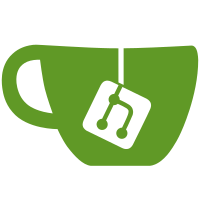
8 changed files with 431 additions and 100 deletions
@ -0,0 +1,89 @@ |
|||||||
|
package com.hnac.hzims.operational.main.vo; |
||||||
|
|
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import com.fasterxml.jackson.databind.annotation.JsonSerialize; |
||||||
|
import com.fasterxml.jackson.databind.ser.std.NullSerializer; |
||||||
|
import com.hnac.hzims.hzimsweather.response.weather.Daily; |
||||||
|
import io.swagger.annotations.ApiModel; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import org.springblade.core.tool.utils.DateUtil; |
||||||
|
import org.springframework.format.annotation.DateTimeFormat; |
||||||
|
|
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author ysj |
||||||
|
* @date 2023/03/29 15:14:34 |
||||||
|
* @version 4.0.0 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@ApiModel(value = "水利站点首页对象") |
||||||
|
public class PhotovoltaicLoadGenerateVo { |
||||||
|
|
||||||
|
@ApiModelProperty(value = "机构Id") |
||||||
|
private Long deptId; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "机构名称") |
||||||
|
private String deptName; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "站点编码") |
||||||
|
private String stationCode; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "地址") |
||||||
|
private String address; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "投运时间") |
||||||
|
@DateTimeFormat(pattern = DateUtil.PATTERN_DATETIME) |
||||||
|
@JsonFormat(pattern = DateUtil.PATTERN_DATETIME) |
||||||
|
private LocalDateTime operationTime; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "总装机容量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double capacity; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "安全运行天数") |
||||||
|
private Integer runDay; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "总发电负荷") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double load; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "日发电量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double generateDay; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "月发电量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double generateMon; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "年发电量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double generateYear; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "总发电量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double generate; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "站点实时温度") |
||||||
|
private String temp; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "站点收益") |
||||||
|
private Double income; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "逆变器数量") |
||||||
|
private Integer inverterCount; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "七日内天气") |
||||||
|
private List<Daily> weather; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "发电排行") |
||||||
|
private List<PhotovoltaicDeviceVo> devices; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "年发电量完成百分比") |
||||||
|
private List<PowerYearVo> generateSurvey; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "设备信息") |
||||||
|
private List<PhotovoltaicDeviceChartsVo> charts; |
||||||
|
} |
@ -0,0 +1,89 @@ |
|||||||
|
package com.hnac.hzims.operational.main.vo; |
||||||
|
|
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import com.fasterxml.jackson.databind.annotation.JsonSerialize; |
||||||
|
import com.fasterxml.jackson.databind.ser.std.NullSerializer; |
||||||
|
import com.hnac.hzims.hzimsweather.response.weather.Daily; |
||||||
|
import io.swagger.annotations.ApiModel; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import org.springblade.core.tool.utils.DateUtil; |
||||||
|
import org.springframework.format.annotation.DateTimeFormat; |
||||||
|
|
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author ysj |
||||||
|
* @date 2023/03/29 15:14:34 |
||||||
|
* @version 4.0.0 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@ApiModel(value = "水利站点首页对象") |
||||||
|
public class PhotovoltaicStationAppVo { |
||||||
|
|
||||||
|
@ApiModelProperty(value = "机构Id") |
||||||
|
private Long deptId; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "机构名称") |
||||||
|
private String deptName; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "站点编码") |
||||||
|
private String stationCode; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "地址") |
||||||
|
private String address; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "投运时间") |
||||||
|
@DateTimeFormat(pattern = DateUtil.PATTERN_DATETIME) |
||||||
|
@JsonFormat(pattern = DateUtil.PATTERN_DATETIME) |
||||||
|
private LocalDateTime operationTime; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "总装机容量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double capacity; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "安全运行天数") |
||||||
|
private Integer runDay; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "总发电负荷") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double load; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "日发电量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double generateDay; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "月发电量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double generateMon; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "年发电量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double generateYear; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "总发电量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double generate; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "站点实时温度") |
||||||
|
private String temp; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "站点收益") |
||||||
|
private Double income; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "逆变器数量") |
||||||
|
private Integer inverterCount; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "七日内天气") |
||||||
|
private List<Daily> weather; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "发电排行") |
||||||
|
private List<PhotovoltaicDeviceVo> devices; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "年发电量完成百分比") |
||||||
|
private List<PowerYearVo> generateSurvey; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "设备信息") |
||||||
|
private List<PhotovoltaicDeviceChartsVo> charts; |
||||||
|
} |
@ -0,0 +1,87 @@ |
|||||||
|
package com.hnac.hzims.operational.main.vo; |
||||||
|
|
||||||
|
|
||||||
|
import com.fasterxml.jackson.databind.annotation.JsonSerialize; |
||||||
|
import com.fasterxml.jackson.databind.ser.std.NullSerializer; |
||||||
|
import com.hnac.hzims.hzimsweather.response.weather.Daily; |
||||||
|
import io.swagger.annotations.ApiModel; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @author ysj |
||||||
|
* @date 2023/03/24 09:58:57 |
||||||
|
* @version 4.0.0 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
@ApiModel(value = "发电量数据") |
||||||
|
public class PhotovoltaicSubordinateAppVo { |
||||||
|
|
||||||
|
@ApiModelProperty(value = "机构ID") |
||||||
|
private Long deptId; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "机构名称") |
||||||
|
private String deptName; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "地市编码") |
||||||
|
private String areaCode; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "地市名称") |
||||||
|
private String areaName; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "光伏站点类型:0-个体光伏站 1-厂房光伏站") |
||||||
|
private Long type; |
||||||
|
|
||||||
|
@ApiModelProperty("经度(东经)") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Float lgtd; |
||||||
|
|
||||||
|
@ApiModelProperty("纬度(北纬)") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Float lttd; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "容量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double capacity; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "功率") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double load; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "发电量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double generation; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "发电占比") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double generationRate; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "容量利用率") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double capacityUse; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "电站利用率") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Double stationUse; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "站点实时温度") |
||||||
|
private String temp; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "七日内天气") |
||||||
|
private List<Daily> weather; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "排序") |
||||||
|
private Integer sort; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "站点数量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Long stationCount; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "站点使用数量") |
||||||
|
@JsonSerialize(nullsUsing = NullSerializer.class) |
||||||
|
private Long stationUseCount; |
||||||
|
|
||||||
|
|
||||||
|
} |
Loading…
Reference in new issue