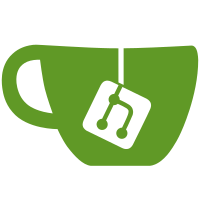
9 changed files with 358 additions and 10 deletions
@ -0,0 +1,70 @@ |
|||||||
|
package com.hnac.hzims.operational.duty.entity; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.SqlCondition; |
||||||
|
import com.baomidou.mybatisplus.annotation.TableName; |
||||||
|
import io.swagger.annotations.ApiModel; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
import org.springblade.core.mp.support.QueryField; |
||||||
|
import org.springblade.core.tenant.mp.TenantEntity; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 实体类 |
||||||
|
* |
||||||
|
* @author ty |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@TableName("hz_ims_duty_analyse_example") |
||||||
|
@EqualsAndHashCode(callSuper = true) |
||||||
|
@ApiModel(value = "AnalyseExample对象", description = "分析实例配置类") |
||||||
|
public class AnalyseExample extends TenantEntity { |
||||||
|
|
||||||
|
private static final long serialVersionUID = 4259568798118459986L; |
||||||
|
/** |
||||||
|
* 配置ID |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "配置ID") |
||||||
|
private Long id; |
||||||
|
/** |
||||||
|
* 站点ID |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "站点ID") |
||||||
|
private Long classId; |
||||||
|
/** |
||||||
|
* 站点名称 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "站点名称") |
||||||
|
private String className; |
||||||
|
/** |
||||||
|
* 设备ID |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "设备ID") |
||||||
|
private Long equipmentId; |
||||||
|
|
||||||
|
/** |
||||||
|
* 设备名称 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "设备名称") |
||||||
|
private String equipmentName; |
||||||
|
/** |
||||||
|
* 分析实例ID |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "分析实例ID") |
||||||
|
private Long analyseId; |
||||||
|
/** |
||||||
|
* 分析实例名称 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "分析实例名称") |
||||||
|
private String analyseName; |
||||||
|
|
||||||
|
/** |
||||||
|
* 属性ID |
||||||
|
*/ |
||||||
|
@QueryField(condition = SqlCondition.LIKE) |
||||||
|
@ApiModelProperty(value = "属性ID") |
||||||
|
private String propertyIds; |
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,16 @@ |
|||||||
|
package com.hnac.hzims.operational.duty.mapper; |
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.operational.duty.entity.AnalyseExample; |
||||||
|
import org.springblade.core.datascope.mapper.UserDataScopeBaseMapper; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* Mapper 接口 |
||||||
|
* |
||||||
|
* @author Chill |
||||||
|
*/ |
||||||
|
public interface ImsAnalyseExampleMapper extends UserDataScopeBaseMapper<AnalyseExample> { |
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,16 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8" ?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" > |
||||||
|
<mapper namespace="com.hnac.hzims.operational.duty.mapper.ImsAnalyseExampleMapper"> |
||||||
|
<!-- 通用查询映射结果 --> |
||||||
|
<resultMap id="ims_duty_recResultMap" type="com.hnac.hzims.operational.duty.entity.AnalyseExample"> |
||||||
|
<result column="ID" property="id"/> |
||||||
|
<result column="CLASS_ID" property="classId"/> |
||||||
|
<result column="CLASS_NAME" property="className"/> |
||||||
|
<result column="EQUIPMENT_ID" property="equipmentId"/> |
||||||
|
<result column="EQUIPMENT_NAME" property="equipmentName"/> |
||||||
|
<result column="ANALYSE_ID" property="analyseId"/> |
||||||
|
<result column="ANALYSE_NAME" property="analyseName"/> |
||||||
|
<result column="PROPERTY_IDS" property="propertyIds"/> |
||||||
|
</resultMap> |
||||||
|
|
||||||
|
</mapper> |
@ -0,0 +1,26 @@ |
|||||||
|
package com.hnac.hzims.operational.duty.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.operational.duty.entity.AnalyseExample; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.domain.AnalyzeDataCondition; |
||||||
|
import org.springblade.core.mp.base.BaseService; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 服务类 |
||||||
|
* |
||||||
|
* @author Chill |
||||||
|
*/ |
||||||
|
public interface IImsAnalyseExampleService extends BaseService<AnalyseExample> { |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
R queryAnalyseExampleData(AnalyzeDataCondition analyzeDataCondition); |
||||||
|
|
||||||
|
R queryAnalyseExample(AnalyseExample analyseExample); |
||||||
|
|
||||||
|
R getAnalyseId(String projectId); |
||||||
|
|
||||||
|
R updateAnalyseExample(AnalyseExample analyseExample); |
||||||
|
} |
@ -0,0 +1,126 @@ |
|||||||
|
package com.hnac.hzims.operational.duty.service.impl; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.QueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.toolkit.IdWorker; |
||||||
|
import com.hnac.hzims.operational.duty.entity.AnalyseExample; |
||||||
|
import com.hnac.hzims.operational.duty.mapper.ImsAnalyseExampleMapper; |
||||||
|
import com.hnac.hzims.operational.duty.mapper.ImsDutyMainMapper; |
||||||
|
import com.hnac.hzims.operational.duty.service.IImsAnalyseExampleService; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.IAnalyseDataSearchClient; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.domain.AnalyzeDataCondition; |
||||||
|
import com.hnac.hzinfo.datasearch.analyse.domain.AnalyzeDataList; |
||||||
|
import com.hnac.hzinfo.sdk.core.response.HzPage; |
||||||
|
import com.hnac.hzinfo.sdk.core.response.Result; |
||||||
|
import com.hnac.hzinfo.sdk.v5.analyse.AnalyseDataHandlerClient; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import net.logstash.logback.encoder.org.apache.commons.lang3.ObjectUtils; |
||||||
|
import org.apache.commons.collections4.CollectionUtils; |
||||||
|
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.beans.BeanUtils; |
||||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import javax.annotation.Resource; |
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 服务实现类 |
||||||
|
* |
||||||
|
* @author ty |
||||||
|
*/ |
||||||
|
@Slf4j |
||||||
|
@Service |
||||||
|
public class ImsAnalyseExampleServiceImpl extends BaseServiceImpl<ImsAnalyseExampleMapper, AnalyseExample> implements IImsAnalyseExampleService { |
||||||
|
@Resource |
||||||
|
private ImsDutyMainMapper imsDutyMainMapper; |
||||||
|
@Autowired |
||||||
|
private IAnalyseDataSearchClient searchClient; |
||||||
|
@Autowired |
||||||
|
private AnalyseDataHandlerClient handlerClient; |
||||||
|
|
||||||
|
/** |
||||||
|
* 根据时间段+分析实例ID查询值班日志 |
||||||
|
* @param analyzeDataCondition |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public R queryAnalyseExampleData(AnalyzeDataCondition analyzeDataCondition) { |
||||||
|
//设备ID:分析实例编码
|
||||||
|
R<HzPage<AnalyzeDataList>> analyzeDataByAnalyzeCode = searchClient.getAnalyzeDataByAnalyzeCode(analyzeDataCondition); |
||||||
|
|
||||||
|
return R.data(analyzeDataByAnalyzeCode); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 查询值班日志模板 |
||||||
|
* @param analyseExample |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public R queryAnalyseExample(AnalyseExample analyseExample) { |
||||||
|
QueryWrapper<AnalyseExample> queryWrapper = getQueryWrapper(analyseExample); |
||||||
|
List<AnalyseExample> analyseExamples = baseMapper.selectList(queryWrapper); |
||||||
|
if (CollectionUtils.isNotEmpty(analyseExamples)){ |
||||||
|
return R.data(analyseExamples); |
||||||
|
}else { |
||||||
|
return R.success("暂无数据"); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public R getAnalyseId(String projectId) { |
||||||
|
Result result = handlerClient.analyseInstanceByProjectId(projectId); |
||||||
|
String data = result.getData().toString(); |
||||||
|
String s="11111111111111"; |
||||||
|
Result result1 = handlerClient.analyseByAnalyseAttr(s); |
||||||
|
String data2 = result1.getData().toString(); |
||||||
|
System.out.println(data2); |
||||||
|
// if ()
|
||||||
|
return R.data(data); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 生成值班日志模板 |
||||||
|
* @param analyseExample |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public R updateAnalyseExample(AnalyseExample analyseExample) { |
||||||
|
AnalyseExample entity = baseMapper.selectOne(new LambdaQueryWrapper<AnalyseExample>() {{ |
||||||
|
eq(AnalyseExample::getClassId, analyseExample.getClassId()); |
||||||
|
eq(AnalyseExample::getEquipmentId,analyseExample.getEquipmentId()); |
||||||
|
last(" limit 1"); |
||||||
|
}}); |
||||||
|
if (ObjectUtils.isNotEmpty(entity)){ |
||||||
|
BeanUtils.copyProperties(analyseExample,entity); |
||||||
|
this.saveOrUpdate(analyseExample); |
||||||
|
}else { |
||||||
|
analyseExample.setId(IdWorker.getId(analyseExample)); |
||||||
|
this.saveOrUpdate(analyseExample); |
||||||
|
} |
||||||
|
return R.success("保存成功"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
private QueryWrapper<AnalyseExample> getQueryWrapper(AnalyseExample analyseExample) { |
||||||
|
QueryWrapper<AnalyseExample> queryWrapper = new QueryWrapper(); |
||||||
|
if (ObjectUtils.isNotEmpty(analyseExample.getId())) { |
||||||
|
queryWrapper.lambda().eq(AnalyseExample::getId, analyseExample.getId()); |
||||||
|
} |
||||||
|
if (ObjectUtils.isNotEmpty(analyseExample.getClassName())) { |
||||||
|
queryWrapper.lambda().like(AnalyseExample::getClassName, analyseExample.getClassName()); |
||||||
|
} |
||||||
|
if (ObjectUtils.isNotEmpty(analyseExample.getEquipmentId())) { |
||||||
|
queryWrapper.lambda().eq(AnalyseExample::getEquipmentId, analyseExample.getEquipmentId()); |
||||||
|
} |
||||||
|
if (ObjectUtils.isNotEmpty(analyseExample.getAnalyseId())) { |
||||||
|
queryWrapper.lambda().eq(AnalyseExample::getAnalyseId, analyseExample.getAnalyseId()); |
||||||
|
} |
||||||
|
return queryWrapper; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
} |
Loading…
Reference in new issue