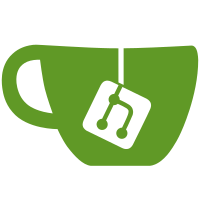
16 changed files with 484 additions and 7 deletions
@ -0,0 +1,35 @@
|
||||
package com.hnac.hzims.alarm.vo; |
||||
|
||||
import io.swagger.annotations.ApiModelProperty; |
||||
import lombok.Data; |
||||
|
||||
/** |
||||
* @author ysj |
||||
*/ |
||||
@Data |
||||
public class AlarmHandleMarkVo { |
||||
|
||||
@ApiModelProperty(value = "是否集中监控右侧列表展示 : 0-展示 1-不展示") |
||||
private Integer isRightTabulation; |
||||
|
||||
@ApiModelProperty(value = "是否集中监控弹窗展示 : 0-展示 1-不展示") |
||||
private Integer isShowAlert; |
||||
|
||||
@ApiModelProperty(value = "是否集中监控铃铛展示 : 0-展示 1-不展示") |
||||
private Integer isSmallBell; |
||||
|
||||
@ApiModelProperty(value = "是否集中监控遮罩展示 : 0-展示 1-不展示") |
||||
private Integer isMask; |
||||
|
||||
@ApiModelProperty(value = "是否语音播报 : 0-播报 1-不播报") |
||||
private Integer isBroadcast; |
||||
|
||||
@ApiModelProperty(value = "是否进行平台消息推送 : 0-推送 1-不推送") |
||||
private Integer isPlatformMessage; |
||||
|
||||
@ApiModelProperty(value = "是否短信推送 :0-推送 1-不推送") |
||||
private Integer isShortMessage; |
||||
|
||||
@ApiModelProperty(value = "是否微信公众号消息推送 :0-推送 1-不推送") |
||||
private Integer isWxMessage; |
||||
} |
@ -0,0 +1,36 @@
|
||||
package com.hnac.hzims.alarm.monitor.controller; |
||||
|
||||
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||
import com.hnac.hzims.alarm.entity.AlarmEntity; |
||||
import com.hnac.hzims.alarm.monitor.service.AlarmSaveService; |
||||
import io.swagger.annotations.Api; |
||||
import io.swagger.annotations.ApiOperation; |
||||
import lombok.AllArgsConstructor; |
||||
import org.springblade.core.boot.ctrl.BladeController; |
||||
import org.springblade.core.tool.api.R; |
||||
import org.springframework.web.bind.annotation.PostMapping; |
||||
import org.springframework.web.bind.annotation.RequestBody; |
||||
import org.springframework.web.bind.annotation.RequestMapping; |
||||
import org.springframework.web.bind.annotation.RestController; |
||||
|
||||
import java.util.List; |
||||
|
||||
/** |
||||
* @author ysj |
||||
*/ |
||||
@RestController |
||||
@AllArgsConstructor |
||||
@RequestMapping("/alarm/test") |
||||
@Api(value = "告警配置", tags = "告警配置") |
||||
public class AlarmTestController extends BladeController { |
||||
|
||||
private final AlarmSaveService alarmSaveService; |
||||
|
||||
@PostMapping("/save") |
||||
@ApiOperationSupport(order = 1) |
||||
@ApiOperation(value = "新增告警", notes = "传入AlarmConfigEntity对象") |
||||
public R save(@RequestBody List<AlarmEntity> entitys) { |
||||
return R.status(alarmSaveService.save(entitys)); |
||||
} |
||||
|
||||
} |
@ -0,0 +1,31 @@
|
||||
package com.hnac.hzims.alarm.monitor.listener; |
||||
|
||||
import com.hnac.hzims.alarm.monitor.service.AlarmMessageSendService; |
||||
import org.springframework.beans.factory.annotation.Autowired; |
||||
import org.springframework.data.redis.connection.Message; |
||||
import org.springframework.data.redis.connection.MessageListener; |
||||
import org.springframework.data.redis.core.RedisTemplate; |
||||
import org.springframework.data.redis.serializer.RedisSerializer; |
||||
import org.springframework.stereotype.Component; |
||||
|
||||
/** |
||||
* @author ysj |
||||
* @version 4.0.0 |
||||
* @create 2023-11-09-14:04 |
||||
*/ |
||||
@Component |
||||
public class AlarmListener implements MessageListener { |
||||
|
||||
@Autowired |
||||
private AlarmMessageSendService alarmMessageSendService; |
||||
|
||||
@Autowired |
||||
private RedisTemplate redisTemplate; |
||||
|
||||
@Override |
||||
public void onMessage(Message message, byte[] pattern) { |
||||
RedisSerializer<String> serializer = redisTemplate.getStringSerializer(); |
||||
String msg = serializer.deserialize(message.getBody()); |
||||
System.out.println("接收到的消息是:" + msg); |
||||
} |
||||
} |
@ -0,0 +1,44 @@
|
||||
package com.hnac.hzims.alarm.monitor.listener; |
||||
|
||||
import com.hnac.hzims.common.config.RedisMessageListener; |
||||
import org.springframework.context.annotation.Bean; |
||||
import org.springframework.data.redis.connection.RedisConnectionFactory; |
||||
import org.springframework.data.redis.listener.PatternTopic; |
||||
import org.springframework.data.redis.listener.RedisMessageListenerContainer; |
||||
import org.springframework.data.redis.listener.adapter.MessageListenerAdapter; |
||||
|
||||
/** |
||||
* @author ysj |
||||
* @version 4.0.0 |
||||
* @create 2023-11-09 14:02 |
||||
*/ |
||||
public class QuqueConfig { |
||||
|
||||
/** |
||||
* 创建连接工厂 |
||||
* |
||||
* @param connectionFactory |
||||
* @param adapter |
||||
* @return |
||||
*/ |
||||
@Bean |
||||
public RedisMessageListenerContainer container(RedisConnectionFactory connectionFactory, |
||||
MessageListenerAdapter adapter) { |
||||
RedisMessageListenerContainer container = new RedisMessageListenerContainer(); |
||||
container.setConnectionFactory(connectionFactory); |
||||
//监听对应的channel
|
||||
container.addMessageListener(adapter, new PatternTopic("alarmchannel")); |
||||
return container; |
||||
} |
||||
|
||||
/** |
||||
* 绑定消息监听者和接收监听的方法 |
||||
* @param message |
||||
* @return |
||||
*/ |
||||
@Bean |
||||
public MessageListenerAdapter adapter(RedisMessageListener message) { |
||||
// onMessage 如果RedisMessage 中 没有实现接口,这个参数必须跟RedisMessage中的读取信息的方法名称一样
|
||||
return new MessageListenerAdapter(message, "onMessage"); |
||||
} |
||||
} |
@ -0,0 +1,9 @@
|
||||
package com.hnac.hzims.alarm.monitor.service; |
||||
|
||||
/** |
||||
* @author ysj |
||||
*/ |
||||
public interface AlarmMessageSendService { |
||||
|
||||
|
||||
} |
@ -0,0 +1,13 @@
|
||||
package com.hnac.hzims.alarm.monitor.service; |
||||
|
||||
import com.hnac.hzims.alarm.entity.AlarmEntity; |
||||
|
||||
import java.util.List; |
||||
|
||||
/** |
||||
* @author ysj |
||||
*/ |
||||
public interface AlarmSaveService { |
||||
|
||||
Boolean save(List<AlarmEntity> alarms); |
||||
} |
@ -0,0 +1,17 @@
|
||||
package com.hnac.hzims.alarm.monitor.service.impl; |
||||
|
||||
import com.hnac.hzims.alarm.monitor.service.AlarmMessageSendService; |
||||
import lombok.AllArgsConstructor; |
||||
import lombok.extern.slf4j.Slf4j; |
||||
import org.springframework.stereotype.Service; |
||||
|
||||
/** |
||||
* @author ysj |
||||
*/ |
||||
@AllArgsConstructor |
||||
@Service |
||||
@Slf4j |
||||
public class AlarmMessageSendServiceImpl implements AlarmMessageSendService { |
||||
|
||||
|
||||
} |
@ -0,0 +1,92 @@
|
||||
package com.hnac.hzims.alarm.monitor.service.impl; |
||||
|
||||
import com.hnac.hzims.alarm.config.service.AlarmConfigService; |
||||
import com.hnac.hzims.alarm.entity.AlarmEntity; |
||||
import com.hnac.hzims.alarm.monitor.service.AlarmSaveService; |
||||
import com.hnac.hzims.alarm.show.service.AlarmService; |
||||
import com.hnac.hzims.alarm.vo.AlarmHandleMarkVo; |
||||
import com.hnac.hzims.operational.station.entity.StationEntity; |
||||
import com.hnac.hzims.operational.station.feign.IStationClient; |
||||
import lombok.AllArgsConstructor; |
||||
import lombok.extern.slf4j.Slf4j; |
||||
import org.springblade.core.tool.api.R; |
||||
import org.springblade.core.tool.utils.CollectionUtil; |
||||
import org.springblade.core.tool.utils.ObjectUtil; |
||||
import org.springframework.beans.factory.annotation.Autowired; |
||||
import org.springframework.data.redis.core.RedisTemplate; |
||||
import org.springframework.stereotype.Service; |
||||
import org.springframework.web.bind.annotation.GetMapping; |
||||
import org.springframework.web.bind.annotation.PathVariable; |
||||
|
||||
import java.util.List; |
||||
import java.util.stream.Collectors; |
||||
|
||||
/** |
||||
* @author ysj |
||||
*/ |
||||
@AllArgsConstructor |
||||
@Service |
||||
@Slf4j |
||||
public class AlarmSaveServiceImpl implements AlarmSaveService { |
||||
|
||||
private final AlarmService alarmService; |
||||
|
||||
private final AlarmConfigService alarmConfigService; |
||||
|
||||
private final IStationClient stationClient; |
||||
|
||||
private final RedisTemplate redisTemplate; |
||||
|
||||
@GetMapping(value = "pub/{msg}") |
||||
public String pubMsg(@PathVariable String msg){ |
||||
|
||||
return msg; |
||||
} |
||||
|
||||
/** |
||||
* 告警数据保存 |
||||
* @param alarms |
||||
* @return |
||||
*/ |
||||
@Override |
||||
public Boolean save(List<AlarmEntity> alarms) { |
||||
|
||||
// 步骤1.查询告警数据对应站点
|
||||
R<List<StationEntity>> result = stationClient.querySatationByCodes(alarms.stream().map(AlarmEntity::getStationId).collect(Collectors.toList())); |
||||
if(!result.isSuccess() || CollectionUtil.isEmpty(result.getData())){ |
||||
log.error("alarm query station is null : {}",alarms.stream().map(AlarmEntity::getAlarmId).collect(Collectors.toList())); |
||||
return false; |
||||
} |
||||
// 步骤2.遍历保存告警数据
|
||||
alarms.forEach(alarm->{ |
||||
|
||||
// 步骤3.根据站点查询配置标识
|
||||
AlarmHandleMarkVo mark = alarmConfigService.mark(alarm.getStationId(),alarm.getAlarmSource(),alarm.getAlarmType()); |
||||
if(ObjectUtil.isEmpty(mark)){ |
||||
log.error("alarm obtain mark is null : {}",alarm.getAlarmId()); |
||||
return; |
||||
} |
||||
|
||||
// 步骤4.告警处理标识赋值
|
||||
alarm.setIsRightTabulation(mark.getIsRightTabulation()); |
||||
alarm.setIsBroadcast(mark.getIsBroadcast()); |
||||
alarm.setIsMask(mark.getIsMask()); |
||||
alarm.setIsPlatformMessage(mark.getIsPlatformMessage()); |
||||
alarm.setIsShowAlert(mark.getIsShowAlert()); |
||||
alarm.setIsSmallBell(mark.getIsSmallBell()); |
||||
alarm.setIsShortMessage(mark.getIsShortMessage()); |
||||
alarm.setIsWxMessage(mark.getIsWxMessage()); |
||||
|
||||
// 步骤5.保存当日告警数据
|
||||
boolean isSave = alarmService.save(alarm); |
||||
if(!isSave){ |
||||
log.error("alarm save fail : {}",alarm.getAlarmId()); |
||||
return; |
||||
} |
||||
|
||||
// 步骤6.发生数据至redis告警队列
|
||||
redisTemplate.convertAndSend("alarmchannel",alarm); |
||||
}); |
||||
return true; |
||||
} |
||||
} |
Loading…
Reference in new issue