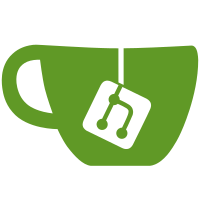
20 changed files with 304 additions and 838 deletions
@ -0,0 +1,41 @@ |
|||||||
|
package com.hnac.hzims.alarm.mqtt; |
||||||
|
|
||||||
|
import com.alibaba.fastjson.JSONObject; |
||||||
|
import com.hnac.hzims.alarm.entity.AlarmEntity; |
||||||
|
import com.hnac.hzims.alarm.handle.service.DroolsAlarmService; |
||||||
|
import com.hnac.hzims.alarm.monitor.service.AlarmSaveService; |
||||||
|
import com.hnac.hzinfo.subscribe.SubscribeCallBack; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
/** |
||||||
|
* |
||||||
|
* @Author: ty |
||||||
|
*/ |
||||||
|
|
||||||
|
@Service |
||||||
|
@Slf4j |
||||||
|
public class DroolsMqttConsumer implements SubscribeCallBack { |
||||||
|
@Autowired |
||||||
|
private DroolsAlarmService droolsAlarmService; |
||||||
|
@Autowired |
||||||
|
private AlarmSaveService alarmSaveService; |
||||||
|
@Override |
||||||
|
public void onMessage(Map<String, Object> data) { |
||||||
|
System.out.println("监听到消息"+data.toString()); |
||||||
|
String s = JSONObject.toJSONString(data); |
||||||
|
//消费代码
|
||||||
|
List<AlarmEntity> alarmEntities = droolsAlarmService.receiveMessage(s); |
||||||
|
//统一数据处理
|
||||||
|
try { |
||||||
|
alarmSaveService.save(alarmEntities); |
||||||
|
}catch (Exception e){ |
||||||
|
log.error("规则引擎告警数据处理报错(DroolsAlarm):"+e); |
||||||
|
System.out.println("规则引擎告警数据处理报错(DroolsAlarm):"+e); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,40 @@ |
|||||||
|
package com.hnac.hzims.alarm.mqtt; |
||||||
|
|
||||||
|
import com.alibaba.fastjson.JSONObject; |
||||||
|
import com.hnac.hzims.alarm.entity.AlarmEntity; |
||||||
|
import com.hnac.hzims.alarm.handle.service.SystemAlarmService; |
||||||
|
import com.hnac.hzims.alarm.monitor.service.AlarmSaveService; |
||||||
|
import com.hnac.hzinfo.subscribe.SubscribeCallBack; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
import java.util.Map; |
||||||
|
|
||||||
|
/** |
||||||
|
* |
||||||
|
* @Author: ty |
||||||
|
*/ |
||||||
|
|
||||||
|
@Service |
||||||
|
@Slf4j |
||||||
|
public class HZ300MqttConsumer implements SubscribeCallBack { |
||||||
|
@Autowired |
||||||
|
private SystemAlarmService systemAlarmService; |
||||||
|
@Autowired |
||||||
|
private AlarmSaveService alarmSaveService; |
||||||
|
@Override |
||||||
|
public void onMessage(Map<String, Object> data) { |
||||||
|
System.out.println("监听到消息"+data.toString()); |
||||||
|
String s = JSONObject.toJSONString(data); |
||||||
|
//消费代码
|
||||||
|
List<AlarmEntity> alarmEntities = systemAlarmService.receiveMessage(s); |
||||||
|
//统一数据处理
|
||||||
|
try { |
||||||
|
alarmSaveService.save(alarmEntities); |
||||||
|
}catch (Exception e){ |
||||||
|
log.error("HZ3000告警数据处理报错(HZ300Alarm):"+e); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,68 @@ |
|||||||
|
package com.hnac.hzims.alarm.mqtt; |
||||||
|
|
||||||
|
import com.hnac.hzinfo.subscribe.AnalysisSubscribe; |
||||||
|
import com.hnac.hzinfo.subscribe.DroolsSoeSubscribe; |
||||||
|
import com.hnac.hzinfo.subscribe.HZ3000SoeSubscribe; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.eclipse.paho.client.mqttv3.MqttAsyncClient; |
||||||
|
import org.eclipse.paho.client.mqttv3.MqttException; |
||||||
|
import org.springframework.beans.factory.annotation.Autowired; |
||||||
|
import org.springframework.beans.factory.annotation.Value; |
||||||
|
import org.springframework.stereotype.Component; |
||||||
|
|
||||||
|
import javax.annotation.PostConstruct; |
||||||
|
import java.util.Collections; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author: ty |
||||||
|
*/ |
||||||
|
|
||||||
|
@Component |
||||||
|
@Slf4j |
||||||
|
public class MqttConfig { |
||||||
|
|
||||||
|
@Autowired |
||||||
|
FdpMqttConsumer fdpMqttConsumer; |
||||||
|
@Autowired |
||||||
|
DroolsMqttConsumer droolsMqttConsumer; |
||||||
|
@Autowired |
||||||
|
HZ300MqttConsumer hz300MqttConsumer; |
||||||
|
@Value("${hzims.mqtt.url}") |
||||||
|
private String mqtt_fdp_url; |
||||||
|
@Value("${hzims.mqtt.group}") |
||||||
|
private String mqtt_fdp_group; |
||||||
|
@Value("${hzims.mqtt.password}") |
||||||
|
private String mqtt_fdp_password; |
||||||
|
@Value("${hzims.mqtt.username}") |
||||||
|
private String mqtt_fdp_username; |
||||||
|
// @Value("${hzims.mqtt.fdp.topname}")
|
||||||
|
// private String mqtt_fdp_topname;
|
||||||
|
// @Value("${hzims.mqtt.drools.topname}")
|
||||||
|
// private String mqtt_drools_topname;
|
||||||
|
// @Value("${hzims.mqtt.system.topname}")
|
||||||
|
// private String mqtt_system_topname;
|
||||||
|
@PostConstruct |
||||||
|
public void registerMqtt(){ |
||||||
|
try { |
||||||
|
com.hnac.hzinfo.subscribe.MqttConfig mqttConfig = new com.hnac.hzinfo.subscribe.MqttConfig(); |
||||||
|
mqttConfig.setUrl(mqtt_fdp_url); |
||||||
|
mqttConfig.setGroup(mqtt_fdp_group); |
||||||
|
mqttConfig.setPassword(mqtt_fdp_password); |
||||||
|
mqttConfig.setUsername(mqtt_fdp_username); |
||||||
|
//fdp
|
||||||
|
AnalysisSubscribe analysisSubscribe = new AnalysisSubscribe(); |
||||||
|
MqttAsyncClient fdpClient = analysisSubscribe.subscribe(mqttConfig, Collections.singletonList("#"), fdpMqttConsumer); |
||||||
|
log.info(fdpClient.toString()); |
||||||
|
//drools
|
||||||
|
DroolsSoeSubscribe droolsSoeSubscribe = new DroolsSoeSubscribe(); |
||||||
|
MqttAsyncClient droolsClient = droolsSoeSubscribe.subscribe(mqttConfig, Collections.singletonList("#"), droolsMqttConsumer); |
||||||
|
log.info(droolsClient.toString()); |
||||||
|
//hz3000
|
||||||
|
HZ3000SoeSubscribe hz3000SoeSubscribe = new HZ3000SoeSubscribe(); |
||||||
|
MqttAsyncClient hz3000Client = hz3000SoeSubscribe.subscribe(mqttConfig, Collections.singletonList("#"), hz300MqttConsumer); |
||||||
|
log.info(hz3000Client.toString()); |
||||||
|
}catch (MqttException e){ |
||||||
|
log.error(e.toString()) ; |
||||||
|
} |
||||||
|
} |
||||||
|
} |
@ -1,53 +0,0 @@ |
|||||||
package com.hnac.hzims.alarm.mqtt; |
|
||||||
|
|
||||||
import com.hnac.hzinfo.subscribe.AnalysisSubscribe; |
|
||||||
import com.hnac.hzinfo.subscribe.MqttConfig; |
|
||||||
import org.eclipse.paho.client.mqttv3.MqttException; |
|
||||||
import org.springframework.beans.factory.annotation.Autowired; |
|
||||||
import org.springframework.beans.factory.annotation.Value; |
|
||||||
import org.springframework.stereotype.Component; |
|
||||||
|
|
||||||
import javax.annotation.PostConstruct; |
|
||||||
import java.util.Collections; |
|
||||||
|
|
||||||
/** |
|
||||||
* @Author: ty |
|
||||||
*/ |
|
||||||
|
|
||||||
@Component |
|
||||||
public class fdpMqttConfig { |
|
||||||
|
|
||||||
@Autowired |
|
||||||
fdpMqttConsumer fdpMqttConsumer; |
|
||||||
/*@Value("${hzims.mqtt.fdp-url}") |
|
||||||
private String mqtt_fdp_url; |
|
||||||
@Value("${hzims.mqtt.fdp-group}") |
|
||||||
private String mqtt_fdp_group; |
|
||||||
@Value("${hzims.mqtt.fdp-password}") |
|
||||||
private String mqtt_fdp_password; |
|
||||||
@Value("${hzims.mqtt.fdp-username}") |
|
||||||
private String mqtt_fdp_username; |
|
||||||
@Value("${hzims.mqtt.fdp-topname}") |
|
||||||
private String mqtt_fdp_topname;*/ |
|
||||||
@PostConstruct |
|
||||||
public void registerMqtt(){ |
|
||||||
try { |
|
||||||
MqttConfig mqttConfig = new MqttConfig(); |
|
||||||
mqttConfig.setUrl("tcp://175.6.40.68:1883"); |
|
||||||
mqttConfig.setGroup("hzims-alarm"); |
|
||||||
mqttConfig.setPassword("hz123456"); |
|
||||||
mqttConfig.setUsername("hzinfo"); |
|
||||||
// mqttConfig.setUrl(mqtt_fdp_url);
|
|
||||||
// mqttConfig.setGroup(mqtt_fdp_group);
|
|
||||||
// mqttConfig.setPassword(mqtt_fdp_password);
|
|
||||||
// mqttConfig.setUsername(mqtt_fdp_username);
|
|
||||||
AnalysisSubscribe analysisSubscribe = new AnalysisSubscribe(); |
|
||||||
analysisSubscribe.subscribe(mqttConfig, Collections.singletonList("iot/sync/analytics/#"), fdpMqttConsumer); |
|
||||||
// analysisSubscribe.subscribe(mqttConfig, mqtt_fdp_topname, fdpMqttConsumer);
|
|
||||||
}catch (MqttException e){ |
|
||||||
e.printStackTrace(); |
|
||||||
} |
|
||||||
|
|
||||||
|
|
||||||
} |
|
||||||
} |
|
@ -1,60 +0,0 @@ |
|||||||
package com.hnac.hzims.alarm.ws.condition; |
|
||||||
|
|
||||||
import com.hnac.hzims.alarm.handle.service.ConditionAlarmService; |
|
||||||
import com.hnac.hzims.alarm.ws.level.LevelAlarmWebSocket; |
|
||||||
import lombok.extern.slf4j.Slf4j; |
|
||||||
import org.springblade.core.tool.utils.ObjectUtil; |
|
||||||
import org.springblade.core.tool.utils.StringUtil; |
|
||||||
import org.springframework.beans.factory.annotation.Autowired; |
|
||||||
import org.springframework.beans.factory.annotation.Value; |
|
||||||
import org.springframework.scheduling.annotation.EnableScheduling; |
|
||||||
import org.springframework.scheduling.annotation.Scheduled; |
|
||||||
import org.springframework.stereotype.Component; |
|
||||||
|
|
||||||
import java.net.URI; |
|
||||||
|
|
||||||
/** |
|
||||||
* @author ysj |
|
||||||
*/ |
|
||||||
@Slf4j |
|
||||||
@Component |
|
||||||
@EnableScheduling |
|
||||||
public class ConditionAlarmRegular { |
|
||||||
|
|
||||||
@Autowired |
|
||||||
private ConditionAlarmService conditionService; |
|
||||||
|
|
||||||
private LevelAlarmWebSocket socket; |
|
||||||
|
|
||||||
@Value("${hzims.condition.wss-url}") |
|
||||||
private String condition_wss_url; |
|
||||||
|
|
||||||
// 两分钟进行一次检查链接是否存活,不存活了重新建立链接
|
|
||||||
@Scheduled(cron = "0 0/2 * * * ?") |
|
||||||
private void regular(){ |
|
||||||
// 检查链接存活状态
|
|
||||||
if(ObjectUtil.isEmpty(socket) || !socket.isOpen()){ |
|
||||||
log.error("level websocket survival check : {}","死亡"); |
|
||||||
this.createSocket(); |
|
||||||
if(ObjectUtil.isNotEmpty(socket) && socket.isOpen()){ |
|
||||||
String message = conditionService.message(); |
|
||||||
if(!StringUtil.isEmpty(message)){ |
|
||||||
socket.send(message); |
|
||||||
} |
|
||||||
} |
|
||||||
log.error("conditionAlarm survival check : {}","重新建立链接"); |
|
||||||
}else{ |
|
||||||
log.error("conditionAlarm survival check : {}","存活"); |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// 创建websocket链接
|
|
||||||
private void createSocket() { |
|
||||||
try{ |
|
||||||
socket = new LevelAlarmWebSocket(new URI(condition_wss_url)); |
|
||||||
socket.connectBlocking(); |
|
||||||
}catch (Exception e){ |
|
||||||
log.error("conditionAlarm create error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
@ -1,136 +0,0 @@ |
|||||||
package com.hnac.hzims.alarm.ws.condition; |
|
||||||
|
|
||||||
import com.hnac.hzims.alarm.entity.AlarmEntity; |
|
||||||
import com.hnac.hzims.alarm.handle.service.ConditionAlarmService; |
|
||||||
import com.hnac.hzims.alarm.show.service.AlarmService; |
|
||||||
import lombok.extern.slf4j.Slf4j; |
|
||||||
import org.java_websocket.client.WebSocketClient; |
|
||||||
import org.java_websocket.handshake.ServerHandshake; |
|
||||||
import org.springblade.core.log.exception.ServiceException; |
|
||||||
import org.springblade.core.tool.utils.SpringUtil; |
|
||||||
import org.springblade.core.tool.utils.StringUtil; |
|
||||||
|
|
||||||
import javax.net.ssl.SSLContext; |
|
||||||
import javax.net.ssl.TrustManager; |
|
||||||
import javax.net.ssl.X509TrustManager; |
|
||||||
import java.net.Socket; |
|
||||||
import java.net.URI; |
|
||||||
import java.security.SecureRandom; |
|
||||||
import java.security.cert.X509Certificate; |
|
||||||
import java.util.List; |
|
||||||
import java.util.Optional; |
|
||||||
|
|
||||||
/** |
|
||||||
* @author ysj |
|
||||||
*/ |
|
||||||
@Slf4j |
|
||||||
public class ConditionAlarmWebSocket extends WebSocketClient { |
|
||||||
|
|
||||||
|
|
||||||
private final ConditionAlarmService conditionService; |
|
||||||
private AlarmService alarmService; |
|
||||||
|
|
||||||
/** |
|
||||||
* 构造等级告警websocket |
|
||||||
* @param uri |
|
||||||
*/ |
|
||||||
public ConditionAlarmWebSocket(URI uri) { |
|
||||||
super(uri); |
|
||||||
conditionService = SpringUtil.getBean(ConditionAlarmService.class); |
|
||||||
connection(this); |
|
||||||
} |
|
||||||
|
|
||||||
// 链接到服务器回调接口
|
|
||||||
@Override |
|
||||||
public void onOpen(ServerHandshake handshakedata) { |
|
||||||
log.error("conditionAlarm websocket open"); |
|
||||||
} |
|
||||||
|
|
||||||
// 接收到服务器消息回调接口
|
|
||||||
@Override |
|
||||||
public void onMessage(String message) { |
|
||||||
if(StringUtil.isEmpty(message)){ |
|
||||||
log.error("conditionAlarm on message is null"); |
|
||||||
}else{ |
|
||||||
// 告警数据转化
|
|
||||||
List<AlarmEntity> alarmEntities = conditionService.receiveMessage(message); |
|
||||||
// 等级告警数据处理
|
|
||||||
try { |
|
||||||
alarmService.dealAlarmEntities(alarmEntities); |
|
||||||
}catch (Exception e){ |
|
||||||
throw new ServiceException("集中监控告警数据处理报错(conditionAlarm):"+e); |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// 与服务器链接中断回调接口
|
|
||||||
@Override |
|
||||||
public void onClose(int code, String reason, boolean remote) { |
|
||||||
log.error("conditionAlarm websocket close"); |
|
||||||
} |
|
||||||
|
|
||||||
// 与服务器通讯异常触发
|
|
||||||
@Override |
|
||||||
public void onError(Exception e) { |
|
||||||
log.error("conditionAlarm websocket error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* 建立链接 |
|
||||||
* @param webSocket |
|
||||||
*/ |
|
||||||
private void connection(ConditionAlarmWebSocket webSocket) { |
|
||||||
SSLContext context = init(); |
|
||||||
if(Optional.ofNullable(context).isPresent()){ |
|
||||||
Socket socket = create(context); |
|
||||||
if(Optional.ofNullable(socket).isPresent()){ |
|
||||||
webSocket.setSocket(socket); |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* 创建Socket |
|
||||||
* @param context |
|
||||||
* @return |
|
||||||
*/ |
|
||||||
private Socket create(SSLContext context) { |
|
||||||
Socket socket = null; |
|
||||||
try{ |
|
||||||
socket = context.getSocketFactory().createSocket(); |
|
||||||
}catch (Exception e){ |
|
||||||
log.error("conditionAlarm socket create error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
return socket; |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* 协议初始化 |
|
||||||
* @return SSLContext |
|
||||||
*/ |
|
||||||
private SSLContext init() { |
|
||||||
SSLContext SSL = null; |
|
||||||
try{ |
|
||||||
SSL = SSLContext.getInstance("TLS"); |
|
||||||
SSL.init(null, new TrustManager[]{new X509TrustManager() { |
|
||||||
@Override |
|
||||||
public void checkClientTrusted(X509Certificate[] chain, |
|
||||||
String authType) { |
|
||||||
} |
|
||||||
|
|
||||||
@Override |
|
||||||
public void checkServerTrusted(X509Certificate[] chain, String authType) { |
|
||||||
} |
|
||||||
|
|
||||||
@Override |
|
||||||
public X509Certificate[] getAcceptedIssuers() { |
|
||||||
return new X509Certificate[0]; |
|
||||||
} |
|
||||||
}}, new SecureRandom()); |
|
||||||
}catch (Exception e){ |
|
||||||
log.error("conditionAlarm SSL init error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
return SSL; |
|
||||||
} |
|
||||||
|
|
||||||
} |
|
@ -1,56 +0,0 @@ |
|||||||
package com.hnac.hzims.alarm.ws.fdp; |
|
||||||
|
|
||||||
import com.hnac.hzims.alarm.handle.service.FdpAlarmService; |
|
||||||
import lombok.extern.slf4j.Slf4j; |
|
||||||
import org.springblade.core.tool.utils.ObjectUtil; |
|
||||||
import org.springframework.beans.factory.annotation.Autowired; |
|
||||||
import org.springframework.beans.factory.annotation.Value; |
|
||||||
import org.springframework.scheduling.annotation.EnableScheduling; |
|
||||||
import org.springframework.scheduling.annotation.Scheduled; |
|
||||||
import org.springframework.stereotype.Component; |
|
||||||
|
|
||||||
import java.net.URI; |
|
||||||
|
|
||||||
/** |
|
||||||
* 等级告警获取数据长链接 |
|
||||||
* @author ty |
|
||||||
*/ |
|
||||||
@Slf4j |
|
||||||
@Component |
|
||||||
@EnableScheduling |
|
||||||
public class FdpAlarmRegular { |
|
||||||
|
|
||||||
@Value("${hzims.fdp.alarm-url}") |
|
||||||
private String fdp_alarm_url; |
|
||||||
|
|
||||||
private FdpAlarmWebSocket client; |
|
||||||
|
|
||||||
@Autowired |
|
||||||
private FdpAlarmService fdpAlarmService; |
|
||||||
|
|
||||||
// 定时发送消息
|
|
||||||
@Scheduled(cron = "0 0/30 * * * ?") |
|
||||||
private void regular(){ |
|
||||||
System.out.println("系统告警链接检查"); |
|
||||||
// 检查链接存活状态
|
|
||||||
if(ObjectUtil.isNotEmpty(client) && client.isOpen()){ |
|
||||||
client.send(fdpAlarmService.sendMessage()); |
|
||||||
System.out.println("FdpAlarm:链接成功"); |
|
||||||
}else { |
|
||||||
System.out.println("FdpAlarm:链接失败"); |
|
||||||
this.createClient(); |
|
||||||
System.out.println("FdpAlarm:重新链接"); |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// 创建websocket链接
|
|
||||||
private void createClient() { |
|
||||||
try{ |
|
||||||
client = new FdpAlarmWebSocket(new URI(fdp_alarm_url)); |
|
||||||
client.connectBlocking(); |
|
||||||
System.out.println("FdpAlarm create success"); |
|
||||||
}catch (Exception e){ |
|
||||||
log.error("FdpAlarm create error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
@ -1,138 +0,0 @@ |
|||||||
package com.hnac.hzims.alarm.ws.fdp; |
|
||||||
|
|
||||||
import com.hnac.hzims.alarm.entity.AlarmEntity; |
|
||||||
import com.hnac.hzims.alarm.handle.service.FdpAlarmService; |
|
||||||
import com.hnac.hzims.alarm.show.service.AlarmService; |
|
||||||
import lombok.extern.slf4j.Slf4j; |
|
||||||
import org.java_websocket.client.WebSocketClient; |
|
||||||
import org.java_websocket.handshake.ServerHandshake; |
|
||||||
import org.springblade.core.log.exception.ServiceException; |
|
||||||
import org.springblade.core.tool.utils.SpringUtil; |
|
||||||
import org.springblade.core.tool.utils.StringUtil; |
|
||||||
import org.springframework.beans.factory.annotation.Autowired; |
|
||||||
|
|
||||||
import javax.net.ssl.SSLContext; |
|
||||||
import javax.net.ssl.TrustManager; |
|
||||||
import javax.net.ssl.X509TrustManager; |
|
||||||
import java.net.Socket; |
|
||||||
import java.net.URI; |
|
||||||
import java.security.SecureRandom; |
|
||||||
import java.security.cert.X509Certificate; |
|
||||||
import java.util.List; |
|
||||||
import java.util.Optional; |
|
||||||
|
|
||||||
/** |
|
||||||
* 等级告警获取数据长链接 |
|
||||||
* @author ty |
|
||||||
*/ |
|
||||||
@Slf4j |
|
||||||
public class FdpAlarmWebSocket extends WebSocketClient { |
|
||||||
@Autowired |
|
||||||
private FdpAlarmService fdpAlarmService; |
|
||||||
private AlarmService alarmService; |
|
||||||
|
|
||||||
/** |
|
||||||
* 构造等级告警websocket |
|
||||||
* @param uri |
|
||||||
*/ |
|
||||||
public FdpAlarmWebSocket(URI uri) { |
|
||||||
super(uri); |
|
||||||
fdpAlarmService = SpringUtil.getBean(FdpAlarmService.class); |
|
||||||
connection(this); |
|
||||||
} |
|
||||||
|
|
||||||
// 链接到服务器回调接口
|
|
||||||
@Override |
|
||||||
public void onOpen(ServerHandshake handshakedata) { |
|
||||||
log.error("FdpAlarm websocket open"); |
|
||||||
} |
|
||||||
|
|
||||||
// 接收到服务器消息回调接口
|
|
||||||
@Override |
|
||||||
public void onMessage(String message) { |
|
||||||
if(StringUtil.isEmpty(message)){ |
|
||||||
log.error("FdpAlarm on message is null"); |
|
||||||
}else{ |
|
||||||
// 告警数据转化
|
|
||||||
List<AlarmEntity> alarmEntities = fdpAlarmService.receiveMessage(message); |
|
||||||
//统一数据处理
|
|
||||||
try { |
|
||||||
//websocket 消息推送保存
|
|
||||||
alarmService.dealAlarmEntities(alarmEntities); |
|
||||||
}catch (Exception e){ |
|
||||||
throw new ServiceException("集中监控告警数据处理报错(FdpAlarm):"+e); |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// 与服务器链接中断回调接口
|
|
||||||
@Override |
|
||||||
public void onClose(int code, String reason, boolean remote) { |
|
||||||
log.error("FdpAlarm websocket close"); |
|
||||||
} |
|
||||||
|
|
||||||
// 与服务器通讯异常触发
|
|
||||||
@Override |
|
||||||
public void onError(Exception e) { |
|
||||||
log.error("FdpAlarm websocket error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* 建立链接 |
|
||||||
* @param webSocket |
|
||||||
*/ |
|
||||||
private void connection(FdpAlarmWebSocket webSocket) { |
|
||||||
SSLContext context = init(); |
|
||||||
if(Optional.ofNullable(context).isPresent()){ |
|
||||||
Socket socket = create(context); |
|
||||||
if(Optional.ofNullable(socket).isPresent()){ |
|
||||||
webSocket.setSocket(socket); |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* 创建Socket |
|
||||||
* @param context |
|
||||||
* @return |
|
||||||
*/ |
|
||||||
private Socket create(SSLContext context) { |
|
||||||
Socket socket = null; |
|
||||||
try{ |
|
||||||
socket = context.getSocketFactory().createSocket(); |
|
||||||
}catch (Exception e){ |
|
||||||
log.error("FdpAlarm socket create error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
return socket; |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* 协议初始化 |
|
||||||
* @return SSLContext |
|
||||||
*/ |
|
||||||
private SSLContext init() { |
|
||||||
SSLContext SSL = null; |
|
||||||
try{ |
|
||||||
SSL = SSLContext.getInstance("TLS"); |
|
||||||
SSL.init(null, new TrustManager[]{new X509TrustManager() { |
|
||||||
@Override |
|
||||||
public void checkClientTrusted(X509Certificate[] chain, |
|
||||||
String authType) { |
|
||||||
} |
|
||||||
|
|
||||||
@Override |
|
||||||
public void checkServerTrusted(X509Certificate[] chain, String authType) { |
|
||||||
} |
|
||||||
|
|
||||||
@Override |
|
||||||
public X509Certificate[] getAcceptedIssuers() { |
|
||||||
return new X509Certificate[0]; |
|
||||||
} |
|
||||||
}}, new SecureRandom()); |
|
||||||
}catch (Exception e){ |
|
||||||
log.error("FdpAlarm SSL init error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
return SSL; |
|
||||||
} |
|
||||||
|
|
||||||
} |
|
@ -1,60 +0,0 @@ |
|||||||
package com.hnac.hzims.alarm.ws.hz3000; |
|
||||||
|
|
||||||
import com.hnac.hzims.alarm.handle.service.SystemAlarmService; |
|
||||||
import lombok.extern.slf4j.Slf4j; |
|
||||||
import org.springblade.core.tool.utils.ObjectUtil; |
|
||||||
import org.springframework.beans.factory.annotation.Autowired; |
|
||||||
import org.springframework.beans.factory.annotation.Value; |
|
||||||
import org.springframework.scheduling.annotation.EnableScheduling; |
|
||||||
import org.springframework.scheduling.annotation.Scheduled; |
|
||||||
import org.springframework.stereotype.Component; |
|
||||||
|
|
||||||
import java.net.URI; |
|
||||||
|
|
||||||
/** |
|
||||||
* 等级告警获取数据长链接 |
|
||||||
* @author ty |
|
||||||
*/ |
|
||||||
@Slf4j |
|
||||||
@Component |
|
||||||
@EnableScheduling |
|
||||||
public class SystemAlarmRegular { |
|
||||||
|
|
||||||
@Value("${hzims.system.wss-url}") |
|
||||||
private String system_wss_url; |
|
||||||
|
|
||||||
private SystemAlarmWebSocket client; |
|
||||||
|
|
||||||
@Autowired |
|
||||||
private SystemAlarmService systemAlarmService; |
|
||||||
|
|
||||||
int count=0; |
|
||||||
// 定时发送消息
|
|
||||||
@Scheduled(cron = "0 0/30 * * * ?") |
|
||||||
private void regular(){ |
|
||||||
// 检查链接存活状态
|
|
||||||
if(ObjectUtil.isNotEmpty(client) && client.isOpen()){ |
|
||||||
client.send(systemAlarmService.sendMessage()); |
|
||||||
System.out.println("systemAlarm:存活"); |
|
||||||
count=0; |
|
||||||
}else { |
|
||||||
System.out.println("systemAlarm:链接失败"); |
|
||||||
this.createClient(); |
|
||||||
count++; |
|
||||||
if (count>=3){ |
|
||||||
|
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// 创建websocket链接
|
|
||||||
private void createClient() { |
|
||||||
try{ |
|
||||||
client = new SystemAlarmWebSocket(new URI(system_wss_url)); |
|
||||||
client.connectBlocking(); |
|
||||||
System.out.println("systemAlarm create success"); |
|
||||||
}catch (Exception e){ |
|
||||||
log.error("systemAlarm create error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
} |
|
||||||
} |
|
@ -1,142 +0,0 @@ |
|||||||
package com.hnac.hzims.alarm.ws.hz3000; |
|
||||||
|
|
||||||
import com.hnac.hzims.alarm.entity.AlarmEntity; |
|
||||||
import com.hnac.hzims.alarm.handle.service.SystemAlarmService; |
|
||||||
import com.hnac.hzims.alarm.show.service.AlarmService; |
|
||||||
import lombok.extern.slf4j.Slf4j; |
|
||||||
import org.java_websocket.client.WebSocketClient; |
|
||||||
import org.java_websocket.handshake.ServerHandshake; |
|
||||||
import org.springblade.core.log.exception.ServiceException; |
|
||||||
import org.springblade.core.tool.utils.SpringUtil; |
|
||||||
import org.springblade.core.tool.utils.StringUtil; |
|
||||||
|
|
||||||
import javax.net.ssl.SSLContext; |
|
||||||
import javax.net.ssl.TrustManager; |
|
||||||
import javax.net.ssl.X509TrustManager; |
|
||||||
import java.net.Socket; |
|
||||||
import java.net.URI; |
|
||||||
import java.security.SecureRandom; |
|
||||||
import java.security.cert.X509Certificate; |
|
||||||
import java.util.List; |
|
||||||
import java.util.Optional; |
|
||||||
|
|
||||||
/** |
|
||||||
* 等级告警获取数据长链接 |
|
||||||
* @author ty |
|
||||||
*/ |
|
||||||
@Slf4j |
|
||||||
public class SystemAlarmWebSocket extends WebSocketClient { |
|
||||||
|
|
||||||
private final SystemAlarmService systemAlarmService; |
|
||||||
|
|
||||||
private AlarmService alarmService; |
|
||||||
|
|
||||||
/** |
|
||||||
* 构造等级告警websocket |
|
||||||
* @param uri |
|
||||||
*/ |
|
||||||
public SystemAlarmWebSocket(URI uri) { |
|
||||||
super(uri); |
|
||||||
systemAlarmService = SpringUtil.getBean(SystemAlarmService.class); |
|
||||||
connection(this); |
|
||||||
} |
|
||||||
|
|
||||||
// 链接到服务器回调接口
|
|
||||||
@Override |
|
||||||
public void onOpen(ServerHandshake handshakedata) { |
|
||||||
log.error("SystemAlarmWebSocket链接到服务器回调接口"); |
|
||||||
} |
|
||||||
|
|
||||||
// 接收到服务器消息回调接口
|
|
||||||
@Override |
|
||||||
public void onMessage(String message) { |
|
||||||
if(StringUtil.isEmpty(message)){ |
|
||||||
log.error("systemAlarm on message is null"); |
|
||||||
}else{ |
|
||||||
// 华自3000告警数据转化
|
|
||||||
List<AlarmEntity> alarmEntities = systemAlarmService.receiveMessage(message); |
|
||||||
//统一数据处理
|
|
||||||
try { |
|
||||||
//websocket 消息推送保存
|
|
||||||
alarmService.dealAlarmEntities(alarmEntities); |
|
||||||
}catch (Exception e){ |
|
||||||
throw new ServiceException("集中监控告警数据处理报错:"+e); |
|
||||||
} |
|
||||||
log.error("华自3000告警数据处理"); |
|
||||||
} |
|
||||||
} |
|
||||||
|
|
||||||
// 与服务器链接中断回调接口
|
|
||||||
@Override |
|
||||||
public void onClose(int code, String reason, boolean remote) { |
|
||||||
log.error("SystemAlarmWebSocket与服务器链接中断回调接口 "); |
|
||||||
} |
|
||||||
|
|
||||||
// 与服务器通讯异常触发
|
|
||||||
@Override |
|
||||||
public void onError(Exception e) { |
|
||||||
log.error("systemAlarm websocket error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* 建立链接 |
|
||||||
* @param webSocket |
|
||||||
*/ |
|
||||||
private void connection(SystemAlarmWebSocket webSocket) { |
|
||||||
SSLContext context = init(); |
|
||||||
if(Optional.ofNullable(context).isPresent()){ |
|
||||||
Socket socket = create(context); |
|
||||||
if(Optional.ofNullable(socket).isPresent()){ |
|
||||||
webSocket.setSocket(socket); |
|
||||||
} |
|
||||||
} |
|
||||||
System.out.println("systemAlarm create success"); |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* 创建Socket |
|
||||||
* @param context |
|
||||||
* @return |
|
||||||
*/ |
|
||||||
private Socket create(SSLContext context) { |
|
||||||
Socket socket = null; |
|
||||||
try{ |
|
||||||
socket = context.getSocketFactory().createSocket(); |
|
||||||
log.error("SystemAlarmWebSocket链接创建成功"); |
|
||||||
}catch (Exception e){ |
|
||||||
log.error("systemAlarm socket create error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
return socket; |
|
||||||
} |
|
||||||
|
|
||||||
/** |
|
||||||
* 协议初始化 |
|
||||||
* @return SSLContext |
|
||||||
*/ |
|
||||||
private SSLContext init() { |
|
||||||
SSLContext SSL = null; |
|
||||||
try{ |
|
||||||
SSL = SSLContext.getInstance("TLS"); |
|
||||||
SSL.init(null, new TrustManager[]{new X509TrustManager() { |
|
||||||
@Override |
|
||||||
public void checkClientTrusted(X509Certificate[] chain, |
|
||||||
String authType) { |
|
||||||
} |
|
||||||
|
|
||||||
@Override |
|
||||||
public void checkServerTrusted(X509Certificate[] chain, String authType) { |
|
||||||
} |
|
||||||
|
|
||||||
@Override |
|
||||||
public X509Certificate[] getAcceptedIssuers() { |
|
||||||
return new X509Certificate[0]; |
|
||||||
} |
|
||||||
}}, new SecureRandom()); |
|
||||||
log.error("SystemAlarmWebSocket初始化成功"); |
|
||||||
}catch (Exception e){ |
|
||||||
log.error("systemAlarm SSL init error : {}",e.getMessage()); |
|
||||||
} |
|
||||||
return SSL; |
|
||||||
} |
|
||||||
|
|
||||||
} |
|
Loading…
Reference in new issue