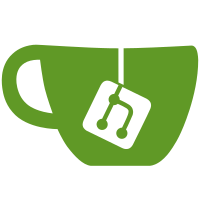
37 changed files with 1550 additions and 952 deletions
@ -0,0 +1,32 @@ |
|||||||
|
package com.hnac.hzims.ticket.areamonthly.feign; |
||||||
|
|
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.StandardTicketVo; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.feign.fallback.StandardTicketInfoFeignClient; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.vo.AreaMonthlyVo; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.cloud.openfeign.FeignClient; |
||||||
|
import org.springframework.cloud.openfeign.SpringQueryMap; |
||||||
|
import org.springframework.web.bind.annotation.*; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/4/10 13:39 |
||||||
|
*/ |
||||||
|
|
||||||
|
@FeignClient(value = "hzims-ticket", fallback = StandardTicketInfoFeignClient.class) |
||||||
|
public interface TicketFeignClient { |
||||||
|
|
||||||
|
/** |
||||||
|
* 根据月份,区域 获取操作详情数据 |
||||||
|
* |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@GetMapping("/standard/ticket/areaMonthlyWithOperation/{page}/{size}") |
||||||
|
R<IPage<StandardTicketVo>> areaMonthlyWithOperation(@PathVariable("page") Long page, |
||||||
|
@PathVariable("size") Long size, |
||||||
|
@SpringQueryMap AreaMonthlyVo areaMonthlyV); |
||||||
|
} |
@ -0,0 +1,30 @@ |
|||||||
|
package com.hnac.hzims.ticket.areamonthly.feign.fallback; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.StandardTicketVo; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.feign.TicketFeignClient; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.vo.AreaMonthlyVo; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/4/10 17:24 |
||||||
|
*/ |
||||||
|
@Service |
||||||
|
public class StandardTicketInfoFeignClient implements TicketFeignClient { |
||||||
|
/** |
||||||
|
* 根据月份,区域 获取操作详情数据 |
||||||
|
* |
||||||
|
* @param page |
||||||
|
* @param size |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public R<IPage<StandardTicketVo>> areaMonthlyWithOperation(Long page, Long size, AreaMonthlyVo areaMonthlyV) { |
||||||
|
|
||||||
|
return R.fail("远程调用失败"); |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,28 @@ |
|||||||
|
package com.hnac.hzims.ticket.areamonthly.vo; |
||||||
|
|
||||||
|
import lombok.Data; |
||||||
|
|
||||||
|
import java.time.YearMonth; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/4/10 11:28 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
public class AreaMonthlyVo { |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 区域编号 |
||||||
|
*/ |
||||||
|
private String areaId; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 月份 |
||||||
|
*/ |
||||||
|
private YearMonth YearMonth; |
||||||
|
|
||||||
|
} |
@ -0,0 +1,71 @@ |
|||||||
|
package com.hnac.hzims.ticket.areamonthly; |
||||||
|
|
||||||
|
import io.swagger.annotations.ApiModelProperty; |
||||||
|
import lombok.Data; |
||||||
|
import lombok.EqualsAndHashCode; |
||||||
|
import org.springblade.core.mp.support.QueryField; |
||||||
|
import org.springblade.core.mp.support.SqlCondition; |
||||||
|
|
||||||
|
import java.io.Serializable; |
||||||
|
import java.time.LocalDateTime; |
||||||
|
import java.util.Date; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/4/10 17:06 |
||||||
|
*/ |
||||||
|
@EqualsAndHashCode |
||||||
|
@Data |
||||||
|
public class StandardTicketVo implements Serializable { |
||||||
|
|
||||||
|
private static final long serialVersionUID = -8870464581684939369L; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 开票种类 |
||||||
|
*/ |
||||||
|
private String ticketType; |
||||||
|
|
||||||
|
/** |
||||||
|
* 单位 |
||||||
|
*/ |
||||||
|
private String company; |
||||||
|
/** |
||||||
|
* 编号 |
||||||
|
*/ |
||||||
|
private String code; |
||||||
|
/** |
||||||
|
* 开票来源 |
||||||
|
*/ |
||||||
|
private String taskType; |
||||||
|
/** |
||||||
|
* 开票任务来源 |
||||||
|
*/ |
||||||
|
private String taskName; |
||||||
|
|
||||||
|
/** |
||||||
|
* 发令人 |
||||||
|
*/ |
||||||
|
private Long issueOrderPerson; |
||||||
|
|
||||||
|
/** |
||||||
|
* 受令人 |
||||||
|
*/ |
||||||
|
private Long accessOrderPerson; |
||||||
|
/** |
||||||
|
* 监护人 |
||||||
|
*/ |
||||||
|
private String guardian; |
||||||
|
|
||||||
|
/** |
||||||
|
* 值班负责人 |
||||||
|
*/ |
||||||
|
private Long principal; |
||||||
|
/** |
||||||
|
* 开票时间 |
||||||
|
*/ |
||||||
|
private Date createTime; |
||||||
|
} |
||||||
|
|
@ -1,104 +1,104 @@ |
|||||||
package com.hnac.hzims.middle.mybatisplus; |
// package com.hnac.hzims.middle.mybatisplus;
|
||||||
|
//
|
||||||
import com.baomidou.mybatisplus.generator.AutoGenerator; |
// import com.baomidou.mybatisplus.generator.AutoGenerator;
|
||||||
import com.baomidou.mybatisplus.generator.InjectionConfig; |
// import com.baomidou.mybatisplus.generator.InjectionConfig;
|
||||||
import com.baomidou.mybatisplus.generator.config.*; |
// import com.baomidou.mybatisplus.generator.config.*;
|
||||||
import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy; |
// import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy;
|
||||||
|
//
|
||||||
import java.util.ArrayList; |
// import java.util.ArrayList;
|
||||||
import java.util.List; |
// import java.util.List;
|
||||||
|
//
|
||||||
/** |
// /**
|
||||||
* @Author WL |
// * @Author WL
|
||||||
* @Version v1.0 |
// * @Version v1.0
|
||||||
* @Serial 1.0 |
// * @Serial 1.0
|
||||||
* @Date 2023/4/4 9:07 |
// * @Date 2023/4/4 9:07
|
||||||
*/ |
// */
|
||||||
public class CodeGenerator { |
// public class CodeGenerator {
|
||||||
|
//
|
||||||
|
//
|
||||||
public static void main(String[] args) { |
// public static void main(String[] args) {
|
||||||
// 代码生成器
|
// // 代码生成器
|
||||||
AutoGenerator mpg = new AutoGenerator(); |
// AutoGenerator mpg = new AutoGenerator();
|
||||||
|
//
|
||||||
// 全局配置
|
// // 全局配置
|
||||||
GlobalConfig gc = new GlobalConfig(); |
// GlobalConfig gc = new GlobalConfig();
|
||||||
String projectPath = System.getProperty("user.dir") + "/hzims-service/hzims-middle"; |
// String projectPath = System.getProperty("user.dir") + "/hzims-service/hzims-middle";
|
||||||
gc.setOutputDir(projectPath + "/src/main/java"); |
// gc.setOutputDir(projectPath + "/src/main/java");
|
||||||
gc.setAuthor("dfy"); |
// gc.setAuthor("dfy");
|
||||||
gc.setOpen(false); |
// gc.setOpen(false);
|
||||||
// gc.setSwagger2(true); 实体属性 Swagger2 注解
|
// // gc.setSwagger2(true); 实体属性 Swagger2 注解
|
||||||
mpg.setGlobalConfig(gc); |
// mpg.setGlobalConfig(gc);
|
||||||
|
//
|
||||||
// 数据源配置
|
// // 数据源配置
|
||||||
DataSourceConfig dsc = new DataSourceConfig(); |
// DataSourceConfig dsc = new DataSourceConfig();
|
||||||
dsc.setUrl("jdbc:mysql://192.168.1.20:3576/dev_hzims_middle?useSSL=false&useUnicode=true&characterEncoding=utf-8&zeroDateTimeBehavior=convertToNull&transformedBitIsBoolean=true&tinyInt1isBit=false&allowMultiQueries=true&serverTimezone=GMT%2B8&nullCatalogMeansCurrent=true&allowPublicKeyRetrieval=true"); |
// dsc.setUrl("jdbc:mysql://192.168.1.20:3576/dev_hzims_middle?useSSL=false&useUnicode=true&characterEncoding=utf-8&zeroDateTimeBehavior=convertToNull&transformedBitIsBoolean=true&tinyInt1isBit=false&allowMultiQueries=true&serverTimezone=GMT%2B8&nullCatalogMeansCurrent=true&allowPublicKeyRetrieval=true");
|
||||||
// dsc.setSchemaName("public");
|
// // dsc.setSchemaName("public");
|
||||||
dsc.setDriverName("com.mysql.cj.jdbc.Driver"); |
// dsc.setDriverName("com.mysql.cj.jdbc.Driver");
|
||||||
dsc.setUsername("root"); |
// dsc.setUsername("root");
|
||||||
dsc.setPassword("123"); |
// dsc.setPassword("123");
|
||||||
mpg.setDataSource(dsc); |
// mpg.setDataSource(dsc);
|
||||||
|
//
|
||||||
// 包配置
|
// // 包配置
|
||||||
PackageConfig pc = new PackageConfig(); |
// PackageConfig pc = new PackageConfig();
|
||||||
pc.setModuleName("systemlog"); |
// pc.setModuleName("systemlog");
|
||||||
pc.setParent("com.hnac.hzims.middle"); |
// pc.setParent("com.hnac.hzims.middle");
|
||||||
mpg.setPackageInfo(pc); |
// mpg.setPackageInfo(pc);
|
||||||
|
//
|
||||||
// 自定义配置
|
// // 自定义配置
|
||||||
InjectionConfig cfg = new InjectionConfig() { |
// InjectionConfig cfg = new InjectionConfig() {
|
||||||
@Override |
// @Override
|
||||||
public void initMap() { |
// public void initMap() {
|
||||||
// to do nothing
|
// // to do nothing
|
||||||
} |
// }
|
||||||
}; |
// };
|
||||||
|
//
|
||||||
// 如果模板引擎是 freemarker
|
// // 如果模板引擎是 freemarker
|
||||||
String templatePath = "/templates/mapper.xml.ftl"; |
// String templatePath = "/templates/mapper.xml.ftl";
|
||||||
// 如果模板引擎是 velocity
|
// // 如果模板引擎是 velocity
|
||||||
// String templatePath = "/templates/mapper.xml.vm";
|
// // String templatePath = "/templates/mapper.xml.vm";
|
||||||
|
//
|
||||||
// 自定义输出配置
|
// // 自定义输出配置
|
||||||
List<FileOutConfig> focList = new ArrayList<>(); |
// List<FileOutConfig> focList = new ArrayList<>();
|
||||||
cfg.setFileOutConfigList(focList); |
// cfg.setFileOutConfigList(focList);
|
||||||
mpg.setCfg(cfg); |
// mpg.setCfg(cfg);
|
||||||
|
//
|
||||||
// 配置模板
|
// // 配置模板
|
||||||
TemplateConfig templateConfig = new TemplateConfig(); |
// TemplateConfig templateConfig = new TemplateConfig();
|
||||||
|
//
|
||||||
// 配置自定义输出模板
|
// // 配置自定义输出模板
|
||||||
//指定自定义模板路径,注意不要带上.ftl/.vm, 会根据使用的模板引擎自动识别
|
// //指定自定义模板路径,注意不要带上.ftl/.vm, 会根据使用的模板引擎自动识别
|
||||||
// templateConfig.setEntity("templates/entity2.java");
|
// // templateConfig.setEntity("templates/entity2.java");
|
||||||
// templateConfig.setService();
|
// // templateConfig.setService();
|
||||||
// templateConfig.setController();
|
// // templateConfig.setController();
|
||||||
|
//
|
||||||
// templateConfig.setXml(null);
|
// // templateConfig.setXml(null);
|
||||||
mpg.setTemplate(templateConfig); |
// mpg.setTemplate(templateConfig);
|
||||||
|
//
|
||||||
// 策略配置
|
// // 策略配置
|
||||||
StrategyConfig strategy = new StrategyConfig(); |
// StrategyConfig strategy = new StrategyConfig();
|
||||||
strategy.setNaming(NamingStrategy.underline_to_camel); |
// strategy.setNaming(NamingStrategy.underline_to_camel);
|
||||||
strategy.setColumnNaming(NamingStrategy.underline_to_camel); |
// strategy.setColumnNaming(NamingStrategy.underline_to_camel);
|
||||||
// strategy.setSuperEntityClass("你自己的父类实体,没有就不用设置!");
|
// // strategy.setSuperEntityClass("你自己的父类实体,没有就不用设置!");
|
||||||
strategy.setEntityLombokModel(true); |
// strategy.setEntityLombokModel(true);
|
||||||
strategy.setRestControllerStyle(true); |
// strategy.setRestControllerStyle(true);
|
||||||
strategy.setEntityBooleanColumnRemoveIsPrefix(true); |
// strategy.setEntityBooleanColumnRemoveIsPrefix(true);
|
||||||
strategy.setEntityLombokModel(true); |
// strategy.setEntityLombokModel(true);
|
||||||
strategy.setEntitySerialVersionUID(true); |
// strategy.setEntitySerialVersionUID(true);
|
||||||
strategy.setChainModel(true); |
// strategy.setChainModel(true);
|
||||||
strategy.setEntityTableFieldAnnotationEnable(true); |
// strategy.setEntityTableFieldAnnotationEnable(true);
|
||||||
// strategy.setFieldPrefix("hzims_");
|
// // strategy.setFieldPrefix("hzims_");
|
||||||
// 公共父类
|
// // 公共父类
|
||||||
// strategy.setSuperControllerClass("你自己的父类控制器,没有就不用设置!");
|
// // strategy.setSuperControllerClass("你自己的父类控制器,没有就不用设置!");
|
||||||
// 写于父类中的公共字段
|
// // 写于父类中的公共字段
|
||||||
// strategy.setSuperEntityColumns("id");
|
// // strategy.setSuperEntityColumns("id");
|
||||||
strategy.setInclude("hzims_statistics"); |
// strategy.setInclude("hzims_statistics");
|
||||||
strategy.setControllerMappingHyphenStyle(true); |
// strategy.setControllerMappingHyphenStyle(true);
|
||||||
strategy.setTablePrefix("hzims_"); |
// strategy.setTablePrefix("hzims_");
|
||||||
mpg.setStrategy(strategy); |
// mpg.setStrategy(strategy);
|
||||||
// mpg.setTemplateEngine(new FreemarkerTemplateEngine());
|
// // mpg.setTemplateEngine(new FreemarkerTemplateEngine());
|
||||||
mpg.execute(); |
// mpg.execute();
|
||||||
} |
// }
|
||||||
|
//
|
||||||
} |
// }
|
||||||
|
//
|
||||||
|
@ -0,0 +1,13 @@ |
|||||||
|
<?xml version="1.0" encoding="UTF-8"?> |
||||||
|
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
||||||
|
<mapper namespace="com.hnac.hzims.middle.systemlog.mapper.SysLogMapper"> |
||||||
|
|
||||||
|
<select id="countStatistics" resultType="com.hnac.hzims.middle.systemlog.vo.SysLogStatisticsVo"> |
||||||
|
select date_format(operation_time, '%Y-%m-%d') operationTime, |
||||||
|
count(title) titleCount, |
||||||
|
count(module_name) moduleNameCount, |
||||||
|
count(path) pathCount |
||||||
|
from hzims_sys_log |
||||||
|
group by operationTime; |
||||||
|
</select> |
||||||
|
</mapper> |
@ -0,0 +1,36 @@ |
|||||||
|
package com.hnac.hzims.middle.systemlog.vo; |
||||||
|
|
||||||
|
import lombok.Data; |
||||||
|
|
||||||
|
import java.time.YearMonth; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/4/10 9:03 |
||||||
|
*/ |
||||||
|
@Data |
||||||
|
public class SysLogStatisticsVo { |
||||||
|
|
||||||
|
/** |
||||||
|
* 操作时间 |
||||||
|
*/ |
||||||
|
private String operationTime; |
||||||
|
|
||||||
|
/** |
||||||
|
* 操作模块统计 |
||||||
|
*/ |
||||||
|
private Integer titleCount; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 操作类型统计 |
||||||
|
*/ |
||||||
|
private Integer moduleNameCount; |
||||||
|
|
||||||
|
/** |
||||||
|
* 根据路径统计 |
||||||
|
*/ |
||||||
|
private Integer pathCount; |
||||||
|
} |
@ -1,5 +0,0 @@ |
|||||||
<?xml version="1.0" encoding="UTF-8"?> |
|
||||||
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd"> |
|
||||||
<mapper namespace="com.hnac.hzims.middle.systemlog.mapper.SysLogMapper"> |
|
||||||
|
|
||||||
</mapper> |
|
@ -0,0 +1,33 @@ |
|||||||
|
package com.hnac.hzims.operational.main.config; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.baomidou.mybatisplus.extension.plugins.pagination.Page; |
||||||
|
import com.fasterxml.jackson.core.JacksonException; |
||||||
|
import com.fasterxml.jackson.core.JsonParser; |
||||||
|
import com.fasterxml.jackson.databind.DeserializationContext; |
||||||
|
import com.fasterxml.jackson.databind.JsonNode; |
||||||
|
import com.fasterxml.jackson.databind.ObjectMapper; |
||||||
|
import com.fasterxml.jackson.databind.deser.std.StdDeserializer; |
||||||
|
|
||||||
|
import java.io.IOException; |
||||||
|
|
||||||
|
/** |
||||||
|
* @Author WL |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/4/10 16:12 |
||||||
|
*/ |
||||||
|
public class IPageDeserializer extends StdDeserializer<IPage> { |
||||||
|
protected IPageDeserializer(Class<?> vc) { |
||||||
|
super(vc); |
||||||
|
} |
||||||
|
|
||||||
|
@Override |
||||||
|
public IPage deserialize(JsonParser jsonParser, DeserializationContext deserializationContext) throws IOException, JacksonException { |
||||||
|
JsonNode node = jsonParser.getCodec().readTree(jsonParser); |
||||||
|
String s = node.toString(); |
||||||
|
ObjectMapper om = new ObjectMapper(); |
||||||
|
Page page = om.readValue(s,Page.class); |
||||||
|
return page; |
||||||
|
} |
||||||
|
} |
@ -0,0 +1,52 @@ |
|||||||
|
package com.hnac.hzims.operational.main.config; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.fasterxml.jackson.databind.DeserializationFeature; |
||||||
|
import com.fasterxml.jackson.databind.ObjectMapper; |
||||||
|
import com.fasterxml.jackson.databind.module.SimpleModule; |
||||||
|
import org.springframework.context.annotation.Bean; |
||||||
|
import org.springframework.context.annotation.Configuration; |
||||||
|
import org.springframework.http.MediaType; |
||||||
|
import org.springframework.http.converter.HttpMessageConverter; |
||||||
|
import org.springframework.http.converter.json.MappingJackson2HttpMessageConverter; |
||||||
|
import org.springframework.web.servlet.config.annotation.WebMvcConfigurationSupport; |
||||||
|
|
||||||
|
import java.util.Arrays; |
||||||
|
import java.util.List; |
||||||
|
|
||||||
|
/** |
||||||
|
* |
||||||
|
* @Author dfy |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/4/10 16:11 |
||||||
|
*/ |
||||||
|
@Configuration |
||||||
|
public class WebDataConvertConfig extends WebMvcConfigurationSupport { |
||||||
|
|
||||||
|
|
||||||
|
@Override |
||||||
|
public void configureMessageConverters(List<HttpMessageConverter<?>> converters) { |
||||||
|
converters.add(mappingJackson2HttpMessageConverter()); |
||||||
|
super.configureMessageConverters(converters); |
||||||
|
} |
||||||
|
|
||||||
|
@Bean |
||||||
|
public MappingJackson2HttpMessageConverter mappingJackson2HttpMessageConverter() { |
||||||
|
MappingJackson2HttpMessageConverter converter = new MappingJackson2HttpMessageConverter(); |
||||||
|
ObjectMapper mapper = new ObjectMapper(); |
||||||
|
mapper.configure(DeserializationFeature.FAIL_ON_UNKNOWN_PROPERTIES, false); |
||||||
|
|
||||||
|
SimpleModule module = new SimpleModule(); |
||||||
|
module.addDeserializer(IPage.class, new IPageDeserializer(IPage.class)); |
||||||
|
mapper.registerModule(module); |
||||||
|
|
||||||
|
converter.setSupportedMediaTypes(Arrays.asList(MediaType.APPLICATION_JSON,MediaType.APPLICATION_JSON_UTF8,MediaType.APPLICATION_OCTET_STREAM)); |
||||||
|
converter.setObjectMapper(mapper); |
||||||
|
|
||||||
|
return converter; |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
} |
||||||
|
|
@ -0,0 +1,52 @@ |
|||||||
|
package com.hnac.hzims.operational.main.controller.web; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.hnac.hzims.operational.main.service.AreaMonthlyDetailsService; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.StandardTicketVo; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.vo.AreaMonthlyVo; |
||||||
|
import lombok.RequiredArgsConstructor; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.web.bind.annotation.GetMapping; |
||||||
|
import org.springframework.web.bind.annotation.PathVariable; |
||||||
|
import org.springframework.web.bind.annotation.RequestMapping; |
||||||
|
import org.springframework.web.bind.annotation.RestController; |
||||||
|
|
||||||
|
/** |
||||||
|
* 水电生成报告详情数据 |
||||||
|
* |
||||||
|
* @Author dfy |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/4/10 11:21 |
||||||
|
*/ |
||||||
|
@Slf4j |
||||||
|
@RequiredArgsConstructor |
||||||
|
@RestController |
||||||
|
@RequestMapping("/areaMonthlyDetails") |
||||||
|
public class AreaMonthlyDetailsController { |
||||||
|
|
||||||
|
|
||||||
|
private final AreaMonthlyDetailsService areaMonthlyDetailsService; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 操作票 根据月份,区域 获取操作详情数据 |
||||||
|
* |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@GetMapping("/areaMonthlyWithOperation/{page}/{size}") |
||||||
|
public R areaMonthlyWithOperation(@PathVariable("page") Long page, |
||||||
|
@PathVariable("size") Long size, |
||||||
|
AreaMonthlyVo areaMonthly) { |
||||||
|
IPage<StandardTicketVo> standardTicketInfoVoIPage = |
||||||
|
areaMonthlyDetailsService.areaMonthlyWithOperation(page,size, areaMonthly); |
||||||
|
return R.data(standardTicketInfoVoIPage); |
||||||
|
} |
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
|
||||||
|
} |
@ -0,0 +1,24 @@ |
|||||||
|
package com.hnac.hzims.operational.main.service; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.StandardTicketVo; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.vo.AreaMonthlyVo; |
||||||
|
|
||||||
|
/** |
||||||
|
* |
||||||
|
* 水电生产运行数据 |
||||||
|
* @Author dfy |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/4/10 13:46 |
||||||
|
*/ |
||||||
|
public interface AreaMonthlyDetailsService { |
||||||
|
/** |
||||||
|
* 根据月份,区域 获取操作详情数据 |
||||||
|
* @param page |
||||||
|
* @param size |
||||||
|
* @param areaMonthly |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
IPage<StandardTicketVo> areaMonthlyWithOperation(Long page, Long size, AreaMonthlyVo areaMonthly); |
||||||
|
} |
@ -0,0 +1,48 @@ |
|||||||
|
package com.hnac.hzims.operational.main.service.impl; |
||||||
|
|
||||||
|
import com.baomidou.mybatisplus.core.metadata.IPage; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.StandardTicketVo; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.feign.TicketFeignClient; |
||||||
|
import com.hnac.hzims.operational.main.service.AreaMonthlyDetailsService; |
||||||
|
import com.hnac.hzims.ticket.areamonthly.vo.AreaMonthlyVo; |
||||||
|
import lombok.RequiredArgsConstructor; |
||||||
|
import lombok.extern.slf4j.Slf4j; |
||||||
|
import org.springblade.core.tool.api.R; |
||||||
|
import org.springframework.stereotype.Service; |
||||||
|
|
||||||
|
/** |
||||||
|
* 水电生产运行数据 |
||||||
|
* |
||||||
|
* @Author dfy |
||||||
|
* @Version v1.0 |
||||||
|
* @Serial 1.0 |
||||||
|
* @Date 2023/4/10 13:46 |
||||||
|
*/ |
||||||
|
@Slf4j |
||||||
|
@RequiredArgsConstructor |
||||||
|
@Service |
||||||
|
public class AreaMonthlyDetailsServiceImpl implements AreaMonthlyDetailsService { |
||||||
|
|
||||||
|
private final TicketFeignClient ticketFeignClient; |
||||||
|
|
||||||
|
|
||||||
|
/** |
||||||
|
* 根据月份,区域 获取操作详情数据 |
||||||
|
* |
||||||
|
* @param page |
||||||
|
* @param size |
||||||
|
* @param areaMonthly |
||||||
|
* @return |
||||||
|
*/ |
||||||
|
@Override |
||||||
|
public IPage<StandardTicketVo> areaMonthlyWithOperation(Long page, Long size, AreaMonthlyVo areaMonthly) { |
||||||
|
R<IPage<StandardTicketVo>> pageR = |
||||||
|
ticketFeignClient.areaMonthlyWithOperation(page, size,areaMonthly); |
||||||
|
if (pageR.isSuccess()) { |
||||||
|
log.info("获取操作详情数据成功"); |
||||||
|
return pageR.getData(); |
||||||
|
} |
||||||
|
System.out.println(pageR.getMsg()); |
||||||
|
return null; |
||||||
|
} |
||||||
|
} |
@ -1,104 +1,104 @@ |
|||||||
package com.hnac.hzims.ticket.processflow; |
// package com.hnac.hzims.ticket.processflow;
|
||||||
|
//
|
||||||
import com.baomidou.mybatisplus.generator.AutoGenerator; |
// import com.baomidou.mybatisplus.generator.AutoGenerator;
|
||||||
import com.baomidou.mybatisplus.generator.InjectionConfig; |
// import com.baomidou.mybatisplus.generator.InjectionConfig;
|
||||||
import com.baomidou.mybatisplus.generator.config.*; |
// import com.baomidou.mybatisplus.generator.config.*;
|
||||||
import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy; |
// import com.baomidou.mybatisplus.generator.config.rules.NamingStrategy;
|
||||||
|
//
|
||||||
import java.util.ArrayList; |
// import java.util.ArrayList;
|
||||||
import java.util.List; |
// import java.util.List;
|
||||||
|
//
|
||||||
/** |
// /**
|
||||||
* 代码生成器 |
// * 代码生成器
|
||||||
* @Author dfy |
// * @Author dfy
|
||||||
* @Version v1.0 |
// * @Version v1.0
|
||||||
* @Serial 1.0 |
// * @Serial 1.0
|
||||||
* @Date 2023/3/29 8:59 |
// * @Date 2023/3/29 8:59
|
||||||
*/ |
// */
|
||||||
public class CodeGenerator { |
// public class CodeGenerator {
|
||||||
|
//
|
||||||
|
//
|
||||||
public static void main(String[] args) { |
// public static void main(String[] args) {
|
||||||
// 代码生成器
|
// // 代码生成器
|
||||||
AutoGenerator mpg = new AutoGenerator(); |
// AutoGenerator mpg = new AutoGenerator();
|
||||||
|
//
|
||||||
// 全局配置
|
// // 全局配置
|
||||||
GlobalConfig gc = new GlobalConfig(); |
// GlobalConfig gc = new GlobalConfig();
|
||||||
String projectPath = System.getProperty("user.dir") + "/hzims-service/ticket"; |
// String projectPath = System.getProperty("user.dir") + "/hzims-service/ticket";
|
||||||
gc.setOutputDir(projectPath + "/src/main/java"); |
// gc.setOutputDir(projectPath + "/src/main/java");
|
||||||
gc.setAuthor("dfy"); |
// gc.setAuthor("dfy");
|
||||||
gc.setOpen(false); |
// gc.setOpen(false);
|
||||||
// gc.setSwagger2(true); 实体属性 Swagger2 注解
|
// // gc.setSwagger2(true); 实体属性 Swagger2 注解
|
||||||
mpg.setGlobalConfig(gc); |
// mpg.setGlobalConfig(gc);
|
||||||
|
//
|
||||||
// 数据源配置
|
// // 数据源配置
|
||||||
DataSourceConfig dsc = new DataSourceConfig(); |
// DataSourceConfig dsc = new DataSourceConfig();
|
||||||
dsc.setUrl("jdbc:mysql://192.168.1.20:3576/dev_hzims_ticket?useSSL=false&useUnicode=true&characterEncoding=utf-8&zeroDateTimeBehavior=convertToNull&transformedBitIsBoolean=true&tinyInt1isBit=false&allowMultiQueries=true&serverTimezone=GMT%2B8&nullCatalogMeansCurrent=true&allowPublicKeyRetrieval=true"); |
// dsc.setUrl("jdbc:mysql://192.168.1.20:3576/dev_hzims_ticket?useSSL=false&useUnicode=true&characterEncoding=utf-8&zeroDateTimeBehavior=convertToNull&transformedBitIsBoolean=true&tinyInt1isBit=false&allowMultiQueries=true&serverTimezone=GMT%2B8&nullCatalogMeansCurrent=true&allowPublicKeyRetrieval=true");
|
||||||
// dsc.setSchemaName("public");
|
// // dsc.setSchemaName("public");
|
||||||
dsc.setDriverName("com.mysql.cj.jdbc.Driver"); |
// dsc.setDriverName("com.mysql.cj.jdbc.Driver");
|
||||||
dsc.setUsername("root"); |
// dsc.setUsername("root");
|
||||||
dsc.setPassword("123"); |
// dsc.setPassword("123");
|
||||||
mpg.setDataSource(dsc); |
// mpg.setDataSource(dsc);
|
||||||
|
//
|
||||||
// 包配置
|
// // 包配置
|
||||||
PackageConfig pc = new PackageConfig(); |
// PackageConfig pc = new PackageConfig();
|
||||||
pc.setModuleName("processflow"); |
// pc.setModuleName("processflow");
|
||||||
pc.setParent("com.hnac.hzims.ticket"); |
// pc.setParent("com.hnac.hzims.ticket");
|
||||||
mpg.setPackageInfo(pc); |
// mpg.setPackageInfo(pc);
|
||||||
|
//
|
||||||
// 自定义配置
|
// // 自定义配置
|
||||||
InjectionConfig cfg = new InjectionConfig() { |
// InjectionConfig cfg = new InjectionConfig() {
|
||||||
@Override |
// @Override
|
||||||
public void initMap() { |
// public void initMap() {
|
||||||
// to do nothing
|
// // to do nothing
|
||||||
} |
// }
|
||||||
}; |
// };
|
||||||
|
//
|
||||||
// 如果模板引擎是 freemarker
|
// // 如果模板引擎是 freemarker
|
||||||
String templatePath = "/templates/mapper.xml.ftl"; |
// String templatePath = "/templates/mapper.xml.ftl";
|
||||||
// 如果模板引擎是 velocity
|
// // 如果模板引擎是 velocity
|
||||||
// String templatePath = "/templates/mapper.xml.vm";
|
// // String templatePath = "/templates/mapper.xml.vm";
|
||||||
|
//
|
||||||
// 自定义输出配置
|
// // 自定义输出配置
|
||||||
List<FileOutConfig> focList = new ArrayList<>(); |
// List<FileOutConfig> focList = new ArrayList<>();
|
||||||
cfg.setFileOutConfigList(focList); |
// cfg.setFileOutConfigList(focList);
|
||||||
mpg.setCfg(cfg); |
// mpg.setCfg(cfg);
|
||||||
|
//
|
||||||
// 配置模板
|
// // 配置模板
|
||||||
TemplateConfig templateConfig = new TemplateConfig(); |
// TemplateConfig templateConfig = new TemplateConfig();
|
||||||
|
//
|
||||||
// 配置自定义输出模板
|
// // 配置自定义输出模板
|
||||||
//指定自定义模板路径,注意不要带上.ftl/.vm, 会根据使用的模板引擎自动识别
|
// //指定自定义模板路径,注意不要带上.ftl/.vm, 会根据使用的模板引擎自动识别
|
||||||
// templateConfig.setEntity("templates/entity2.java");
|
// // templateConfig.setEntity("templates/entity2.java");
|
||||||
// templateConfig.setService();
|
// // templateConfig.setService();
|
||||||
// templateConfig.setController();
|
// // templateConfig.setController();
|
||||||
|
//
|
||||||
// templateConfig.setXml(null);
|
// // templateConfig.setXml(null);
|
||||||
mpg.setTemplate(templateConfig); |
// mpg.setTemplate(templateConfig);
|
||||||
|
//
|
||||||
// 策略配置
|
// // 策略配置
|
||||||
StrategyConfig strategy = new StrategyConfig(); |
// StrategyConfig strategy = new StrategyConfig();
|
||||||
strategy.setNaming(NamingStrategy.underline_to_camel); |
// strategy.setNaming(NamingStrategy.underline_to_camel);
|
||||||
strategy.setColumnNaming(NamingStrategy.underline_to_camel); |
// strategy.setColumnNaming(NamingStrategy.underline_to_camel);
|
||||||
// strategy.setSuperEntityClass("你自己的父类实体,没有就不用设置!");
|
// // strategy.setSuperEntityClass("你自己的父类实体,没有就不用设置!");
|
||||||
strategy.setEntityLombokModel(true); |
// strategy.setEntityLombokModel(true);
|
||||||
strategy.setRestControllerStyle(true); |
// strategy.setRestControllerStyle(true);
|
||||||
strategy.setEntityBooleanColumnRemoveIsPrefix(true); |
// strategy.setEntityBooleanColumnRemoveIsPrefix(true);
|
||||||
strategy.setEntityLombokModel(true); |
// strategy.setEntityLombokModel(true);
|
||||||
strategy.setEntitySerialVersionUID(true); |
// strategy.setEntitySerialVersionUID(true);
|
||||||
strategy.setChainModel(true); |
// strategy.setChainModel(true);
|
||||||
strategy.setEntityTableFieldAnnotationEnable(true); |
// strategy.setEntityTableFieldAnnotationEnable(true);
|
||||||
// strategy.setFieldPrefix("hzims_");
|
// // strategy.setFieldPrefix("hzims_");
|
||||||
// 公共父类
|
// // 公共父类
|
||||||
// strategy.setSuperControllerClass("你自己的父类控制器,没有就不用设置!");
|
// // strategy.setSuperControllerClass("你自己的父类控制器,没有就不用设置!");
|
||||||
// 写于父类中的公共字段
|
// // 写于父类中的公共字段
|
||||||
// strategy.setSuperEntityColumns("id");
|
// // strategy.setSuperEntityColumns("id");
|
||||||
strategy.setInclude("hzims_process_type"); |
// strategy.setInclude("hzims_process_type");
|
||||||
strategy.setControllerMappingHyphenStyle(true); |
// strategy.setControllerMappingHyphenStyle(true);
|
||||||
strategy.setTablePrefix("hzims_"); |
// strategy.setTablePrefix("hzims_");
|
||||||
mpg.setStrategy(strategy); |
// mpg.setStrategy(strategy);
|
||||||
// mpg.setTemplateEngine(new FreemarkerTemplateEngine());
|
// // mpg.setTemplateEngine(new FreemarkerTemplateEngine());
|
||||||
mpg.execute(); |
// mpg.execute();
|
||||||
} |
// }
|
||||||
|
//
|
||||||
} |
// }
|
||||||
|
File diff suppressed because it is too large
Load Diff
Loading…
Reference in new issue