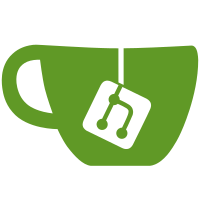
8 changed files with 581 additions and 90 deletions
@ -0,0 +1,153 @@ |
|||||||
|
package com.hnac.hzims.ticket.twoTicket.handler; |
||||||
|
|
||||||
|
import com.alibaba.excel.write.handler.SheetWriteHandler; |
||||||
|
import com.alibaba.excel.write.metadata.WriteSheet; |
||||||
|
import com.alibaba.excel.write.metadata.holder.WriteSheetHolder; |
||||||
|
import com.alibaba.excel.write.metadata.holder.WriteWorkbookHolder; |
||||||
|
import org.apache.poi.hssf.usermodel.HSSFCellStyle; |
||||||
|
import org.apache.poi.hssf.usermodel.HSSFFont; |
||||||
|
import org.apache.poi.ss.usermodel.*; |
||||||
|
import org.apache.poi.ss.util.CellRangeAddress; |
||||||
|
import org.apache.poi.xssf.usermodel.XSSFCellStyle; |
||||||
|
import org.aspectj.bridge.MessageWriter; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/7/6 11:08 |
||||||
|
*/ |
||||||
|
public class TicketCountSheetWriteHandler implements SheetWriteHandler { |
||||||
|
|
||||||
|
/** |
||||||
|
* Called before create the sheet |
||||||
|
* |
||||||
|
* @param writeWorkbookHolder |
||||||
|
* @param writeSheetHolder |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public void beforeSheetCreate(WriteWorkbookHolder writeWorkbookHolder, WriteSheetHolder writeSheetHolder) { |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* Called after the sheet is created |
||||||
|
* |
||||||
|
* @param writeWorkbookHolder |
||||||
|
* @param writeSheetHolder |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public void afterSheetCreate(WriteWorkbookHolder writeWorkbookHolder, WriteSheetHolder writeSheetHolder) { |
||||||
|
Workbook workbook = writeWorkbookHolder.getWorkbook(); |
||||||
|
CellStyle headerStyle = createHeadCellStyle(workbook); |
||||||
|
Sheet sheet = workbook.getSheetAt(0); |
||||||
|
sheet.addMergedRegion(new CellRangeAddress(0, 1, 0, 0)); |
||||||
|
sheet.addMergedRegion(new CellRangeAddress(0, 0, 1, 4)); |
||||||
|
sheet.addMergedRegion(new CellRangeAddress(0, 0, 5, 8)); |
||||||
|
sheet.addMergedRegion(new CellRangeAddress(0, 0, 9, 12)); |
||||||
|
sheet.addMergedRegion(new CellRangeAddress(0, 0, 13, 15)); |
||||||
|
sheet.setHorizontallyCenter(true); |
||||||
|
sheet.setVerticallyCenter(true); |
||||||
|
sheet.setDefaultRowHeight((short) 20); |
||||||
|
//设置填表日期,填报人,联系方式
|
||||||
|
Row row1 = sheet.createRow(0); |
||||||
|
row1.setHeightInPoints((short) 35); |
||||||
|
Cell row1Cell = row1.createCell(0); |
||||||
|
row1Cell.setCellValue("站点"); |
||||||
|
row1Cell.setCellStyle(headerStyle); |
||||||
|
|
||||||
|
// row1.setHeight((short) 500);
|
||||||
|
Cell cell = row1.createCell(1); |
||||||
|
cell.setCellValue("第一种工作票"); |
||||||
|
cell.setCellStyle(headerStyle); |
||||||
|
|
||||||
|
|
||||||
|
Cell cell1 = row1.createCell(5); |
||||||
|
cell1.setCellValue("第二种工作票"); |
||||||
|
cell1.setCellStyle(headerStyle); |
||||||
|
|
||||||
|
|
||||||
|
Cell cell2 = row1.createCell(9); |
||||||
|
cell2.setCellValue("机械工作票"); |
||||||
|
cell2.setCellStyle(headerStyle); |
||||||
|
|
||||||
|
Cell cell3 = row1.createCell(13); |
||||||
|
cell3.setCellValue("倒闸操作票"); |
||||||
|
cell3.setCellStyle(headerStyle); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 创建表头样式 |
||||||
|
* @param wb |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
private static CellStyle createHeadCellStyle(Workbook wb) { |
||||||
|
CellStyle cellStyle = wb.createCellStyle(); |
||||||
|
cellStyle.setWrapText(true);// 设置自动换行
|
||||||
|
cellStyle.setFillForegroundColor(IndexedColors.GREY_25_PERCENT.getIndex());//背景颜色
|
||||||
|
cellStyle.setAlignment(HorizontalAlignment.CENTER); //水平居中
|
||||||
|
cellStyle.setVerticalAlignment(VerticalAlignment.CENTER); //垂直对齐
|
||||||
|
cellStyle.setFillPattern(FillPatternType.SOLID_FOREGROUND); |
||||||
|
cellStyle.setBottomBorderColor(IndexedColors.BLACK.index); |
||||||
|
cellStyle.setBorderBottom(BorderStyle.THIN); //下边框
|
||||||
|
cellStyle.setBorderLeft(BorderStyle.THIN); //左边框
|
||||||
|
cellStyle.setBorderRight(BorderStyle.THIN); //右边框
|
||||||
|
cellStyle.setBorderTop(BorderStyle.THIN); //上边框
|
||||||
|
|
||||||
|
Font headerFont = wb.createFont(); // 创建字体样式
|
||||||
|
headerFont.setBold(true); //字体加粗
|
||||||
|
headerFont.setFontName("黑体"); // 设置字体类型
|
||||||
|
headerFont.setFontHeightInPoints((short) 12); // 设置字体大小
|
||||||
|
cellStyle.setFont(headerFont); // 为标题样式设置字体样式
|
||||||
|
|
||||||
|
return cellStyle; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 创建内容样式 |
||||||
|
* @param wb |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
private static CellStyle createContentCellStyle(Workbook wb) { |
||||||
|
CellStyle cellStyle = wb.createCellStyle(); |
||||||
|
cellStyle.setVerticalAlignment(VerticalAlignment.CENTER);// 垂直居中
|
||||||
|
cellStyle.setAlignment(HorizontalAlignment.CENTER);// 水平居中
|
||||||
|
cellStyle.setWrapText(true);// 设置自动换行
|
||||||
|
cellStyle.setBorderBottom(BorderStyle.THIN); //下边框
|
||||||
|
cellStyle.setBorderLeft(BorderStyle.THIN); //左边框
|
||||||
|
cellStyle.setBorderRight(BorderStyle.THIN); //右边框
|
||||||
|
cellStyle.setBorderTop(BorderStyle.THIN); //上边框
|
||||||
|
|
||||||
|
// 生成12号字体
|
||||||
|
Font font = wb.createFont(); |
||||||
|
font.setColor((short)8); |
||||||
|
font.setFontHeightInPoints((short) 12); |
||||||
|
cellStyle.setFont(font); |
||||||
|
return cellStyle; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 创建标题样式 |
||||||
|
* @param wb |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
private static CellStyle createTitleCellStyle(Workbook wb) { |
||||||
|
CellStyle cellStyle = wb.createCellStyle(); |
||||||
|
cellStyle.setAlignment(HorizontalAlignment.CENTER);//水平居中
|
||||||
|
cellStyle.setVerticalAlignment(VerticalAlignment.CENTER);//垂直对齐
|
||||||
|
cellStyle.setFillPattern(FillPatternType.SOLID_FOREGROUND); |
||||||
|
cellStyle.setFillForegroundColor(IndexedColors.GREY_40_PERCENT.getIndex());//背景颜色
|
||||||
|
|
||||||
|
Font headerFont1 = wb.createFont(); // 创建字体样式
|
||||||
|
headerFont1.setBold(true); //字体加粗
|
||||||
|
headerFont1.setFontName("黑体"); // 设置字体类型
|
||||||
|
headerFont1.setFontHeightInPoints((short) 15); // 设置字体大小
|
||||||
|
cellStyle.setFont(headerFont1); // 为标题样式设置字体样式
|
||||||
|
|
||||||
|
return cellStyle; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,157 @@ |
|||||||
|
package com.hnac.hzims.ticket.twoTicket.vo.ticket; |
||||||
|
|
||||||
|
import com.alibaba.excel.annotation.ExcelIgnore; |
||||||
|
import com.alibaba.excel.annotation.ExcelProperty; |
||||||
|
import com.alibaba.excel.annotation.write.style.ColumnWidth; |
||||||
|
import com.alibaba.excel.annotation.write.style.ContentRowHeight; |
||||||
|
import com.alibaba.excel.annotation.write.style.HeadRowHeight; |
||||||
|
import lombok.Data; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/7/6 8:02 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@ContentRowHeight(30) |
||||||
|
@HeadRowHeight(30) |
||||||
|
public class TicketCountResponseVo { |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 站点名称 |
||||||
|
*/ |
||||||
|
@ColumnWidth(20) |
||||||
|
@ExcelProperty(value = "站点",index = 0) |
||||||
|
private String deptName; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 第一种工作票开票数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "开票数量",index = 1) |
||||||
|
private Integer oneTicketNumber = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 第一种工作票完成数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "完成数量",index = 2) |
||||||
|
private Integer oneTicketCompleteNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 第一种工作票延期数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "延期数量",index = 3) |
||||||
|
private Integer oneTicketDelayNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 第一种工作票合格数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "合格数量",index = 4) |
||||||
|
private Integer oneTicketProportionNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 第二种工作票开票数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "开票数量",index = 5) |
||||||
|
private Integer twoTicketNumber = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 第二种工作票完成数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "完成数量",index = 6) |
||||||
|
private Integer twoTicketCompleteNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 第二种工作票延期数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "延期数量",index = 7) |
||||||
|
private Integer twoTicketDelayNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 第二种工作票合格数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "合格数量",index = 8) |
||||||
|
private Integer twoTicketProportionNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 水力机械工作票开票数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "开票数量",index = 9) |
||||||
|
private Integer threeTicketNumber = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 水力机械工作票完成数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "完成数量",index = 10) |
||||||
|
private Integer threeTicketCompleteNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 水力机械工作票延期数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "延期数量",index = 11) |
||||||
|
private Integer threeTicketDelayNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 水力机械工作票合格数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "合格数量",index = 12) |
||||||
|
private Integer threeTicketProportionNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 操作票开票数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "开票数量",index = 13) |
||||||
|
private Integer fourTicketNumber = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 操作票开票完成数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "完成数量",index = 14) |
||||||
|
private Integer fourTicketCompleteNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 操作票延期数量 |
||||||
|
*/ |
||||||
|
@ExcelIgnore |
||||||
|
private Integer fourTicketDelayNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 操作票作票合格数量 |
||||||
|
*/ |
||||||
|
@ColumnWidth(14) |
||||||
|
@ExcelProperty(value = "合格数量",index = 15) |
||||||
|
private Integer fourTicketProportionNum = 0; |
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,16 @@ |
|||||||
|
package com.hnac.hzims.ticket.twoTicket.vo.ticket; |
||||||
|
|
||||||
|
import lombok.Data; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/7/6 8:04 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
public class TicketCountVo { |
||||||
|
|
||||||
|
|
||||||
|
private String keyword; |
||||||
|
} |
Loading…
Reference in new issue