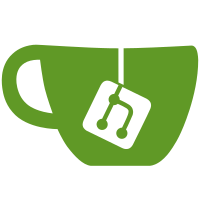
9 changed files with 106 additions and 15 deletions
@ -0,0 +1,51 @@ |
|||||||
|
package com.hnac.hzims.operational.alert.vo; |
||||||
|
|
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
|
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author ysj |
||||||
|
* @date 2023/03/17 10:02:33 |
||||||
|
* @version 4.0.0 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
public class AlarmHandleVo{ |
||||||
|
|
||||||
|
@ApiModelProperty("站点编码") |
||||||
|
private String stationCode; |
||||||
|
|
||||||
|
@ApiModelProperty("告警类型") |
||||||
|
private Long alarmType; |
||||||
|
|
||||||
|
@ApiModelProperty("告警内容") |
||||||
|
private String alarmContent; |
||||||
|
|
||||||
|
@ApiModelProperty("告警时间") |
||||||
|
private Date alarmTime; |
||||||
|
|
||||||
|
@ApiModelProperty("处理方式:0-处理 1-延后 2-误报") |
||||||
|
private Long handleWay; |
||||||
|
|
||||||
|
@ApiModelProperty("延后处理时间") |
||||||
|
private Date delayTime; |
||||||
|
|
||||||
|
@ApiModelProperty("延后处理时间") |
||||||
|
private String delayCause; |
||||||
|
|
||||||
|
@ApiModelProperty("误报描述") |
||||||
|
private String falseAlarmDesc; |
||||||
|
|
||||||
|
@ApiModelProperty("附件路径") |
||||||
|
private String filePath; |
||||||
|
|
||||||
|
@ApiModelProperty("通知处理人") |
||||||
|
private String handleUser; |
||||||
|
|
||||||
|
@ApiModelProperty("现象Id") |
||||||
|
private Long phenomenonId; |
||||||
|
|
||||||
|
@ApiModelProperty("检修ID") |
||||||
|
private Long accessId; |
||||||
|
} |
@ -1,21 +1,49 @@ |
|||||||
package com.hnac.hzims.operational.alert.service.impl; |
package com.hnac.hzims.operational.alert.service.impl; |
||||||
|
|
||||||
|
import com.hnac.hzims.operational.alert.constants.AbnormalAlarmConstant; |
||||||
|
import com.hnac.hzims.operational.alert.entity.AlarmHandleDetailEntity; |
||||||
import com.hnac.hzims.operational.alert.entity.AlarmHandleEntity; |
import com.hnac.hzims.operational.alert.entity.AlarmHandleEntity; |
||||||
import com.hnac.hzims.operational.alert.mapper.AlarmHandleMapper; |
import com.hnac.hzims.operational.alert.mapper.AlarmHandleMapper; |
||||||
|
import com.hnac.hzims.operational.alert.service.AlarmHandleDetailService; |
||||||
import com.hnac.hzims.operational.alert.service.AlarmHandleService; |
import com.hnac.hzims.operational.alert.service.AlarmHandleService; |
||||||
|
import com.hnac.hzims.operational.alert.vo.AlarmHandleVo; |
||||||
import lombok.RequiredArgsConstructor; |
import lombok.RequiredArgsConstructor; |
||||||
import lombok.extern.slf4j.Slf4j; |
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springblade.core.log.exception.ServiceException; |
||||||
import org.springblade.core.mp.base.BaseServiceImpl; |
import org.springblade.core.mp.base.BaseServiceImpl; |
||||||
|
import org.springframework.beans.BeanUtils; |
||||||
import org.springframework.stereotype.Service; |
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
/** |
/** |
||||||
* @author ysj |
* @author ysj |
||||||
* @date 2023/03/09 09:29:34 |
|
||||||
* @version 4.0.0 |
* @version 4.0.0 |
||||||
|
* @date 2023/03/09 09:29:34 |
||||||
*/ |
*/ |
||||||
@Slf4j |
@Slf4j |
||||||
@Service |
@Service |
||||||
@RequiredArgsConstructor |
@RequiredArgsConstructor |
||||||
public class AlarmHandleServiceImpl extends BaseServiceImpl<AlarmHandleMapper, AlarmHandleEntity> implements AlarmHandleService { |
public class AlarmHandleServiceImpl extends BaseServiceImpl<AlarmHandleMapper, AlarmHandleEntity> implements AlarmHandleService { |
||||||
|
|
||||||
|
private final AlarmHandleDetailService detailService; |
||||||
|
|
||||||
|
/** |
||||||
|
* 告警处理 |
||||||
|
* |
||||||
|
* @param param |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public boolean execute(AlarmHandleVo param) { |
||||||
|
Long way = param.getHandleWay(); |
||||||
|
if (!AbnormalAlarmConstant.HANDLE_WAY.contains(way)) { |
||||||
|
throw new ServiceException("无效处理!"); |
||||||
|
} |
||||||
|
AlarmHandleDetailEntity detail = new AlarmHandleDetailEntity(); |
||||||
|
BeanUtils.copyProperties(param,detail); |
||||||
|
detailService.save(detail); |
||||||
|
AlarmHandleEntity entity = new AlarmHandleEntity(); |
||||||
|
entity.setDetailId(detail.getId()); |
||||||
|
BeanUtils.copyProperties(param,entity); |
||||||
|
return this.save(entity); |
||||||
|
} |
||||||
} |
} |
||||||
|
Loading…
Reference in new issue