (Func.isBlank(param)) { |
||||
param = DateUtil.format(new Date(), "yyyy-MM-dd"); |
||||
} |
||||
DateFormat dateFormat = new SimpleDateFormat("yyyy-MM-dd"); |
||||
Date datyTime = dateFormat.parse(param); |
||||
String dateAddOne = DateUtil.format(DateUtil.plusDays(datyTime, 1), "yyyy-MM-dd"); |
||||
String dateAddTwo = DateUtil.format(DateUtil.plusDays(datyTime, 2), "yyyy-MM-dd"); |
||||
String dateAddThree = DateUtil.format(DateUtil.plusDays(datyTime, 3), "yyyy-MM-dd"); |
||||
List<Long> deptByDate = service.getDeptByMainDate(param); |
||||
List<Long> deptAndSendNotice1 = service.getDeptByMainDate(dateAddOne); |
||||
List<Long> deptAndSendNotice2 = service.getDeptByMainDate(dateAddTwo); |
||||
List<Long> deptAndSendNotice3 = service.getDeptByMainDate(dateAddThree); |
||||
//去除一天后有排版计划部门
|
||||
for (Long deptIdByDayOne : deptAndSendNotice1) { |
||||
if (deptByDate.contains(deptIdByDayOne)) { |
||||
deptByDate.remove(deptIdByDayOne); |
||||
} |
||||
} |
||||
sendMessage(deptByDate, 1); |
||||
//去除二天有排版计划的部门
|
||||
for (Long deptIdByDayTwo : deptAndSendNotice2) { |
||||
if (deptAndSendNotice3.contains(deptIdByDayTwo)) { |
||||
deptByDate.remove(deptIdByDayTwo); |
||||
} |
||||
} |
||||
sendMessage(deptByDate, 2); |
||||
//去除三天有排版计划的部门
|
||||
for (Long deptIdByDayTwo : deptAndSendNotice3) { |
||||
if (deptAndSendNotice3.contains(deptIdByDayTwo)) { |
||||
deptByDate.remove(deptIdByDayTwo); |
||||
} |
||||
} |
||||
sendMessage(deptByDate, 3); |
||||
return new ReturnT<>("SUCCESS"); |
||||
|
||||
} |
||||
|
||||
private void sendMessage(List<Long> deptIds, Integer date) { |
||||
for (Long deptId : deptIds) { |
||||
BusinessMessageDTO message = new BusinessMessageDTO(); |
||||
message.setBusinessClassify("business"); |
||||
message.setBusinessKey(MessageConstants.BusinessClassifyEnum.OPERATIONDEFECTMESSAGE.getKey()); |
||||
message.setSubject(MessageConstants.BusinessClassifyEnum.OPERATIONDEFECTMESSAGE.getDescription()); |
||||
message.setTaskId(System.currentTimeMillis()); |
||||
message.setTenantId("200000"); |
||||
String countent = |
||||
date + "天后,排班计划到期,请及时更新排班计划,以免影响正常工作"; |
||||
message.setContent(countent); |
||||
message.setDeptId(deptId); |
||||
R<String> deptName = sysClient.getDeptName(deptId); |
||||
if (deptName.isSuccess()) { |
||||
message.setDeptName(deptName.getData()); |
||||
} |
||||
//通知项目经理这个角色
|
||||
List<User> userListByRoleAlias = userClient.relationUserListByRoleAlias("200000", deptId, PROJECT_MANAGER).getData(); |
||||
List<String> userList = userListByRoleAlias.stream().map(s -> s.getId().toString()).collect(Collectors.toList()); |
||||
String userIds = String.join(",", userList); |
||||
if (StringUtils.isBlank(userIds)) { |
||||
log.error("推送的消息不能为空哦,{}", userIds); |
||||
throw new ServiceException("推送的消息不能为空"); |
||||
} |
||||
message.setCreateUser(Long.valueOf(userList.get(0))); |
||||
messageClient.sendAppAndWsMsgByUsers(message); |
||||
} |
||||
} |
||||
|
||||
|
||||
} |
Loading…
Reference in new issue