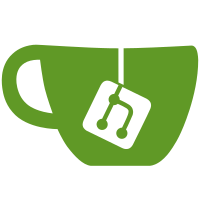
34 changed files with 566 additions and 66 deletions
@ -0,0 +1,30 @@ |
|||||||
|
package com.hnac.hzims.fdp.feign; |
||||||
|
|
||||||
|
import com.hnac.hzims.EquipmentConstants; |
||||||
|
import io.swagger.annotations.ApiParam; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.cloud.openfeign.FeignClient; |
||||||
|
import org.springframework.web.bind.annotation.GetMapping; |
||||||
|
import org.springframework.web.bind.annotation.RequestParam; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author hx |
||||||
|
* @version 1.0 |
||||||
|
* @date 2023/3/20 10:17 |
||||||
|
*/ |
||||||
|
@FeignClient(value = EquipmentConstants.APP_NAME,fallback = QuestionClientFallback.class) |
||||||
|
public interface IQuestionClient { |
||||||
|
|
||||||
|
String API_PREFIX = "/feign/fdp/question"; |
||||||
|
String SUBMIT_STATION_INFO = API_PREFIX + "/submitStationInfo"; |
||||||
|
|
||||||
|
/** |
||||||
|
* 提交站点信息 |
||||||
|
* @param stationCode 站点编码 |
||||||
|
* @param stationDesc 站点描述 |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@GetMapping(SUBMIT_STATION_INFO) |
||||||
|
R<Boolean> submitStationInfo(@RequestParam String stationCode, @RequestParam(required = false) String stationDesc); |
||||||
|
|
||||||
|
} |
@ -0,0 +1,20 @@ |
|||||||
|
package com.hnac.hzims.fdp.feign; |
||||||
|
|
||||||
|
import org.springblade.core.log.exception.ServiceException; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.stereotype.Component; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author hx |
||||||
|
* @version 1.0 |
||||||
|
* @date 2023/3/20 10:19 |
||||||
|
*/ |
||||||
|
@Component |
||||||
|
public class QuestionClientFallback implements IQuestionClient { |
||||||
|
|
||||||
|
|
||||||
|
@Override |
||||||
|
public R<Boolean> submitStationInfo(String stationCode, String stationDesc) { |
||||||
|
return R.fail("智能诊断提交站点失败!"); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,55 @@ |
|||||||
|
package com.hnac.hzims.fdp.controller; |
||||||
|
|
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.monitor.server.IModelService; |
||||||
|
import com.hnac.hzinfo.sdk.v5.model.vo.ModelAttrVO; |
||||||
|
import com.hnac.hzinfo.sdk.v5.model.vo.ModelGroupVO; |
||||||
|
import com.hnac.hzinfo.sdk.v5.model.vo.ModelVO; |
||||||
|
import io.swagger.annotations.Api; |
||||||
|
import io.swagger.annotations.ApiOperation; |
||||||
|
import io.swagger.annotations.ApiParam; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.web.bind.annotation.GetMapping; |
||||||
|
import org.springframework.web.bind.annotation.PathVariable; |
||||||
|
import org.springframework.web.bind.annotation.RequestMapping; |
||||||
|
import org.springframework.web.bind.annotation.RestController; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author hx |
||||||
|
* @version 1.0 |
||||||
|
* @date 2023/3/18 16:32 |
||||||
|
*/ |
||||||
|
@RestController |
||||||
|
@RequestMapping("/model") |
||||||
|
@Api(value = "数据平台模型定义管理",tags = "数据平台模型定义管理") |
||||||
|
@AllArgsConstructor |
||||||
|
public class ModelController extends BladeController { |
||||||
|
|
||||||
|
private final IModelService modelService; |
||||||
|
|
||||||
|
@GetMapping("/getAllModelGroup") |
||||||
|
@ApiOperation("获取所有模型分组") |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
public R<List<ModelGroupVO>> getAllModelGroup() { |
||||||
|
return R.data(modelService.getAllModelGroup()); |
||||||
|
} |
||||||
|
|
||||||
|
@GetMapping("/getAllModelGroup/{modelGroupId}") |
||||||
|
@ApiOperation("根据模型分组ID获取模型列表") |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
public R<List<ModelVO>> mGroupByModel(@PathVariable @ApiParam("模型分组ID") Long modelGroupId) { |
||||||
|
return R.data(modelService.mGroupByModel(modelGroupId)); |
||||||
|
} |
||||||
|
|
||||||
|
@GetMapping("/getAttr/{modelSignage}") |
||||||
|
@ApiOperation("根据模型标识获取物模型属性") |
||||||
|
@ApiOperationSupport(order = 3) |
||||||
|
public R<List<ModelAttrVO>> getAttr(@PathVariable @ApiParam("模型标识") String modelSignage) { |
||||||
|
return R.data(modelService.getAttr(modelSignage)); |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,27 @@ |
|||||||
|
package com.hnac.hzims.fdp.feign; |
||||||
|
|
||||||
|
import com.hnac.hzims.fdp.service.IFdpQuestionService; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.web.bind.annotation.GetMapping; |
||||||
|
import org.springframework.web.bind.annotation.RequestParam; |
||||||
|
import org.springframework.web.bind.annotation.RestController; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author hx |
||||||
|
* @version 1.0 |
||||||
|
* @date 2023/3/20 10:28 |
||||||
|
*/ |
||||||
|
@RestController |
||||||
|
@AllArgsConstructor |
||||||
|
public class QuestionClient implements IQuestionClient { |
||||||
|
|
||||||
|
private final IFdpQuestionService fdpQuestionService; |
||||||
|
|
||||||
|
@GetMapping(SUBMIT_STATION_INFO) |
||||||
|
@Override |
||||||
|
public R<Boolean> submitStationInfo(@RequestParam String stationCode,@RequestParam String stationDesc) { |
||||||
|
return R.status(fdpQuestionService.submitStationInfo(stationCode,stationDesc)); |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,44 @@ |
|||||||
|
package com.hnac.hzims.monitor.controller; |
||||||
|
|
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.fdp.request.SubmitAnswerReq; |
||||||
|
import com.hnac.hzims.monitor.server.IEquipmentInstanceService; |
||||||
|
import com.hnac.hzinfo.sdk.v5.device.vo.DeviceInstanceAttrVO; |
||||||
|
import io.swagger.annotations.Api; |
||||||
|
import io.swagger.annotations.ApiOperation; |
||||||
|
import io.swagger.annotations.ApiParam; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import org.springblade.core.boot.ctrl.BladeController; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
|
||||||
|
import javax.validation.Valid; |
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author hx |
||||||
|
* @version 1.0 |
||||||
|
* @date 2023/3/17 13:54 |
||||||
|
*/ |
||||||
|
@RestController |
||||||
|
@RequestMapping("/equipment/instance") |
||||||
|
@AllArgsConstructor |
||||||
|
@Api(value = "设备实例化管理",tags = "设备实例化管理") |
||||||
|
public class EquipmentInstanceController extends BladeController { |
||||||
|
|
||||||
|
private final IEquipmentInstanceService equipmentInstanceService; |
||||||
|
|
||||||
|
@PostMapping("/instanceAndSubmit") |
||||||
|
@ApiOperation("实例化设备以及提交答案") |
||||||
|
@ApiOperationSupport(order = 1) |
||||||
|
public R<Boolean> instanceAndSubmit(@RequestBody @Valid SubmitAnswerReq submitAnswerReq) { |
||||||
|
return R.status(equipmentInstanceService.instance(submitAnswerReq)); |
||||||
|
} |
||||||
|
|
||||||
|
@GetMapping("/getOnlineAttr/{emCode}") |
||||||
|
@ApiOperation("根据设备code获取设备实例物模型") |
||||||
|
@ApiOperationSupport(order = 2) |
||||||
|
public R<List<DeviceInstanceAttrVO>> getOnlineAttr(@PathVariable @ApiParam("设备编码") String emCode) { |
||||||
|
return R.data(equipmentInstanceService.getOnlineAttr(emCode)); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,29 @@ |
|||||||
|
package com.hnac.hzims.monitor.server; |
||||||
|
|
||||||
|
import com.hnac.hzims.fdp.request.SubmitAnswerReq; |
||||||
|
import com.hnac.hzinfo.sdk.v5.device.vo.DeviceInstanceAttrVO; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author hx |
||||||
|
* @version 1.0 |
||||||
|
* @date 2023/3/17 14:08 |
||||||
|
*/ |
||||||
|
public interface IEquipmentInstanceService { |
||||||
|
|
||||||
|
/** |
||||||
|
* 设备实例化 + 提交FDP答案 |
||||||
|
* @param submitAnswerReq |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
boolean instance(SubmitAnswerReq submitAnswerReq); |
||||||
|
|
||||||
|
/** |
||||||
|
* 根据设备code获取设备实例物模型 |
||||||
|
* @param emCode 设备编码 |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
List<DeviceInstanceAttrVO> getOnlineAttr(String emCode); |
||||||
|
|
||||||
|
} |
@ -0,0 +1,38 @@ |
|||||||
|
package com.hnac.hzims.monitor.server; |
||||||
|
|
||||||
|
import com.hnac.hzinfo.sdk.v5.model.vo.ModelAttrVO; |
||||||
|
import com.hnac.hzinfo.sdk.v5.model.vo.ModelGroupVO; |
||||||
|
import com.hnac.hzinfo.sdk.v5.model.vo.ModelVO; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author hx |
||||||
|
* @version 1.0 |
||||||
|
* @date 2023/3/18 16:13 |
||||||
|
*/ |
||||||
|
public interface IModelService { |
||||||
|
|
||||||
|
/** |
||||||
|
* 获取所有模型分组 |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
List<ModelGroupVO> getAllModelGroup(); |
||||||
|
|
||||||
|
/*** |
||||||
|
* 根据模型分组ID获取模型列表 |
||||||
|
* @param modelGroupId 模型分组ID |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
List<ModelVO> mGroupByModel(Long modelGroupId); |
||||||
|
|
||||||
|
/** |
||||||
|
* 根据模型标识获取物模型属性 |
||||||
|
* @param modelSignage 模型标识 |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
List<ModelAttrVO> getAttr(String modelSignage); |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,88 @@ |
|||||||
|
package com.hnac.hzims.monitor.server.impl; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.update.LambdaUpdateWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.toolkit.Wrappers; |
||||||
|
import com.hnac.hzims.equipment.entity.EmInfoEntity; |
||||||
|
import com.hnac.hzims.equipment.service.IEmInfoService; |
||||||
|
import com.hnac.hzims.fdp.request.AnswerFormatReq; |
||||||
|
import com.hnac.hzims.fdp.request.SubmitAnswerReq; |
||||||
|
import com.hnac.hzims.fdp.service.IFdpQuestionService; |
||||||
|
import com.hnac.hzims.monitor.server.IEquipmentInstanceService; |
||||||
|
import com.hnac.hzinfo.datasearch.device.IDeviceClient; |
||||||
|
import com.hnac.hzinfo.sdk.v5.device.client.DeviceClient; |
||||||
|
import com.hnac.hzinfo.sdk.v5.device.vo.DeviceInstanceAttrVO; |
||||||
|
import com.hnac.hzinfo.sdk.v5.device.vo.VirtualDeviceDTO; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import lombok.RequiredArgsConstructor; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springblade.core.log.exception.ServiceException; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.Func; |
||||||
|
import org.springframework.beans.factory.annotation.Value; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
import org.springframework.util.Assert; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author hx |
||||||
|
* @version 1.0 |
||||||
|
* @date 2023/3/17 14:08 |
||||||
|
*/ |
||||||
|
@Service |
||||||
|
@RequiredArgsConstructor |
||||||
|
@Slf4j |
||||||
|
public class EquipmentInstanceServiceImpl implements IEquipmentInstanceService { |
||||||
|
private final DeviceClient deviceClient; |
||||||
|
private final IFdpQuestionService fdpQuestionService; |
||||||
|
private final IEmInfoService emInfoService; |
||||||
|
|
||||||
|
@Value("${hzinfo.model.commonSignage}") |
||||||
|
private String commonSignage; |
||||||
|
@Value("${hzinfo.model.deviceSignage}") |
||||||
|
private String deviceSignage; |
||||||
|
|
||||||
|
@Override |
||||||
|
public boolean instance(SubmitAnswerReq submitAnswerReq) { |
||||||
|
VirtualDeviceDTO virtualDeviceDTO = new VirtualDeviceDTO(); |
||||||
|
virtualDeviceDTO.setProjectId(submitAnswerReq.getStationId()); |
||||||
|
virtualDeviceDTO.setDeviceCode(submitAnswerReq.getEmCode()); |
||||||
|
virtualDeviceDTO.setDeviceName(submitAnswerReq.getEmName()); |
||||||
|
// 模型标识根据题目答案获取
|
||||||
|
virtualDeviceDTO.setModelSignage(this.getModelSignageByAnswer(submitAnswerReq)); |
||||||
|
R saveResult = deviceClient.saveVirtualDevice(virtualDeviceDTO); |
||||||
|
Assert.isTrue(saveResult.isSuccess() && Func.isNotEmpty(saveResult.getData()),() -> { |
||||||
|
throw new ServiceException(saveResult.getMsg()); |
||||||
|
}); |
||||||
|
Long instanceId = Long.valueOf(String.valueOf(saveResult.getData())); |
||||||
|
LambdaUpdateWrapper<EmInfoEntity> luw = Wrappers.<EmInfoEntity>lambdaUpdate() |
||||||
|
.set(EmInfoEntity::getDeviceInstanceId, instanceId) |
||||||
|
.eq(EmInfoEntity::getNumber, submitAnswerReq.getEmCode()); |
||||||
|
Assert.isTrue(emInfoService.update(luw),() -> { |
||||||
|
throw new ServiceException("保存设备实例ID失败"); |
||||||
|
}); |
||||||
|
submitAnswerReq.setInstanceId(instanceId.toString()); |
||||||
|
return fdpQuestionService.submitDeviceAnswer(submitAnswerReq); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<DeviceInstanceAttrVO> getOnlineAttr(String emCode) { |
||||||
|
R<List<DeviceInstanceAttrVO>> attrListResult = deviceClient.getOnlineAttr(emCode); |
||||||
|
Assert.isTrue(attrListResult.isSuccess(),() -> { |
||||||
|
throw new ServiceException(attrListResult.getMsg()); |
||||||
|
}); |
||||||
|
return attrListResult.getData(); |
||||||
|
} |
||||||
|
|
||||||
|
private String getModelSignageByAnswer(SubmitAnswerReq submitAnswerReq) { |
||||||
|
if(IFdpQuestionService.COMMON_TYPE.equals(submitAnswerReq.getDeviceType())) { |
||||||
|
return commonSignage; |
||||||
|
} |
||||||
|
else if (IFdpQuestionService.DEVICE_TYPE.equals(submitAnswerReq.getDeviceType())) { |
||||||
|
return deviceSignage; |
||||||
|
} |
||||||
|
else { |
||||||
|
throw new ServiceException("设备类型传参错误"); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,59 @@ |
|||||||
|
package com.hnac.hzims.monitor.server.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.monitor.server.IModelService; |
||||||
|
import com.hnac.hzinfo.sdk.v5.model.ModelClient; |
||||||
|
import com.hnac.hzinfo.sdk.v5.model.ModelGroupClient; |
||||||
|
import com.hnac.hzinfo.sdk.v5.model.vo.ModelAttrVO; |
||||||
|
import com.hnac.hzinfo.sdk.v5.model.vo.ModelGroupVO; |
||||||
|
import com.hnac.hzinfo.sdk.v5.model.vo.ModelVO; |
||||||
|
import lombok.AllArgsConstructor; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springblade.core.log.exception.ServiceException; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
import org.springframework.util.Assert; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author hx |
||||||
|
* @version 1.0 |
||||||
|
* @date 2023/3/18 16:13 |
||||||
|
*/ |
||||||
|
@Service |
||||||
|
@AllArgsConstructor |
||||||
|
@Slf4j |
||||||
|
public class ModelServiceImpl implements IModelService { |
||||||
|
|
||||||
|
private final ModelClient modelClient; |
||||||
|
|
||||||
|
private final ModelGroupClient modelGroupClient; |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<ModelGroupVO> getAllModelGroup() { |
||||||
|
R<List<ModelGroupVO>> modelGroupList = modelGroupClient.getAllModelGroup(); |
||||||
|
Assert.isTrue(modelGroupList.isSuccess(),() -> { |
||||||
|
throw new ServiceException(modelGroupList.getMsg()); |
||||||
|
}); |
||||||
|
return modelGroupList.getData(); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<ModelAttrVO> getAttr(String modelSignage) { |
||||||
|
R<List<ModelAttrVO>> attrResult = modelClient.getAttr(modelSignage); |
||||||
|
Assert.isTrue(attrResult.isSuccess(),() -> { |
||||||
|
throw new ServiceException(attrResult.getMsg()); |
||||||
|
}); |
||||||
|
return attrResult.getData(); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public List<ModelVO> mGroupByModel(Long modelGroupId) { |
||||||
|
R<List<ModelVO>> listR = modelClient.mGroupByModel(modelGroupId); |
||||||
|
Assert.isTrue(listR.isSuccess(),() -> { |
||||||
|
throw new ServiceException(listR.getMsg()); |
||||||
|
}); |
||||||
|
return listR.getData(); |
||||||
|
} |
||||||
|
|
||||||
|
} |
Loading…
Reference in new issue