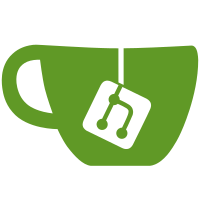
6 changed files with 51 additions and 84 deletions
@ -1,31 +1,51 @@
|
||||
package com.hnac.hzims.alarm.monitor.listener; |
||||
|
||||
import com.hnac.hzims.alarm.monitor.service.AlarmMessageSendService; |
||||
import com.alibaba.fastjson.JSONObject; |
||||
import com.hnac.hzims.alarm.entity.AlarmEntity; |
||||
import com.hnac.hzims.alarm.handle.service.MessageService; |
||||
import lombok.extern.slf4j.Slf4j; |
||||
import org.springblade.core.tool.utils.StringUtil; |
||||
import org.springblade.queue.annotation.RedisQueue; |
||||
import org.springblade.queue.consume.IQueueConsume; |
||||
import org.springframework.beans.factory.annotation.Autowired; |
||||
import org.springframework.data.redis.connection.Message; |
||||
import org.springframework.data.redis.connection.MessageListener; |
||||
import org.springframework.data.redis.core.RedisTemplate; |
||||
import org.springframework.data.redis.serializer.RedisSerializer; |
||||
import org.springframework.stereotype.Component; |
||||
import org.springframework.data.redis.core.StringRedisTemplate; |
||||
import org.springframework.stereotype.Service; |
||||
|
||||
/** |
||||
* @author ysj |
||||
* @version 4.0.0 |
||||
* @create 2023-11-09-14:04 |
||||
*/ |
||||
@Component |
||||
public class AlarmListener implements MessageListener { |
||||
@Service |
||||
@RedisQueue(topicName = "hzims:queue:alarm") |
||||
@Slf4j |
||||
public class AlarmListener implements IQueueConsume { |
||||
|
||||
@Autowired |
||||
private AlarmMessageSendService alarmMessageSendService; |
||||
private MessageService messageService; |
||||
|
||||
@Autowired |
||||
private RedisTemplate redisTemplate; |
||||
private StringRedisTemplate redisTemplate; |
||||
|
||||
@Override |
||||
public void onMessage(Message message, byte[] pattern) { |
||||
RedisSerializer<String> serializer = redisTemplate.getStringSerializer(); |
||||
String msg = serializer.deserialize(message.getBody()); |
||||
System.out.println("接收到的消息是:" + msg); |
||||
public void handlerMessage(String message) { |
||||
if(StringUtil.isEmpty(message)){ |
||||
return; |
||||
} |
||||
// 步骤1.消息对象转换
|
||||
AlarmEntity alarm = JSONObject.parseObject(message,AlarmEntity.class); |
||||
|
||||
// 步骤2.WEB/APP消息推送
|
||||
if(alarm.getIsPlatformMessage() == 0){ |
||||
messageService.webAppMessage(alarm); |
||||
} |
||||
|
||||
// 步骤3.短信推送
|
||||
if(alarm.getIsShortMessage() == 0){ |
||||
messageService.shortMessage(alarm); |
||||
} |
||||
|
||||
// 步骤4.微信公众号发送
|
||||
if(alarm.getIsWxMessage() == 0){ |
||||
messageService.weChatMessage(alarm); |
||||
} |
||||
} |
||||
} |
||||
} |
@ -1,44 +0,0 @@
|
||||
package com.hnac.hzims.alarm.monitor.listener; |
||||
|
||||
import com.hnac.hzims.common.config.RedisMessageListener; |
||||
import org.springframework.context.annotation.Bean; |
||||
import org.springframework.data.redis.connection.RedisConnectionFactory; |
||||
import org.springframework.data.redis.listener.PatternTopic; |
||||
import org.springframework.data.redis.listener.RedisMessageListenerContainer; |
||||
import org.springframework.data.redis.listener.adapter.MessageListenerAdapter; |
||||
|
||||
/** |
||||
* @author ysj |
||||
* @version 4.0.0 |
||||
* @create 2023-11-09 14:02 |
||||
*/ |
||||
public class QuqueConfig { |
||||
|
||||
/** |
||||
* 创建连接工厂 |
||||
* |
||||
* @param connectionFactory |
||||
* @param adapter |
||||
* @return |
||||
*/ |
||||
@Bean |
||||
public RedisMessageListenerContainer container(RedisConnectionFactory connectionFactory, |
||||
MessageListenerAdapter adapter) { |
||||
RedisMessageListenerContainer container = new RedisMessageListenerContainer(); |
||||
container.setConnectionFactory(connectionFactory); |
||||
//监听对应的channel
|
||||
container.addMessageListener(adapter, new PatternTopic("alarmchannel")); |
||||
return container; |
||||
} |
||||
|
||||
/** |
||||
* 绑定消息监听者和接收监听的方法 |
||||
* @param message |
||||
* @return |
||||
*/ |
||||
@Bean |
||||
public MessageListenerAdapter adapter(RedisMessageListener message) { |
||||
// onMessage 如果RedisMessage 中 没有实现接口,这个参数必须跟RedisMessage中的读取信息的方法名称一样
|
||||
return new MessageListenerAdapter(message, "onMessage"); |
||||
} |
||||
} |
Loading…
Reference in new issue