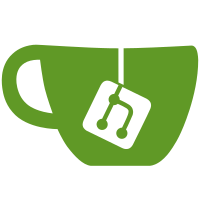
14 changed files with 863 additions and 71 deletions
@ -0,0 +1,69 @@ |
|||||||
|
package com.hnac.hzims.ticket.processflow.strategy.serviceimpl; |
||||||
|
|
||||||
|
import com.hnac.hzims.ticket.processflow.service.ProcessDictService; |
||||||
|
import com.hnac.hzims.ticket.processflow.strategy.abstracts.ProcessAbstractService; |
||||||
|
import com.hnac.hzims.ticket.processflow.strategy.entity.WorkflowQueue; |
||||||
|
import com.hnac.hzims.ticket.response.ProcessWorkFlowResponse; |
||||||
|
import com.hnac.hzims.ticket.standardTicket.service.OperationTicketService; |
||||||
|
import com.hnac.hzims.ticket.twoTicket.service.TicketProcessService; |
||||||
|
import lombok.RequiredArgsConstructor; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import static com.hnac.hzims.ticket.processflow.constant.TicketProcessConstant.OPERATION_TICKET_KEY; |
||||||
|
|
||||||
|
/** |
||||||
|
* 开工作票流程实现类 |
||||||
|
* |
||||||
|
* @Author dfy |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/3/28 8:47 |
||||||
|
*/ |
||||||
|
@Slf4j |
||||||
|
@Service |
||||||
|
@RequiredArgsConstructor |
||||||
|
public class OperationTicketProcessServiceImpl extends ProcessAbstractService { |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
private final OperationTicketService operationTicketService; |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
private final ProcessDictService processDictService; |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 设置执行那种实现类 |
||||||
|
* |
||||||
|
* @param flowQueue |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public Boolean isWorkflowProcess(WorkflowQueue flowQueue) { |
||||||
|
log.info("是否执行开操作票流程环节操作~~~~,流程flowQueue: {}", flowQueue); |
||||||
|
String dictValue = processDictService.selectDictValueByKey(OPERATION_TICKET_KEY); |
||||||
|
if (dictValue.equals(flowQueue.getProcessDefinitionKey())) { |
||||||
|
log.info("已执行操作票流程环节操作~~~~"); |
||||||
|
return true; |
||||||
|
} |
||||||
|
log.error("未是否执行操作票流程环节操作,请联系管理员~~~~"); |
||||||
|
return false; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 两票管理业务方法 |
||||||
|
* |
||||||
|
* @param response |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public void calculate(ProcessWorkFlowResponse response) { |
||||||
|
operationTicketService.findPending(response); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,47 @@ |
|||||||
|
package com.hnac.hzims.ticket.standardTicket.controller; |
||||||
|
|
||||||
|
import com.hnac.hzims.ticket.standardTicket.service.OperationTicketService; |
||||||
|
import com.hnac.hzims.ticket.twoTicket.vo.operation.StandardTicketInfoVo; |
||||||
|
import com.hnac.hzims.ticket.twoTicket.vo.process.WorkTicketVo; |
||||||
|
import io.swagger.annotations.ApiOperation; |
||||||
|
import lombok.RequiredArgsConstructor; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.web.bind.annotation.PostMapping; |
||||||
|
import org.springframework.web.bind.annotation.RequestBody; |
||||||
|
import org.springframework.web.bind.annotation.RequestMapping; |
||||||
|
import org.springframework.web.bind.annotation.RestController; |
||||||
|
|
||||||
|
/** |
||||||
|
* 操作票 |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/6 13:46 |
||||||
|
*/ |
||||||
|
@RequiredArgsConstructor |
||||||
|
@Slf4j |
||||||
|
@RequestMapping("/operation-ticket") |
||||||
|
@RestController |
||||||
|
public class OperationTicketController { |
||||||
|
|
||||||
|
|
||||||
|
private final OperationTicketService operationTicketService; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 启动流程 |
||||||
|
* |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@ApiOperation("启动流程") |
||||||
|
@PostMapping("/startUp") |
||||||
|
public R start(@RequestBody StandardTicketInfoVo StandardTicketInfoVo) { |
||||||
|
operationTicketService.startUp(StandardTicketInfoVo); |
||||||
|
return R.success("申请开票成功"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,28 @@ |
|||||||
|
package com.hnac.hzims.ticket.standardTicket.service; |
||||||
|
|
||||||
|
import com.hnac.hzims.ticket.response.ProcessWorkFlowResponse; |
||||||
|
import com.hnac.hzims.ticket.twoTicket.vo.operation.StandardTicketInfoVo; |
||||||
|
import com.hnac.hzims.ticket.twoTicket.vo.process.WorkTicketVo; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/6 13:48 |
||||||
|
*/ |
||||||
|
public interface OperationTicketService { |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 启动流程 |
||||||
|
* @param standardTicketInfoVo |
||||||
|
*/ |
||||||
|
void startUp(StandardTicketInfoVo standardTicketInfoVo); |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 待处理 |
||||||
|
* @param response |
||||||
|
*/ |
||||||
|
void findPending(ProcessWorkFlowResponse response); |
||||||
|
} |
@ -0,0 +1,360 @@ |
|||||||
|
package com.hnac.hzims.ticket.standardTicket.service.impl; |
||||||
|
|
||||||
|
import com.alibaba.fastjson.JSON; |
||||||
|
import com.alibaba.fastjson.JSONObject; |
||||||
|
import com.baomidou.mybatisplus.core.toolkit.ObjectUtils; |
||||||
|
import com.hnac.hzims.common.logs.utils.StringUtils; |
||||||
|
import com.hnac.hzims.message.MessageConstants; |
||||||
|
import com.hnac.hzims.message.dto.MessagePushRecordDto; |
||||||
|
import com.hnac.hzims.message.fegin.IMessageClient; |
||||||
|
import com.hnac.hzims.operational.station.entity.StationEntity; |
||||||
|
import com.hnac.hzims.operational.station.feign.IStationClient; |
||||||
|
import com.hnac.hzims.ticket.processflow.service.ProcessDictService; |
||||||
|
import com.hnac.hzims.ticket.processflow.strategy.core.ProcessIdWorker; |
||||||
|
import com.hnac.hzims.ticket.response.ProcessWorkFlowResponse; |
||||||
|
import com.hnac.hzims.ticket.standardTicket.entity.StandardTicketInfoEntity; |
||||||
|
import com.hnac.hzims.ticket.standardTicket.entity.StandardTicketMeasureEntity; |
||||||
|
import com.hnac.hzims.ticket.standardTicket.service.IStandardTicketInfoService; |
||||||
|
import com.hnac.hzims.ticket.standardTicket.service.IStandardTicketMeasureService; |
||||||
|
import com.hnac.hzims.ticket.standardTicket.service.OperationTicketService; |
||||||
|
import com.hnac.hzims.ticket.twoTicket.vo.operation.StandardTicketInfoVo; |
||||||
|
import com.hnac.hzims.ticket.twoTicket.vo.operation.StandardTicketMeasureVo; |
||||||
|
import lombok.RequiredArgsConstructor; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.apache.commons.collections4.CollectionUtils; |
||||||
|
import org.apache.commons.lang3.math.NumberUtils; |
||||||
|
import org.springblade.core.log.exception.ServiceException; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.BeanUtil; |
||||||
|
import org.springblade.flow.core.entity.BladeFlow; |
||||||
|
import org.springblade.flow.core.feign.IFlowClient; |
||||||
|
import org.springblade.system.feign.ISysClient; |
||||||
|
import org.springblade.system.user.cache.UserCache; |
||||||
|
import org.springblade.system.user.entity.User; |
||||||
|
import org.springframework.beans.BeanUtils; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import java.time.Duration; |
||||||
|
import java.time.Instant; |
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.util.*; |
||||||
|
import java.util.stream.Collectors; |
||||||
|
|
||||||
|
import static com.hnac.hzims.ticket.processflow.constant.TicketProcessConstant.OPERATION_TICKET_KEY; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/6 13:49 |
||||||
|
*/ |
||||||
|
|
||||||
|
@Slf4j |
||||||
|
@Service |
||||||
|
@RequiredArgsConstructor |
||||||
|
public class OperationTicketServiceImpl implements OperationTicketService { |
||||||
|
|
||||||
|
private final IStandardTicketInfoService standardTicketInfoService; |
||||||
|
|
||||||
|
private final IStandardTicketMeasureService standardTicketMeasureService; |
||||||
|
|
||||||
|
private final IStationClient stationClient; |
||||||
|
|
||||||
|
|
||||||
|
private final ProcessIdWorker processIdWorker; |
||||||
|
|
||||||
|
|
||||||
|
private final ProcessDictService processDictService; |
||||||
|
|
||||||
|
|
||||||
|
private final IFlowClient flowClient; |
||||||
|
|
||||||
|
|
||||||
|
private final IMessageClient messageClient; |
||||||
|
|
||||||
|
|
||||||
|
private final ISysClient sysClient; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 启动流程 |
||||||
|
* |
||||||
|
* @param standardTicketInfoVo |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public void startUp(StandardTicketInfoVo standardTicketInfoVo) { |
||||||
|
log.info("执行 com.hnac.hzims.ticket.standardTicket.service.impl【startUp】 。。。方法"); |
||||||
|
if (standardTicketInfoVo.getSignage() == null) { |
||||||
|
throw new ServiceException("票据抬头未配置,请您到站点管理配置"); |
||||||
|
} |
||||||
|
StationEntity stationEntity = new StationEntity(); |
||||||
|
stationEntity.setCode(standardTicketInfoVo.getSignageCode()); |
||||||
|
R<StationEntity> stationClientOne = stationClient.getOne(stationEntity); |
||||||
|
if (!stationClientOne.isSuccess()) { |
||||||
|
throw new IllegalArgumentException("票据抬头未配置,请您到站点管理配置?"); |
||||||
|
} |
||||||
|
StationEntity station = stationClientOne.getData(); |
||||||
|
if (station == null) { |
||||||
|
throw new ServiceException("票据抬头未配置,请您到站点管理配置"); |
||||||
|
} |
||||||
|
log.info("前端查的站点编号 :{}", standardTicketInfoVo.getSignage()); |
||||||
|
log.info("后端查寻的站点编码 :{}", station.getSignage()); |
||||||
|
if (!standardTicketInfoVo.getSignage().equals(station.getSignage())) { |
||||||
|
throw new ServiceException("票据抬头未配置,请您到站点管理配置"); |
||||||
|
} |
||||||
|
|
||||||
|
//获取站点编号
|
||||||
|
String signage = station.getSignage(); |
||||||
|
if (StringUtils.isBlank(signage) || signage.length() < 2) { |
||||||
|
throw new ServiceException("票据抬头未配置,请您到站点管理配置"); |
||||||
|
} |
||||||
|
signage = signage.length() == 2 ? station.getSignage() : station.getSignage().substring(0, 2); |
||||||
|
|
||||||
|
standardTicketInfoVo.setSignage(signage); |
||||||
|
standardTicketInfoVo.setSignageCode(station.getCode()); |
||||||
|
//2. 获取编码
|
||||||
|
String code = processIdWorker.getTicketByCode(standardTicketInfoVo.getSignage(), this.getTicketWichCode(standardTicketInfoVo.getTicketType()), LocalDateTime.now()); |
||||||
|
standardTicketInfoVo.setCode(code); |
||||||
|
//3. 保存操作对象
|
||||||
|
this.saveStandardTicketInfo(standardTicketInfoVo); |
||||||
|
//5. 保存安全措施
|
||||||
|
List<StandardTicketMeasureVo> standardTicketInfoVos = this.saveStandardTicketMeasure( |
||||||
|
this.saveStandardTicketMeasureWichTicket(standardTicketInfoVo)); |
||||||
|
standardTicketInfoVo.setStandardTicketMeasureVos(standardTicketInfoVos); |
||||||
|
//6. 查询第一种工作票值
|
||||||
|
String dictValue = processDictService.selectDictValueByKey(OPERATION_TICKET_KEY); |
||||||
|
// 7.启动流程
|
||||||
|
this.startProcess(standardTicketInfoVo, dictValue); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 待处理 |
||||||
|
* |
||||||
|
* @param response |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public void findPending(ProcessWorkFlowResponse response) { |
||||||
|
log.info("获取businessKey: {}", response.getBusinessKey()); |
||||||
|
log.info("获取taskId: {} ", response.getTaskId()); |
||||||
|
log.info("获取下一个审批人是: {} ", response.getNextStepOperator()); |
||||||
|
log.info("获取下一个用户Id是: {} ", response.getUserId()); |
||||||
|
log.info("获取当前任务名称是: {} ", response.getTaskName()); |
||||||
|
log.info("获取根据handleType区分是用户还是候选组角色: {}", response.getHandleType()); |
||||||
|
//json转换表单
|
||||||
|
String formData = JSON.toJSONString(response.getVariables()); |
||||||
|
log.info("获取表单的数据:{}", formData); |
||||||
|
StandardTicketInfoVo standardTicketInfoVo = null; |
||||||
|
try { |
||||||
|
JSONObject jsonObject = JSONObject.parseObject(formData); |
||||||
|
standardTicketInfoVo = JSONObject.toJavaObject(jsonObject, StandardTicketInfoVo.class); |
||||||
|
} catch (Exception e) { |
||||||
|
log.error("获取表单出现异常了~~~~"); |
||||||
|
throw new IllegalArgumentException(e.getMessage()); |
||||||
|
} |
||||||
|
|
||||||
|
//1.查询操作票信息
|
||||||
|
Long id = NumberUtils.toLong(response.getBusinessKey()); |
||||||
|
StandardTicketInfoEntity standardTicketInfoEntity = standardTicketInfoService.getById(id); |
||||||
|
if (ObjectUtils.isEmpty(standardTicketInfoEntity)) { |
||||||
|
log.error("获取操作票不存在"); |
||||||
|
return; |
||||||
|
} |
||||||
|
//设置id
|
||||||
|
StandardTicketInfoEntity newStandardTicketInfoEntity = new StandardTicketInfoEntity(); |
||||||
|
BeanUtils.copyProperties(standardTicketInfoVo, newStandardTicketInfoEntity); |
||||||
|
newStandardTicketInfoEntity.setId(id); |
||||||
|
//填充操作票信息
|
||||||
|
saveStandardTicketInfoEntity(newStandardTicketInfoEntity, response); |
||||||
|
newStandardTicketInfoEntity.setProcessInstanceId(response.getProcessInstanceId()); |
||||||
|
standardTicketInfoService.updateById(newStandardTicketInfoEntity); |
||||||
|
|
||||||
|
//更新安全措施
|
||||||
|
List<StandardTicketMeasureVo> standardTicketMeasureVos = standardTicketInfoVo.getStandardTicketMeasureVos(); |
||||||
|
if (CollectionUtils.isNotEmpty(standardTicketMeasureVos)) { |
||||||
|
List<StandardTicketMeasureEntity> standardTicketMeasureEntities = standardTicketMeasureVos.stream().map(standardTicketMeasureVo -> { |
||||||
|
StandardTicketMeasureEntity standardTicketMeasureEntity = new StandardTicketMeasureEntity(); |
||||||
|
BeanUtils.copyProperties(standardTicketMeasureVo, standardTicketMeasureEntity); |
||||||
|
return standardTicketMeasureEntity; |
||||||
|
}).collect(Collectors.toList()); |
||||||
|
standardTicketMeasureService.updateBatchById(standardTicketMeasureEntities); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
//推送消息
|
||||||
|
if (response.getTaskId() != null) { |
||||||
|
MessagePushRecordDto message = new MessagePushRecordDto(); |
||||||
|
message.setBusinessClassify("business"); |
||||||
|
message.setBusinessKey(MessageConstants.BusinessClassifyEnum.OPERATIONTICKETMESSAGE.getKey()); |
||||||
|
message.setSubject(MessageConstants.BusinessClassifyEnum.OPERATIONTICKETMESSAGE.getDescription()); |
||||||
|
message.setTaskId(standardTicketInfoEntity.getId()); |
||||||
|
message.setTenantId("200000"); |
||||||
|
message.setTypes(Arrays.asList(MessageConstants.APP_PUSH, MessageConstants.WS_PUSH)); |
||||||
|
message.setPushType(MessageConstants.IMMEDIATELY); |
||||||
|
//您有一张工作票待审批,工作内容:*****,审批环节:*****;
|
||||||
|
String countent = |
||||||
|
"您有一张工作票待审批,工作内容:".concat(standardTicketInfoEntity.getTitle()) |
||||||
|
.concat(",审批环节:") |
||||||
|
.concat(response.getTaskName()); |
||||||
|
message.setContent(countent); |
||||||
|
message.setDeptId(standardTicketInfoEntity.getCreateDept()); |
||||||
|
R<String> deptName = sysClient.getDeptName(standardTicketInfoEntity.getCreateDept()); |
||||||
|
if (deptName.isSuccess()) { |
||||||
|
message.setDeptName(deptName.getData()); |
||||||
|
} |
||||||
|
String userIds = response.getUserId(); |
||||||
|
if (StringUtils.isBlank(userIds)) { |
||||||
|
log.error("推送的消息不能为空哦,{}", userIds); |
||||||
|
return; |
||||||
|
} |
||||||
|
String[] split = userIds.split(","); |
||||||
|
for (String userId : split) { |
||||||
|
message.setPusher(userId); |
||||||
|
User user = UserCache.getUser(NumberUtils.toLong(userId)); |
||||||
|
if (ObjectUtils.isNotEmpty(user)) { |
||||||
|
message.setPusherName(user.getName()); |
||||||
|
} |
||||||
|
message.setAccount(userId); |
||||||
|
|
||||||
|
message.setCreateUser(NumberUtils.toLong(userId)); |
||||||
|
messageClient.sendMessage(message); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 填充数据 |
||||||
|
* |
||||||
|
* @param newStandardTicketInfoEntity |
||||||
|
* @param response |
||||||
|
*/ |
||||||
|
private void saveStandardTicketInfoEntity(StandardTicketInfoEntity newStandardTicketInfoEntity, ProcessWorkFlowResponse response) { |
||||||
|
String flowDescription = ""; |
||||||
|
//如果response.getHandleType() 是0使用户
|
||||||
|
newStandardTicketInfoEntity.setFlowUserId(response.getUserId()); |
||||||
|
newStandardTicketInfoEntity.setNextStepOperator(response.getNextStepOperator()); |
||||||
|
flowDescription = "审批中,当前环节是".concat(response.getTaskName()).concat(",待").concat(response.getNextStepOperator()).concat("审批"); |
||||||
|
//如果taskId为空
|
||||||
|
String taskId = response.getTaskId(); |
||||||
|
if (StringUtils.isEmpty(taskId)) { |
||||||
|
newStandardTicketInfoEntity.setFlowDescription("结束"); |
||||||
|
newStandardTicketInfoEntity.setFlowTaskId(" "); |
||||||
|
newStandardTicketInfoEntity.setFlowTaskName("结束"); |
||||||
|
newStandardTicketInfoEntity.setNextStepOperator(" "); |
||||||
|
newStandardTicketInfoEntity.setFlowStatus(999); |
||||||
|
} else { |
||||||
|
newStandardTicketInfoEntity.setFlowTaskId(taskId); |
||||||
|
newStandardTicketInfoEntity.setFlowTaskName(response.getTaskName()); |
||||||
|
newStandardTicketInfoEntity.setFlowDescription(flowDescription); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 启动流程 |
||||||
|
* |
||||||
|
* @param standardTicketInfoVo |
||||||
|
* @param dictValue |
||||||
|
*/ |
||||||
|
private void startProcess(StandardTicketInfoVo standardTicketInfoVo, String dictValue) { |
||||||
|
Instant start = Instant.now(); |
||||||
|
Map<String, Object> params = new HashMap<>(4); |
||||||
|
params.put("standardTicketInfoVo", standardTicketInfoVo); |
||||||
|
params.put("taskId", standardTicketInfoVo.getId()); |
||||||
|
params.put("guardianUserIds", "taskUser_".concat(standardTicketInfoVo.getGuardian().toString())); |
||||||
|
//已开启流程
|
||||||
|
R<BladeFlow> processInstanceContainNameByKey = flowClient.startProcessInstanceContainNameByKey(dictValue, |
||||||
|
String.valueOf(standardTicketInfoVo.getId()), standardTicketInfoVo.getTitle(), params); |
||||||
|
if (!processInstanceContainNameByKey.isSuccess()) { |
||||||
|
log.error("processInstanceContainNameByKey {}", processInstanceContainNameByKey.getMsg()); |
||||||
|
throw new ServiceException("不好意思,您暂无权限..."); |
||||||
|
} |
||||||
|
Duration between = Duration.between(start, Instant.now()); |
||||||
|
log.info("================================================================"); |
||||||
|
log.info("耗时: " + (between.getSeconds()) + "秒"); |
||||||
|
log.info("耗时: " + (between.toMillis()) + "毫秒"); |
||||||
|
log.info("================================================================"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 设置安全措施值 |
||||||
|
* |
||||||
|
* @param standardTicketInfoVo |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
private List<StandardTicketMeasureEntity> saveStandardTicketMeasureWichTicket(StandardTicketInfoVo standardTicketInfoVo) { |
||||||
|
List<StandardTicketMeasureVo> standardTicketMeasureVos = standardTicketInfoVo.getStandardTicketMeasureVos(); |
||||||
|
if (CollectionUtils.isNotEmpty(standardTicketMeasureVos)) { |
||||||
|
List<StandardTicketMeasureEntity> measureEntityList = BeanUtil.copyProperties(standardTicketMeasureVos, |
||||||
|
StandardTicketMeasureEntity.class); |
||||||
|
List<StandardTicketMeasureEntity> collect = measureEntityList.stream().map(item -> { |
||||||
|
item.setTicketId(standardTicketInfoVo.getId()); |
||||||
|
return item; |
||||||
|
}).collect(Collectors.toList()); |
||||||
|
return collect; |
||||||
|
|
||||||
|
} |
||||||
|
return null; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 保存安全措施 |
||||||
|
* |
||||||
|
* @param measureEntityList |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
private List<StandardTicketMeasureVo> saveStandardTicketMeasure(List<StandardTicketMeasureEntity> measureEntityList) { |
||||||
|
if (CollectionUtils.isEmpty(measureEntityList)) { |
||||||
|
log.error("安全措施为空哦"); |
||||||
|
return new ArrayList<>(); |
||||||
|
} |
||||||
|
boolean save = standardTicketMeasureService.saveBatch(measureEntityList); |
||||||
|
if (!save) { |
||||||
|
throw new ServiceException("Save failed"); |
||||||
|
} |
||||||
|
|
||||||
|
return measureEntityList.stream().map(item -> { |
||||||
|
StandardTicketMeasureVo standardTicketMeasureVo = new StandardTicketMeasureVo(); |
||||||
|
BeanUtils.copyProperties(item, standardTicketMeasureVo); |
||||||
|
return standardTicketMeasureVo; |
||||||
|
}).collect(Collectors.toList()); |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 保存工作对象 |
||||||
|
* |
||||||
|
* @param standardTicketInfoVo |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
private void saveStandardTicketInfo(StandardTicketInfoVo standardTicketInfoVo) { |
||||||
|
StandardTicketInfoEntity standardTicketInfo = new StandardTicketInfoEntity(); |
||||||
|
BeanUtils.copyProperties(standardTicketInfoVo, standardTicketInfo); |
||||||
|
boolean save = standardTicketInfoService.save(standardTicketInfo); |
||||||
|
if (!save) { |
||||||
|
throw new ServiceException("Save failed"); |
||||||
|
} |
||||||
|
BeanUtils.copyProperties(standardTicketInfo, standardTicketInfoVo); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 工作类型生成工作票类型 |
||||||
|
*/ |
||||||
|
private String getTicketWichCode(String type) { |
||||||
|
String result = null; |
||||||
|
switch (type) { |
||||||
|
case "3": |
||||||
|
result = "DZ"; |
||||||
|
break; |
||||||
|
case "4": |
||||||
|
result = type; |
||||||
|
break; |
||||||
|
case "5": |
||||||
|
result = type; |
||||||
|
break; |
||||||
|
default: |
||||||
|
result = "DZ"; |
||||||
|
break; |
||||||
|
} |
||||||
|
return result; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,120 @@ |
|||||||
|
package com.hnac.hzims.ticket.twoTicket.vo.operation; |
||||||
|
|
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.experimental.Accessors; |
||||||
|
import org.springblade.core.tenant.mp.TenantEntity; |
||||||
|
import org.springframework.format.annotation.DateTimeFormat; |
||||||
|
|
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/6 15:39 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@Accessors(chain = true) |
||||||
|
public class StandardTicketInfoVo extends TenantEntity { |
||||||
|
|
||||||
|
|
||||||
|
@ApiModelProperty("票据类型") |
||||||
|
private String ticketType; |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
@ApiModelProperty("单位") |
||||||
|
private String company; |
||||||
|
|
||||||
|
@ApiModelProperty("工作流ID") |
||||||
|
private String processInstanceId; |
||||||
|
|
||||||
|
|
||||||
|
@ApiModelProperty("编号") |
||||||
|
private String code; |
||||||
|
|
||||||
|
@ApiModelProperty("发令人") |
||||||
|
private Long issueOrderPerson; |
||||||
|
|
||||||
|
@ApiModelProperty("受令人") |
||||||
|
private Long accessOrderPerson; |
||||||
|
|
||||||
|
@ApiModelProperty("发令时间") |
||||||
|
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
private LocalDateTime issueOrderTime; |
||||||
|
|
||||||
|
@ApiModelProperty("操作开始时间") |
||||||
|
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
private LocalDateTime startTime; |
||||||
|
|
||||||
|
@ApiModelProperty("操作结束时间") |
||||||
|
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
private LocalDateTime endTime; |
||||||
|
|
||||||
|
@ApiModelProperty("操作任务") |
||||||
|
private String title; |
||||||
|
|
||||||
|
@ApiModelProperty("任务类型") |
||||||
|
private String taskType; |
||||||
|
|
||||||
|
@ApiModelProperty("关联业务任务ID") |
||||||
|
private Long taskId; |
||||||
|
|
||||||
|
@ApiModelProperty("关联业务名称") |
||||||
|
private String taskName; |
||||||
|
|
||||||
|
@ApiModelProperty("备注") |
||||||
|
private String remark; |
||||||
|
|
||||||
|
@ApiModelProperty("监护人") |
||||||
|
private Long guardian; |
||||||
|
|
||||||
|
@ApiModelProperty("操作人") |
||||||
|
private Long operator; |
||||||
|
|
||||||
|
@ApiModelProperty("操作时间") |
||||||
|
private LocalDateTime operateTime; |
||||||
|
|
||||||
|
@ApiModelProperty("负责人确认时间") |
||||||
|
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
private LocalDateTime guardianshipTime; |
||||||
|
|
||||||
|
@ApiModelProperty("值班负责人") |
||||||
|
private Long principal; |
||||||
|
|
||||||
|
@ApiModelProperty("值班负责人确认时间") |
||||||
|
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
private LocalDateTime principalTime; |
||||||
|
|
||||||
|
@ApiModelProperty("是否评价") |
||||||
|
private Integer isEvaluate; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* AA/AAA/AAAA由建站时建站人填写必须系统验证唯一性 |
||||||
|
* 站点标识-两票编码 |
||||||
|
*/ |
||||||
|
@ApiModelProperty("站点标识-两票编码") |
||||||
|
private String signage; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 编号(原站点ID) |
||||||
|
*/ |
||||||
|
@ApiModelProperty("编号(原站点ID)") |
||||||
|
private String signageCode; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 安全措施 |
||||||
|
*/ |
||||||
|
private List<StandardTicketMeasureVo> standardTicketMeasureVos; |
||||||
|
} |
@ -0,0 +1,57 @@ |
|||||||
|
package com.hnac.hzims.ticket.twoTicket.vo.operation; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.annotation.FieldStrategy; |
||||||
|
import com.baomidou.mybatisplus.annotation.TableField; |
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import com.fasterxml.jackson.databind.annotation.JsonSerialize; |
||||||
|
import com.fasterxml.jackson.databind.ser.std.NullSerializer; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import org.springblade.core.mp.support.QueryField; |
||||||
|
import org.springblade.core.mp.support.SqlCondition; |
||||||
|
import org.springblade.core.tenant.mp.TenantEntity; |
||||||
|
import org.springframework.format.annotation.DateTimeFormat; |
||||||
|
|
||||||
|
import java.time.LocalDateTime; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/6 15:47 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
public class StandardTicketMeasureVo extends TenantEntity { |
||||||
|
|
||||||
|
|
||||||
|
private static final long serialVersionUID = 8503146008417850183L; |
||||||
|
|
||||||
|
@ApiModelProperty("标准票ID") |
||||||
|
private Long ticketId; |
||||||
|
|
||||||
|
@ApiModelProperty("分组") |
||||||
|
private Long matterGroup; |
||||||
|
|
||||||
|
@ApiModelProperty("安全措施ID") |
||||||
|
private Long measureId; |
||||||
|
|
||||||
|
@ApiModelProperty("安全措施") |
||||||
|
private String measure; |
||||||
|
|
||||||
|
@ApiModelProperty("执行人") |
||||||
|
private Long executor; |
||||||
|
|
||||||
|
@ApiModelProperty("执行时间") |
||||||
|
@DateTimeFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
@JsonFormat(pattern = "yyyy-MM-dd HH:mm:ss") |
||||||
|
private LocalDateTime executeTime; |
||||||
|
|
||||||
|
@ApiModelProperty("执行图片") |
||||||
|
private String executeImgUrl; |
||||||
|
|
||||||
|
@ApiModelProperty("执行备注") |
||||||
|
private String remark; |
||||||
|
|
||||||
|
@ApiModelProperty("操作事项排序") |
||||||
|
private Integer sort; |
||||||
|
} |
Loading…
Reference in new issue