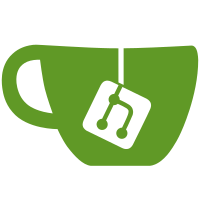
29 changed files with 977 additions and 98 deletions
@ -0,0 +1,30 @@ |
|||||||
|
package com.hnac.hzims.operational.maintenance.feign; |
||||||
|
|
||||||
|
import com.hnac.hzims.operational.OperationalConstants; |
||||||
|
import com.hnac.hzims.operational.maintenance.feign.fallback.MaintenanceFeignClientFallback; |
||||||
|
import com.hnac.hzims.operational.maintenance.vo.ProcessWorkFlowResponse; |
||||||
|
import io.swagger.annotations.ApiOperation; |
||||||
|
import org.springframework.cloud.openfeign.FeignClient; |
||||||
|
import org.springframework.stereotype.Repository; |
||||||
|
import org.springframework.web.bind.annotation.PostMapping; |
||||||
|
import org.springframework.web.bind.annotation.RequestBody; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/14 15:12 |
||||||
|
*/ |
||||||
|
@FeignClient( |
||||||
|
value = OperationalConstants.APP_NAME, |
||||||
|
fallback = MaintenanceFeignClientFallback.class |
||||||
|
) |
||||||
|
@Repository |
||||||
|
public interface MaintenanceFeignClient { |
||||||
|
|
||||||
|
|
||||||
|
@PostMapping("/maintenance/findPending") |
||||||
|
@ApiOperation(value = "日常维护待处理") |
||||||
|
public Boolean findPending(@RequestBody ProcessWorkFlowResponse response); |
||||||
|
|
||||||
|
} |
@ -0,0 +1,19 @@ |
|||||||
|
package com.hnac.hzims.operational.maintenance.feign.fallback; |
||||||
|
|
||||||
|
import com.hnac.hzims.operational.maintenance.feign.MaintenanceFeignClient; |
||||||
|
import com.hnac.hzims.operational.maintenance.vo.ProcessWorkFlowResponse; |
||||||
|
import org.springframework.stereotype.Component; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/14 15:13 |
||||||
|
*/ |
||||||
|
@Component |
||||||
|
public class MaintenanceFeignClientFallback implements MaintenanceFeignClient { |
||||||
|
@Override |
||||||
|
public Boolean findPending(ProcessWorkFlowResponse response) { |
||||||
|
throw new IllegalArgumentException("日常维护流程出现异常呢"); |
||||||
|
} |
||||||
|
} |
@ -1,4 +1,4 @@ |
|||||||
package com.hnac.hzims.ticket.response; |
package com.hnac.hzims.operational.maintenance.vo; |
||||||
|
|
||||||
import lombok.Data; |
import lombok.Data; |
||||||
import lombok.EqualsAndHashCode; |
import lombok.EqualsAndHashCode; |
@ -0,0 +1,66 @@ |
|||||||
|
package com.hnac.hzims.operational.maintenance.controller; |
||||||
|
|
||||||
|
import com.github.xiaoymin.knife4j.annotations.ApiOperationSupport; |
||||||
|
import com.hnac.hzims.operational.maintenance.service.MaintenanceService; |
||||||
|
import com.hnac.hzims.operational.maintenance.vo.ProcessWorkFlowResponse; |
||||||
|
import io.swagger.annotations.ApiOperation; |
||||||
|
import io.swagger.annotations.ApiParam; |
||||||
|
import lombok.RequiredArgsConstructor; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springblade.core.log.exception.ServiceException; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* 日常维护控制层 |
||||||
|
* |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/14 8:08 |
||||||
|
*/ |
||||||
|
@Slf4j |
||||||
|
@RequiredArgsConstructor |
||||||
|
@RestController |
||||||
|
@RequestMapping("/maintenance") |
||||||
|
public class MaintenanceController { |
||||||
|
|
||||||
|
|
||||||
|
private final MaintenanceService maintenanceService; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 日常维护生成任务 |
||||||
|
* @param ids |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@PostMapping("/createTask") |
||||||
|
@ApiOperationSupport(order = 60) |
||||||
|
@ApiOperation(value = "根据计划ID生成任务") |
||||||
|
public R generateTask(@ApiParam(value = "计划ID", required = true) @RequestBody List<String> ids) { |
||||||
|
maintenanceService.createTask(ids); |
||||||
|
return R.success("日常维护生成任务成功"); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 日常维护待处理 |
||||||
|
* @param response |
||||||
|
*/ |
||||||
|
@PostMapping("/findPending") |
||||||
|
@ApiOperation(value = "日常维护待处理") |
||||||
|
public Boolean findPending(@RequestBody ProcessWorkFlowResponse response){ |
||||||
|
try { |
||||||
|
maintenanceService.findPending(response); |
||||||
|
return true; |
||||||
|
} catch (Exception e) { |
||||||
|
throw new ServiceException("日常维护待处理:" + e.getMessage()); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,29 @@ |
|||||||
|
package com.hnac.hzims.operational.maintenance.service; |
||||||
|
|
||||||
|
|
||||||
|
import com.hnac.hzims.operational.maintenance.vo.ProcessWorkFlowResponse; |
||||||
|
|
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/14 8:24 |
||||||
|
*/ |
||||||
|
public interface MaintenanceService { |
||||||
|
|
||||||
|
/** |
||||||
|
* 日常维护生成任务 |
||||||
|
* @param ids |
||||||
|
*/ |
||||||
|
|
||||||
|
void createTask(List<String> ids); |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 日常维护待处理 |
||||||
|
* @param response |
||||||
|
*/ |
||||||
|
void findPending(ProcessWorkFlowResponse response); |
||||||
|
} |
@ -0,0 +1,343 @@ |
|||||||
|
package com.hnac.hzims.operational.maintenance.service.impl; |
||||||
|
|
||||||
|
import com.alibaba.fastjson.JSON; |
||||||
|
import com.alibaba.fastjson.JSONObject; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.query.LambdaQueryWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.conditions.update.LambdaUpdateWrapper; |
||||||
|
import com.baomidou.mybatisplus.core.toolkit.ObjectUtils; |
||||||
|
import com.hnac.hzims.common.utils.DateUtil; |
||||||
|
import com.hnac.hzims.message.MessageConstants; |
||||||
|
import com.hnac.hzims.message.dto.MessagePushRecordDto; |
||||||
|
import com.hnac.hzims.message.dto.PlanMsgRecordDto; |
||||||
|
import com.hnac.hzims.message.entity.config.MessageTemplateEntity; |
||||||
|
import com.hnac.hzims.message.fegin.IMessageClient; |
||||||
|
import com.hnac.hzims.operational.duty.entity.ImsDutyMainEntity; |
||||||
|
import com.hnac.hzims.operational.duty.service.IImsDutyMainService; |
||||||
|
import com.hnac.hzims.operational.maintenance.entity.OperMaintenancePlanEntity; |
||||||
|
import com.hnac.hzims.operational.maintenance.entity.OperMaintenanceTaskEntity; |
||||||
|
import com.hnac.hzims.operational.maintenance.service.IOperMaintenancePlanService; |
||||||
|
import com.hnac.hzims.operational.maintenance.service.IOperMaintenanceTaskService; |
||||||
|
import com.hnac.hzims.operational.maintenance.service.MaintenanceService; |
||||||
|
import com.hnac.hzims.operational.maintenance.template.service.TemplateService; |
||||||
|
import com.hnac.hzims.operational.maintenance.vo.OperMaintenanceTaskEntityVo; |
||||||
|
import com.hnac.hzims.operational.util.StringObjUtils; |
||||||
|
import com.hnac.hzims.operational.maintenance.vo.ProcessWorkFlowResponse; |
||||||
|
import lombok.RequiredArgsConstructor; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.apache.commons.lang.StringUtils; |
||||||
|
import org.apache.commons.lang3.math.NumberUtils; |
||||||
|
import org.springblade.core.log.exception.ServiceException; |
||||||
|
import org.springblade.core.mp.base.BaseEntity; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springblade.core.tool.utils.*; |
||||||
|
import org.springblade.flow.core.feign.IFlowClient; |
||||||
|
import org.springblade.system.feign.ISysClient; |
||||||
|
import org.springblade.system.user.cache.UserCache; |
||||||
|
import org.springblade.system.user.entity.User; |
||||||
|
import org.springframework.beans.BeanUtils; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
import org.springframework.transaction.annotation.Transactional; |
||||||
|
|
||||||
|
import java.time.Instant; |
||||||
|
import java.time.LocalDate; |
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.time.ZoneId; |
||||||
|
import java.time.format.DateTimeFormatter; |
||||||
|
import java.util.*; |
||||||
|
import java.util.stream.Collectors; |
||||||
|
import java.util.stream.Stream; |
||||||
|
|
||||||
|
import static org.springblade.core.tool.utils.DateUtil.PATTERN_DATE; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/14 8:24 |
||||||
|
*/ |
||||||
|
@Slf4j |
||||||
|
@Service |
||||||
|
@RequiredArgsConstructor |
||||||
|
public class MaintenanceServiceImpl implements MaintenanceService { |
||||||
|
|
||||||
|
|
||||||
|
private final IOperMaintenancePlanService maintenancePlanService; |
||||||
|
private final IImsDutyMainService mainService; |
||||||
|
private final IOperMaintenanceTaskService taskService; |
||||||
|
private final IMessageClient messageClient; |
||||||
|
private final List<TemplateService> templateService; |
||||||
|
private final IFlowClient flowClient; |
||||||
|
private final ISysClient sysClient; |
||||||
|
|
||||||
|
/** |
||||||
|
* 日常维护生成任务 |
||||||
|
* |
||||||
|
* @param ids |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
@Transactional(rollbackFor = Exception.class) |
||||||
|
public void createTask(List<String> ids) { |
||||||
|
//获取日常维护计划
|
||||||
|
LambdaQueryWrapper<OperMaintenancePlanEntity> planEntityLambdaQueryWrapper = new LambdaQueryWrapper<>(); |
||||||
|
planEntityLambdaQueryWrapper.in(BaseEntity::getId, ids); |
||||||
|
List<OperMaintenancePlanEntity> planEntities = maintenancePlanService |
||||||
|
.list(planEntityLambdaQueryWrapper); |
||||||
|
//筛选当月没生成任务的计划
|
||||||
|
List<OperMaintenancePlanEntity> finalPlanEntities = planEntities.stream().filter(planEntity -> |
||||||
|
ObjectUtil.isEmpty(planEntity.getCreateTaskTime()) |
||||||
|
|| !DateUtil.judgeSameDay( |
||||||
|
DateUtil.DateToLocalDateTime(planEntity.getCreateTaskTime()), LocalDate.now()) |
||||||
|
).collect(Collectors.toList()); |
||||||
|
if (CollectionUtil.isEmpty(finalPlanEntities)) { |
||||||
|
throw new ServiceException("所选计划当天已生成任务"); |
||||||
|
} |
||||||
|
for (OperMaintenancePlanEntity finalPlanEntity : finalPlanEntities) { |
||||||
|
// 通过计划生成任务
|
||||||
|
this.createTaskByPlan(finalPlanEntity); |
||||||
|
//更新任务派发时间
|
||||||
|
LambdaUpdateWrapper<OperMaintenancePlanEntity> planEntityLambdaUpdateWrapper = |
||||||
|
new LambdaUpdateWrapper<>(); |
||||||
|
planEntityLambdaUpdateWrapper.set(OperMaintenancePlanEntity::getCreateTaskTime, new Date()); |
||||||
|
planEntityLambdaUpdateWrapper.eq(OperMaintenancePlanEntity::getId, finalPlanEntity.getId()); |
||||||
|
boolean update = maintenancePlanService.update(planEntityLambdaUpdateWrapper); |
||||||
|
if (!update) { |
||||||
|
log.error("maintenance:generateTask 更新任务派发失败"); |
||||||
|
throw new ServiceException("更新任务派发失败"); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 日常维护待处理 |
||||||
|
* |
||||||
|
* @param response |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public void findPending(ProcessWorkFlowResponse response) { |
||||||
|
log.info("获取businessKey: {}", response.getBusinessKey()); |
||||||
|
log.info("获取taskId: {} ", response.getTaskId()); |
||||||
|
log.info("获取下一个审批人是: {} ", response.getNextStepOperator()); |
||||||
|
log.info("获取下一个用户Id是: {} ", response.getUserId()); |
||||||
|
log.info("获取当前任务名称是: {} ", response.getTaskName()); |
||||||
|
log.info("获取根据handleType区分是用户还是候选组角色: {}", response.getHandleType()); |
||||||
|
//json转换表单
|
||||||
|
String formData = JSON.toJSONString(response.getVariables()); |
||||||
|
log.info("获取表单的数据:{}", formData); |
||||||
|
OperMaintenanceTaskEntityVo standardTicketInfoVo = null; |
||||||
|
try { |
||||||
|
JSONObject jsonObject = JSONObject.parseObject(formData); |
||||||
|
standardTicketInfoVo = JSONObject.toJavaObject(jsonObject, OperMaintenanceTaskEntityVo.class); |
||||||
|
} catch (Exception e) { |
||||||
|
log.error("获取表单出现异常了~~~~"); |
||||||
|
throw new IllegalArgumentException(e.getMessage()); |
||||||
|
} |
||||||
|
//1.查询日常维护信息
|
||||||
|
Long id = NumberUtils.toLong(response.getBusinessKey()); |
||||||
|
OperMaintenanceTaskEntity dbOperMaintenanceTaskEntity = taskService.getById(id); |
||||||
|
if (ObjectUtils.isEmpty(dbOperMaintenanceTaskEntity)) { |
||||||
|
log.error("获取日常维护数据不存在"); |
||||||
|
return; |
||||||
|
} |
||||||
|
OperMaintenanceTaskEntity entity = new OperMaintenanceTaskEntity(); |
||||||
|
BeanUtils.copyProperties(standardTicketInfoVo,entity); |
||||||
|
entity.setId(id); |
||||||
|
//填充日常维护信息
|
||||||
|
saveOperMaintenanceTaskEntity(entity, response); |
||||||
|
entity.setProcessInstanceId(response.getProcessInstanceId()); |
||||||
|
taskService.updateById(entity); |
||||||
|
|
||||||
|
//推送消息
|
||||||
|
if (response.getTaskId() != null) { |
||||||
|
MessagePushRecordDto message = new MessagePushRecordDto(); |
||||||
|
message.setBusinessClassify("business"); |
||||||
|
message.setBusinessKey(MessageConstants.BusinessClassifyEnum.OPERATIONTICKETMESSAGE.getKey()); |
||||||
|
message.setSubject(MessageConstants.BusinessClassifyEnum.OPERATIONTICKETMESSAGE.getDescription()); |
||||||
|
message.setTaskId(entity.getId()); |
||||||
|
message.setTenantId("200000"); |
||||||
|
message.setTypes(Arrays.asList(MessageConstants.APP_PUSH, MessageConstants.WS_PUSH)); |
||||||
|
message.setPushType(MessageConstants.IMMEDIATELY); |
||||||
|
//您有一张工作票待审批,工作内容:*****,审批环节:*****;
|
||||||
|
String countent = |
||||||
|
"您有一条日常维护任务待审批,工作内容:".concat(entity.getTitle()) |
||||||
|
.concat(",审批环节:") |
||||||
|
.concat(response.getTaskName()); |
||||||
|
message.setContent(countent); |
||||||
|
message.setDeptId(entity.getCreateDept()); |
||||||
|
R<String> deptName = sysClient.getDeptName(entity.getCreateDept()); |
||||||
|
if (deptName.isSuccess()) { |
||||||
|
message.setDeptName(deptName.getData()); |
||||||
|
} |
||||||
|
String userIds = response.getUserId(); |
||||||
|
if (com.hnac.hzims.common.logs.utils.StringUtils.isBlank(userIds)) { |
||||||
|
log.error("推送的消息不能为空哦,{}", userIds); |
||||||
|
return; |
||||||
|
} |
||||||
|
String[] split = userIds.split(","); |
||||||
|
for (String userId : split) { |
||||||
|
message.setPusher(userId); |
||||||
|
User user = UserCache.getUser(NumberUtils.toLong(userId)); |
||||||
|
if (ObjectUtils.isNotEmpty(user)) { |
||||||
|
message.setPusherName(user.getName()); |
||||||
|
} |
||||||
|
message.setAccount(userId); |
||||||
|
message.setCreateUser(NumberUtils.toLong(userId)); |
||||||
|
messageClient.sendMessage(message); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 填充日常维护信息 |
||||||
|
* @param entity |
||||||
|
* @param response |
||||||
|
*/ |
||||||
|
private void saveOperMaintenanceTaskEntity(OperMaintenanceTaskEntity entity, ProcessWorkFlowResponse response) { |
||||||
|
String flowDescription = ""; |
||||||
|
//如果response.getHandleType() 是0使用户
|
||||||
|
entity.setFlowUserId(response.getUserId()); |
||||||
|
entity.setNextStepOperator(response.getNextStepOperator()); |
||||||
|
flowDescription = "审批中,当前环节是".concat(response.getTaskName()).concat(",待").concat(response.getNextStepOperator()).concat("审批"); |
||||||
|
//如果taskId为空
|
||||||
|
String taskId = response.getTaskId(); |
||||||
|
if (StringUtils.isEmpty(taskId)) { |
||||||
|
entity.setFlowDescription("结束"); |
||||||
|
entity.setFlowTaskId(" "); |
||||||
|
entity.setFlowTaskName("结束"); |
||||||
|
entity.setNextStepOperator(" "); |
||||||
|
entity.setFlowStatus(999); |
||||||
|
} else { |
||||||
|
entity.setFlowTaskId(taskId); |
||||||
|
entity.setFlowTaskName(response.getTaskName()); |
||||||
|
entity.setFlowDescription(flowDescription); |
||||||
|
} |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 通过计划生成任务 |
||||||
|
* |
||||||
|
* @param finalPlanEntity |
||||||
|
*/ |
||||||
|
private void createTaskByPlan(OperMaintenancePlanEntity finalPlanEntity) { |
||||||
|
OperMaintenanceTaskEntity taskEntity = BeanUtil.copy(finalPlanEntity, OperMaintenanceTaskEntity.class); |
||||||
|
//拆分设备 每个设备生成一条任务
|
||||||
|
if (StringUtil.isNotBlank(finalPlanEntity.getEmCode())) { |
||||||
|
log.info("finalPlanEntity.getEmCode() : {}", finalPlanEntity.getEmCode()); |
||||||
|
List<String> emCodeList = Arrays.asList(finalPlanEntity.getEmCode().split(",")); |
||||||
|
for (String emCode : emCodeList) { |
||||||
|
taskEntity.setEmCode(emCode); |
||||||
|
this.fillTask(taskEntity, finalPlanEntity); |
||||||
|
} |
||||||
|
} else { |
||||||
|
this.fillTask(taskEntity, finalPlanEntity); |
||||||
|
} |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 补充日常维护任务内容 |
||||||
|
* |
||||||
|
* @param taskEntity |
||||||
|
* @param finalPlanEntity |
||||||
|
*/ |
||||||
|
private void fillTask(OperMaintenanceTaskEntity taskEntity, OperMaintenancePlanEntity finalPlanEntity) { |
||||||
|
taskEntity.setId(null); |
||||||
|
taskEntity.setCreateTime(new Date()); |
||||||
|
taskEntity.setUpdateTime(new Date()); |
||||||
|
taskEntity.setPlanId(finalPlanEntity.getId()); |
||||||
|
taskEntity.setTaskCode(UUID.randomUUID().toString()); |
||||||
|
if (taskEntity.getRefLibraryId() != 0) { |
||||||
|
taskEntity.setCreateTime(org.springblade.core.tool.utils.DateUtil.now()); |
||||||
|
} |
||||||
|
taskEntity.setDisposer(StringObjUtils.toString(finalPlanEntity.getDisposer())); |
||||||
|
//补充计划结束时间
|
||||||
|
Instant instant = taskEntity.getDisposeTime().toInstant(); |
||||||
|
ZoneId zoneId = ZoneId.systemDefault(); |
||||||
|
LocalDateTime disposeTime = instant.atZone(zoneId).toLocalDateTime(); |
||||||
|
taskEntity.setPlanEndTime(disposeTime.plusHours(taskEntity.getHours())); |
||||||
|
//获取值班人员
|
||||||
|
if (ObjectUtil.isNotEmpty(finalPlanEntity.getMaintenanceModel()) && finalPlanEntity.getMaintenanceModel() == 2) { |
||||||
|
//查询值班信息
|
||||||
|
LambdaQueryWrapper<ImsDutyMainEntity> entityLambdaQueryWrapper = new LambdaQueryWrapper<>(); |
||||||
|
entityLambdaQueryWrapper.eq(ImsDutyMainEntity::getDutyDate, |
||||||
|
DateTimeFormatter.ofPattern(PATTERN_DATE).format(disposeTime)); |
||||||
|
entityLambdaQueryWrapper.eq(ImsDutyMainEntity::getClassId, finalPlanEntity.getImsDutyClassId()); |
||||||
|
ImsDutyMainEntity entity = mainService.getOne(entityLambdaQueryWrapper); |
||||||
|
if (ObjectUtil.isNotEmpty(entity) && StringUtils.isNotEmpty(entity.getDutyPersonIds())) { |
||||||
|
String userId = entity.getDutyPersonIds(); |
||||||
|
taskEntity.setDisposer(userId.replaceAll("\\^", ",")); |
||||||
|
} else { |
||||||
|
log.info("计划ID为:{}未查询到排班记录", finalPlanEntity.getId()); |
||||||
|
return; |
||||||
|
} |
||||||
|
} |
||||||
|
taskService.save(taskEntity); |
||||||
|
//生成工作流实例
|
||||||
|
String processInstanceId = this.startProcess(finalPlanEntity.getProcDefId(), taskEntity); |
||||||
|
taskEntity.setProcessInstanceId(processInstanceId); |
||||||
|
taskService.updateById(taskEntity); |
||||||
|
finalPlanEntity.setCreateTaskTime(new Date()); |
||||||
|
maintenancePlanService.updateById(finalPlanEntity); |
||||||
|
|
||||||
|
taskEntity = taskService.getById(taskEntity.getId()); |
||||||
|
//推送消息
|
||||||
|
this.pushTaskMessage(taskEntity); |
||||||
|
|
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 开启一个流程实例 |
||||||
|
* |
||||||
|
* @author xiashandong |
||||||
|
**/ |
||||||
|
private String startProcess(String processDefinitionKey, OperMaintenanceTaskEntity taskEntity) { |
||||||
|
OperMaintenanceTaskEntityVo operMaintenanceTaskEntityVo = new OperMaintenanceTaskEntityVo(); |
||||||
|
BeanUtils.copyProperties(taskEntity,operMaintenanceTaskEntityVo); |
||||||
|
Map<String,Object> params = new HashMap<>(); |
||||||
|
params.put("taskId", taskEntity.getId()); |
||||||
|
List<String> userIdList = Stream.of(taskEntity.getDisposer().split(",")).collect(Collectors.toList()); |
||||||
|
String taskUsers = userIdList.stream().filter(o -> StringUtils.isNotBlank(o)).map(s -> { |
||||||
|
return "taskUser_".concat(s); |
||||||
|
}).collect(Collectors.joining(",")); |
||||||
|
params.put("initUserIds", taskUsers); |
||||||
|
params.put("operMaintenanceTaskEntityVo", operMaintenanceTaskEntityVo); |
||||||
|
|
||||||
|
return flowClient.startProcessInstanceContainNameByKey(processDefinitionKey, |
||||||
|
String.valueOf(taskEntity.getId()),taskEntity.getTitle(), params) |
||||||
|
.getData() |
||||||
|
.getProcessInstanceId(); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 推送日常维护任务消息 |
||||||
|
* @return void |
||||||
|
* @Param taskEntity 日常维护任务 |
||||||
|
**/ |
||||||
|
private void pushTaskMessage(OperMaintenanceTaskEntity taskEntity) { |
||||||
|
log.info("开始保存{}的日常维护消息推送记录", taskEntity.getDisposer()); |
||||||
|
R<MessageTemplateEntity> templateR = messageClient.getMsgTemplateById(taskEntity.getMessageTemplateId()); |
||||||
|
if (templateR.isSuccess() && ObjectUtil.isNotEmpty(templateR.getData())) { |
||||||
|
Func.toLongList(",", taskEntity.getDisposer()).forEach(userId -> { |
||||||
|
//推送消息
|
||||||
|
ZoneId zoneId = ZoneId.systemDefault(); |
||||||
|
PlanMsgRecordDto planMsgRecordDto = new PlanMsgRecordDto(); |
||||||
|
planMsgRecordDto.setTemplateId(taskEntity.getMessageTemplateId()); |
||||||
|
planMsgRecordDto.setCreateUser(taskEntity.getCreateUser()); |
||||||
|
planMsgRecordDto.setPlanTime(LocalDateTime.ofInstant(taskEntity.getDisposeTime().toInstant(), zoneId)); |
||||||
|
planMsgRecordDto.setTaskId(taskEntity.getId()); |
||||||
|
planMsgRecordDto.setDeptId(taskEntity.getCreateDept()); |
||||||
|
planMsgRecordDto.setReceiver(userId); |
||||||
|
TemplateService templateService1 = templateService.stream().filter(item -> item.isTemplateStatus(1)).findFirst().orElse(null); |
||||||
|
templateService1.execute(planMsgRecordDto); |
||||||
|
log.info("推送内容体为:{}", JSON.toJSONString(taskEntity.getDisposer())); |
||||||
|
}); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,222 @@ |
|||||||
|
package com.hnac.hzims.operational.maintenance.vo; |
||||||
|
|
||||||
|
import com.fasterxml.jackson.annotation.JsonFormat; |
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import org.springblade.core.tool.utils.DateUtil; |
||||||
|
import org.springframework.format.annotation.DateTimeFormat; |
||||||
|
|
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
/** |
||||||
|
* 日常维护对象 |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/13 17:20 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
public class OperMaintenanceTaskEntityVo { |
||||||
|
|
||||||
|
|
||||||
|
private static final long serialVersionUID = 1L; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 主键id |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "主键id") |
||||||
|
private Long id; |
||||||
|
|
||||||
|
/** |
||||||
|
* 创建人 |
||||||
|
*/ |
||||||
|
private Long createUser; |
||||||
|
|
||||||
|
/** |
||||||
|
* 创建部门 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "创建部门") |
||||||
|
private Long createDept; |
||||||
|
|
||||||
|
/** |
||||||
|
* 创建时间 |
||||||
|
*/ |
||||||
|
@DateTimeFormat(pattern = DateUtil.PATTERN_DATETIME) |
||||||
|
@JsonFormat(pattern = DateUtil.PATTERN_DATETIME) |
||||||
|
@ApiModelProperty(value = "创建时间") |
||||||
|
private Date createTime; |
||||||
|
|
||||||
|
/** |
||||||
|
* 更新人 |
||||||
|
*/ |
||||||
|
private Long updateUser; |
||||||
|
|
||||||
|
/** |
||||||
|
* 更新时间 |
||||||
|
*/ |
||||||
|
@DateTimeFormat(pattern = DateUtil.PATTERN_DATETIME) |
||||||
|
@JsonFormat(pattern = DateUtil.PATTERN_DATETIME) |
||||||
|
@ApiModelProperty(value = "更新时间") |
||||||
|
private Date updateTime; |
||||||
|
|
||||||
|
/** |
||||||
|
* 状态[1:正常] |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "业务状态") |
||||||
|
private Integer status; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 设备编码 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "设备编码") |
||||||
|
private String emCode; |
||||||
|
/** |
||||||
|
* 维护类型 01=定期试验 02=定期保养 03=定期操作 04=其他 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "维护类型 01=定期试验 02=定期保养 03=定期操作 04=其他") |
||||||
|
private String typeCode; |
||||||
|
/** |
||||||
|
* 任务编码 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "任务编码,后台自动生成") |
||||||
|
private String taskCode; |
||||||
|
/** |
||||||
|
* 维护项目 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "维护项目") |
||||||
|
private Long refLibraryId; |
||||||
|
|
||||||
|
/** |
||||||
|
* 维护标题 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "维护标题") |
||||||
|
private String title; |
||||||
|
/** |
||||||
|
* 工作流实例ID |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "工作流实例ID") |
||||||
|
private String processInstanceId; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 执行人 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "执行人") |
||||||
|
private String disposer; |
||||||
|
/** |
||||||
|
* 执行日期 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "任务按周期生成计划时间") |
||||||
|
private Date disposeTime; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "执行时间") |
||||||
|
private LocalDateTime executeTime; |
||||||
|
/** |
||||||
|
* 标准工时 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "标准工时") |
||||||
|
private Integer hours; |
||||||
|
/** |
||||||
|
* 实际工时 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "实际工时") |
||||||
|
private Integer actHours; |
||||||
|
/** |
||||||
|
* 维护内容 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "维护内容") |
||||||
|
private String content; |
||||||
|
/** |
||||||
|
* 操作规范 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "操作规范") |
||||||
|
private String workStandards; |
||||||
|
/** |
||||||
|
* 安全警告 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "安全警告") |
||||||
|
private String safo; |
||||||
|
/** |
||||||
|
* 是否异常 0=否 1=是 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "是否异常 0=否 1=是") |
||||||
|
private Integer isAnomaly; |
||||||
|
/** |
||||||
|
* 遗留问题 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "遗留问题") |
||||||
|
private String legacy; |
||||||
|
/** |
||||||
|
* 问题描述 |
||||||
|
*/ |
||||||
|
@ApiModelProperty(value = "问题描述") |
||||||
|
private String description; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "任务类型") |
||||||
|
private String taskType; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "票据ID") |
||||||
|
private Long ticketId; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "延期状态") |
||||||
|
private Integer delayStatus; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "延期时间") |
||||||
|
private LocalDateTime delayDate; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "计划结束时间") |
||||||
|
@DateTimeFormat(pattern = DateUtil.PATTERN_DATETIME) |
||||||
|
@JsonFormat(pattern = DateUtil.PATTERN_DATETIME) |
||||||
|
private LocalDateTime planEndTime; |
||||||
|
|
||||||
|
@ApiModelProperty("计划ID") |
||||||
|
private Long planId; |
||||||
|
|
||||||
|
@ApiModelProperty(value = "消息推送模板ID") |
||||||
|
private Long messageTemplateId; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 流程状态' |
||||||
|
*/ |
||||||
|
@ApiModelProperty("流程状态") |
||||||
|
private Integer flowStatus; |
||||||
|
|
||||||
|
/** |
||||||
|
* 流程描述 |
||||||
|
*/ |
||||||
|
@ApiModelProperty("流程描述") |
||||||
|
private String flowDescription; |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 下一个审批人 |
||||||
|
*/ |
||||||
|
@ApiModelProperty("下一个审批人") |
||||||
|
private String nextStepOperator; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 用户Id |
||||||
|
*/ |
||||||
|
@ApiModelProperty("用户Id") |
||||||
|
private String flowUserId; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 当前任务名 |
||||||
|
*/ |
||||||
|
@ApiModelProperty("当前任务名") |
||||||
|
private String flowTaskName; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 当前任务名 |
||||||
|
*/ |
||||||
|
@ApiModelProperty("当前任务名") |
||||||
|
private String flowTaskId; |
||||||
|
} |
@ -0,0 +1,23 @@ |
|||||||
|
package com.hnac.hzims.operational.util; |
||||||
|
|
||||||
|
import org.apache.commons.lang3.StringUtils; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/6/14 9:24 |
||||||
|
*/ |
||||||
|
public class StringObjUtils extends StringUtils { |
||||||
|
|
||||||
|
/** |
||||||
|
* object转Str |
||||||
|
* |
||||||
|
* @param obj |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
public static String toString(Object obj) { |
||||||
|
return obj == null ? (String) obj : obj.toString(); |
||||||
|
} |
||||||
|
|
||||||
|
} |
@ -0,0 +1,65 @@ |
|||||||
|
package com.hnac.hzims.ticket.processflow.strategy.serviceimpl; |
||||||
|
|
||||||
|
import com.hnac.hzims.operational.maintenance.feign.MaintenanceFeignClient; |
||||||
|
import com.hnac.hzims.ticket.processflow.service.ProcessDictService; |
||||||
|
import com.hnac.hzims.ticket.processflow.strategy.abstracts.ProcessAbstractService; |
||||||
|
import com.hnac.hzims.ticket.processflow.strategy.entity.WorkflowQueue; |
||||||
|
import com.hnac.hzims.operational.maintenance.vo.ProcessWorkFlowResponse; |
||||||
|
import lombok.RequiredArgsConstructor; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springblade.core.log.exception.ServiceException; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
import static com.hnac.hzims.ticket.processflow.constant.TicketProcessConstant.MAINTENANCE_KEY; |
||||||
|
|
||||||
|
/** |
||||||
|
* 日常维护实现类 |
||||||
|
* |
||||||
|
* @Author dfy |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/3/28 8:47 |
||||||
|
*/ |
||||||
|
@Slf4j |
||||||
|
@Service |
||||||
|
@RequiredArgsConstructor |
||||||
|
public class MaintenanceProcessServiceImpl extends ProcessAbstractService { |
||||||
|
|
||||||
|
|
||||||
|
private final MaintenanceFeignClient maintenanceFeignClient; |
||||||
|
|
||||||
|
|
||||||
|
private final ProcessDictService processDictService; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 设置执行那种实现类 |
||||||
|
* |
||||||
|
* @param flowQueue |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public Boolean isWorkflowProcess(WorkflowQueue flowQueue) { |
||||||
|
log.info("是否执日常维护流程环节操作~~~~,流程ticket: {}", flowQueue); |
||||||
|
String dictValue = processDictService.selectDictValueByKey(MAINTENANCE_KEY); |
||||||
|
if (dictValue.equals(flowQueue.getProcessDefinitionKey())) { |
||||||
|
log.info("已执行日常维护流程环节操作~~~~"); |
||||||
|
return true; |
||||||
|
} |
||||||
|
log.error("未是否执行日常维护流程环节操作,请联系管理员~~~~"); |
||||||
|
return false; |
||||||
|
} |
||||||
|
|
||||||
|
/** |
||||||
|
* 日常维护业务方法 |
||||||
|
* |
||||||
|
* @param response |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public void calculate(ProcessWorkFlowResponse response) { |
||||||
|
Boolean pending = maintenanceFeignClient.findPending(response); |
||||||
|
if (!pending) { |
||||||
|
throw new ServiceException("日常维护业务流程出错呢"); |
||||||
|
} |
||||||
|
} |
||||||
|
} |
Loading…
Reference in new issue